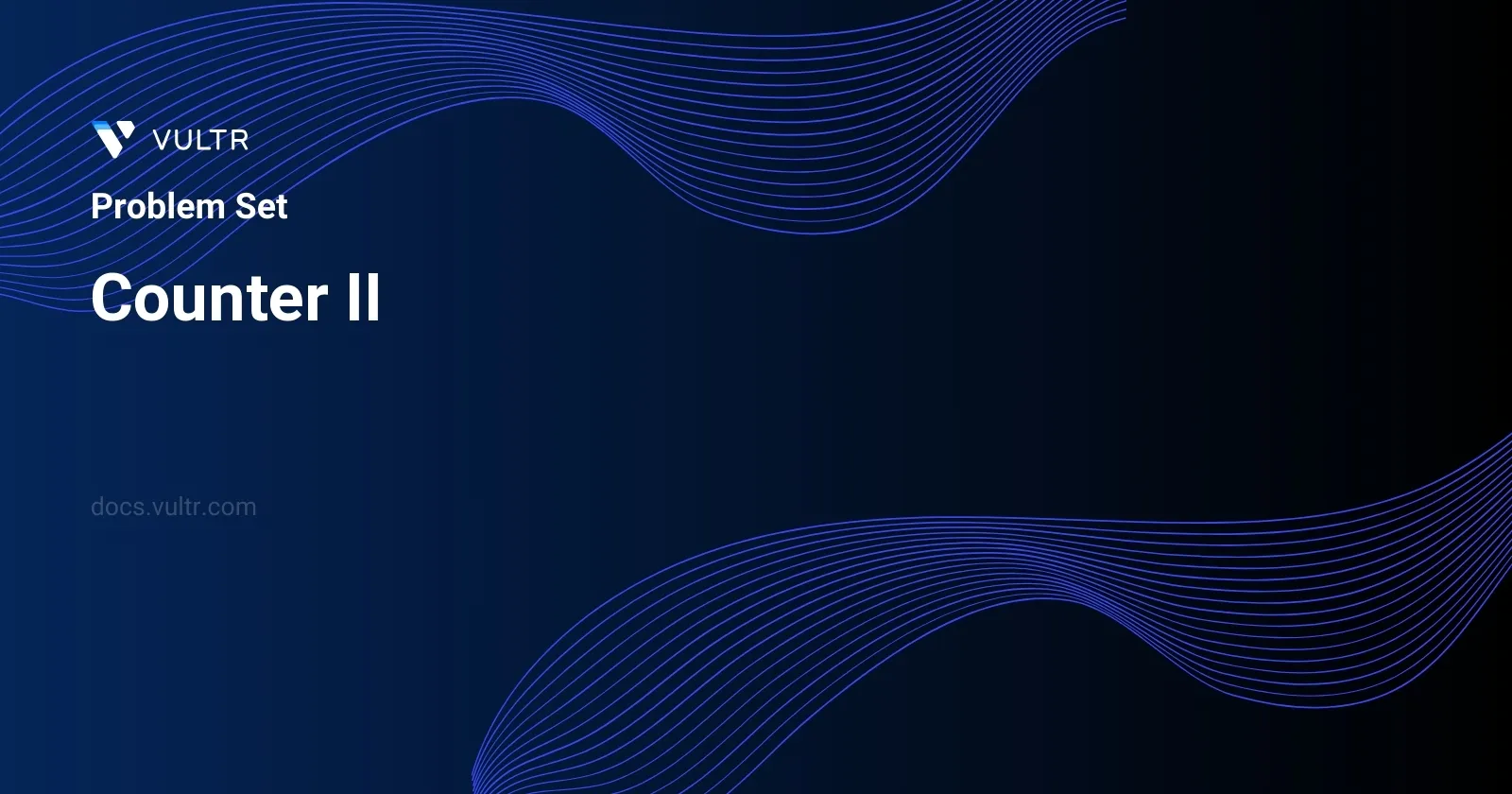
Problem Statement
The task is to develop a function named createCounter
which takes an integer init
as its input parameter. This function should construct and return an object containing three methods: increment()
, decrement()
, and reset()
. Each of these methods modifies or resets a counter based on the initial value provided. Specifically, these functions perform the following actions:
- The
increment()
method increases the counter's current value by one and then returns the new value. - The
decrement()
method decreases the counter's current value by one and then returns the new value. - The
reset()
method reverts the counter to the originalinit
value and then returns this reset value.
This structure allows for an encapsulated approach to managing the counter's state, making the counter's current value accessible and modifiable only through these three defined operations.
Examples
Example 1
Input:
init = 5, calls = ["increment","reset","decrement"]
Output:
[6,5,4]
Explanation:
const counter = createCounter(5); counter.increment(); // 6 counter.reset(); // 5 counter.decrement(); // 4
Example 2
Input:
init = 0, calls = ["increment","increment","decrement","reset","reset"]
Output:
[1,2,1,0,0]
Explanation:
const counter = createCounter(0); counter.increment(); // 1 counter.increment(); // 2 counter.decrement(); // 1 counter.reset(); // 0 counter.reset(); // 0
Constraints
-1000 <= init <= 1000
0 <= calls.length <= 1000
calls[i]
is one of "increment", "decrement" and "reset"
Approach and Intuition
By examining the examples provided, we can deduce the behavioral essence of the createCounter
function and its methods.
Initializing the counter:
- Upon calling
createCounter(init)
, an object with three methods is initialized with the counter set to the value ofinit
. This is straightforward initialization.
- Upon calling
Incrementing the counter:
- When
increment()
is invoked, it simply adds one to the current counter value. This can be implemented using a pre-defined variable that keeps track of the counter's state.
- When
Decrementing the counter:
- Similar to
increment()
,decrement()
reduces the current counter value by one. This operates directly on the same state variable thatincrement()
modifies.
- Similar to
Resetting the counter:
- Whenever
reset()
is called, it sets the counter's value back to the initialinit
value provided at the time of the object’s creation. This function is useful for reusing the counter for a new set of operations without needing to reinitialize the object completely.
- Whenever
Each operation is intended to modify or revert the counter and immediately provide feedback by returning the modified value. This immediate feedback is useful for scenarios where subsequent actions might depend on the result of the previous operation.
Constraints considerations:
- The initial value
init
can range from -1000 to 1000, giving a broad spectrum of negative and positive integers to start from. - The number of actions (calls) on the counter can be as high as 1000, which the counter implementation needs to handle efficiently.
- Each call action is strictly one of "increment", "decrement", or "reset", simplifying the error handling since there are no unexpected commands.
In essence, the createCounter
function and its methods offer a controlled way to manipulate a simple integer value, with the counter’s operations being predictable and confined to the specific actions defined. This makes it relatively straightforward to implement and ensures that the counter's behavior is consistent and transparent to the user.
Solutions
- JavaScript
var initializeCounter = function(initialValue) {
let count = initialValue;
return new Proxy({}, {
get: (target, property) => {
switch(property) {
case "increment":
return () => ++count;
case "decrement":
return () => --count;
case "reset":
return () => (count = initialValue);
default:
throw Error("Unrecognized Operation");
}
},
});
};
In the provided JavaScript code, a function named initializeCounter
is designed to create a counter that can be manipulated through incrementing, decrementing, or resetting to its initial value. This functionality is achieved using a JavaScript Proxy object.
- The
initializeCounter
function takes a single argumentinitialValue
which sets the starting point for the counter. - Within the function, a local variable
count
is initialized with the value ofinitialValue
. - A Proxy object is then returned. This object intercepts access to properties that are assumed to be functions:
increment
,decrement
, andreset
. - Accessing the
increment
property on the proxy returns a function that, when called, increases thecount
by one. - Accessing the
decrement
property returns a function that decreases thecount
by one. - The
reset
property returns a function that setscount
back to the initial value provided when the counter was first initialized. - If an accessed property does not match any of the specified cases (
increment
,decrement
,reset
), an error is thrown indicating an "Unrecognized Operation".
This implementation provides a flexible way of managing the state of a counter through a neatly encapsulated interface, leveraging advanced JavaScript features like closures for state encapsulation and proxies for custom behavior on property access.
No comments yet.