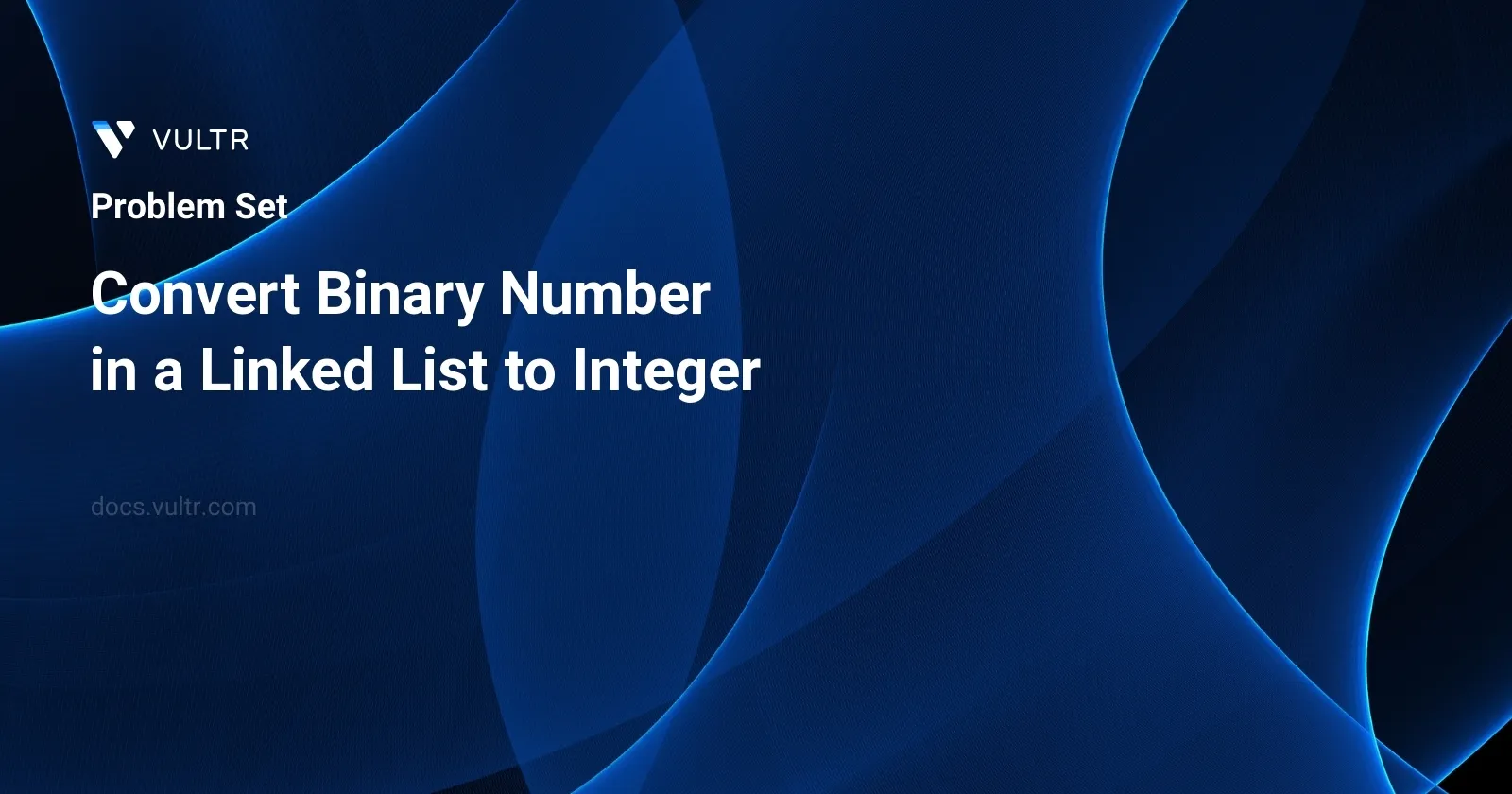
Problem Statement
In this task, you are provided with the head node of a singly-linked list that represents a binary number with each node containing a binary digit (either '0' or '1'). The binary number is given such that the most significant bit (MSB) is at the head of the list, and this list structure deciphers the binary number from left to right, which is how we generally read numbers. The objective is to convert this binary representation directly from the linked list format into its decimal (base 10) numeric form.
Examples
Example 1
Input:
head = [1,0,1]
Output:
5
Explanation:
(101) in base 2 = (5) in base 10
Example 2
Input:
head = [0]
Output:
0
Constraints
- The Linked List is not empty.
- Number of nodes will not exceed
30
. - Each node's value is either
0
or1
.
Approach and Intuition
Understanding how to approach the problem efficiently involves acknowledging a few key operations and concepts:
- Since each node can be traversed only once in a singly-linked list and we cannot access any node directly (except by traversing), the conversion process involves reading and processing each node sequentially from the head to the end.
- The most significant bit is at the head. This setup hints that if you start reading the linked list from the head, each subsequent node simply needs to be considered as the next least significant bit in the binary number.
- As you read each node's value, you can build up the decimal value step-by-step. This can be accomplished efficiently using bit manipulation - specifically bit shifting.
Here's a visual breakdown using the examples provided:
For example 1 (input
head = [1,0,1]
):- Start with an initial value
0
(let's call itnum
). - Read the first node (value
1
), updatenum
to1
(num = 1 << 1 | 1
which simplifies tonum = 1
). - Read the second node (value
0
), no change in value since0
OR with any number remains unchanged (num = 1 << 1 | 0
equals2
, but OR with0
does not alter it). - Read the third node (value
1
), updatenum
to5
(num = 2 << 1 | 1
which equals5
).
- Start with an initial value
For example 2 (input
head = [0]
):- Start with initial value
0
. - Only one node with value
0
, so the resulting decimal value remains0
.
- Start with initial value
This conversion process is systematic and constant regardless of the binary number's length (as constrained by a maximum of 30 nodes), ensuring efficient computational time. Leveraging bitwise operations for constructing the number, as you traverse the linked list, gives a clear and direct approach to solving the problem.
Solutions
- Java
- Python
class Solution {
public int convertBinaryToDecimal(ListNode node) {
int decimal = node.val;
while (node.next != null) {
decimal = (decimal << 1) | node.next.val;
node = node.next;
}
return decimal;
}
}
The solution for converting a binary number represented by a linked list into an integer in Java involves the following approach:
Initialize an integer
decimal
with the value of the first node in the linked list.Use a
while
loop to traverse through the linked list. As long as thenext
node of the current node is notnull
, execute the following steps:Use bitwise left shift operation on
decimal
to make space for the next binary digit and then use bitwise OR operation to add the value of the next node todecimal
.Move to the next node in the linked list.
Return the
decimal
value once all nodes have been processed.
This method leverages bitwise operations to assemble the binary number from individual digits stored in nodes as it traverses the linked list. The solution ensures efficient processing with a clear, logical flow from start to finish.
class Solution:
def binaryToDecimal(self, node: ListNode) -> int:
binary_value = node.val
while node.next:
binary_value = (binary_value << 1) | node.next.val
node = node.next
return binary_value
The provided solution in Python converts a binary number represented by a linked list into its integer form. Each node in the linked list contains a single digit (0 or 1) of the binary number, starting from the most significant bit (MSB) at the head of the list.
Here’s how the solution works:
- Initialize
binary_value
with the value of the first node. - Traverse the linked list starting from the head. For each node:
- Shift
binary_value
left by 1 bit to make space for the next bit. - Use the bitwise OR operation to add the next node’s value to
binary_value
. - Move to the next node in the list.
- Shift
- Continue until all nodes are processed.
- Return
binary_value
, which now represents the decimal value of the binary number.
The operations like shifting (<<
) and bitwise OR (|
) efficiently construct the decimal number from its binary representation. The use of bitwise operations ensures that the conversion is both time and space efficient, leveraging bit manipulation directly to form the integer.
No comments yet.