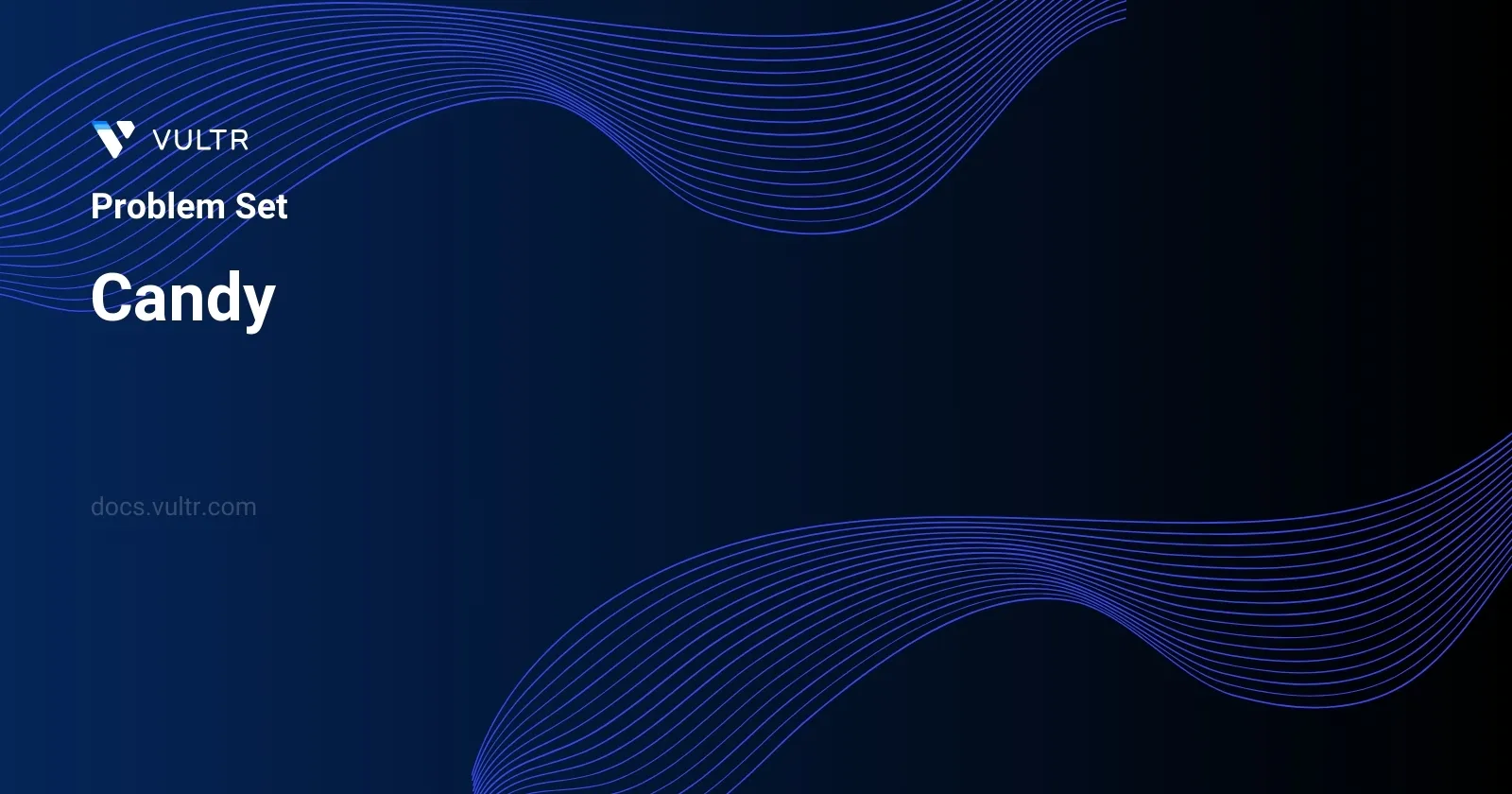
Problem Statement
In this problem, we are given a line of n
children, each assigned a specific rating which is represented by an integer array called ratings
. The task involves distributing candies such that:
- Every child receives at least one candy.
- A child with a higher rating than his/her adjacent neighbors receives more candies compared to those neighbors.
The goal is to determine the minimum number of candies required to meet these distribution rules.
Examples
Example 1
Input:
ratings = [1,0,2]
Output:
5
Explanation:
You can allocate to the first, second and third child with 2, 1, 2 candies respectively.
Example 2
Input:
ratings = [1,2,2]
Output:
4
Explanation:
You can allocate to the first, second and third child with 1, 2, 1 candies respectively. The third child gets 1 candy because it satisfies the above two conditions.
Constraints
n == ratings.length
1 <= n <= 2 * 104
0 <= ratings[i] <= 2 * 104
Approach and Intuition
To solve this problem efficiently while ensuring all given conditions are met, we can approach it using two passes through the ratings
array:
First Pass (Left to Right): Start by allocating each child one candy. Then, iterate from the beginning to the end of the
ratings
array. If a child has a higher rating than the preceding child, give him/her one more candy than the preceding child.Second Pass (Right to Left): After the first pass, sometimes the left neighbor might have a higher rating, but fewer candies than the right neighbor due to adjustments made for other children. To rectify this, make another pass through the list from the end to the beginning. If a child has a higher rating than the next child but has equal or fewer candies, then increase their candy count to one more than the child with the next rating.
This approach ensures that each child gets more candies than their lower-rated neighbors after adjustments from both directions, meeting the conditions. Count the total candies distributed to find the minimum number required. Here's how it appears with the given examples:
- Example 1 demonstrates allocating 2, 1, 2 candies which ensures the child with rating 0 (second child) gets fewer candies than its neighbors.
- Example 2 demonstrates allocating 1, 2, 1 candy where even with the last two children having the same rating, they receive the same count of candies.
Through these steps, while traversing the list twice, we can efficiently calculate the exact number of candies required.
Solutions
- C++
- Java
- C
- JavaScript
- Python
class Solution {
public:
int sumOfNaturalNumbers(int n) { return (n * (n + 1)) / 2; }
int distributeCandies(vector<int>& ratings) {
if (ratings.size() <= 1) {
return ratings.size();
}
int totalCandies = 0;
int ascending = 0;
int descending = 0;
int previousSlope = 0;
for (int i = 1; i < ratings.size(); i++) {
int currentSlope = (ratings[i] > ratings[i - 1])
? 1
: (ratings[i] < ratings[i - 1] ? -1 : 0);
if ((previousSlope > 0 && currentSlope == 0) || (previousSlope < 0 && currentSlope >= 0)) {
totalCandies += sumOfNaturalNumbers(ascending) + sumOfNaturalNumbers(descending) + max(ascending, descending);
ascending = 0;
descending = 0;
}
if (currentSlope > 0) {
ascending++;
} else if (currentSlope < 0) {
descending++;
} else {
totalCandies++;
}
previousSlope = currentSlope;
}
totalCandies += sumOfNaturalNumbers(ascending) + sumOfNaturalNumbers(descending) + max(ascending, descending) + 1;
return totalCandies;
}
};
The provided C++ code implements a function to distribute candies based on an array of child ratings. Each child must receive at least one candy, and children with a higher rating than their immediate neighbors should receive more candies. The logic centers on maintaining and adjusting a count of candies distributed as you iterate through the ratings array.
- The code starts by checking if the size of the
ratings
vector is less than or equal to 1, returning the size directly as each child (or no child) gets exactly 1 candy. - Variables
totalCandies
,ascending
,descending
, andpreviousSlope
are initialized.ascending
anddescending
track the length of consecutive sequences where ratings increase or decrease.previousSlope
helps determine changes in rating trends. - A loop goes through the
ratings
vector, computing the slope —whether it's ascending, descending, or neutral— between consecutive ratings. This helps in determining if sequences of increasing or decreasing ratings end, which is crucial for candy distribution.- If a rating slope change (from ascending to neutral/descending or descending to ascending/neutral) is detected, candies are distributed based on the sums of natural numbers up to the lengths of the sequences (
ascending
anddescending
), plus the greater of the two to ensure fairness around peaks. - Reset
ascending
anddescending
accordingly.
- If a rating slope change (from ascending to neutral/descending or descending to ascending/neutral) is detected, candies are distributed based on the sums of natural numbers up to the lengths of the sequences (
- After iterating through the vector, wrap up by calculating candies for any remaining sequences.
- The function uses an auxiliary function
sumOfNaturalNumbers
to calculate the sum of the firstn
natural numbers, which is central to distributing candies according to consecutive sequences of higher or lower ratings.
This solution efficiently handles the complex criteria for candy distribution in (O(n)) time, where (n) is the number of ratings, thus ensuring each child receives the correct amount of candy according to their relative ratings.
public class Solution {
public int sum_natural_numbers(int x) {
return (x * (x + 1)) / 2;
}
public int distribute_candies(int[] scores) {
if (scores.length <= 1) {
return scores.length;
}
int totalCandies = 0;
int increasing = 0;
int decreasing = 0;
int previousSlope = 0;
for (int j = 1; j < scores.length; j++) {
int currentSlope = (scores[j] > scores[j - 1])
? 1
: (scores[j] < scores[j - 1] ? -1 : 0);
if (
(previousSlope > 0 && currentSlope == 0) ||
(previousSlope < 0 && currentSlope >= 0)
) {
totalCandies += sum_natural_numbers(increasing) + sum_natural_numbers(decreasing) + Math.max(increasing, decreasing);
increasing = 0;
decreasing = 0;
}
if (currentSlope > 0) {
increasing++;
} else if (currentSlope < 0) {
decreasing++;
} else {
totalCandies++;
}
previousSlope = currentSlope;
}
totalCandies += sum_natural_numbers(increasing) + sum_natural_numbers(decreasing) + Math.max(increasing, decreasing) + 1;
return totalCandies;
}
}
Implement the solution for candy distribution based on the scores using the Java programming language through two main functions in the Solution
class.
sum_natural_numbers(int x)
- Calculate the sum of the first
x
natural numbers. Utilize the mathematical formula(x * (x + 1)) / 2
. This function supports efficient calculation of the total candies to give during increasing or decreasing streaks of scores.
- Calculate the sum of the first
distribute_candies(int[] scores)
- Allocate candies to participants based on their scores, ensuring that participants with higher scores than their neighboring participant(s) receive more candies.
- Initialize variables to manage the total candies distributed, the length of increasing and decreasing sequences of scores, and tracking the previous comparison state between two continuous scores.
- Iterate through the
scores
array to update the direction of the slope (increasing, decreasing, or flat) based on consecutive scores. - Adjust candy calculation when a transition between increasing and decreasing sequences is detected, ensuring that sequences are appropriately rewarded with candies by making use of the
sum_natural_numbers
function. - Apply the formula for an unfinished sequence at the end of the loop to ensure all candies are counted.
- The method returns the computed total candies, effectively ensuring fairness and meeting the condition that a higher score receives more candies relative to its immediate neighbors.
This structured approach ensures clarity in distributing candies according to a given scoring pattern while maintaining time efficiency with direct calculations for sequences of scores.
int sum_natural_numbers(int num) { return (num * (num + 1)) / 2; }
int maximum(int x, int y) { return ((x) > (y) ? (x) : (y)); }
int distribute_candies(int* scores, int size) {
if (size <= 1) {
return size;
}
int total_candies = 0;
int ascent = 0;
int descent = 0;
int previous_slope = 0;
for (int i = 1; i < size; i++) {
int current_slope = (scores[i] > scores[i - 1])
? 1
: ((scores[i] < scores[i - 1]) ? -1 : 0);
if ((previous_slope > 0 && current_slope == 0) ||
(previous_slope < 0 && current_slope >= 0)) {
total_candies += sum_natural_numbers(ascent) + sum_natural_numbers(descent) + maximum(ascent, descent);
ascent = 0;
descent = 0;
}
if (current_slope > 0) {
ascent++;
}
else if (current_slope < 0) {
descent++;
}
else {
total_candies++;
}
previous_slope = current_slope;
}
total_candies += sum_natural_numbers(ascent) + sum_natural_numbers(descent) + maximum(ascent, descent) + 1;
return total_candies;
}
The provided C program is designed to distribute candies to a list of students based on their scores in a way that students with higher scores than their immediate neighbors receive more candies. The solution computes the total amount of candies needed through these steps:
Define helper functions:
- A function,
sum_natural_numbers(int num)
, computes the sum of the firstnum
natural numbers. This is useful for calculating the total candies given to players on a upward or downward streak (ascent or descent). - Another function,
maximum(int x, int y)
, returns the maximum of two integers. It's used to determine which slope, ascent or descent, should contribute an extra candy when transitioning.
- A function,
The main logic in
distribute_candies(int* scores, int size)
function:- Edge cases handle scenarios with
size <= 1
. If there's one or zero students, the candies needed are equal to the number of students. - Iterate through scores using a
for
loop from the second score to analyze the relationship between consecutive scores:- Determine the current slope (increase, decrease, or no change) between successive scores.
- Handle changes in slope (from ascent to descent and vice versa). Upon each change:
- Calculate candies for the previous streaks by summing up natural numbers up to the lengths of
ascent
anddescent
and add the maximum of the two streak lengths to ensure the highest peak gets an adequate number of candies. - Reset both
ascent
anddescent
.
- Calculate candies for the previous streaks by summing up natural numbers up to the lengths of
- Update
total_candies
directly for unchanged scores. - Maintain a count of
ascent
anddescent
based on the current slope.
- After looping, add to
total_candies
any remaining candies based on the final streak lengths using similar calculations outside the loop.
- Edge cases handle scenarios with
Return the total candies calculated which ensures a fair distribution where each student only gets more candies than their closest lesser-scored neighbor.
By dissecting the code in this manner, gain control over candy distribution according to varying score sequences among students, adapting dynamically to any score pattern. The implementation strives for optimal space and time complexity while ensuring clarity and efficiency in execution.
var sum_natural_numbers = function (n) {
return (n * (n + 1)) / 2;
};
var distribute_candies = function (ratings) {
if (ratings.length <= 1) {
return ratings.length;
}
var total_candies = 0;
var ascend = 0;
var descend = 0;
var previous_slope = 0;
for (var index = 1; index < ratings.length; index++) {
var current_slope =
ratings[index] > ratings[index - 1]
? 1
: ratings[index] < ratings[index - 1]
? -1
: 0;
if (
(previous_slope > 0 && current_slope == 0) ||
(previous_slope < 0 && current_slope >= 0)
) {
total_candies += sum_natural_numbers(ascend) + sum_natural_numbers(descend) + Math.max(ascend, descend);
ascend = 0;
descend = 0;
}
if (current_slope > 0) {
ascend++;
}
else if (current_slope < 0) {
descend++;
}
else {
total_candies++;
}
previous_slope = current_slope;
}
total_candies += sum_natural_numbers(ascend) + sum_natural_numbers(descend) + Math.max(ascend, descend) + 1;
return total_candies;
};
The given JavaScript solution addresses the problem of candy distribution based on ratings where each student must have more candies than an adjacent student with a higher rating. The solution comprises two primary functions:
sum_natural_numbers
: This function efficiently calculates the sum of the firstn
natural numbers using the formulan * (n + 1) / 2
. This is used to determine the total number of candies during increasing or decreasing rating sequences.distribute_candies
: This function is designed to handle the logic for distributing candies according to the ratings array provided. The algorithm iteratively evaluates the ratings to determine increases and decreases in the value:- Track current slopes (increase or decrease) using the
ascend
anddescend
counters. - Adjust candy totals when transitions between slopes occur.
- Use the
sum_natural_numbers
function to add up the candies for sequences of ascending or descending ratings. - Ensure that the peak between an ascending and descending sequence gets the maximum possible candies from either side of the sequence.
- Track current slopes (increase or decrease) using the
Handle edge cases where:
- The ratings array has length 0 or 1, returning 0 or 1 respectively.
- When ratings are not strictly changing, ensuring each student in a flat portion of ratings receives only one candy.
In conclusion, the algorithm effectively adjusts the candy distribution using slope analysis and accumulates the total candies needed, making sure to optimize candy distribution during transitions in the student rating slope. This method guarantees that all conditions for the candy distribution problem are met with optimal efficiency.
class Solution:
# Implements logic to find the total candy distribution count
def sum_natural(self, x):
return (x * (x + 1)) // 2
def distribute_candy(self, scores):
if len(scores) <= 1:
return len(scores)
total_candies = 0
ascend = 0
descend = 0
previous_slope = 0
for index in range(1, len(scores)):
current_slope = (
1
if scores[index] > scores[index - 1]
else (-1 if scores[index] < scores[index - 1] else 0)
)
if (previous_slope > 0 and current_slope == 0) or (
previous_slope < 0 and current_slope >= 0
):
total_candies += self.sum_natural(ascend) + self.sum_natural(descend) + max(ascend, descend)
ascend = 0
descend = 0
if current_slope > 0:
ascend += 1
elif current_slope < 0:
descend += 1
else:
total_candies += 1
previous_slope = current_slope
total_candies += self.sum_natural(ascend) + self.sum_natural(descend) + max(ascend, descend) + 1
return total_candies
The Python solution for the "Candy" problem determines the minimum number of candies required for distribution based on an array of scores, where each score represents a child. The algorithm aims to ensure every child has at least one candy, and any child with a higher score than an adjacent child receives more candies than that child.
Key Steps in the Solution:
Define an auxiliary function
sum_natural(x)
which calculates the sum of the firstx
natural numbers. This helps in summing up the total candies for a group of children either ascending or descending in their scores.In the primary function
distribute_candy(scores)
, check if the scores length is 1 or less, returning the length directly if true, as each child gets at least one candy.Initialize variables
ascend
,descend
,previous_slope
, andtotal_candies
to manage candy distribution among ascending and descending sequences of scores, as well as tracking previous score comparisons.Iterate through each score from the second child to the last. Determine the slope (increasing, decreasing, or neutral) by comparing the current score with the previous one.
Adjust
total_candies
based on the changes in sequences (from ascending to descending or at the end of a sequence). The candies are calculated using the natural sum of ascent or descent streak lengths, also considering the maximum of these values for the crucial point where the sequence changes.At the end of the loop, include the remaining candies calculation for the last sequence of children.
The resulting
total_candies
represents the minimum candies required following the constraints provided by the scores array.
Considerations:
- Iterable through scores is necessary for comparing each child to its adjacent counterpart, crucial for relative candy distribution.
- The solution effectively handles varying patterns in the score sequences using the
previous_slope
and adjusting the candy distribution each time the pattern switches. - The algorithm ensures fairness in candy distribution with a linear time complexity relative to the number of children.
By following these steps, ensure an efficient distribution of candies such that the requirements of more candies for higher scores in neighbors are met.
No comments yet.