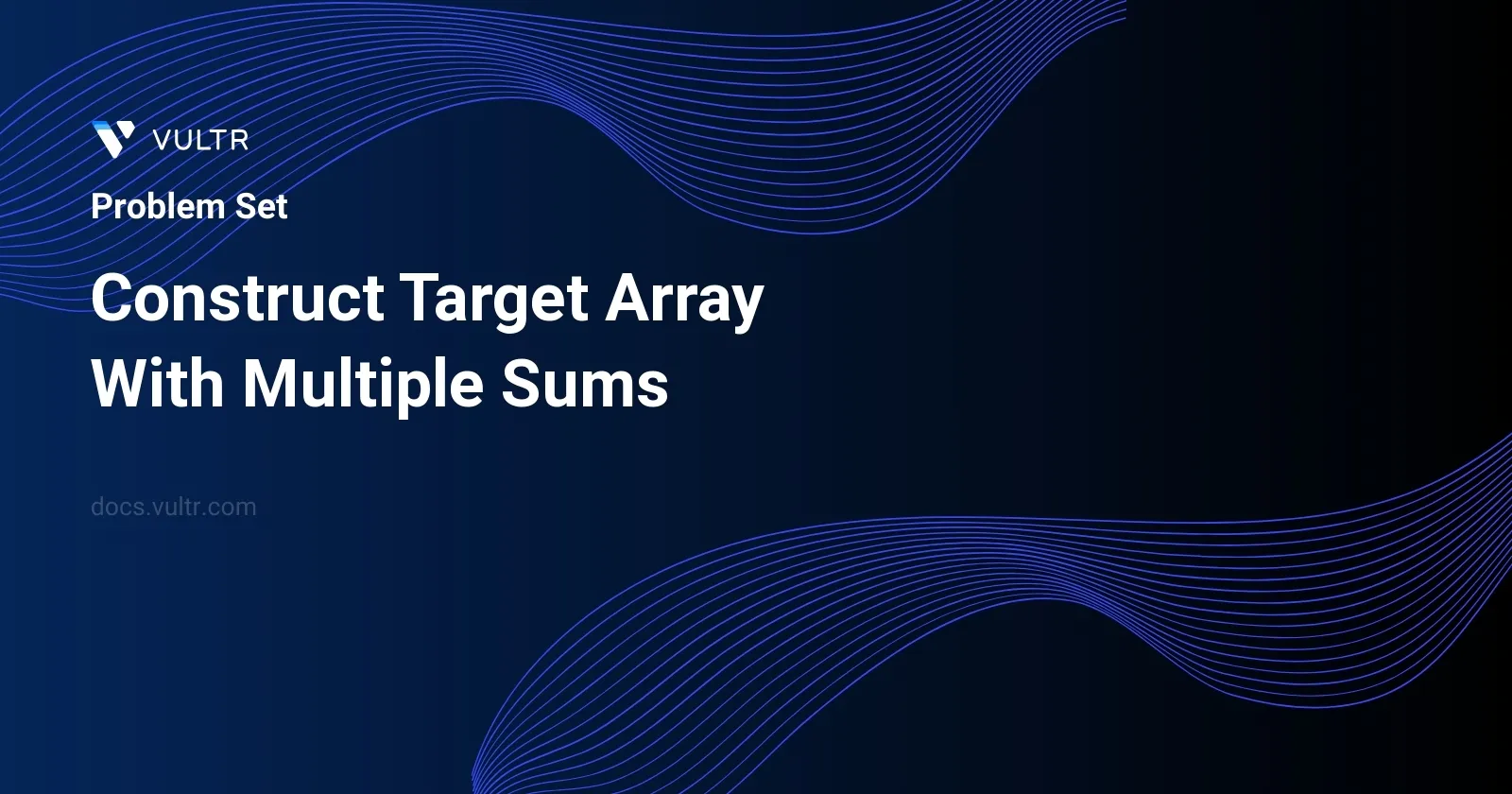
Problem Statement
In this task, you are provided with an array referred to as target
which contains n
integers. To address the challenge, you start with another array called arr
initialized with n
elements, all of which are 1s. Through a sequence of operations, you aim to transform arr
into exactly matching the target
array. Each allowed operation involves calculating x
, the sum of all current elements in arr
, and then replacing the content of arr
at a particular index i
(where 0 <= i < n
) with this sum x
. The process can be iterated as many times as necessary. You need to determine if it is possible to use these operations to turn arr
into target
and return true
if you can, or false
if you cannot.
Examples
Example 1
Input:
target = [9,3,5]
Output:
true
Explanation:
Start with arr = [1, 1, 1] [1, 1, 1], sum = 3 choose index 1 [1, 3, 1], sum = 5 choose index 2 [1, 3, 5], sum = 9 choose index 0 [9, 3, 5] Done
Example 2
Input:
target = [1,1,1,2]
Output:
false
Explanation:
Impossible to create target array from [1,1,1,1].
Example 3
Input:
target = [8,5]
Output:
true
Constraints
n == target.length
1 <= n <= 5 * 104
1 <= target[i] <= 109
Approach and Intuition
The operations on the arr
can be visualized as iteratively building the target
by accumulating the current sum of arr
into its individual elements until arr
equals to target
. Let's go step by step to understand and solve this:
Visual Verification Through Backward Construction:
- Instead of trying to transform
arr
intotarget
directly, the intuition is to think of howtarget
could have been formed in reverse. This involves working backwards to see if a validx
can be identified for each position intarget
.
- Instead of trying to transform
Simulate the Operations:
- From the examples given, clearly, the operations accumulate values in the
arr
. Start fromtarget
and repeatedly work backwards. Identifyprev
(the previous state ofarr
), which would be derived from subtracting from the largest item in currentarr
and setting it into the next biggest index until the initial state[1, 1, ..., 1]
is reached or consistency falls apart.
- From the examples given, clearly, the operations accumulate values in the
Validating the Process and Integer Constraints:
- With each iteration, validate if the transformation (or backward step) adheres to the constraints and actually edges closer to the initial state without breaking any rule (like turning any number negative or zero, which isn't feasible operationally according to problem constraints).
Optimal Checks:
- The possibilities can be quickly pruned or decided by comparing the sums of
arr
andtarget
. If at any step the sum of values in thearr
derived via backward reconstruction doesn’t match the summed integers series leading totarget
, it’s an indication that formingtarget
is infeasible under the given operations.
- The possibilities can be quickly pruned or decided by comparing the sums of
Continue Until Completion
- Continue this simulated process with control mechanisms until a conclusive decision point (true or false) is reached based on feasible backtracking or resolvable series matching.
The intuition here relies significantly on these transformations and validation checkpoints, making sure the starting arr
can truly evolve into the target
with the given procedures.
Solutions
- Java
class Solution {
public boolean canTransform(int[] target) {
// Early return for single element arrays
if (target.length == 1) {
return target[0] == 1;
}
int sumElements = Arrays.stream(target).sum();
PriorityQueue<Integer> maxHeap = new PriorityQueue<Integer>(Collections.reverseOrder());
for (int element : target) {
maxHeap.add(element);
}
while (maxHeap.peek() > 1) {
int maxElement = maxHeap.poll();
int remainingSum = sumElements - maxElement;
// Directly return for two elements scenario
if (remainingSum == 1) {
return true;
}
int remainder = maxElement % remainingSum;
// Check for invalid or non-changing cases
if (remainder == 0 || remainder == maxElement) {
return false;
}
maxHeap.add(remainder);
sumElements = sumElements - maxElement + remainder;
}
return true;
}
}
This Java solution addresses the problem of checking whether it's possible to transform a given target array to an all-ones array by reversing the operation that initially built it from an array of ones. The operations allowed are replacing any element with the sum of the entire array excluding that element.
Here's a breakdown of the key steps in the provided solution:
Begin by checking if the array has a single element: return true if it's 1, otherwise false, since a single-element array can only be transformed if it's already 1.
Calculate the sum of all elements in the array using Java's
Arrays.stream()
method.A
PriorityQueue
(max heap) is utilized to always process the maximum element in the array, significantly optimizing operations related to finding and handling the largest current value.The main loop continues processing while the largest item in the heap is more than 1. Inside the loop:
- Extract the largest element and compute the sum of the remaining elements.
- For a two-element target, return true if the other element is 1 (since it's straightforward to transform).
- Calculate the remainder when the largest element is divided by the sum of the rest. This step is crucial as it essentially simulates the reverse operation.
- Check for non-changing cases where the remainder might be 0 or equal to the max element itself, which implies transformation isn't possible and returns false.
- Update the heap with the remainder and adjust the total sum accordingly.
If the algorithm exits the loop, this means all elements have been transformed to 1, returning true.
This method ensures an efficient transformation check using a max-heap (priority queue) to continuously focus on the largest challenges (elements) first, and effectively reduces the problem size using modulo operations, adhering to the problem's constraints and targets.
No comments yet.