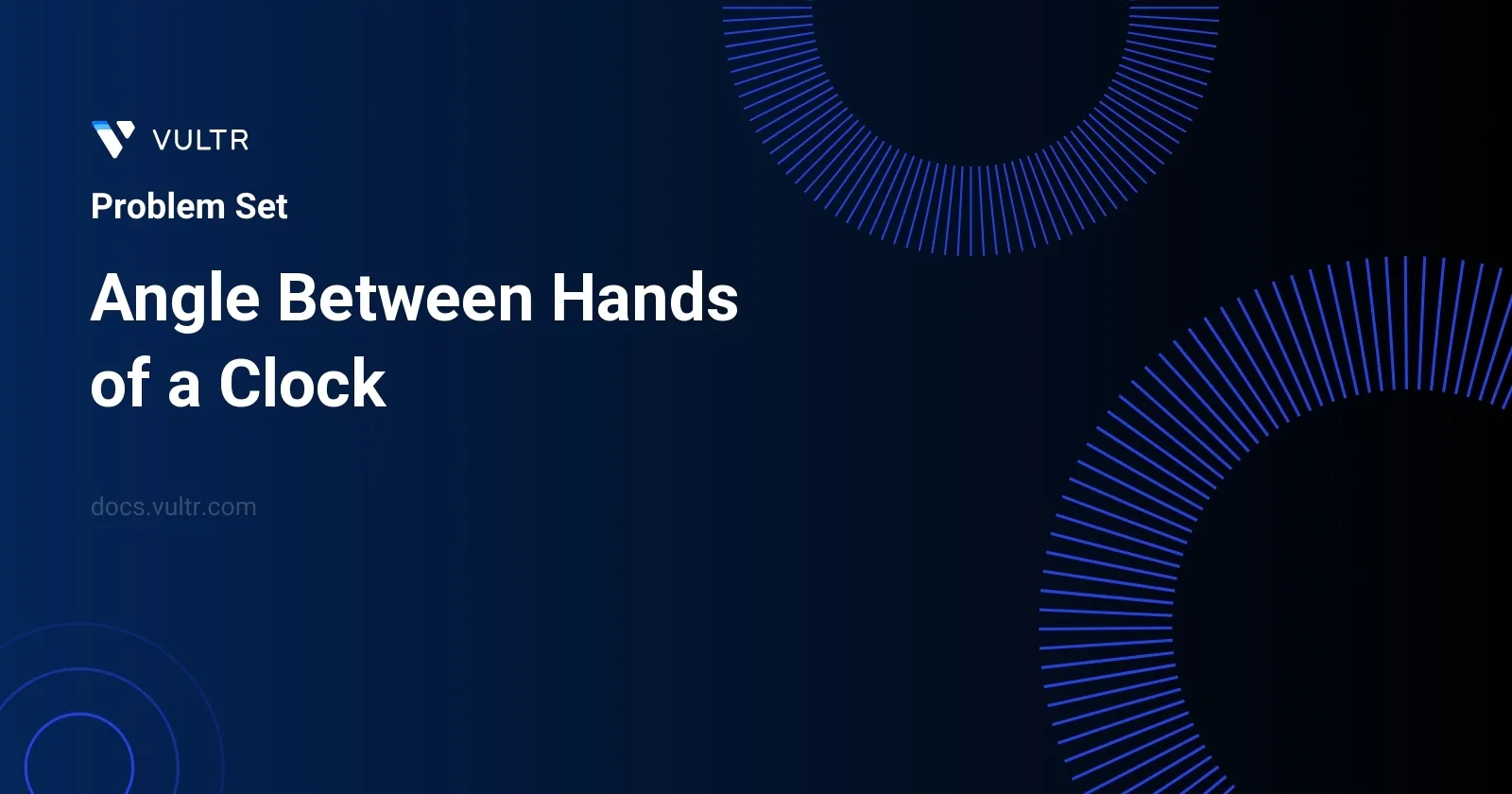
Problem Statement
The challenge involves calculating the smaller angle in degrees between the hour and minute hands on a traditional clock based on given "hour" and "minutes" input values. The objective is to determine the lesser of the two potential angles that could be formed from the 12 o'clock position. This calculation must consider the continuous movement of the hour hand as minutes pass, which also influences the hour hand's position relative to the minute hand. Accuracy is essential, as the result must be within a very small tolerance of the exact angle.
Examples
Example 1
Input:
hour = 12, minutes = 30
Output:
165
Example 2
Input:
hour = 3, minutes = 30
Output:
75
Example 3
Input:
hour = 3, minutes = 15
Output:
7.5
Constraints
1 <= hour <= 12
0 <= minutes <= 59
Approach and Intuition
Calculating the smaller angle between the hour and minute hands on a clock involves understanding the movement dynamics of these hands:
- Minute Hand Movement: The minute hand moves by 360 degrees over 60 minutes, which is 6 degrees per minute.
- Hour Hand Movement: The hour hand, covering 360 degrees over 12 hours, moves 30 degrees per hour. In addition, it progresses further as the minutes pass, at a rate of 0.5 degrees per minute (since 30 degrees/hour over 60 minutes/hour equals 0.5 degrees/minute).
Steps to Compute the Angle
- Calculate Minute Hand Angle: This is simply 6 degrees multiplied by the number of minutes past the hour.
- Calculate Hour Hand Angle: This is 30 degrees multiplied by the hour, plus 0.5 degrees multiplied by the minutes.
- Compute the Difference: The absolute difference between the angles gives the larger of the two possible angles formed.
- Determine the Smaller Angle: Since a full circle is 360 degrees, subtract the larger angle from 360 if the initial difference is greater than 180 degrees, as the hands could form a smaller angle going the other way round the dial.
These steps will yield the smaller of the two possible angles between the hour and minute hands of a clock, adjusted correctly for the movement of both hands, while considering only valid input ranges based on clock mechanics.
Solutions
- C++
- Java
- Python
class Solution {
public:
double findClockAngle(int h, int m) {
int anglePerMinute = 6;
int anglePerHour = 30;
double minutesPosition = anglePerMinute * m;
double hoursPosition = (h % 12 + m / 60.0) * anglePerHour;
double angleDifference = abs(hoursPosition - minutesPosition);
return min(angleDifference, 360 - angleDifference);
}
};
Calculate the angle between the hour and minute hands of a clock using C++. First, define constants for the degrees that each minute and hour contribute to the respective positions of the hands. Compute the position of the minute hand by multiplying the minutes by degrees per minute. Calculate the hours hand's position by taking the hour modulo 12, adjusting for the fraction of the hour passed by minutes, and then multiplying by degrees per hour. Determine the absolute difference between the two positions for the angle. Return the smaller angle derived by considering the full circle of 360 degrees if needed. This approach ensures that the resulting angle is the minimal angle between two hands at any given time of the clock.
class ClockAngleCalculator {
public double calculateAngle(int hour, int minute) {
int anglePerMinute = 6;
int anglePerHour = 30;
double minuteHandAngle = anglePerMinute * minute;
double hourHandAngle = (hour % 12 + minute / 60.0) * anglePerHour;
double angleDifference = Math.abs(hourHandAngle - minuteHandAngle);
return Math.min(angleDifference, 360 - angleDifference);
}
}
The Java solution provided calculates the angle between the hour and minute hands of a clock based on the hour and minute input values.
- Define a class named
ClockAngleCalculator
. - A method called
calculateAngle(int hour, int minute)
calculates the angle.- Initialize
anglePerMinute
as 6 degrees. - Initialize
anglePerHour
as 30 degrees.
- Initialize
- Compute the position of the minute hand in degrees:
minuteHandAngle = anglePerMinute * minute
. - Calculate the position of the hour hand in degrees:
hourHandAngle = (hour % 12 + minute / 60.0) * anglePerHour
to include minor adjustments as the hour hand moves with the minute hand. - Determine the absolute difference between both hand angles:
angleDifference = Math.abs(hourHandAngle - minuteHandAngle)
. - Return the smaller angle between the direct angle and its complementary angle:
Math.min(angleDifference, 360 - angleDifference)
.
This method accurately provides the smallest angle between the hands of a clock at any given time, represented in degrees.
class Solution:
def clockAngle(self, hour: int, minutes: int) -> float:
angle_per_minute = 6
angle_per_hour = 30
minute_hand_angle = angle_per_minute * minutes
hour_hand_angle = (hour % 12 + minutes / 60) * angle_per_hour
angle_difference = abs(hour_hand_angle - minute_hand_angle)
return min(angle_difference, 360 - angle_difference)
Calculate the angle between the hour hand and minute hand of a clock using Python. The function clockAngle
provides the angle considering the inputs for hour and minutes. The solution takes advantage of the fact that the minute hand moves 6 degrees per minute and the hour hand moves 30 degrees per hour.
- Calculate the minute hand's position using the formula
minute_hand_angle = angle_per_minute * minutes
. - For the hour hand's position, adjust the hour to a 12-hour format using modulo and account for the partial advancement due to minutes:
hour_hand_angle = (hour % 12 + minutes / 60) * angle_per_hour
. - Determine the absolute difference between both hands:
angle_difference = abs(hour_hand_angle - minute_hand_angle)
. - Return the smaller of the two possible angles on a clock using:
min(angle_difference, 360 - angle_difference)
.
This function effectively provides the smallest angle formed between the two clock hands for any given time, ensuring it handles overlaps and the circle's 360-degree nature accurately.
No comments yet.