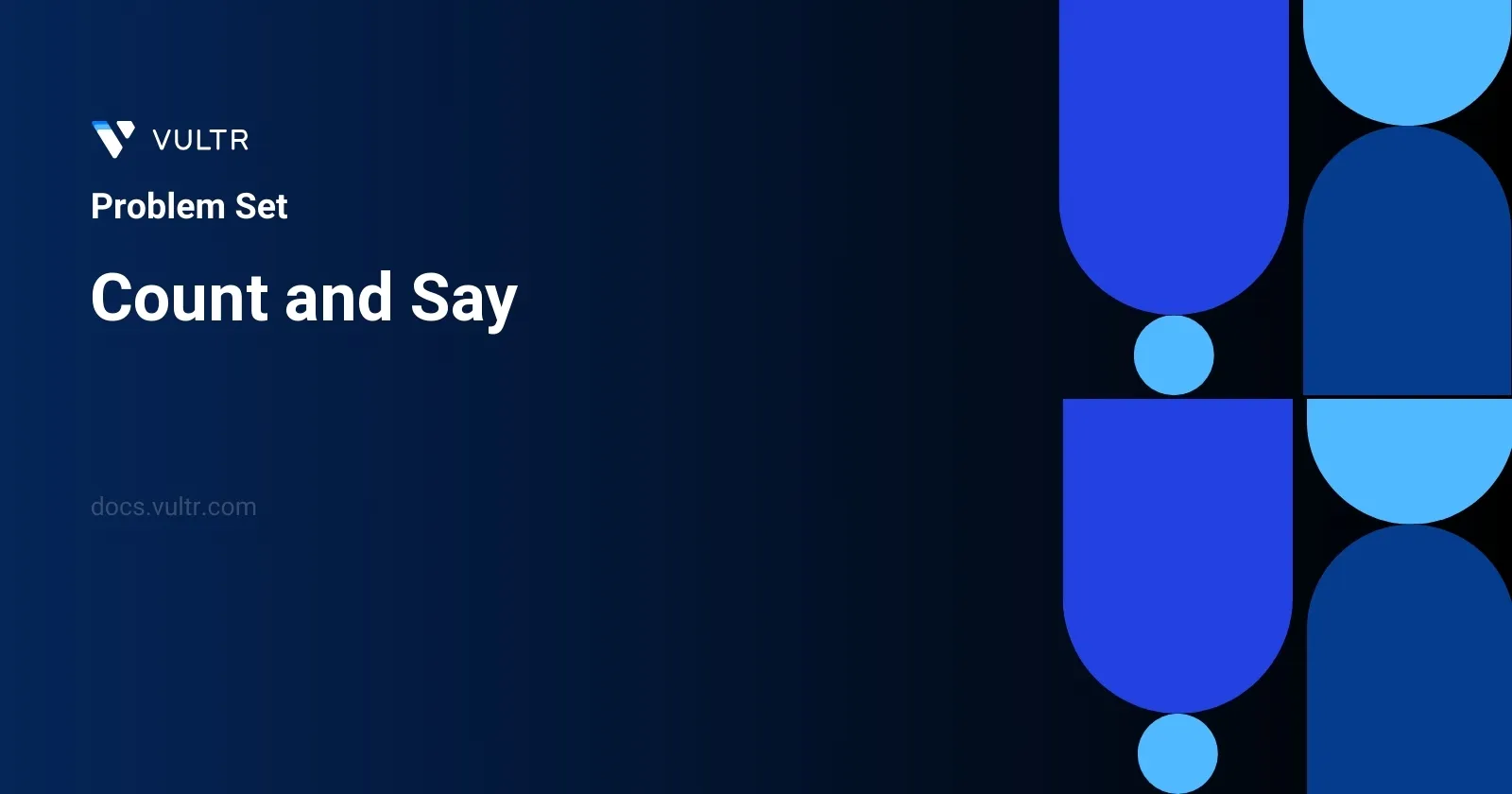
Problem Statement
The count-and-say sequence is a fascinating numerical sequence commonly seen in programming context puzzles. It starts with "1" and each subsequent term is generated by describing the previous term in terms of consecutive digits. Every new term in the sequence is the description (not the sum) of the previous term. For instance, starting with 1, the sequence is produced as follows: countAndSay(1) = "1"
, and countAndSay(n)
is derived by applying a Run-Length Encoding (RLE) on countAndSay(n-1)
.
RLE is a basic form of data compression where sequences of the same data values are stored as a single data value and count. This method is applied recursively for each term in the count-and-say sequence. For each integer n
, the task is to determine the nth
term of this sequence using the given recursive rules.
Examples
Example 1
Input:
n = 4
Output:
"1211"
Explanation:
countAndSay(1) = "1" countAndSay(2) = RLE of "1" = "11" countAndSay(3) = RLE of "11" = "21" countAndSay(4) = RLE of "21" = "1211"
Example 2
Input:
n = 1
Output:
"1"
Explanation:
This is the base case.
Constraints
1 <= n <= 30
Approach and Intuition
The key to solving the count-and-say sequence problem lies in understanding the recursive description method through Run-Length Encoding (RLE). Let's break this down for clarity:
Understand the Base Case:
- According to the problem, the base case for the sequence is
countAndSay(1) = "1"
.
- According to the problem, the base case for the sequence is
Comprehend the Recursive Case:
- For every integer
n > 1
,countAndSay(n)
is obtained by describingcountAndSay(n-1)
. In practical terms, this means looking for consecutive runs of the same character in the previous term, counting these runs, and then noting down the number (the count) followed by the character (the value being repeated).
- For every integer
Process the Examples:
- Consider
n=4
:- Start with "1" (
countAndSay(1)
). - Describe "1" as "one 1" -> "11" (
countAndSay(2)
). - Now take "11", describe it as "two 1s" -> "21" (
countAndSay(3)
). - Describe "21" as "one 2, then one 1" -> "1211" (
countAndSay(4)
).
- Start with "1" (
- Thus, for inputs following similar patterns, you keep converting based on counts of consecutive characters.
- Consider
Recursion vs. Iteration:
- While the definition is recursive, it may be more efficient to use an iterative approach to avoid excessive stack depth, particularly as n can go up to 30 based on the constraints.
Finally, this sequence problem emphasizes description rather than arithmetic transformation, making it an interesting challenge that tests your ability to convert direct observations into encoded string formats using basic coding techniques.
Solutions
- C++
- Java
- C
- JavaScript
- Python
class Solution {
public:
string countAndSay(int n) {
regex pattern("(.)\\1*");
string result = "1";
for (int i = 2; i <= n; i++) {
string temp;
for (sregex_iterator it = sregex_iterator(result.begin(), result.end(), pattern);
it != sregex_iterator(); it++) {
temp += to_string(it->str().length()) + it->str(1);
}
result = temp;
}
return result;
}
};
The solution for the "Count and Say" problem in C++ involves generating a sequence where each term is a verbal description of the previous term's digits, starting with "1". The provided code achieves this using a regex pattern to group consecutive similar digits in the string.
- The
countAndSay
function takes an integern
as its parameter and returns then
-th term of the count-and-say sequence. - To create the sequence, start with the string
"1"
. - Use a loop to iteratively build each term from the second to the
n
-th term. - Utilize the
regex
library with the pattern"(.)\\1*"
which matches any digit followed by zero or more occurrences of the same digit. - Iterate over matches using
sregex_iterator
, construct the next sequence term by concatenating the count of digits and the digit itself. - Update
result
with this sequence for each iteration until reaching termn
. - Finally, the function returns the
n
-th term of the sequence.
This approach efficiently builds each term based on the description of the previous term, leveraging regular expressions for pattern matching to count and describe consecutive digits. This C++ solution is an elegant implementation leveraging string manipulation and regular expressions to solve the problem without auxiliary functions or complex logic.
import java.util.regex.Matcher;
import java.util.regex.Pattern;
class Solution {
public String sequenceGenerator(int count) {
String output = "1";
Pattern regex = Pattern.compile("(.)\\1*");
for (int index = 1; index < count; ++index) {
Matcher match = regex.matcher(output);
StringBuffer newString = new StringBuffer();
while (match.find()) {
newString.append(match.group().length() + match.group().charAt(0));
}
output = newString.toString();
}
return output;
}
}
The "Count and Say" problem involves generating the n-th term of a sequence where each term is a read-off of the count of each group of similar digits in the previous term. The Java solution provided implements a method called sequenceGenerator
in a class Solution
. This method takes an integer count
as an argument and generates the count
-th sequence string following these steps:
- Initialize the
output
string with the starting sequence "1". - Define a regex pattern to match consecutive identical characters using
Pattern.compile("(.)\\1*")
. - Iterate from 1 to
count - 1
. For each iteration:- Use a
Matcher
to find and process each sequence of identical characters in the currentoutput
. - For each match found, append to a new
StringBuffer
the length of the match and the character of the match (essentially "saying" how many of a particular character there are).
- Use a
- Update
output
with the newly constructed string fromStringBuffer
. - After completing all iterations, return the final
output
.
The approach efficiently creates each sequence in the series by using regex to group and count consecutive identical characters, and then constructing the next sequence based on these counts. This solution harnesses Java's Pattern
and Matcher
classes for regex operations and string manipulation capabilities to build the sequence iteratively.
char *sequenceGenerate(int length) {
char *result = (char *)malloc(5000 * sizeof(char));
strcpy(result, "1");
for (int step = 2; step <= length; step++) {
char *next = (char *)malloc(5000 * sizeof(char));
int index = 0;
for (int pos = 0; pos < strlen(result);) {
int freq = 0;
while (result[pos] == result[pos + freq]) freq++;
next[index++] = (char)(freq + '0');
next[index++] = result[pos];
pos += freq;
}
next[index] = '\0';
free(result);
result = next;
}
return result;
}
The provided C code implements a solution to the "Count and Say" problem, generating the sequence up to a given length. Follow these steps to understand how the sequence is constructed and how the function operates:
Memory Allocation for Result: Initially, memory is allocated for the
result
string which can hold up to 5000 characters. This string stores the current sequence.Initial Sequence Setup: Starts with the "1" sequence.
Loop Through Each Step: Iterations begin from 2 up to the desired sequence
length
.Generate Next Sequence:
- Allocate memory for the
next
string which will store the new sequence generated in the current iteration. - Initialize an index to keep track of the position in the next sequence string.
- Allocate memory for the
Count Frequencies:
- For each character in
result
, count how many times it consecutively appears using a nested loop. - Convert the count (frequency) to a character and store it followed by the character itself into the
next
string.
- For each character in
Update and Clean Up:
- Null-terminate the
next
string. - Free the memory allocated for the
result
of the previous sequence. - Update
result
to point tonext
.
- Null-terminate the
Final Output:
- After completing all iterations, return the final
result
string, which represents the sequence for the givenlength
.
- After completing all iterations, return the final
This efficient procedure leverages dynamic memory allocation, careful counting of character frequencies, and iterative building of the sequence string. Each step carefully builds upon the last, ensuring that the sequence is correctly formed for each integer increment up to the desired length. Memory management is crucial as each iteration involves freeing and reallocating memory to avoid leaks.
var countSaySequence = function (sequenceNumber) {
let current = "1";
for (let index = 2; index <= sequenceNumber; index++) {
let nextSeq = "";
const sequencePattern = /(.)\1*/g;
let patternMatch;
while ((patternMatch = sequencePattern.exec(current)) !== null) {
nextSeq += patternMatch[0].length.toString() + patternMatch[1];
}
current = nextSeq;
}
return current;
};
The "Count and Say" problem involves generating a sequence where each term describes the count of each group of consecutive digits in the previous term. The solution provided is a JavaScript function that computes the sequence up to the desired term number. Follow these steps within the code to understand how it achieves this:
- Initialize
current
as the starting sequence string"1"
. - Use a loop starting from
2
up to the givensequenceNumber
. This loop iterates through each sequence number counting and saying based oncurrent
. - Inside the loop, create an empty string
nextSeq
that builds the next sequence. - Define a regular expression
sequencePattern = /(.)\1*/g
to match groups of consecutive identical digits. - Utilize a while loop to process matches found by executing the regex pattern on
current
. This loop continues until no more matches exist. - For each match found, append to
nextSeq
the length of the consecutive digits (number of repeats) followed by the digit itself. - Assign
nextSeq
tocurrent
after processing all groups of digits for the current sequence.
At the end of the function, return current
which contains the desired count and say sequence for the specified term number.
Remember to:
- Ensure the input
sequenceNumber
is a positive integer greater than 0 to avoid errors or unexpected behaviors. - Observe how the regular expression effectively identifies and groups the recurring digits, which is pivotal for constructing the sequence.
- Keep in mind that the output sequence’s length grows exponentially as
sequenceNumber
increases, thus managing larger values might impact performance.
class Solution:
def compute_sequence(self, num: int) -> str:
current = "1"
for _ in range(num - 1):
current = re.sub(
r"(.)\1*", lambda match: str(len(match.group(0))) + match.group(1), current
)
return current
The provided Python solution addresses the "Count and Say" problem by utilizing regular expressions to generate sequential terms. The solution class, named Solution
, contains the method compute_sequence
, which takes an integer num
as the input and returns the num
-th term of the Count and Say sequence as a string.
Follow these steps to understand the solution:
- Initialize a variable
current
with the string "1", which is the base case of the sequence. - Iterate
num - 1
times to generate the sequence up to the desired term. - In each iteration, use a regular expression to group consecutive identical characters in the
current
string. - Replace each group with the count of characters in the group followed by the character itself, using
re.sub()
function. - The lambda function in
re.sub()
computes the replacement string for each match, concatenating the length of the match and the matched character. - After completing all iterations, the function returns the final value of
current
representing thenum
-th term of the sequence.
This solution efficiently constructs each term by building upon the previous term, iterating through the sequence only as many times as required, making it scalable for larger inputs.
No comments yet.