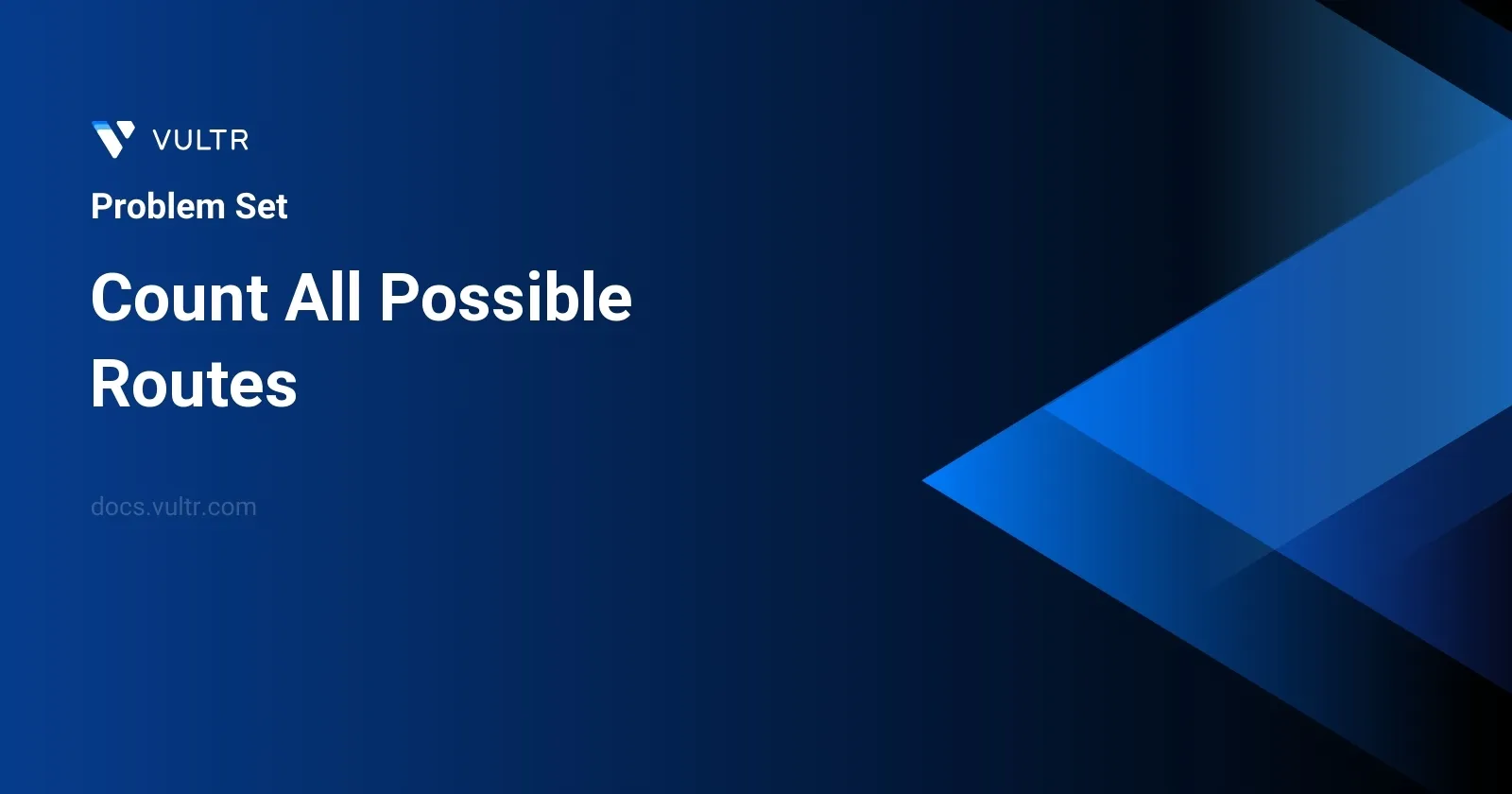
Problem Statement
The task involves an array locations
of distinct positive integers. Each integer locations[i]
corresponds to the position of city i
. With you having a specified start
city, finish
city, and an initial amount of fuel, you need to determine all the possible routes from the starting city to the finishing city without the fuel becoming negative. The critical dynamics of moving between cities include:
- You can travel between any two distinct cities.
- The fuel cost for traveling between two cities
i
andj
is determined by the absolute difference in their positions:|locations[i] - locations[j]|
. - If you run out of fuel (fuel goes negative), that route is invalid.
- Cities can be revisited multiple times in seeking valid routes from
start
tofinish
.
You should calculate the total number of valid routes and return this number modulo 10^9 + 7
to handle potentially large numbers.
Examples
Example 1
Input:
locations = [2,3,6,8,4], start = 1, finish = 3, fuel = 5
Output:
4
Explanation:
The following are all possible routes, each uses 5 units of fuel: 1 -> 3 1 -> 2 -> 3 1 -> 4 -> 3 1 -> 4 -> 2 -> 3
Example 2
Input:
locations = [4,3,1], start = 1, finish = 0, fuel = 6
Output:
5
Explanation:
The following are all possible routes: 1 -> 0, used fuel = 1 1 -> 2 -> 0, used fuel = 5 1 -> 2 -> 1 -> 0, used fuel = 5 1 -> 0 -> 1 -> 0, used fuel = 3 1 -> 0 -> 1 -> 0 -> 1 -> 0, used fuel = 5
Example 3
Input:
locations = [5,2,1], start = 0, finish = 2, fuel = 3
Output:
0
Explanation:
It is impossible to get from 0 to 2 using only 3 units of fuel since the shortest route needs 4 units of fuel.
Constraints
2 <= locations.length <= 100
1 <= locations[i] <= 109
- All integers in
locations
are distinct. 0 <= start, finish < locations.length
1 <= fuel <= 200
Approach and Intuition
To solve this problem, we need to traverse the potential paths from the start city to the finish city, being mindful of fuel constraints and the unique costs associated with moving between cities. Here's a condensed approach considering given constraints:
Understanding Movement: You move from a city
i
to a cityj
and this costs you some fuel calculated by the absolute difference in their coordinates in thelocations
array.Modulo Operation's Role: Given the constraints, solutions might involve a large number of routes. To manage this, the result needs to be returned modulo
10^9 + 7
.Exploring Routes: Starting from your initial city and fuel, determine possible moves. You should consider each city that can be reached with the remaining fuel and recursively calculate the potential routes from there.
Utilizing Recursion with Memoization:
- Use a recursive function to explore each possible move, diminishing the fuel correspondingly.
- Employ memoization to store results of specific states (current city and remaining fuel) to avoid recalculating them and reduce computation time.
Base Case and Modulus Returns:
- If you're out of fuel or trying to move results in negative fuel, this state isn't valid.
- If you reach the destination
finish
and still have non-negative fuel, this state contributes to one possible route.
Insights from Examples: From the provided examples, scenarios vary from multiple potential paths using exact fuel amounts to no possible paths due to fuel constraints. Mapping out these scenarios helps contextualize the problem and solution path.
This approach will allow the efficient exploration of routes while keeping the computation feasible within the problem's constraints.
Solutions
- C++
- Java
- Python
class Solution {
public:
int routeCounter(vector<int>& locs, int origin, int destination, int maxFuel) {
int count = locs.size();
vector<vector<int>> memo(count, vector<int>(maxFuel + 1));
for(int f = 0; f <= maxFuel; f++) {
memo[destination][f] = 1;
}
int result = 0;
for (int fuel = 0; fuel <= maxFuel; fuel++) {
for (int current = 0; current < count; current++) {
for (int next = 0; next < count; next++) {
if (next == current) {
continue;
}
int cost = abs(locs[current] - locs[next]);
if (cost <= fuel) {
memo[current][fuel] = (memo[current][fuel] + memo[next][fuel - cost]) % 1000000007;
}
}
}
}
return memo[origin][maxFuel];
}
};
The provided C++ solution introduces a method to count all possible routes from a given origin to a destination using a vehicle with a specified amount of fuel. The approach utilizes dynamic programming to solve the problem efficiently.
- Start by defining a
routeCounter
function that accepts a vector of locations, an origin index, a destination index, and the maximum fuel available. - Establish a 2D
memo
vector wherememo[i][j]
represents the number of ways to reach locationi
withj
units of fuel remaining. - Initialize the memoization table by setting all ways to reach the destination with any fuel level to
1
, since reaching the destination with any fuel left is a valid route. - Use a triple nested loop to calculate the number of ways to reach each location with varying amounts of fuel:
- The outer loop iterates over the fuel levels from
0
tomaxFuel
. - The second loop iterates over all possible current locations.
- The inner loop iterates over all potential next locations, making sure to avoid the current location itself.
- For each valid move (where the fuel cost to move to the next location does not exceed the current fuel level), update the
memo
table to add the count of possible ways to get to the next location after subtracting the fuel cost.
- The outer loop iterates over the fuel levels from
- Ensure results remain manageable by applying modulo
1000000007
to each computation. - Ultimately, return
memo[origin][maxFuel]
, which signifies the total number of ways to reach the destination from the origin withmaxFuel
units of fuel available based on the computedmemo
table.
This solution effectively uses memoization to break down the problem of counting routes into manageable subproblems, each representing a state defined by the current location and the remaining fuel. The computation of each state's value depends only on previously solved states, ensuring efficiency and correctness.
class Solution {
public int calculateRoutes(int[] locs, int strt, int end, int f) {
int len = locs.length;
int routes[][] = new int[len][f + 1];
Arrays.fill(routes[end], 1);
int result = 0;
for (int j = 0; j <= f; j++) {
for (int i = 0; i < len; i++) {
for (int k = 0; k < len; k++) {
if (k == i) {
continue;
}
if (Math.abs(locs[i] - locs[k]) <= j) {
routes[i][j] = (routes[i][j] + routes[k][j - Math.abs(locs[i] - locs[k])]) %
1000000007;
}
}
}
}
return routes[strt][f];
}
}
The Java solution detailed above is designed to count all possible routes one can take from a starting point (strt
) to a destination (end
) given a number of locations and a fuel limit (f
). This is implemented in the calculateRoutes
method inside the Solution
class. Here's a breakdown of the method:
Start by determining the number of locations (
len
) and initialize a 2D arrayroutes
to store the interim results. The array dimensions are based on the number of locations and the fuel capacity plus one.Initially, set all possible fuel states at the destination (
end
) to 1 because reaching the destination with any remaining fuel is a valid scenario.Utilize a nested loop structure to compute the possibilities. The outermost loop iterates over each fuel state from 0 to
f
. For each fuel state:- Loop through each location
i
.- For each location
i
, consider each other locationk
.- Skip the iteration where
k
equalsi
. - Calculate the fuel required to move from location
k
toi
. If it's within the available fuel (j
), updateroutes[i][j]
. The modulo operation ensures that the results stay within the limit of1000000007
to avoid overflow issues.
- Skip the iteration where
- For each location
- Loop through each location
After populating the
routes
array, the solution for the givenstrt
position and fuelf
can be directly accessed and returned fromroutes[strt][f]
.
This approach efficiently handles the problem using dynamic programming by building off the number of routes from one location to another based on available fuel, which in turn saves significantly on computational resources and time compared to a naive recursive approach.
class PathFinder:
def calculateRoutes(self, locs: List[int], init: int, goal: int, maxFuel: int) -> int:
totalLocs = len(locs)
route = [[0] * (maxFuel + 1) for _ in range(totalLocs)]
for f in range(maxFuel + 1):
route[goal][f] = 1
for fuelLeft in range(maxFuel + 1):
for currentPosition in range(totalLocs):
for altPosition in range(totalLocs):
if altPosition == currentPosition:
continue
fuelCost = abs(locs[currentPosition] - locs[altPosition])
if fuelCost <= fuelLeft:
route[currentPosition][fuelLeft] = (route[currentPosition][fuelLeft] + route[altPosition][fuelLeft - fuelCost]) % 1000000007
return route[init][maxFuel]
The solution provided calculates all possible routes from an initial location to a goal location within a given amount of fuel. The implementation uses dynamic programming in Python. Here is a breakdown of the solution:
Define a class
PathFinder
with a methodcalculateRoutes
.Variables:
locs
: List of integers representing different locations.init
: Starting location index.goal
: Goal location index.maxFuel
: Maximum fuel available for the journey.
An auxiliary matrix
route
is initialized to track the number of possible routes ending at each location with varying amounts of fuel left.The dynamic programming transition involves:
- Setting base cases where reaching the goal location with any fuel amount is always 1 possible route.
- Iterating over each amount of leftover fuel and each current position.
- For each current position, considering alternative positions that could be moved to by evaluating the fuel cost between them.
- Updating the count of routes to the current position based on reachable alternative positions and the fuel costs.
Results are fetched from the
route
matrix for the initial location and the maximum fuel provided, giving the total possible routes.
The solution handles the problem efficiently using limited space and ensuring calculations are modular with a large constant to prevent overflow. This approach guarantees the accurate counting of routes under given constraints.
No comments yet.