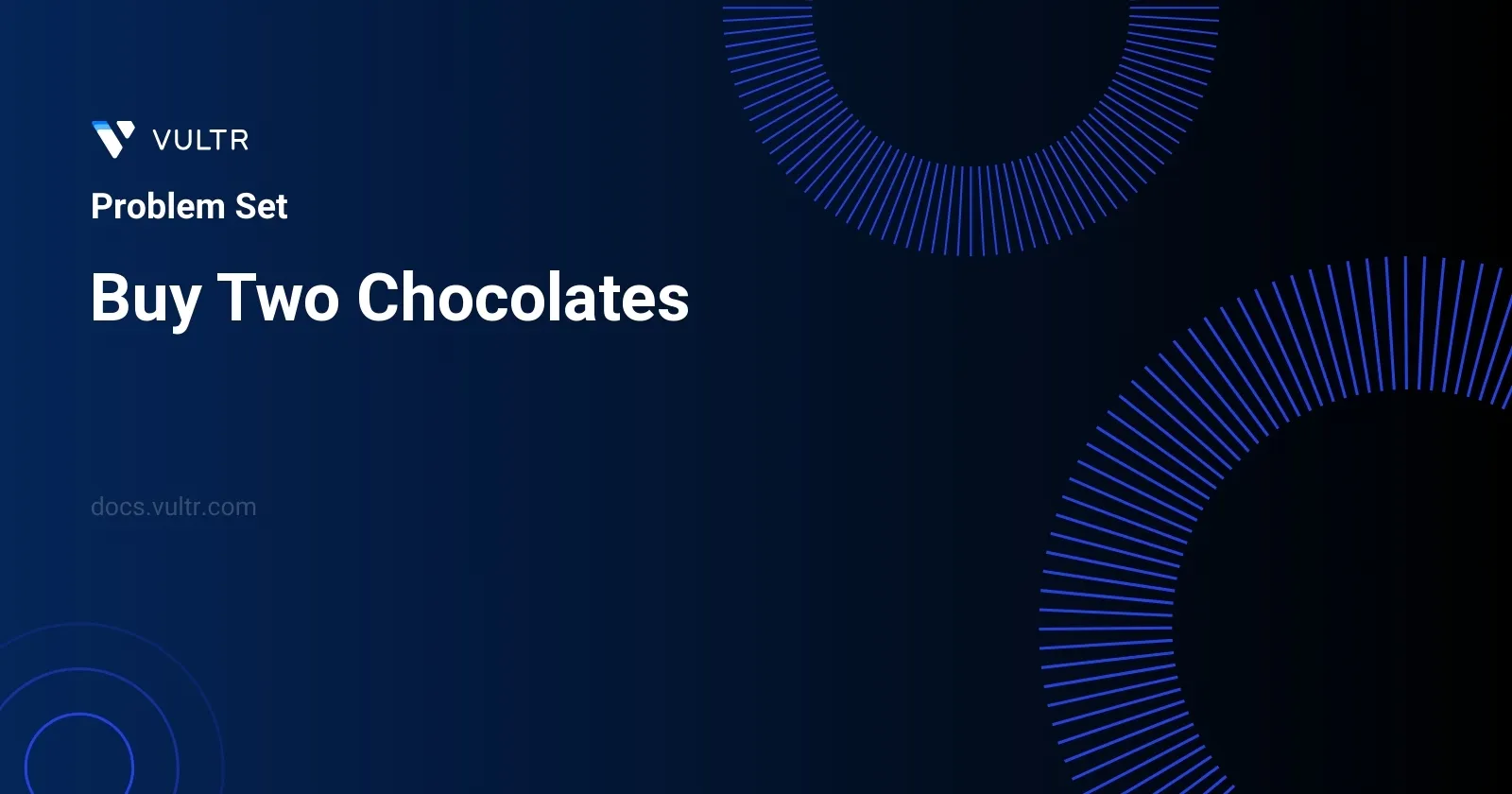
Problem Statement
In this problem, you are provided with an array of integers called prices
, representing the cost of different chocolates in a store. Alongside, you are given a single integer money
representing the total amount of money you have. The objective is to purchase exactly two chocolates from this list. However, the sum of the prices of these two chocolates should allow you to have some money left, specifically a non-negative amount. Your goal is to spend as much of your money as possible on these two chocolates without going into debt, thereby minimizing the leftover money after the purchase. If it's not possible to buy two chocolates without exceeding your budget, simply return the initial amount of money you have. The solution should effectively determine the optimal pair of chocolates to purchase, so that the leftover money is minimized.
Examples
Example 1
Input:
prices = [1,2,2], money = 3
Output:
0
Explanation:
Purchase the chocolates priced at 1 and 2 units respectively. You will have 3 - 3 = 0 units of money afterwards. Thus, we return 0.
Example 2
Input:
prices = [3,2,3], money = 3
Output:
3
Explanation:
You cannot buy 2 chocolates without going in debt, so we return 3.
Constraints
2 <= prices.length <= 50
1 <= prices[i] <= 100
1 <= money <= 100
Approach and Intuition
To solve this problem optimally, consider the following approach based on the given constraints:
- If there are only two chocolates in the list (
prices.length == 2
), simply sum their costs. If the sum is less than or equal tomoney
, return the remaining amount after the purchase. If greater, return the initial amount of money as no purchase is possible without debt. - For more chocolates, iterate through each possible combination of two distinct chocolates:
- For each combination (let’s say chocolates at
i
andj
withi < j
), calculate the combined cost. - If this combined cost is less than or equal to your
money
:- Check how much money will be leftover after this purchase.
- Keep track of the maximum amount spent in order to minimize the leftover money.
- If no valid pairs are found (all combinations exceed the budget), the returned result should be the original amount of
money
, indicating that no purchase is feasible.
- For each combination (let’s say chocolates at
This method ensures that you check all possible pairs and find the one that maximizes your expenditure without going into debt. In computational terms, this algorithm runs in O(n^2)
time complexity because every pair of chocolates is considered exactly once, where n
refers to the total number of chocolates, which is feasible given the constraint (maximum of 50 chocolates). This brute-force approach ensures that you find the solution by explicitly checking each viable pair.
Solutions
- C++
- Java
- JavaScript
- Python
class Solution {
public:
int purchaseChocolates(vector<int>& costList, int budget) {
int cheapest = min(costList[0], costList[1]);
int secondCheapest = max(costList[0], costList[1]);
for (int idx = 2; idx < costList.size(); idx++) {
if (costList[idx] < cheapest) {
secondCheapest = cheapest;
cheapest = costList[idx];
} else if (costList[idx] < secondCheapest) {
secondCheapest = costList[idx];
}
}
int totalMinimumCost = cheapest + secondCheapest;
if (totalMinimumCost <= budget) {
return budget - totalMinimumCost;
}
return budget;
}
};
This solution in C++ aims to help determine how many chocolates can be purchased within a given budget. The function purchaseChocolates
accepts two parameters: a vector costList
that stores the costs of available chocolates, and an integer budget
representing the total money available.
Follow these steps to understand the functioning of the code:
- Initialize two integers,
cheapest
andsecondCheapest
, to store the minimum and second minimum values from the first two elements of thecostList
. - Loop through the
costList
starting from the third element. - If the current chocolate cost is less than the
cheapest
, updatesecondCheapest
to becheapest
and then setcheapest
to the current cost. - If the current chocolate cost is less than
secondCheapest
but more thancheapest
, updatesecondCheapest
to the current cost. - Calculate the sum of
cheapest
andsecondCheapest
. - Check if this total is within the
budget
. - If the total cost is less than or equal to the budget, return the remaining budget after purchasing the two cheapest chocolates.
- If not, return the original budget indicating that it's not possible to buy two chocolates.
- Initialize two integers,
Key points:
- The function efficiently finds the two lowest costs using a single pass through the chocolate costs list.
- It ensures that even with the minimum cost chocolates, the purchase should not exceed the available budget.
- The solution returns how much budget remains after the potential purchase, or the full budget if a purchase isn't possible, which can help in further planning or decision-making.
class Solution {
public int purchaseChocolate(int[] costs, int budget) {
int least = Math.min(costs[0], costs[1]);
int secondLeast = Math.max(costs[0], costs[1]);
for (int i = 2; i < costs.length; i++) {
if (costs[i] < least) {
secondLeast = least;
least = costs[i];
} else if (costs[i] < secondLeast) {
secondLeast = costs[i];
}
}
int totalCost = least + secondLeast;
if (totalCost <= budget) {
return budget - totalCost;
}
return budget;
}
}
The Java code provided defines a method purchaseChocolate
in a class named Solution
that aims to determine how much budget remains after purchasing the two cheapest chocolates from a list of chocolate costs, given a specified budget.
Here's an overview of the steps involved:
- Initialize
least
andsecondLeast
with the first two elements of thecosts
array, ensuring they represent the minimum and second minimum values respectively. - Traverse through the chocolate costs array starting from the third element.
- Update the
least
andsecondLeast
variables appropriately; if a cost in the array is less thanleast
, update bothleast
andsecondLeast
. If it's only less thansecondLeast
, updatesecondLeast
. - Once all elements are checked, sum
least
andsecondLeast
to get the total cost of the two cheapest chocolates. - If this total cost is within the provided budget, subtract it from the budget to calculate the remaining amount.
- Return the remaining budget or, if the total cost exceeds the budget, return the original budget.
This algorithm efficiently finds the two lowest values in a single sweep of the array, ensuring that you can calculate how much budget is left after making the two cheapest purchases within the constraints.
var purchaseChocolate = function(costs, budget) {
// Initialize the lowest and second lowest cost values
let lowCost = Math.min(costs[0], costs[1]);
let secondLowCost = Math.max(costs[0], costs[1]);
// Determine the two lowest costs from the array
for (let j = 2; j < costs.length; j++) {
if (costs[j] < lowCost) {
secondLowCost = lowCost;
lowCost = costs[j];
} else if (costs[j] < secondLowCost) {
secondLowCost = costs[j];
}
}
// Calculate the combined lowest cost
let totalMinCost = lowCost + secondLowCost;
// Check financial capability to purchase
if (totalMinCost <= budget) {
return budget - totalMinCost; // Return remaining money after purchase
}
return budget; // Not enough funds, return original budget
};
The problem "Buy Two Chocolates" involves creating a JavaScript function that determines if a user can purchase the two least expensive chocolates from an array of costs, given a specific budget. The function should also calculate the remaining budget after the purchase if feasible.
- Start by identifying the two least expensive chocolates. Initialize two variables,
lowCost
andsecondLowCost
, with the smallest and second smallest values from the first two elements in the array. - Iterate over the remaining items in the
costs
array, updatinglowCost
andsecondLowCost
accordingly whenever a new lower or second-lower cost is found. - After determining the two lowest prices, sum them to get
totalMinCost
. - If
totalMinCost
is within the providedbudget
, calculate and return the remaining amount (budget - totalMinCost
) indicating the successful purchase. - If the combined price of the two chocolates exceeds the budget, simply return the original
budget
, indicating that the purchase is not possible without exceeding the budget limit.
This solution efficiently finds the two cheapest items and checks the feasibility of purchasing them within the budget, all while optimizing cost-related decisions.
class Solution:
def purchaseChocolate(self, costs: List[int], budget: int) -> int:
# Initialize with the first two costs assuming minimum and second minimum
small = min(costs[0], costs[1])
second_small = max(costs[0], costs[1])
# Loop through the prices from the third item
for cost in costs[2:]:
if cost < small:
second_small = small
small = cost
elif cost < second_small:
second_small = cost
# Calculate the combined cost of the two cheapest chocolates
total_min_cost = small + second_small
# Check if the total minimum cost is within the budget
if total_min_cost <= budget:
return budget - total_min_cost
# If not enough budget, return the original budget
return budget
This Python3 solution outlines a method for determining how much of a given budget remains after purchasing the two cheapest items from a list, specifically chocolates in this case. The method purchaseChocolate
accepts a list of integers, costs
, which represents the cost of each chocolate, and an integer, budget
, representing the buyer's total available funds.
The approach involves:
- Identifying the two least expensive chocolates.
- Ensuring their combined cost does not exceed the budget.
- Returning the remaining budget after these purchases.
Here's a step-by-step breakdown of the implementation:
- Initialize two variables
small
andsecond_small
to the cost of the first and second items respectively, determining which is less expensive. - Iterate through the remaining chocolates' costs.
- Update
small
andsecond_small
appropriately to always hold the lowest and the second lowest costs found so far. - Compute the total cost of the two cheapest chocolates.
- Check if the budget can cover this cost. If so, return the remaining budget after the purchase. If not, simply return the total budget, indicating no purchase was made.
This algorithm ensures you spend the least amount possible on the two chocolates, maximizing the remaining budget.
No comments yet.