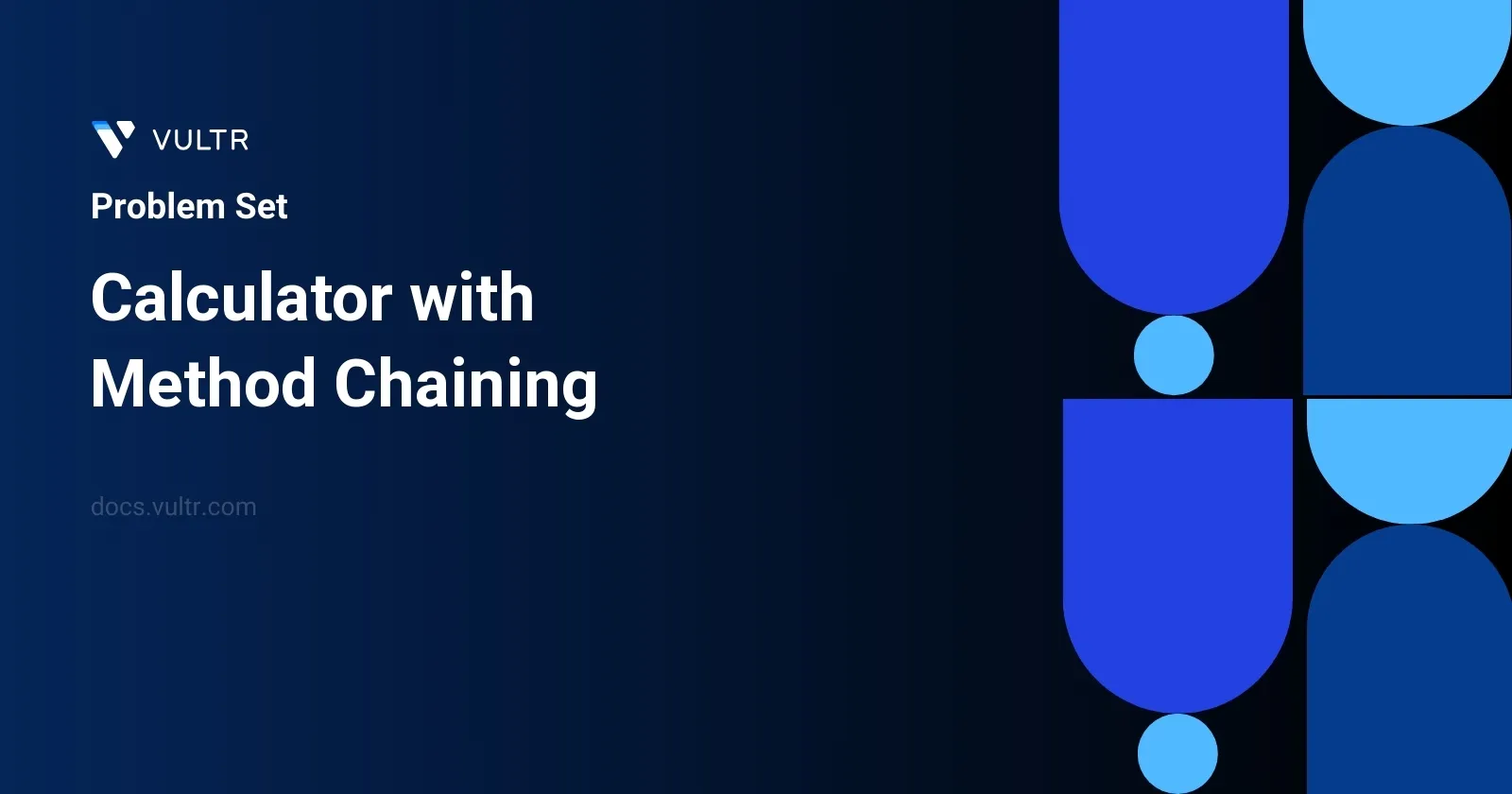
Problem Statement
The task is to design a Calculator
class capable of performing basic and advanced arithmetic operations including addition, subtraction, multiplication, division, and exponentiation. This class should be flexible enough to allow consecutive operations through method chaining, making the code neat and readable. The initial state of the calculator, including the starting value for these operations, is set through the class constructor.
The Calculator
class includes multiple methods:
add(value)
: This method can increase the current result by a specified value and return the modified Calculator instance for possible further chained operations.subtract(value)
: This method can decrease the current result by a specified value, allowing continuation of operations if necessary.multiply(value)
: Multiplication of the current result by the provided value is performed, and the Calculator can continue to operate with subsequent operations.divide(value)
: Divides the current result by the given value. It is critical to note that division by zero should trigger an error, preventing incorrect mathematical operations.power(value)
: This method can elevate the current result by the power of the specified value, expanding the capabilities of the Calculator to more complex mathematical functions.getResult()
: This method provides the end result of all the consecutive operations performed up until its call.
The accuracy of the operations should be maintained to very close approximations, as differences within 10-5
of the actual result are considered acceptable.
Examples
Example 1
Input:
actions = ["Calculator", "add", "subtract", "getResult"], values = [10, 5, 7]
Output:
8
Explanation:
new Calculator(10).add(5).subtract(7).getResult() // 10 + 5 - 7 = 8
Example 2
Input:
actions = ["Calculator", "multiply", "power", "getResult"], values = [2, 5, 2]
Output:
100
Explanation:
new Calculator(2).multiply(5).power(2).getResult() // (2 * 5) ^ 2 = 100
Example 3
Input:
actions = ["Calculator", "divide", "getResult"], values = [20, 0]
Output:
"Division by zero is not allowed"
Explanation:
new Calculator(20).divide(0).getResult() // 20 / 0 The error should be thrown because we cannot divide by zero.
Constraints
actions
is a valid JSON array of stringsvalues
is a valid JSON array of numbers2 <= actions.length <= 2 * 104
1 <= values.length <= 2 * 104 - 1
actions[i]
is one of "Calculator", "add", "subtract", "multiply", "divide", "power", and "getResult"- First action is always "Calculator"
- Last action is always "getResult"
Approach and Intuition
Given the structure and examples of how the Calculator class should function, it's clear that each method in the class not only performs a specific arithmetic operation but also returns the calculator object itself to facilitate method chaining. This design pattern is quite effective for scenarios where multiple operations on the same object are common, as it allows for streamlined and more readable usage.
Initialization: Upon instantiation, initialize the Calculator with a starting result value.
Method Chaining: Each operation method (
add
,subtract
,multiply
,divide
,power
) modifies the internal state of theresult
and returns theCalculator
object itself to enable further chained method calls.Handling Edge Cases: Ensure robust error handling specifically in the
divide
method where division by zero should throw a well-defined error, making the function safe against common mathematical faults.Returning Results: After performing all needed operations, the
getResult
method is used to fetch and return the final computation outcome. This is typically the last method called after a series of chained operations.Following the examples:
- Example 1 showcases a sequence of addition followed by a subtraction, concluding with fetching the result. It’s a straightforward use-case demonstrating method chaining.
- Example 2 demonstrates operations involving multiplication followed by an exponentiation, highlighting the calculator’s capability to handle more complex scenarios.
- Example 3 attempts a division by zero, which is correctly handled by throwing an error message, showcasing the calculator’s error handling capability in erroneous mathematical operations.
This class can be utilized in various computational applications where arithmetic operations are needed, providing a layer of abstraction that handles method chaining and error management internally.
Solutions
- JavaScript
class BasicCalculator {
constructor(initialValue) {
this.currentValue = initialValue;
}
add(term) {
this.currentValue += term;
return this;
}
subtract(subtrahend) {
this.currentValue -= subtrahend;
return this;
}
multiply(factor) {
this.currentValue *= factor;
return this;
}
divide(divisor) {
if (divisor === 0) throw "Division by zero is not allowed";
this.currentValue /= divisor;
return this;
}
power(exponent) {
this.currentValue **= exponent;
return this;
}
getCurrentResult() {
return this.currentValue;
}
}
The solution provided is a JavaScript class named BasicCalculator
that implements basic arithmetic operations with the capability for method chaining. This approach allows multiple operations to be performed sequentially on a single object.
The class is instantiated with an initial value for the calculation. Each method for performing arithmetic operations modifies the internal state of the calculator object and returns the object itself. This returning of the object allows for the chaining of multiple method calls.
The arithmetic operations supported are:
add(term)
: Adds the specified term to the current value.subtract(subtrahend)
: Subtracts the specified subtrahend from the current value.multiply(factor)
: Multiplies the current value by the specified factor.divide(divisor)
: Divides the current value by the specified divisor. The method throws an exception if an attempt is made to divide by zero, ensuring the calculator handles this edge case gracefully.power(exponent)
: Raises the current value to the power of the specified exponent.
The method
getCurrentResult()
returns the result of the calculations performed up to that point.
This class provides a clear and modular way to perform and chain together multiple operations, making the code cleaner and easier to manage compared to managing individual operations separately. This structure also enhances readability and maintainability.
No comments yet.