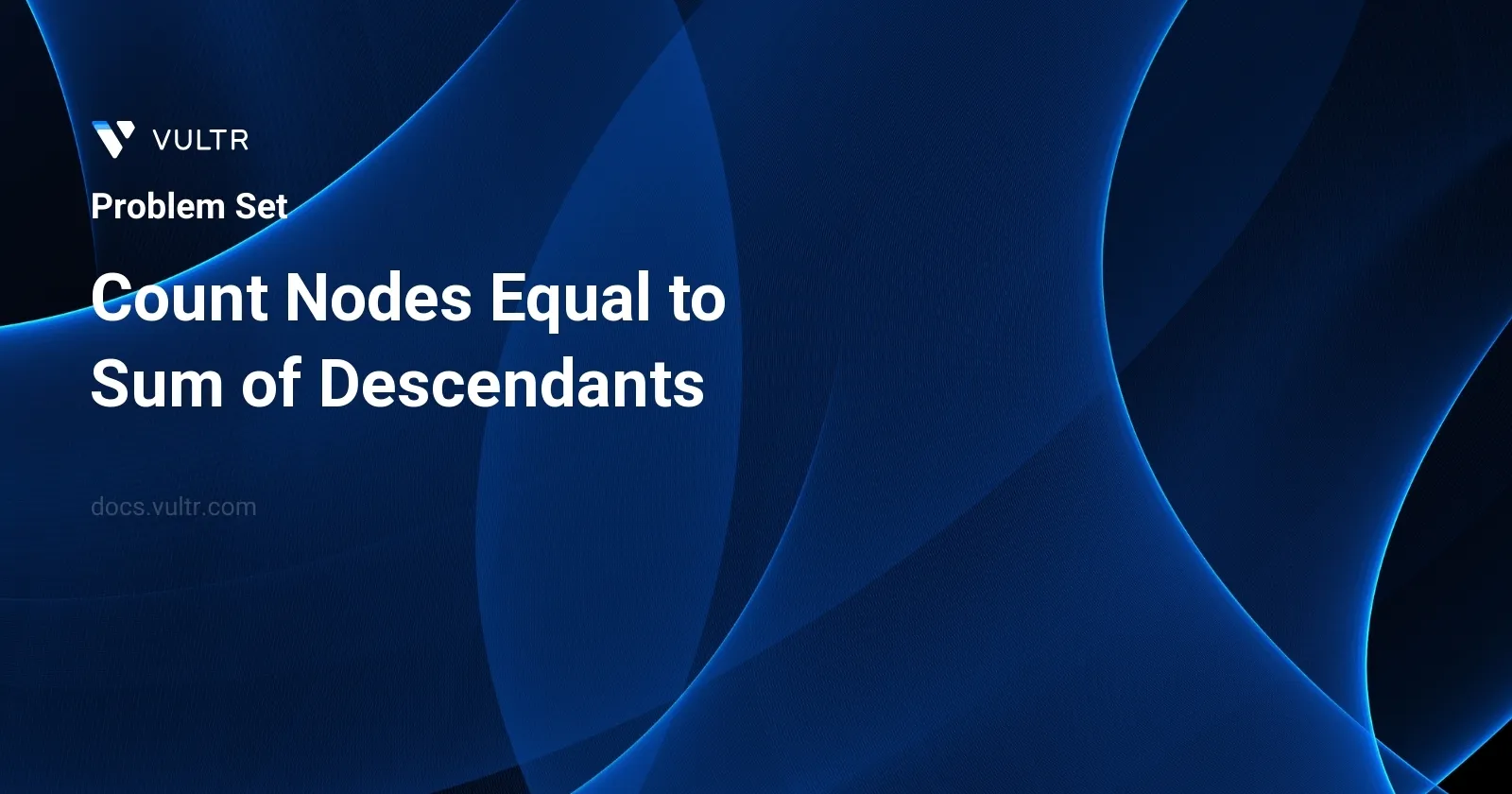
Problem Statement
In this challenge, we are provided with the root of a binary tree and our task is to determine the number of nodes where the node's own value equals the sum of the values of all its descendants. To be clear, a descendant of a node x in this context is defined as any node that lies on the path from node x down to any leaf node. It is important to note that if a node does not have any descendants, the sum of its descendants is considered to be 0. This setup asks for how many such nodes in a given tree satisfy this particular condition.
Examples
Example 1
Input:
root = [10,3,4,2,1]
Output:
2
Explanation:
For the node with value 10: The sum of its descendants is 3+4+2+1 = 10. For the node with value 3: The sum of its descendants is 2+1 = 3.
Example 2
Input:
root = [2,3,null,2,null]
Output:
0
Explanation:
No node has a value that is equal to the sum of its descendants.
Example 3
Input:
root = [0]
Output:
1 For the node with value 0: The sum of its descendants is 0 since it has no descendants.
Constraints
- The number of nodes in the tree is in the range
[1, 105]
. 0 <= Node.val <= 105
Approach and Intuition
To solve this problem, one can adopt a depth-first search (DFS) approach that traverses through the tree and calculates the sum of the descendant nodes for each node. While doing so, it also checks if the condition of the node's value being equal to this sum is met. Here's a step-by-step breakdown:
- Begin by establishing a recursive function, often termed
dfs
or similar, that will take in a node of the tree as its parameter. - For each node, if it's null (indicative of reaching past a leaf), return a sum of 0.
- Recursively call this function on the left child and right child of the current node to get the sum of values from both subtrees.
- Add the two sums derived from the left and right subtree calls. This sum represents the total descendant sum for the current node.
- Check if the current node's value equals this sum. If it does, increment a global or external counter that tracks the number of such nodes.
- Finally, update the return value of the
dfs
function to include the current node's value plus the sum of its descendants, as this total sum is passed up the call stack to the parent node.
The main idea is recursively to accumulate descendant sums up the tree while also comparing each node's value to its descendant sum. This approach implicitly handles edge cases such as:
- Nodes with no children where the descendant sum should be 0.
- Trees with just one node, which technically meets the condition trivially as its own value is necessarily equal to its descendant sum (which is 0).
When implemented efficiently, this solution will traverse each node a single time, resulting in a time complexity of O(n), where n is the number of nodes in the tree. The recursive nature of the DFS approach makes the space complexity O(h), where h is the height of the tree, due to the stack space used by recursive calls.
Solutions
- C++
- Java
class Solution {
public:
int nodeCount;
long recursiveNodeSum(TreeNode* node) {
if (node == nullptr) {
return 0;
}
long sumLeft = recursiveNodeSum(node->left);
long sumRight = recursiveNodeSum(node->right);
if (node->val == sumLeft + sumRight) {
nodeCount++;
}
return sumLeft + sumRight + node->val;
}
int nodesEqualToSumDescendants(TreeNode* node) {
recursiveNodeSum(node);
return nodeCount;
}
};
The solution focuses on counting the nodes in a binary tree where the node's value is equal to the sum of its descendants. Implement this operation in C++ using a simple yet effective recursive approach that traverses the tree and compares each node's value to the computed sum of values from its left and right children.
The implementation can be dissected into the following functional pieces:
A function
recursiveNodeSum(TreeNode* node)
:- Checks if the node is null. If yes, returns zero which indicates that there are no values to sum.
- Recursively calls itself for the left child and the right child nodes.
- Computes the sum of values returned from both children.
- Checks if the node's value is equal to the sum of its two children. If they are equal, it increments the
nodeCount
. - Returns the total value computed which includes the node's own value plus the sum of its descendants.
A main function
nodesEqualToSumDescendants(TreeNode* node)
:- Calls the
recursiveNodeSum(TreeNode* node)
to initiate the recursive tree traversal from the given node. - Returns the
nodeCount
, which reflects the number of nodes satisfying the condition of value being equal to the sum of their descendants.
- Calls the
This approach ensures a thorough examination of each node precisely once and efficiently calculates the required conditions, suitable for situations where a clear and reliable understanding of binary tree structures is essential.
class Solution {
int totalEqual;
private long sumNodes(TreeNode node) {
if (node == null) {
return 0;
}
long sumLeft = sumNodes(node.left);
long sumRight = sumNodes(node.right);
if (node.val == sumLeft + sumRight) {
totalEqual++;
}
return sumLeft + sumRight + node.val;
}
public int countEqualDescendants(TreeNode root) {
sumNodes(root);
return totalEqual;
}
}
The code provided in Java is designed to solve the problem of counting tree nodes whose values equal the sum of their descendants' values. It defines a class Solution
with a method to traverse the tree and another method to initiate this calculation.
- The class contains an instance variable
totalEqual
which keeps track of the number of nodes meeting the condition. - The method
sumNodes(TreeNode node)
recursively calculates the sum of values of all descendant nodes of a given node. - If a node’s value is equal to the sum of its descendants (left and right child nodes),
totalEqual
is incremented. - The sums of the left and right children are combined and added to the current node’s value to provide a cumulative total for higher recursive calls.
- The
countEqualDescendants(TreeNode root)
method is the entry point of the calculation, which invokessumNodes
starting from the root of the tree and returns the value oftotalEqual
.
By following these steps, this Java class efficiently counts how many nodes in a binary tree have values equal to the sum of all values stored in their descendants, fulfilling the problem's requirements.
No comments yet.