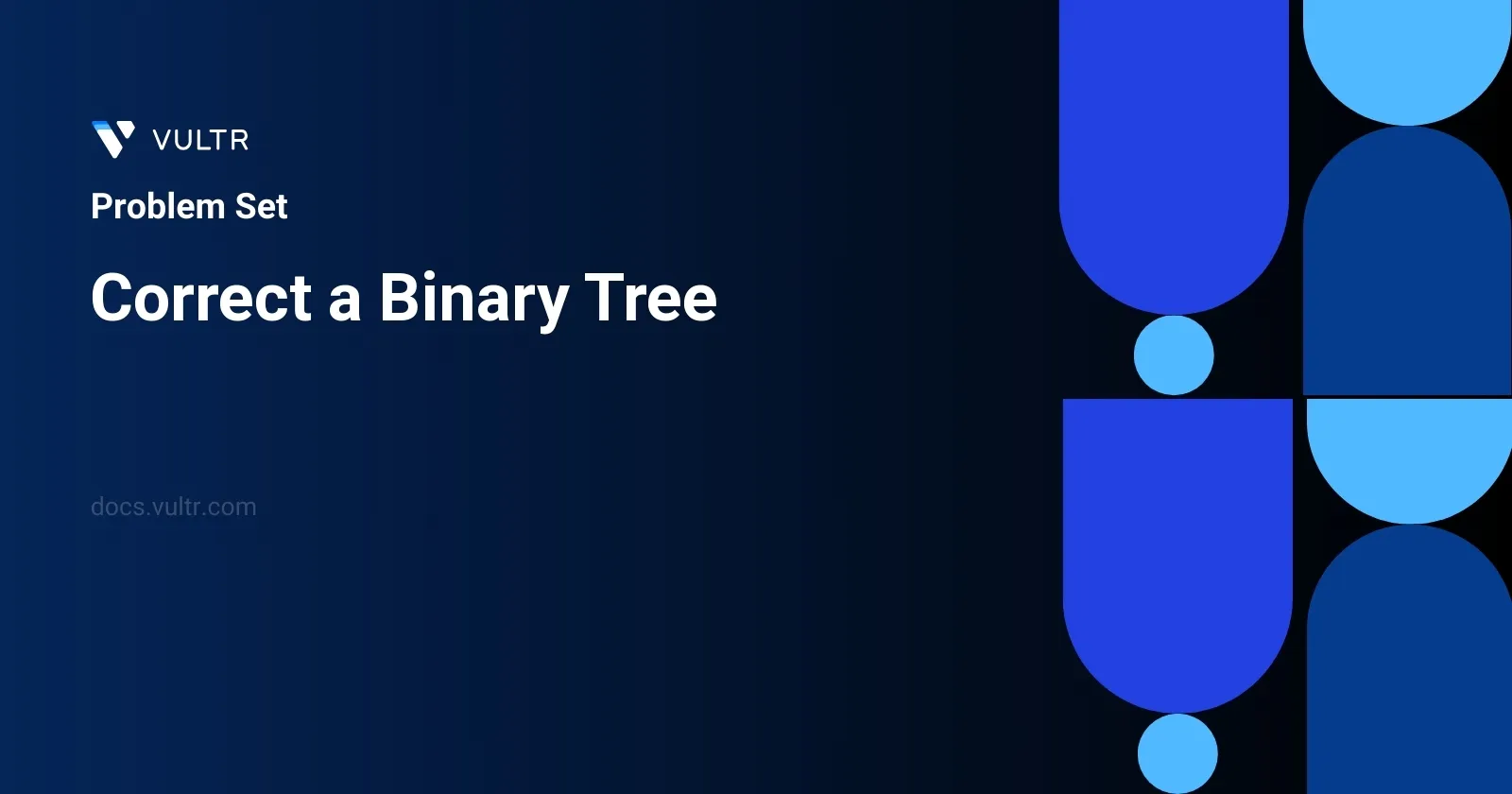
Problem Statement
In this problem, we are given a binary tree that contains a specific defect: there is one node (referred to as fromNode
) whose right child incorrectly points to another node (toNode
) that exists at the same depth but on its right. This scenario is anomalous as it defies the typical structure of a binary tree where each node can only have children directly beneath it.
The task is to modify this tree such that the subtree rooted at the defective fromNode
, including all its descendants, is removed from the tree. However, the node to which it incorrectly points (toNode
) should remain untouched, along with the rest of the tree. This ensures we correct the structure while preserving as much of the original tree as possible.
Examples
Example 1
Input:
root = [1,2,3], fromNode = 2, toNode = 3
Output:
[1,null,3]
Explanation:
The node with value 2 is invalid, so remove it.
Example 2
Input:
root = [8,3,1,7,null,9,4,2,null,null,null,5,6], fromNode = 7, toNode = 4
Output:
[8,3,1,null,null,9,4,null,null,5,6]
Explanation:
The node with value 7 is invalid, so remove it and the node underneath it, node 2.
Constraints
- The number of nodes in the tree is in the range
[3, 104]
. -109 <= Node.val <= 109
- All
Node.val
are unique. fromNode != toNode
fromNode
andtoNode
will exist in the tree and will be on the same depth.toNode
is to the right offromNode
.fromNode.right
isnull
in the initial tree from the test data.
Approach and Intuition
First, we need to identify the invalid node (
fromNode
), which has an incorrect right child pointing to another node on the same level but to its right (toNode
).As we traverse the tree, we maintain a record of nodes visited at each depth using a set or dictionary. This will help in detecting when a node’s right child is already present in the set of that particular depth, indicating the existence of the incorrect linkage.
To detect the invalid node, during each step of the tree traversal (typically a breadth-first search (BFS) to ensure level-order access), check if the right child of the current node is a node that exists in our depth-tracking structure. If so, this node is our
fromNode
.Once the invalid node is identified, modify the tree by removing this node and all nodes beneath it, while ensuring not to remove the node that was incorrectly pointed to (
toNode
).The traversal and modification should be carefully implemented to ensure that the tree’s integrity and node relationships outside the defective structure are maintained.
By following the above steps, we correct the binary tree consistently according to the problem's requirements. This methodology leverages the unique constraints specified, such as the position relationship between fromNode
and toNode
and their presence at the same depth.
Solutions
- C++
- Java
- JavaScript
- Python
class Solution {
public:
TreeNode* modifyTree(TreeNode* root) {
// BFS processing queue, keeps node along with its parent
queue<pair<TreeNode*, TreeNode*>> bfsQueue;
bfsQueue.push({root, nullptr});
// Process tree level-by-level
while (!bfsQueue.empty()) {
// Get number of nodes at current level
int levelSize = bfsQueue.size();
// Current level nodes tracking
unordered_set<TreeNode*> currentLevelNodes;
// Handle each node at this level
for (int idx = 0; idx < levelSize; ++idx) {
// Retrieve node and its parent from queue
auto [currentNode, parentNode] = bfsQueue.front();
bfsQueue.pop();
// Check if right child is already processed
if (currentLevelNodes.count(currentNode->right)) {
// Correct tree by removing defective node
if (parentNode->left == currentNode) {
parentNode->left = nullptr;
} else {
parentNode->right = nullptr;
}
return root;
}
// Mark current node as visited
currentLevelNodes.insert(currentNode);
// Enqueue children for next level exploration
if (currentNode->right) {
bfsQueue.push({currentNode->right, currentNode});
}
if (currentNode->left) {
bfsQueue.push({currentNode->left, currentNode});
}
}
}
// Return modified root
return root;
}
};
This C++ solution implements a function to correct a binary tree by using Breadth-First Search (BFS). It identifies and removes any tree nodes that are incorrectly duplicated within the same level of the tree.
Follow the detailed breakdown of how the function works:
- A queue is utilized to manage the BFS process, storing each tree node alongside its parent.
- The algorithm iterates through each tree level:
- It determines the number of nodes in the current level.
- For each node:
- It retrieves the node and its parent.
- If the current node's right child is a duplicate (already visited on the same level), the tree is corrected by removing the connection from the parent node to this current node.
- The function returns the corrected tree root if a correction is made.
- Both children of the current node are added to the queue for further processing in the next level.
- If the loop completes without any corrections, the original root of the tree is returned.
- The approach ensures that any node that is a duplicate within the same level is removed, effectively correcting the tree structure.
class Solution {
public TreeNode fixBinaryTree(TreeNode root) {
// Queue to perform BFS traversal, holds node and its parent
Queue<TreeNode[]> nodeQueue = new LinkedList<>();
nodeQueue.add(new TreeNode[]{root, null});
while (!nodeQueue.isEmpty()) {
// Store number of nodes at current level
int levelSize = nodeQueue.size();
// HashSet to keep track of nodes at current level
Set<TreeNode> currentLevelNodes = new HashSet<>();
for (int i = 0; i < levelSize; i++) {
// Retrieve a node and its parent from queue
TreeNode[] nodePair = nodeQueue.poll();
TreeNode currentNode = nodePair[0], parentNode = nodePair[1];
// Check if the right child of the current node was already visited
if (currentLevelNodes.contains(currentNode.right)) {
// Correct binary tree by removing defective node
if (parentNode.left == currentNode) {
parentNode.left = null;
} else {
parentNode.right = null;
}
return root;
}
// Mark this node as visited
currentLevelNodes.add(currentNode);
// Queue the children for the next level
if (currentNode.right != null) {
nodeQueue.add(new TreeNode[]{currentNode.right, currentNode});
}
if (currentNode.left != null) {
nodeQueue.add(new TreeNode[]{currentNode.left, currentNode});
}
}
}
// Return root as the corrected binary tree
return root;
}
}
In this Java solution for correcting a binary tree, a BFS (Breadth First Search) is used to traverse the tree, while checking and correcting if any node's right child has already been visited at that level, indicating it is misplaced. Essential components are employed to accomplish this:
- Queue of TreeNode Arrays: Utilized for BFS traversal. Each element comprises of a node and its parent node reference. The queue is initially seeded with the root node and a null parent.
- HashSet for Current Level Nodes: Stores the nodes present at the current level. This set is used to identify if a right child of the node has already been added to the current level, which would indicate a defective structure.
During the traversal:
- Record the number of nodes at the current level with
levelSize
. - For each node, if the right child is detected as already visited (exists in the current level set), then the tree is corrected by setting the corresponding parent’s child (left or right depending on the position) to null.
- Furthermore, each node's left and right children are added to the queue if they exist, ensuring the node itself is marked as visited by adding it to the
currentLevelNodes
set.
Ultimately, if a correction is made, the modified tree’s root is returned. If not, the original root is returned, signifying no defects were present. This approach leverages the queue for level-wise node processing and a set to quickly check and respond to anomalies, maintaining an efficient handling of the tree structure.
var fixBinaryTree = function(rootNode) {
const bfsQueue = [[rootNode, null]];
while (bfsQueue.length) {
const levelNodeCount = bfsQueue.length;
const levelNodes = new Set();
for (let index = 0; index < levelNodeCount; index++) {
const [currentNode, parentNode] = bfsQueue.shift();
if (levelNodes.has(currentNode.right)) {
if (parentNode.left === currentNode) {
parentNode.left = null;
} else {
parentNode.right = null;
}
return rootNode;
}
levelNodes.add(currentNode);
if (currentNode.right) {
bfsQueue.push([currentNode.right, currentNode]);
}
if (currentNode.left) {
bfsQueue.push([currentNode.left, currentNode]);
}
}
}
return rootNode;
};
The solution provided addresses the task of correcting a binary tree that may have errors in node connections in JavaScript. The function fixBinaryTree
is implemented using Breadth-First Search (BFS) to ensure all levels of the tree are inspected for inconsistencies sequentially.
- Initialize a queue,
bfsQueue
, with the root node and anull
indicating no parent. - Utilize a loop to continue the search until the queue is empty.
- Within each iteration, determine the number of nodes at the current level using the
bfsQueue
length and create aSet
,levelNodes
, to keep track of the nodes at this level. - The loop through each node at this level allows for checking if a node’s right child already exists in the
levelNodes
, implying a false connection. - If a duplicate right child is detected, the erroneous connection is identified and removed by setting the appropriate child (left or right) of the parent node to
null
. - Push the left and right children of the current node into the
bfsQueue
if they exist, to explore in the next level. - The function finally returns the corrected
rootNode
.
This solution is efficient in identifying and correcting the first erroneous linkage found in a tree, thereby ensuring that the structure satisfies binary tree properties before proceeding further.
class Solution:
def fixTreeNode(self, treeRoot: TreeNode) -> TreeNode:
# Use deque for BFS where each item is [currentNode, parentNode]
bfsQueue = deque([[treeRoot, None]])
# Process each level of the tree
while bfsQueue:
# Current level node count
levelCount = len(bfsQueue)
# Set to keep track of nodes at the current level
currentLevelNodes = set()
# Process each node in the current level
for _ in range(levelCount):
# Dequeue the first node
currentNode, parentNode = bfsQueue.popleft()
# Check if the right child of the current node has been processed or not
if currentNode.right in currentLevelNodes:
# Disconnect the defective node
if parentNode.left == currentNode:
parentNode.left = None
else:
parentNode.right = None
return treeRoot # Return the modified tree root
# Mark current node as processed
currentLevelNodes.add(currentNode)
# Enqueue children of the current node
if currentNode.right:
bfsQueue.append([currentNode.right, currentNode])
if currentNode.left:
bfsQueue.append([currentNode.left, currentNode])
The provided Python solution aims at correcting a binary tree where a node might be incorrectly linked. Employing a Breadth-First Search (BFS) strategy, the approach utilizes a double-ended queue (deque
) for efficient insertion and removal operations. Here's a brief summarization of how the tree correction process works:
- Initialize the BFS with the root node of the tree. Each element in the queue is a pair consisting of the current node and its parent node.
- Process each tree level iteratively.
- For each node,
- Check its children and specifically monitor the right child to determine if it has already been encountered at the current level, indicating an erroneous linkage.
- If a wrong connection is detected, sever the incorrect link by updating the parent's reference to
None
, thus removing the defective node from the tree. - Add the valid children of the current node to the BFS queue for subsequent level processing, maintaining accurate parent-child pairs.
This correction stops as soon as an erroneous link is found and corrected, ensuring minimal adjustments for tree recovery. The function then outputs the adjusted tree's root, reflecting the rectified structure.
No comments yet.