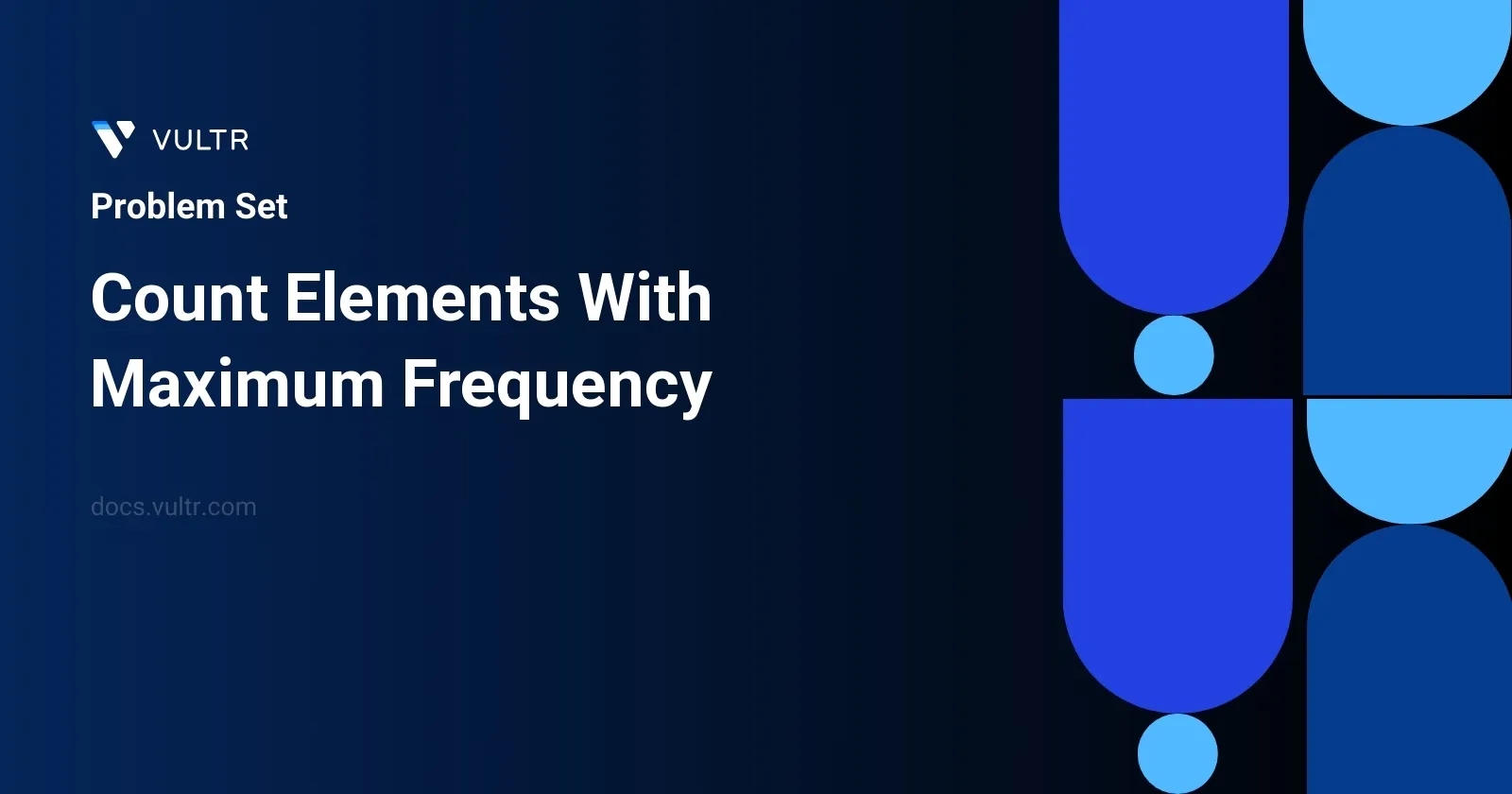
Problem Statement
In the given problem, you are provided with an array nums
composed entirely of positive integers. The task is to determine the total occurrences of the elements in the array that share the highest frequency of appearance. Specifically, the frequency of an element is defined as the number of times it appears in that array. The objective is to sum up the frequencies of all such elements that have the most repetitions in the array, and return this sum.
Examples
Example 1
Input:
nums = [1,2,2,3,1,4]
Output:
4
Explanation:
The elements 1 and 2 have a frequency of 2 which is the maximum frequency in the array. So the number of elements in the array with maximum frequency is 4.
Example 2
Input:
nums = [1,2,3,4,5]
Output:
5
Explanation:
All elements of the array have a frequency of 1 which is the maximum. So the number of elements in the array with maximum frequency is 5.
Constraints
1 <= nums.length <= 100
1 <= nums[i] <= 100
Approach and Intuition
The solution to this problem involves a few clear computational steps:
Count the occurrences of each number in the given array. This can be efficiently done using a dictionary where the keys are the elements of the array and the values are their corresponding counts.
Determine the maximum frequency from these counts. This involves comparing the frequency values and capturing the highest one.
Aggregate the frequencies of all numbers that share this maximum frequency by simply iterating over our counts and summing the ones that match the maximum frequency.
Here's the conceptual breakdown using the provided examples:
For Example 1:
nums = [1,2,2,3,1,4]
- Frequencies would look like
{1:2, 2:2, 3:1, 4:1}
- The maximum frequency is 2.
- Sum the counts of all elements that have the frequency 2 which gives us
2 + 2 = 4
.
For Example 2:
nums = [1,2,3,4,5]
- Each element appears exactly once, so the frequencies are
{1:1, 2:1, 3:1, 4:1, 5:1}
- The maximum frequency again, in this case, is 1.
- Summate the frequencies, resulting in
1 + 1 + 1 + 1 + 1 = 5
.
By leveraging the properties of dictionary data structures for counting and simple iterations for evaluating conditions, the problem can be efficiently solved even as the size of the array reaches its upper constraint.
Solutions
- C++
- Java
- Python
class Solution {
public:
int retrieveMaxFrequencyTotal(vector<int>& elements) {
unordered_map<int, int> elemFreq;
int highestFreq = 0;
int frequencySum = 0;
for (int element : elements) {
elemFreq[element]++;
int currentFreq = elemFreq[element];
if (currentFreq > highestFreq) {
highestFreq = currentFreq;
frequencySum = currentFreq;
} else if (currentFreq == highestFreq) {
frequencySum += currentFreq;
}
}
return frequencySum;
}
};
The provided C++ solution focuses on determining the sum of the maximum frequencies of elements in an array. Here’s a step-by-step breakdown:
- Define a function
retrieveMaxFrequencyTotal
that accepts a vector of integerselements
. - Utilize an
unordered_map
namedelemFreq
to track the frequency of each element. - Initialize two integer variables:
highestFreq
to track the highest frequency encountered, andfrequencySum
to store the sum of frequencies at which the highest frequency occurs. - Loop through each
element
inelements
:- Increment the frequency of the current element in the map.
- If this frequency is greater than
highestFreq
, updatehighestFreq
with this new frequency and resetfrequencySum
to this frequency since it starts counting a new highest frequency. - If the frequency of the element matches
highestFreq
, add this frequency tofrequencySum
.
- Return
frequencySum
which holds the sum of all occurrences of elements having the maximum frequency.
This solution efficiently computes the required sum by using a hashmap to keep count of occurrences, and adjusting the sum and highest frequency on-the-fly as the elements are processed.
public class Solution {
public int findMostFrequentElements(int[] elements) {
Map<Integer, Integer> countMap = new HashMap<>();
int highestFreq = 0;
int sumOfMaxFreq = 0;
for (int element : elements) {
countMap.put(element, countMap.getOrDefault(element, 0) + 1);
int currentFreq = countMap.get(element);
if (currentFreq > highestFreq) {
highestFreq = currentFreq;
sumOfMaxFreq = currentFreq;
} else if (currentFreq == highestFreq) {
sumOfMaxFreq += currentFreq;
}
}
return sumOfMaxFreq;
}
}
Solution Summary
The problem requires counting the sum of the frequencies of the elements that appear most frequently in an array. The solution is implemented in Java.
- Begin by creating a
HashMap
calledcountMap
to store each element and its frequency from the input array. - Initialize two integers,
highestFreq
to track the highest frequency of any element, andsumOfMaxFreq
to keep the sum of the frequencies of the most frequently occurring elements. - Iterate through the array of elements:
- Update the frequency of each element in the
countMap
. If the element isn't already in the map, add it with an initial frequency of one; otherwise, increment its current frequency. - Check the frequency of the current element:
- If this frequency is greater than
highestFreq
, updatehighestFreq
to this new frequency. Also, resetsumOfMaxFreq
to this frequency as the current element is now the most frequent. - If this frequency matches
highestFreq
, incrementsumOfMaxFreq
by this frequency.
- If this frequency is greater than
- Update the frequency of each element in the
- Finally, return
sumOfMaxFreq
, which holds the cumulative frequency of elements that are most frequent in the array.
class Solution:
def highestFrequencySum(self, elements):
element_counts = {}
highest_freq = 0
sum_highest_freq = 0
for element in elements:
element_counts[element] = element_counts.get(element, 0) + 1
count = element_counts[element]
if count > highest_freq:
highest_freq = count
sum_highest_freq = count
elif count == highest_freq:
sum_highest_freq += count
return sum_highest_freq
Count Elements With Maximum Frequency solution is implemented in Python and effectively determines the sum of elements that appear with the highest frequency in a given list called elements
.
The solution involves a few key steps:
- Initialize a dictionary
element_counts
to store the frequency of each element in the list. - Initialize variables
highest_freq
to zero, which will store the maximum frequency found, andsum_highest_freq
to zero, which will store the cumulative sum of the elements that appear with this frequency. - Iterate over each element in the provided list, updating its count in the
element_counts
dictionary. - For each element update, check if the current element's count surpasses the
highest_freq
. If it does, updatehighest_freq
with this new count and resetsum_highest_freq
to the element value multiplied by its count. - If the current element’s count equals
highest_freq
, add the element's count tosum_highest_freq
. - Ultimately, return
sum_highest_freq
, which will be the sum of the counts of all elements appearing the most frequently in the list.
By following the above steps, you will be able to compute the sum of the frequencies of the elements that are most frequent in the elements
list.
No comments yet.