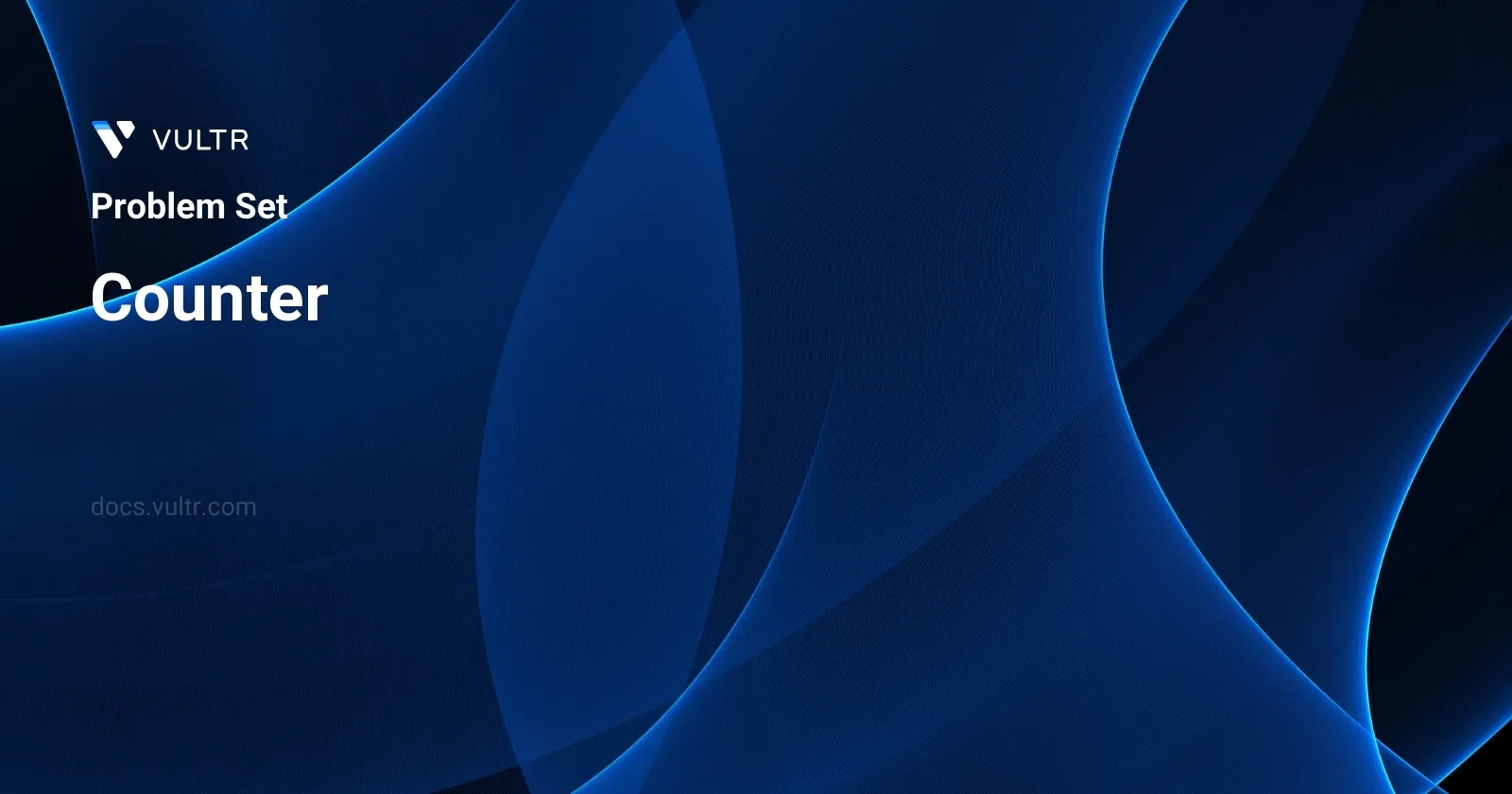
Problem Statement
In this scenario, you are asked to design a function known as counter
, which behaves in a specific manner when invoked. The function is instantiated with an integer n
. Upon the first invocation, it should return the value of n
. For every subsequent call, the function must return a value that is incremented by 1 from the last value it returned. The challenge involves maintaining state between function calls to achieve this behavior, ensuring that with each invocation, the output is as expected: n
, n+1
, n+2
, and so forth.
Examples
Example 1
Input:
n = 10 ["call","call","call"]
Output:
[10,11,12]
Explanation: counter() = 10 // The first time counter() is called, it returns n. counter() = 11 // Returns 1 more than the previous time. counter() = 12 // Returns 1 more than the previous time.
Example 2
Input:
n = -2 ["call","call","call","call","call"]
Output:
[-2,-1,0,1,2]
Explanation:
counter() initially returns -2. Then increases after each sebsequent call.
Constraints
-1000 <= n <= 1000
0 <= calls.length <= 1000
calls[i] === "call"
Approach and Intuition
The key task here is to implement a function that can remember its state between calls. A simple and effective way to achieve this is by utilizing closures in programming, where a function remembers its lexical scope even when the function is executed outside that scope. Here’s a logical breakdown of the process using the examples provided:
Initialization:
- The function
counter
starts with an initial valuen
. Thus, the first call should merely return this value.
- The function
State Retention:
- Post the initial call, the function needs to remember its last return value. Thus, each subsequent execution should increase this tracked value by 1 and then return the new value.
Considerations from Examples:
- Example 1 illustrates the function starting at
n = 10
. The expected return values after successive calls are 10, 11, and 12. This sequence confirms that the function maintains the state and increments correctly starting fromn
. - Example 2 starts with
n = -2
, providing us insight into how the function should intuitively handle negative numbers, yet adhering to the same principle of incrementing by 1.
- Example 1 illustrates the function starting at
Constraints Compliance:
- The function's design should also respect the given constraints like the value range for
n
and the maximum number of times (calls.length
) the function could be called, ensuring that the increments do not cause overflows or underflows beyond what typical integers can handle.
- The function's design should also respect the given constraints like the value range for
By ensuring these steps are handled within our function, the outcome, as demonstrated, will consistently adhere to the pattern of starting at n
and incrementing by 1 with each call.
Solutions
- JavaScript
var makeCounter = function(value) {
return () => { return value++; };
};
The provided JavaScript code defines a function makeCounter
which generates another function that counts upwards from a given initial value. Here's how the solution operates:
makeCounter
accepts an initial valuevalue
.- This function returns another function, essentially a closure, which when invoked, returns the current value of
value
and increments it afterwards.
The key aspect of this code is the use of a closure that retains access to its own private value
which it modifies upon each call. Access this counter through a variable initialized by makeCounter
. For example:
var counter = makeCounter(5);
console.log(counter()); // Outputs: 5
console.log(counter()); // Outputs: 6
This mechanism provides a simple way to keep track of a count without exposing the count variable to the global scope, promoting encapsulation and preventing unintended modifications from outside the closure.
No comments yet.