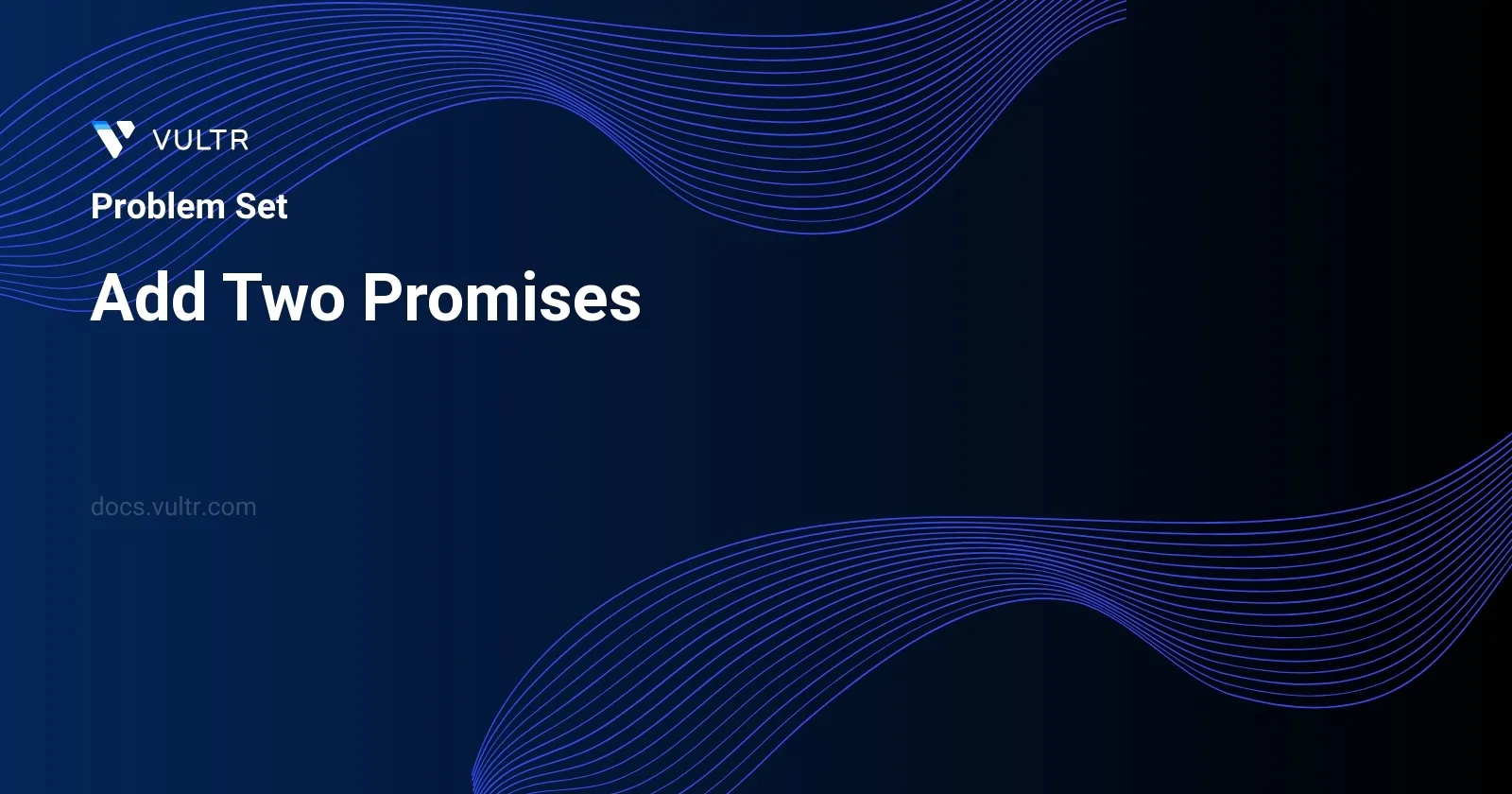
Problem Statement
In this task, you are provided with two promises: promise1
and promise2
. Each promise, when resolved, produces a numerical value. The core challenge is to craft a new promise which, upon resolution, provides the sum of the numbers obtained from the resolutions of promise1
and promise2
. This problem tests your ability to handle asynchronous JavaScript operations and combine their results effectively.
Examples
Example 1
Input:
promise1 = new Promise(resolve => setTimeout(() => resolve(2), 20)), promise2 = new Promise(resolve => setTimeout(() => resolve(5), 60))
Output:
7
Explanation:
The two input promises resolve with the values of 2 and 5 respectively. The returned promise should resolve with a value of 2 + 5 = 7. The time the returned promise resolves is not judged for this problem.
Example 2
Input:
promise1 = new Promise(resolve => setTimeout(() => resolve(10), 50)), promise2 = new Promise(resolve => setTimeout(() => resolve(-12), 30))
Output:
-2
Explanation:
The two input promises resolve with the values of 10 and -12 respectively. The returned promise should resolve with a value of 10 + -12 = -2.
Constraints
promise1
andpromise2
are promises that resolve with a number
Approach and Intuition
When approaching the problem of combining the results of two resolved promises, it's essential to handle the asynchronous nature of JavaScript promises. Here's how you can think about and solve this problem:
- Both
promise1
andpromise2
resolve to a numerical value as confirmed by the constraints. - Your task is to ensure that you only attempt to sum these numbers once both promises have fully resolved. Attempting to access their values prematurely will not yield the correct results since promises are asynchronous.
Using Promise Handling Functions:
- Promise.all():
- Utilize
Promise.all()
to await the resolution of bothpromise1
andpromise2
. Promise.all()
takes an array of promises and returns a new promise that resolves when all the promises in the array have resolved or if any promise is rejected.- The output of
Promise.all()
is an array containing the resolved values of each promise in the same order as they were passed in. - You can then sum the numerical values from the resolved array.
- Utilize
Implementation Steps:
- Pass
promise1
andpromise2
within an array toPromise.all()
. This will handle waiting for both promises to resolve. - Use
.then()
on the result ofPromise.all()
to access the array of resolved values. - Inside the
.then()
callback, extract the values, sum them, and resolve this sum with a new promise. - You also need to consider error handling. If any promise rejects, handle this gracefully.
This approach ensures that you're combining the data from both promises accurately and efficiently, respecting the asynchronous nature of JavaScript operations.
Solutions
- JavaScript
/**
* @param {Promise} firstPromise
* @param {Promise} secondPromise
* @return {Promise}
*/
var concatenatePromises = async function (firstPromise, secondPromise) {
return new Promise((resolve, reject) => {
let pendingPromises = 2;
let totalResult = 0;
[firstPromise, secondPromise].forEach(async singlePromise => {
try {
const partialResult = await singlePromise;
totalResult += partialResult;
pendingPromises--;
if (pendingPromises === 0) {
resolve(totalResult);
}
} catch (error) {
reject(error);
}
});
});
};
The JavaScript function concatenatePromises
takes two promises as inputs and returns a new promise. This new promise sums the resolved values of the input promises if both are successfully resolved. If either of the input promises is rejected, the new promise is rejected as well.
Here is a breakdown of the function's operation:
- Define a constructor for the new promise that takes
resolve
andreject
functions. - Initialize a counter
pendingPromises
set to 2, tracking the completion of both input promises. - Initialize a variable
totalResult
to 0 to accumulate the results. - Use a
forEach
loop on an array containing the two input promises, handling each promise independently. - Inside the loop, use
async
in front of the loop function to handle asynchronous promise resolution usingawait
. - Use
try
andcatch
blocks within each loop iteration:- In the
try
block, wait for the promise to resolve and add its value tototalResult
. Decrement thependingPromises
counter. - If
pendingPromises
reaches zero, all input promises have resolved, and thusresolve(totalResult)
is called. - The
catch
block handles any rejection by callingreject(error)
with the respective error.
- In the
This function effectively demonstrates handling multiple promises and performing actions based on their collective result within a single structured promise scenario. It leverages modern JavaScript async-await syntax for clarity and performance in asynchronous code executions.
No comments yet.