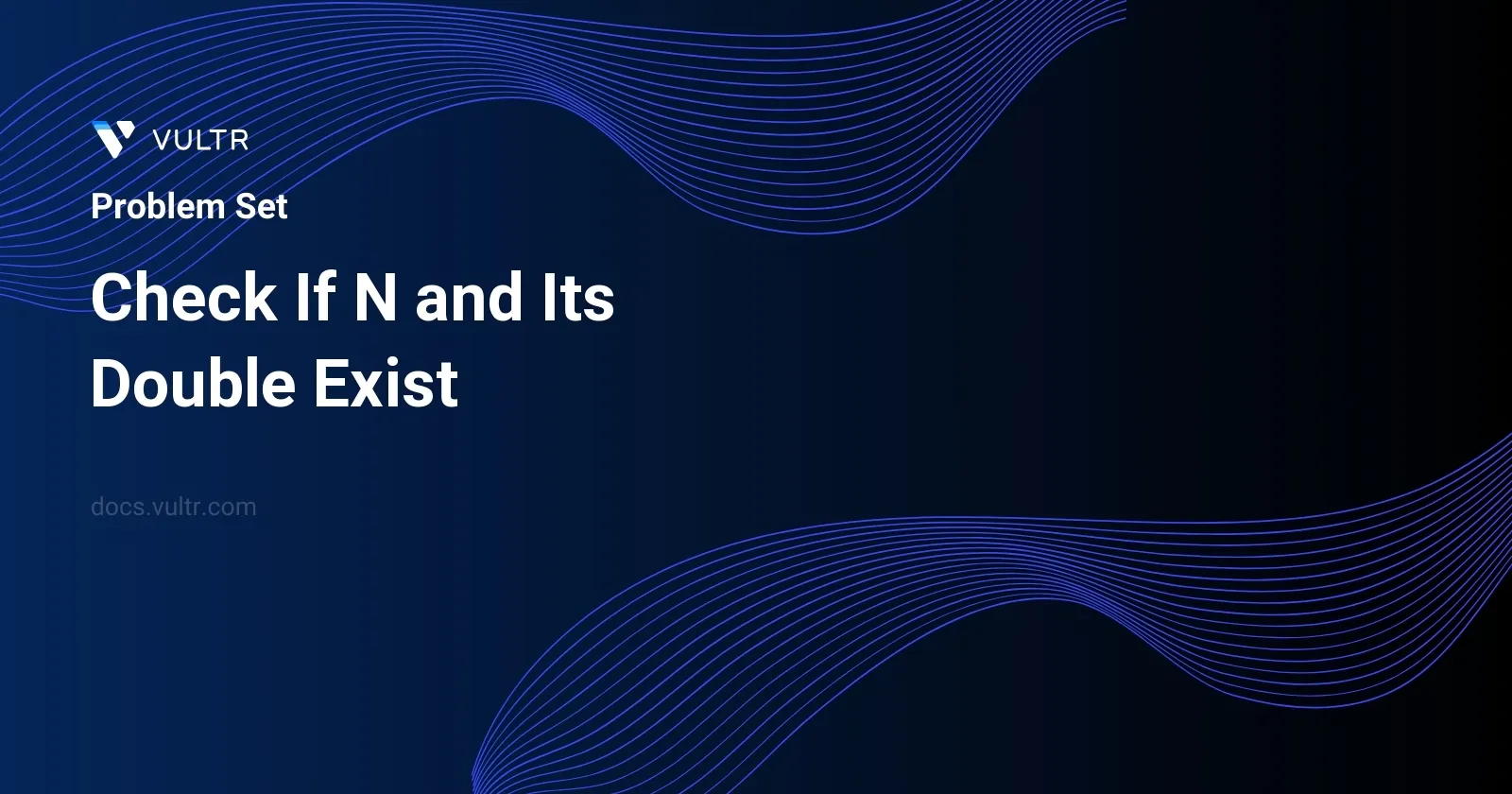
Problem Statement
In this problem, you are provided with an array of integers arr
. The task is to determine if there are two distinct indices i
and j
in the array such that the value at index i
is double the value at index j
. More formally, the conditions to satisfy are:
- the indices
i
andj
should be different (i != j
), - both indices must be valid within the array bounds (
0 <= i, j < arr.length
), - and the value at
i
should be exactly twice the value atj
(arr[i] == 2 * arr[j]
).
Understanding whether such a pair (i, j)
exists in the array based on the given conditions would effectively address the problem.
Examples
Example 1
Input:
arr = [10,2,5,3]
Output:
true
Explanation:
For i = 0 and j = 2, arr[i] == 10 == 2 * 5 == 2 * arr[j]
Example 2
Input:
arr = [3,1,7,11]
Output:
false
Explanation:
There is no i and j that satisfy the conditions.
Constraints
2 <= arr.length <= 500
-103 <= arr[i] <= 103
Approach and Intuition
The given problem revolves around finding a particular relationship between two array elements denoted by their indices. Given the constraints and properties, here's an intuitive approach to solve it:
- Traverse through the array using a loop where each element is considered as potential
arr[j]
. - Inside this loop, run another loop to check each other element in the array that could be
arr[i]
(wherearr[i] == 2 * arr[j]
). - Keeping track of already seen numbers could greatly improve the efficiency. For this purpose, employing a hash map (or hash set) to store numbers that have already been checked can be helpful because:
- You can look up the existence of the required doubled value (or half value, depending on which is smaller and which is already seen) in constant time.
- Continue this process throughout the array. If at any point, a valid
(i, j)
pair is found that satisfiesarr[i] == 2 * arr[j]
, returntrue
. - If the end of the array is reached without finding such a pair, then return
false
.
This method ensures that each potential pair is checked efficiently, respecting the constraints provided, which specify an array length up to 500 elements, ensuring the approach will run effectively within these bounds.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool hasPairWithDouble(vector<int>& nums) {
unordered_map<int, int> countMap;
// Populate counts of each number
for (int value : nums) {
countMap[value]++;
}
for (int value : nums) {
// Check if there's a double of the current element
if (value != 0 && countMap.find(value * 2) != countMap.end()) {
return true;
}
// Special case for zero to have two occurrences
if (value == 0 && countMap[value] > 1) {
return true;
}
}
return false;
}
};
The provided C++ solution aims to determine if there exists a pair of elements in an integer array where one element is double the value of another.
- Initialize an
unordered_map
to count occurrences of each element in the array. - Iterate through the array to populate this map with counts.
- Iterate through the array again and perform two checks for every number:
- If the number is not zero, check if its double exists in the map.
- If the number is zero, check if it appears at least twice (since zero doubled is still zero).
This method ensures that you effectively identify any pair where one number is twice as large as its counterpart. Using a hash map allows for constant time complexity look-up operations, making the check efficient even for larger arrays. If either condition is satisfied during the loop, the function returns true, otherwise it completes its iteration and returns false, indicating no such pair exists.
class Solution {
public boolean checkDoubleExists(int[] elements) {
Map<Integer, Integer> occurrences = new HashMap<>();
for (int element : elements) {
occurrences.put(element, occurrences.getOrDefault(element, 0) + 1);
}
for (int element : elements) {
if (element != 0 && occurrences.containsKey(element * 2)) {
return true;
}
if (element == 0 && occurrences.get(element) > 1) {
return true;
}
}
return false;
}
}
This Java solution checks if any integer N
in the given array and its double 2N
exist within the same array. The key steps of the method checkDoubleExists
in the Solution
class follow:
- Create a map
occurrences
to store each integer from the array as a key and its frequency as a value. - Iterate through the array, updating the
occurrences
map with each element's frequency. - Re-iterate through the array. During each iteration:
- If the current element is not zero and the map contains the key
element * 2
, returntrue
. - If the current element is zero and its frequency in the map is greater than one, return
true
.
- If the current element is not zero and the map contains the key
- If no such pair
(N, 2N)
is found after evaluating all elements, returnfalse
.
This approach efficiently identifies the presence of an element and its double by leveraging a hashmap to store and retrieve element frequencies quickly. The solution ensures all cases, including the zero-double condition, are correctly handled.
class Solution:
def checkIfExists(self, numbers: List[int]) -> bool:
number_map = {}
# Record each number's occurrences in the list
for value in numbers:
number_map[value] = number_map.get(value, 0) + 1
for value in numbers:
# Check for a value's double
if value != 0 and 2 * value in number_map:
return True
# Special check for zero (must be more than one zero)
if value == 0 and number_map[value] > 1:
return True
return False
This Python solution addresses the problem of checking whether an array called numbers
contains a number N
for which 2*N
(its double) also exists in the array, with a special condition for the number zero.
The approach utilizes a dictionary number_map
to store the frequency of each element as the program iterates through the list. Here's how the solution is structured:
Initialize an empty dictionary
number_map
.Loop through each number in
numbers
:- Update
number_map
with the count of each number using a standard map-get pattern, where the default is zero if the number does not exist.
- Update
Loop through the
numbers
again to check for the problem condition:- If the current number is not zero and there exists a double of this number in the dictionary (
2 * value
), returnTrue
. - Check specifically for zero: if the count of zero in
number_map
is greater than one, meaning there is more than one zero, returnTrue
.
- If the current number is not zero and there exists a double of this number in the dictionary (
If no such pair (N and 2*N, or two zeros) is found by the end of the loop, return
False
.
This method efficiently checks the necessary condition by leveraging dictionary lookups, which are on average O(1) in time complexity, ensuring that the solution remains efficient even for large lists.
No comments yet.