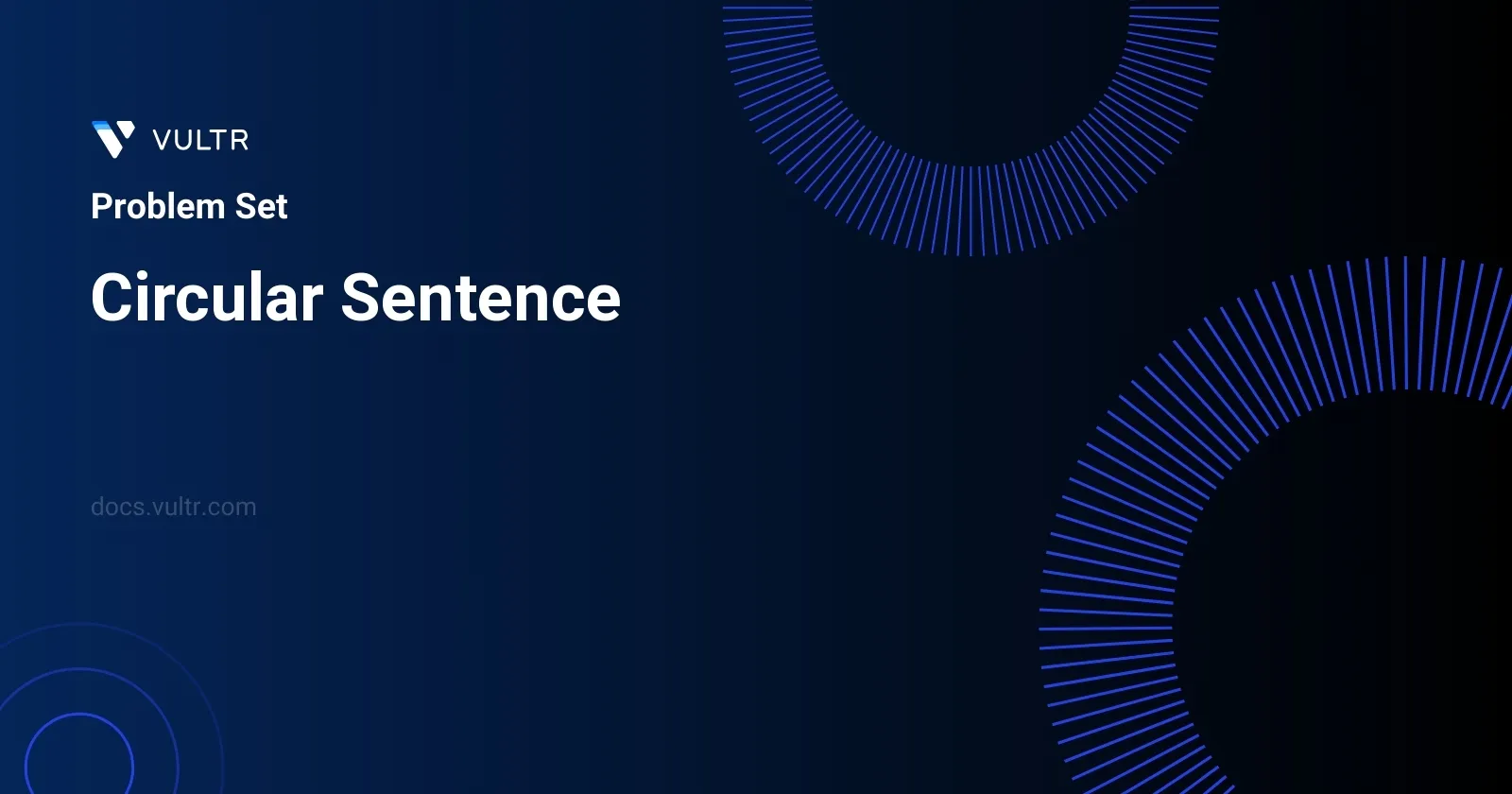
Problem Statement
In this scenario, the challenge is to evaluate whether a given sentence qualifies as a "circular sentence." The definition of a circular sentence relies on the relationship between the last character of one word and the first character of the next. A sentence can be deemed circular if, and only if, for every word present in the sentence, the last character matches the first character of the subsequent word, and importantly, the sequence wraps around such that the last character of the entire sentence matches the first character of its beginning. While processing the sentence, considerations are given to the distinctiveness of uppercase and lowercase English letters, and multiple spaces are not permitted between words.
Examples
Example 1
Input:
sentence = "leetcode exercises sound delightful"
Output:
true
Explanation:
The words in sentence are ["leetcode", "exercises", "sound", "delightful"]. - leetcode's last character is equal to exercises's first character. - exercises's last character is equal to sound's first character. - sound's last character is equal to delightful's first character. - delightful's last character is equal to leetcode's first character. The sentence is circular.
Example 2
Input:
sentence = "eetcode"
Output:
true
Explanation:
The words in sentence are ["eetcode"]. - eetcode's last character is equal to eetcode's first character. The sentence is circular.
Example 3
Input:
sentence = "Leetcode is cool"
Output:
false
Explanation:
The words in sentence are ["Leetcode", "is", "cool"].
- Leetcode's last character is not equal to is's first character. The sentence is not circular.
Constraints
1 <= sentence.length <= 500
sentence
consist of only lowercase and uppercase English letters and spaces.- The words in
sentence
are separated by a single space. - There are no leading or trailing spaces.
Approach and Intuition
Extraction of Words:
Start by splitting the sentence string into an array of words. This parsing step prepares for the subsequent character comparisons.Comparison for Circular Condition:
- Perform a loop over the list of words and compare the last character of the current word with the first character of the next word.
- Ensure circularity by checking if the last character of the last word matches the first character of the first word.
- This looping and matching ascertain if the cyclical connections between the beginning and end of words (and the start and end of the sequence) hold true.
Edge Case Consideration:
- If there is only one word, check if its first and last characters are the same.
- Handle the corner cases considering the words might vary in length, from very short to matching the maximum allowed characters.
Complexity and Execution:
- Given constraints suggest the necessity for efficient checking since the sentences can be quite long (up to 500 characters).
- The operation where each word’s boundary characters are compared involves linear time complexity relative to the number of words, which is optimal for this problem size.
The constraints ensure no leading or trailing spaces and that words are correctly formed only from English letters, simplifying the parsing task and focusing efforts on the cyclical pattern checks. This approach avoids unnecessary complexity and efficiently determines whether the sentence structure meets the circular criteria.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool circularPhraseCheck(string phrase) {
for (int idx = 0; idx < phrase.length(); ++idx)
if (phrase[idx] == ' ' && phrase[idx - 1] != phrase[idx + 1])
return false;
return phrase.front() == phrase.back();
}
};
The given C++ solution defines a function circularPhraseCheck
that checks if a given sentence is circular based on specific conditions. The function works as follows:
- It iterates through each character of the input string
phrase
. For each character at positionidx
, it checks:- If the current character is a space and the character before the space is not the same as the character after the space, the function returns
false
.
- If the current character is a space and the character before the space is not the same as the character after the space, the function returns
- After the loop, it checks whether the first and the last character of the phrase are the same. If they are, it returns
true
; otherwise,false
.
This implementation effectively ensures that a phrase is considered circular if it starts and ends with the same character, under the condition that no inconsistencies (unequal characters around spaces) are found during the iteration through the phrase.
public class Solution {
public boolean isRotationalPhrase(String phrase) {
for (int index = 0; index < phrase.length(); index++) {
if (phrase.charAt(index) == ' ' && phrase.charAt(index - 1) != phrase.charAt(index + 1)) return false;
}
return phrase.charAt(0) == phrase.charAt(phrase.length() - 1);
}
}
The solution presented in Java is for determining if the input string, referred to here as a 'phrase', is a rotational phrase. A rotational phrase in this context means the phrase ends and starts with the same character, and after each space, the next character is not the same as the previous one.
The method isRotationalPhrase
accomplishes this by:
- Iterating through each character of the phrase.
- Checking if the character at the current index is a space and ensuring that the character immediately after the space is not equal to the character immediately before it. If this condition fails for any space, the method returns
false
. - After the loop, confirming that the first and last characters of the phrase are the same. If they are, it returns
true
; otherwise, it returnsfalse
.
This method of checking ensures a specific consistency in the structure of the input phrase, making it fit the definition of a rotational phrase as per the provided conditions. If the phrase meets these criteria, the function acknowledges it as rotational; otherwise, it doesn't.
class Solution:
def check_circular_text(self, text: str) -> bool:
length = len(text)
for char_index in range(length):
if text[char_index] == ' ' and text[char_index - 1] != text[(char_index + 1) % length]:
return False
return text[0] == text[-1]
The given Python code defines a method check_circular_text
within the Solution
class that evaluates if a given text string can be considered circular. The method operates by checking each character in the string for specific conditions:
- It iterates through each character in the string.
- If the character is a space, the method checks that the character before the space is the same as the one after the space, considering the string as wrapped around (circular). For this, modulo operation is used to wrap around when checking characters after the space.
- Additionally, the first character of the text must be the same as the last character to maintain a circular continuity.
The method returns True
if all spaces are flanked by the same characters (considering wrapping) and the first and last characters are the same; otherwise, it returns False
. This function effectively allows for checking if the string can be read the same way from any point, with spaces as possible resets, assuming character continuation around the string as if it is circular.
No comments yet.