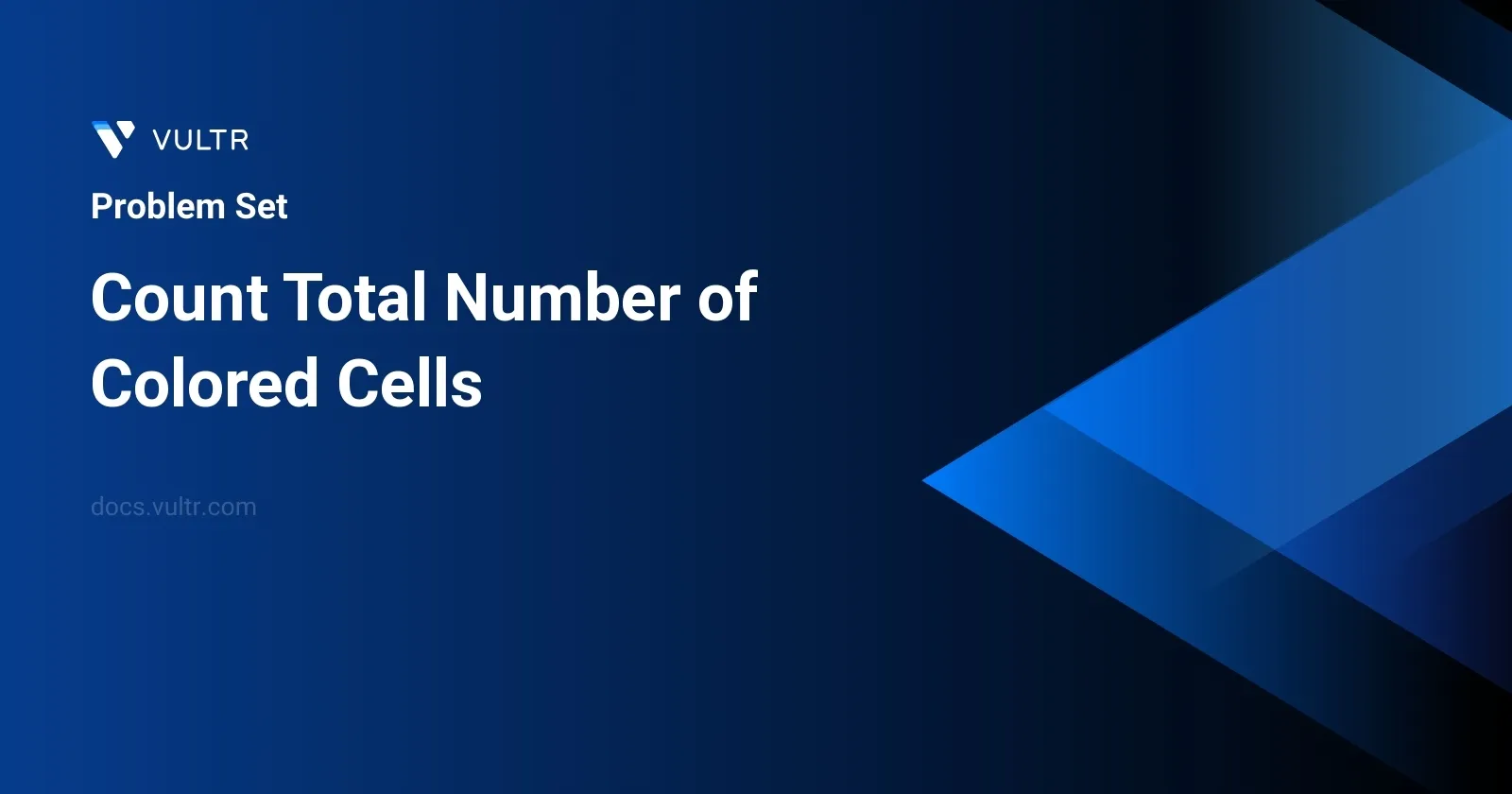
Problem Statement
In this problem, we are working with an infinitely large two-dimensional grid made up of unit cells. Initially, all cells are uncolored. Over a series of n
minutes, a coloring process takes place. Here’s how it works:
- In the first minute, we start by coloring any chosen cell blue.
- For every subsequent minute, each uncolored cell that is adjacent (touches at least one side) to a blue cell is also colored blue.
The goal is to determine the total number of blue colored cells on the grid after n
minutes.
Examples
Example 1
Input:
n = 1
Output:
1
Explanation:
After 1 minute, there is only 1 blue cell, so we return 1.
Example 2
Input:
n = 2
Output:
5
Explanation:
After 2 minutes, there are 4 colored cells on the boundary and 1 in the center, so we return 5.
Constraints
1 <= n <= 105
Approach and Intuition
Given the problem's unique way of coloring cells across minutes, we can derive the pattern of cell expansion:
- In the first minute, we start with one blue cell.
- Every minute thereafter, the squared area of colored cells grows, primarily due to the cells touching the sides of the already colored area. Specifically, for each subsequent minute:
- Each side of the current square of blue cells "expands" outward, adding a layer of newly colored cells that surrounds the existing blue region.
Given a cell selected randomly at the beginning, the growth of the colored area can be visualized as follows:
- Minute 1: A single cell is colored.
- Minute 2: Additional cells surrounding the initial cell are colored, resulting in a total of 5 cells - forming a plus shape.
- Minute 3 and beyond: This pattern continues, where each minute adds a layer around the existing blue cells, expanding the blue region in a square shape with increasingly larger perimeters.
Calculation:
The number of blue cells can thus be calculated using the growth pattern:
- Basically, the area grows in a diamond (or square when rotated) pattern. The maximum distance from the initially colored cell to any cell in the boundary of the square formed by the end of
n
minutes isn - 1
cells away in the cardinal directions. - The cells formed by this growth in
n
minutes is2n^2 - 2n + 1
. This formula comes from observing that the expansion forms concentric diamonds of increasing size, where each side of the diamond i layers away from the center is2i
cells plus the center cell.
Understanding these points helps in visualizing and solving the problem efficiently without the need for simulating the process on an actual grid, thus optimizing for larger values of n
within computational constraints.
Solutions
- C++
- Java
- Python
class Solution {
public:
long long countColoredCells(int m) { return 1 + (long long)m * (m - 1) * 2; }
};
The provided C++ solution comprises a class named Solution
responsible for counting the total number of colored cells. The function countColoredCells(int m)
calculates the total number of colored cells in a grid, utilizing the formula 1 + (long long)m * (m - 1) * 2
. This formula strategically uses mathematical operations to derive the result based on the input value of m
. The method correctly returns a long long type output, ensuring that it can handle large numbers that might result from large inputs for m
. This solution is efficient as it computes the result in constant time, O(1), given that it uses only arithmetic calculations without any loops or recursion.
class Solution {
public long paintedCells(int size) {
return 1 + (long) size * (size - 1) * 2;
}
}
You'll solve the problem of counting the total number of colored cells based on a given size using Java. The provided method, paintedCells(int size)
, efficiently calculates the result by using a simple mathematical formula.
The function:
- Takes an integer
size
as input - Returns a
long
type result - Computes the number of colored cells using the formula
1 + (long) size * (size - 1) * 2
This calculation directly offers the result without iteration or recursion, leveraging arithmetic operations to figure out the total colored cells based on input size. This is achieved by first converting part of the calculation to a long
type to prevent overflow or loss of data due to large numbers, then executing multiplication and addition as defined by the pattern derived from the specific conditions of the problem. This method ensures efficient and quick computation even for large input values.
class Solution:
def countColoredCells(self, size: int) -> int:
return 1 + size * (size - 1) * 2
The provided Python solution defines a method countColoredCells
within a class Solution
, which calculates the total number of colored cells based on a given size parameter. The calculation involves using the formula 1 + size * (size - 1) * 2
. This formula seems to be derived from a geometric pattern or sequence depending on the structure of the problem. The return statement directly computes and returns the total count of colored cells using this formula. When you use this function, pass an integer value for size
, and the function will give the total count of colored cells accordingly. Remember to initiate an instance of the Solution
class and call the countColoredCells
method to get the result.
No comments yet.