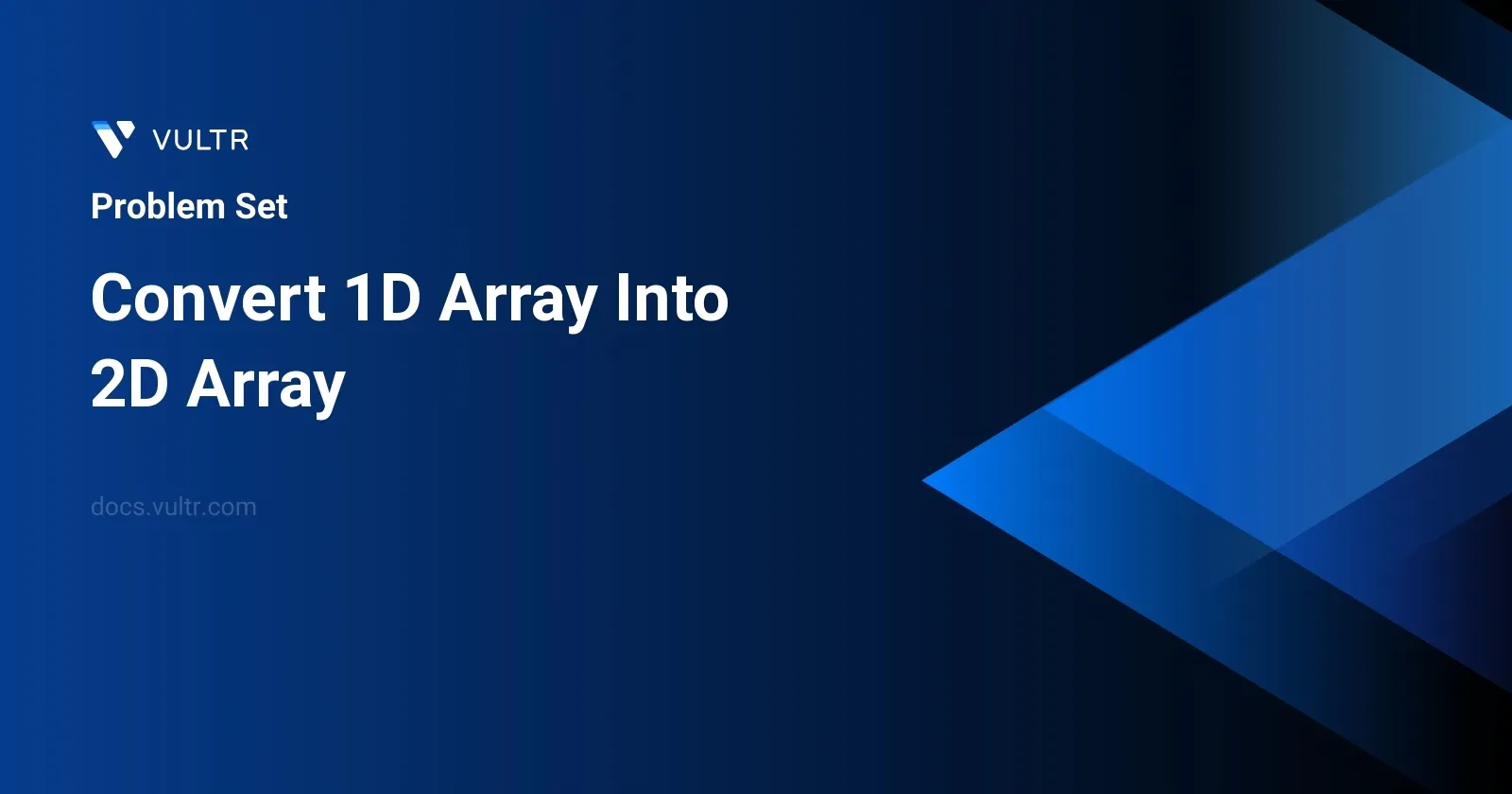
Problem Statement
Suppose you have been provided with a one-dimensional (1D) integer array named original
and two integers, m
(the number of rows) and n
(the number of columns). Your objective is to construct a two-dimensional (2D) array using all the elements from the original
array. Each row in the resulting 2D array should sequentially take n
elements from the original
array based on its indices, starting with indices 0
to n-1
for the first row, then n
to 2*n-1
for the second row, and so forth until all elements are used. You need to ensure that the total number of elements required to fill the 2D array (m*n
) matches the length of original
. If it is not possible to fill the array completely and exactly, you are required to return an empty 2D array.
Examples
Example 1
Input:
original = [1,2,3,4], m = 2, n = 2
Output:
[[1,2],[3,4]]
Explanation:
The constructed 2D array should contain 2 rows and 2 columns. The first group of n=2 elements in original, [1,2], becomes the first row in the constructed 2D array. The second group of n=2 elements in original, [3,4], becomes the second row in the constructed 2D array.
Example 2
Input:
original = [1,2,3], m = 1, n = 3
Output:
[[1,2,3]]
Explanation:
The constructed 2D array should contain 1 row and 3 columns. Put all three elements in original into the first row of the constructed 2D array.
Example 3
Input:
original = [1,2], m = 1, n = 1
Output:
[]
Explanation:
There are 2 elements in original. It is impossible to fit 2 elements in a 1x1 2D array, so return an empty 2D array.
Constraints
1 <= original.length <= 5 * 104
1 <= original[i] <= 105
1 <= m, n <= 4 * 104
Approach and Intuition
The total number of elements in the input list (original
) must be exactly equal to the product of m
and n
for a valid transformation. If this condition is not met, an immediate return of an empty array is warranted. Here's how you can conceptualize your approach:
Check for Valid Input Dimension: Start by verifying if the total count of elements, when grouped into
n
elements per group, equals exactlym
rows. This is a preliminary check to see if a complete and valid 2D array can be constructed.Construct the 2D Array: Provided the preliminary check is successful, process the
original
array:- Create groups of
n
elements for each of them
rows. - Iterate through the original array and slice it into segments of
n
elements; - Append each segment as a row into your 2D array.
- Create groups of
Return the Result: After processing the complete list, the result should be a 2D array that conforms to the defined row (
m
) and column (n
) structure. If there was a size mismatch, you should have already returned an empty array before this step.
- Example Overview: For example, given
original = [1,2,3,4]
andm = 2, n = 2
, the method ensures 4 elements can exactly fill a2 x 2
array. It forms the first row with the first two elements[1,2]
and the second row with the next two[3,4]
.
This approach is direct and primarily takes advantage of Python's capabilities to slice lists and handle basic conditional checks efficiently. Compliance with the constraints ensures no overflow or excessive computations.
Solutions
- C++
- Java
- Python
class Solution {
public:
vector<vector<int>> createMatrix(vector<int>& inputVector, int rows, int cols) {
// Return an empty matrix if the total number of elements does not match with rows*cols
if (rows * cols != inputVector.size()) {
return {};
}
// Prepare a matrix with proper dimensions
vector<vector<int>> matrix(rows, vector<int>(cols));
// Populate the matrix using elements from inputVector
for (int idx = 0; idx < inputVector.size(); idx++) {
matrix[idx / cols][idx % cols] = inputVector[idx];
}
return matrix;
}
};
This article describes the solution to convert a one-dimensional vector into a two-dimensional matrix using C++. The provided C++ function named createMatrix
takes three parameters:
inputVector
- a reference to a 1D vector of integers.rows
- the desired number of rows in the resulting 2D matrix.cols
- the desired number of columns in the resulting 2D matrix.
To achieve the transformation, follow these steps:
- Confirm whether the product of
rows
andcols
equals the size of theinputVector
. If not, return an empty matrix as the dimensions do not allow a perfect reshaping without losing or truncating data. - Initialize a 2D matrix
matrix
with dimensions specified byrows
andcols
. - Populate this matrix by iterating over
inputVector
. For each element ininputVector
, calculate the relevant row index as integer division ofidx
(current index) bycols
and column index as the remainder of dividingidx
bycols
. Assign the value from the 1DinputVector
to the correct position in the 2D matrix using these indices. - After looping through all elements, return the populated matrix.
The function handles edge cases like mismatched dimensions by returning an empty matrix, ensuring the integrity of data conversion is preserved regardless of input scenarios. Therefore, you have a robust, reusable function for matrix transformations in C++.
class Solution {
public int[][] buildMatrix(int[] sourceArray, int rows, int cols) {
if (rows * cols != sourceArray.length) {
return new int[0][0];
}
int[][] matrix = new int[rows][cols];
for (int index = 0; index < sourceArray.length; index++) {
matrix[index / cols][index % cols] = sourceArray[index];
}
return matrix;
}
}
This Java solution converts a 1D array into a 2D array based on specified row and column parameters. Ensure the product of the rows and columns matches the length of the 1D array; otherwise, the method returns an empty 2D array.
- Start by checking if the product of
rows
andcols
equals the length ofsourceArray
. If not, return an empty matrix to indicate invalid dimensions. - Initialize a 2D array,
matrix
, of size[rows][cols]
. - Iterate over the
sourceArray
, converting 1D index positions to 2D positions withinmatrix
. Use integer division and modulus operations to determine the correct row and column for each element. - Finally, return the populated
matrix
.
This method efficiently maps the elements from a 1D array to a 2D matrix using direct index manipulation, ensuring optimal performance with minimal overhead.
class Solution:
def createMatrix(self, flat_list: list[int], rows: int, cols: int) -> list[list[int]]:
if rows * cols != len(flat_list):
return []
matrix = [[0] * cols for _ in range(rows)]
for idx in range(len(flat_list)):
r, c = divmod(idx, cols)
matrix[r][c] = flat_list[idx]
return matrix
The provided Python code resolves the task of converting a one-dimensional list into a two-dimensional array or matrix, given specific dimensions for rows and columns.
The primary function createMatrix takes three parameters:
flat_list
as the list of integers,rows
as the intended number of rows,cols
as the intended number of columns.
Initially, it checks if the product of the rows and columns matches the length of
flat_list
. If it does not, an empty list is returned signaling an error or incompatibility in inputs.The function then initializes a matrix of zeros with dimensions defined by the number of rows and columns.
A loop then iterates through each element in
flat_list
where:- The current index is converted into row and column indices using the divmod function.
- The value from the
flat_list
is placed into the matrix at the respective [row, column] location.
Finally, the filled matrix is returned, providing a two-dimensional representation of the input list. This process requires both functional and loop-based operations tailored to the nature and size of
flat_list
as well as the desired matrix dimensions.
No comments yet.