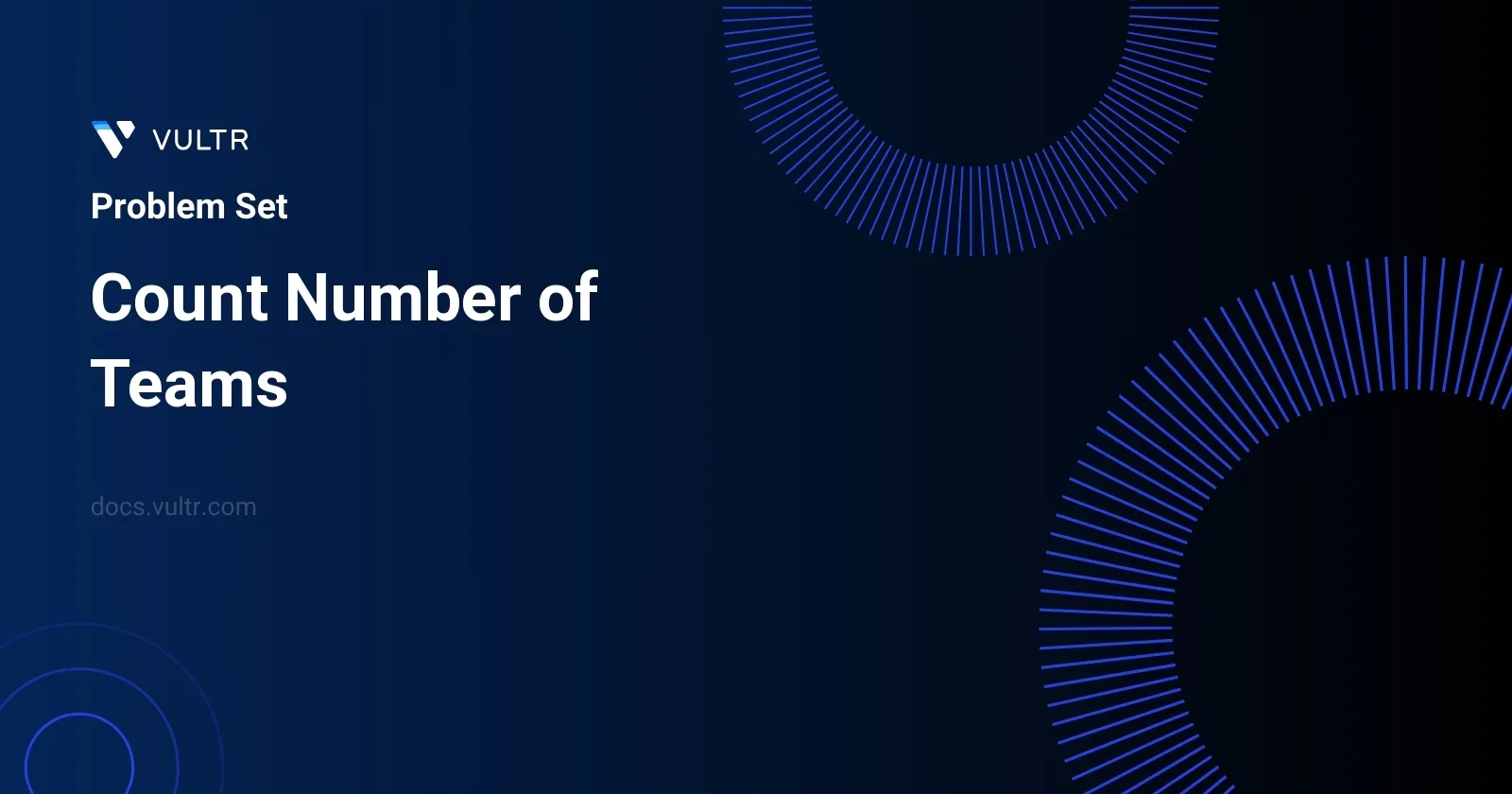
Problem Statement
In this problem, you are given an array named rating
which represents the unique rating values of n
soldiers standing in a line. Your task is to identify and count all possible teams of three soldiers that can be formed under specific conditions.
A team of three soldiers indexed as (i
, j
, k
) with the respective ratings (rating[i]
, rating[j]
, rating[k]
) is considered valid if:
- Their ratings are either strictly increasing: (
rating[i] < rating[j] < rating[k]
), - Or their ratings are strictly decreasing: (
rating[i] > rating[j] > rating[k]
).
Additionally, the selection of these indices must adhere to the order 0 <= i < j < k < n
. You are required to return the total number of valid teams that can be formed.
Examples
Example 1
Input:
rating = [2,5,3,4,1]
Output:
3
Explanation:
We can form three teams given the conditions. (2,3,4), (5,4,1), (5,3,1).
Example 2
Input:
rating = [2,1,3]
Output:
0
Explanation:
We can't form any team given the conditions.
Example 3
Input:
rating = [1,2,3,4]
Output:
4
Constraints
n == rating.length
3 <= n <= 1000
1 <= rating[i] <= 105
- All the integers in
rating
are unique.
Approach and Intuition
The objective here is to count all the valid combinations of soldiers forming a team based on specific ordered conditions.
Firstly, for any position
j
in therating
array, you can determine how many elements to the left (i < j
) are smaller thanrating[j]
and how many are greater. These counts help in identifying possible increasing and decreasing sequences respectively.Similarly, for the same position
j
, determine how many elements to the right (k > j
) are greater and how many are smaller thanrating[j]
.For a triplet formation where
i < j < k
:Increading triplet count: If there are
countLeftLess
numbers less thanrating[j]
to the left andcountRightMore
numbers greater thanrating[j]
to the right, then the combinations of forming a increasing triplet withj
as the middle element iscountLeftLess * countRightMore
.Decreasing triplet count: If there are
countLeftMore
numbers greater thanrating[j]
to the left andcountRightLess
numbers less thanrating[j]
to the right, then the combinations for forming a decreasing triplet withj
as the middle element iscountLeftMore * countRightLess
.
By iterating over each element and calculating the above mentioned counts and combinations, you can sum them up to get the total number of valid teams.
This logical approach utilizes a blend of combinatorial mathematics and array manipulation, iterating through potential mid-points and calculating viable triplets, both increasing and decreasing, from their respective bounds.
Solutions
- C++
- Java
- Python
class Solution {
public:
int countTeams(vector<int>& arr) {
// Find the highest rating
int highestRating = 0;
for (int n : arr) {
highestRating = max(highestRating, n);
}
// Initialize BITs for calculation
vector<int> bitLeft(highestRating + 1, 0);
vector<int> bitRight(highestRating + 1, 0);
// Fill the right BIT with the initial values
for (int n : arr) {
bitUpdate(bitRight, n, 1);
}
int resultCount = 0;
for (int r : arr) {
// Remove the current element from the right BIT
bitUpdate(bitRight, r, -1);
// Calculate smaller and larger counts on both sides
int leftLess = queryBIT(bitLeft, r - 1);
int rightLess = queryBIT(bitRight, r - 1);
int leftMore = queryBIT(bitLeft, highestRating) - queryBIT(bitLeft, r);
int rightMore = queryBIT(bitRight, highestRating) - queryBIT(bitRight, r);
// Compute results for valid sequences
resultCount += (leftLess * rightMore) + (leftMore * rightLess);
// Insert current into the BIT for left side
bitUpdate(bitLeft, r, 1);
}
return resultCount;
}
private:
// Function to update a BIT
void bitUpdate(vector<int>& bit, int index, int delta) {
while (index < bit.size()) {
bit[index] += delta;
index += index & (-index); // Next index calculation
}
}
// Function to compute prefix sum in a BIT
int queryBIT(vector<int>& bit, int index) {
int sum = 0;
while (index > 0) {
sum += bit[index];
index -= index & (-index); // Move to the parent
}
return sum;
}
};
The problem "Count Number of Teams" demands an efficient solution to count special sequences within a list of ratings. The given C++ solution implements this by utilizing Binary Indexed Trees (BITs) to handle some specific BIT manipulations like updates and queries for prefix sums.
Here’s a breakdown of how the solution works:
Define the Highest Rating in the Rating Array: First, iterate over the ratings to determine the largest value, necessary for defining the size of two Binary Indexed Trees (BITs) used in the solution:
bitLeft
andbitRight
.Initialize the BITs:
bitLeft
is initialized to keep track of how many elements with a certain rating have been processed as we iterate from left to right.bitRight
is used to track how many elements of each rating exist to the right of the current element in the traversal.
Populate
bitRight
with the initial counts of each rating.Iterate Over the Ratings to Compute Result: For each team member's rating in the array:
- Update
bitRight
to indicate that the current member's rating is now permanent and is moving frombitRight
tobitLeft
. - Query both
bitLeft
andbitRight
to ascertain the count of ratings both less than and greater than the current rating, to the left and right. - Calculate the number of valid sequences using combinations of these counts.
- Update
bitLeft
to reflect the addition of the current rating now being considered as processed.
- Update
Update and Query Functions: Define helper functions
bitUpdate
andqueryBIT
to respectively update the counts in the BITs and obtain prefix sum queries, both critical operations made efficient by the BIT structure.
This effectively allows maintaining and querying count information about ratings dynamically through the array traversal, crucial for calculating the result without resorting to less efficient brute force methods. The careful and efficient use of BIT operations provides the necessary mechanisms to compute the desired result of how many special sequences can be formed, optimizing the approach substantially over simpler iterative checks.
class Solution {
public int calculateTeams(int[] ratings) {
int highestRating = 0;
for (int rate : ratings) {
highestRating = Math.max(highestRating, rate);
}
int[] leftIndexTree = new int[highestRating + 1];
int[] rightIndexTree = new int[highestRating + 1];
for (int rate : ratings) {
updateIndexTree(rightIndexTree, rate, 1);
}
int totalTeams = 0;
for (int current : ratings) {
updateIndexTree(rightIndexTree, current, -1);
int leftLesser = sumPrefix(rightIndexTree, current - 1);
int rightLesser = sumPrefix(leftIndexTree, current - 1);
int leftGreater = sumPrefix(leftIndexTree, highestRating) - sumPrefix(leftIndexTree, current);
int rightGreater = sumPrefix(rightIndexTree, highestRating) - sumPrefix(rightIndexTree, current);
totalTeams += (leftLesser * rightGreater);
totalTeams += (leftGreater * rightLesser);
updateIndexTree(leftIndexTree, current, 1);
}
return totalTeams;
}
private void updateIndexTree(int[] tree, int idx, int val) {
while (idx < tree.length) {
tree[idx] += val;
idx += idx & (-idx);
}
}
private int sumPrefix(int[] tree, int idx) {
int sum = 0;
while (idx > 0) {
sum += tree[idx];
idx -= idx & (-idx);
}
return sum;
}
}
In the provided Java code, the algorithm focuses on counting the number of valid teams based on a ranking system given in the array 'ratings'. The problem is approached by using two Fenwick Trees (Binary Indexed Trees), one to manage the left index updates and another for right index calculations.
The code functions based on the following sequence of operations:
Initialize Variables: Two Fenwick Trees are initialized and the maximum value in the ratings array is determined to set the maximal bounds of the trees.
Fill Right Index Tree: Initially, the right index tree is filled with the frequencies of respective ratings, enabling queries about how many soldiers have a rating less than or equal to the current during the main computation.
Calculate Teams:
Loop through each rating.
Before processing each rating, decrement its count in the right index tree to exclude the current soldier from subsequent calculations.
Calculate the number of valid combinations for forming teams by determining:
leftLesser
: The number of soldiers to the left with a rating less than the current soldier.rightLesser
: Using the right tree's state at this point to determine how many soldiers can be right of the current with a lower rating.leftGreater
: Soldiers to the left with a larger rating.rightGreater
: Soldiers to the right with a larger rating.
For each soldier, the number of teams that the soldier can form where he/she is the middle member is incremented by the product of the lesser counterparts on one side and the greater counterparts on the other side.
Final Result: At the end of iterations, the
totalTeams
variable holds the total count of 3-member teams sorted by the rank that can be formed.Helper Functions:
updateIndexTree
facilitates updating values in the Fenwick Trees, whilesumPrefix
helps in querying the prefix sums to count how many ratings are below a certain threshold.
This method efficiently computes possible teams by leveraging the logarithmic update and query capabilities of the Fenwick Tree, thus ensuring that the solution is scalable for larger datasets.
class Solution:
def countTeams(self, ratings: List[int]) -> int:
highest_rating = 0
for rate in ratings:
highest_rating = max(highest_rating, rate)
left_index_tree = [0] * (highest_rating + 1)
right_index_tree = [0] * (highest_rating + 1)
for rate in ratings:
self._updateTree(right_index_tree, rate, 1)
result = 0
for rate in ratings:
self._updateTree(right_index_tree, rate, -1)
count_smaller_left = self._fetchSum(left_index_tree, rate - 1)
count_smaller_right = self._fetchSum(right_index_tree, rate - 1)
count_larger_left = self._fetchSum(left_index_tree, highest_rating) - self._fetchSum(left_index_tree, rate)
count_larger_right = self._fetchSum(right_index_tree, highest_rating) - self._fetchSum(right_index_tree, rate)
result += count_smaller_left * count_larger_right
result += count_larger_left * count_smaller_right
self._updateTree(left_index_tree, rate, 1)
return result
def _updateTree(self, index_tree: List[int], position: int, increment: int) -> None:
while position < len(index_tree):
index_tree[position] += increment
position += position & (-position)
def _fetchSum(self, index_tree: List[int], position: int) -> int:
total = 0
while position > 0:
total += index_tree[position]
position -= position & (-position)
return total
This solution tackles the problem of counting the number of valid teams based on a rating system where a team can be formed by any three participants such that their ratings strictly increase or decrease.
The provided Python code uses a class Solution
with the method countTeams
which accepts a list of integers ratings
representing the ratings of individuals. The process involves:
- Finding the highest rating to limit the size of Binary Indexed Trees (BIT) used for efficient range sum queries and updates.
- Initializing two BITs (
left_index_tree
andright_index_tree
) to handle frequencies of ratings to the left and right of the current element respectively. - Iterating over each rating to update
right_index_tree
indicating the presence of each rating. - For each rating:
- Decrease count in
right_index_tree
(because moving past the current rating). - Counting the numbers smaller and larger on both left and right of each rating using the helper method
_fetchSum
which returns the sum of the elements up to a given position in BIT. - Calculate potential valid teams and aggregate to previous results.
- Update
left_index_tree
to include the current rating.
- Decrease count in
- The method
_updateTree
helps in modifying the BIT at a specified position, and_fetchSum
is used to compute prefix sums from BIT for range queries.
This approach efficiently counts potential teams with the BIT managing frequency and partial sums computation, allowing quick updates and range sum calculations which are fundamental in this problem, accommodating dynamic changes in data within the rating sequence.
No comments yet.