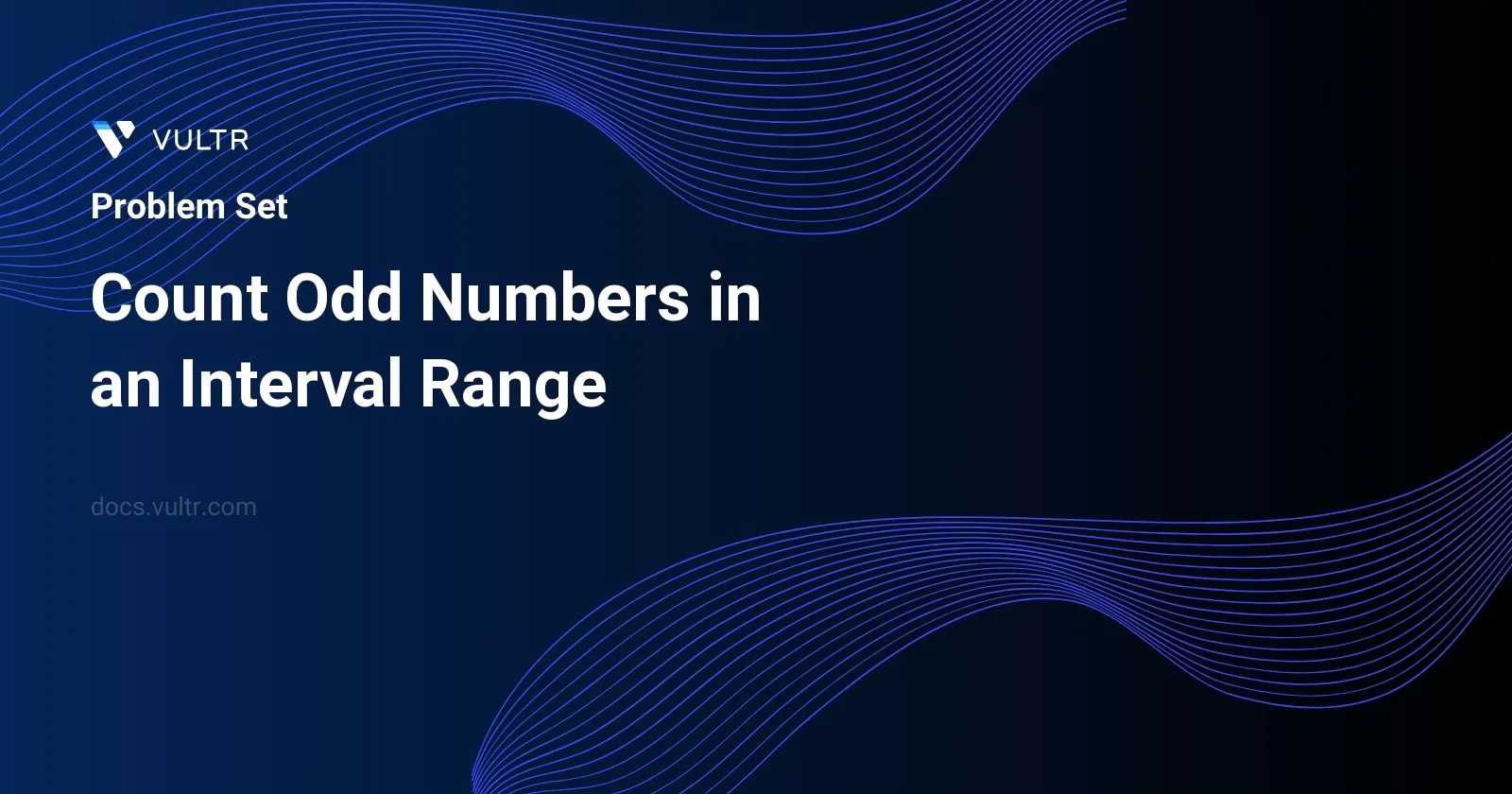
Problem Statement
The primary task is to determine the number of odd numbers that lie between two specified non-negative integers, including these two integers themselves. The two integers, termed as low
and high
, serve as the boundaries for this range. In essence, the function should return a count indicating how many numbers within this inclusive range are odd.
Examples
Example 1
Input:
low = 3, high = 7
Output:
3
Explanation:
The odd numbers between 3 and 7 are [3,5,7].
Example 2
Input:
low = 8, high = 10
Output:
1
Explanation:
The odd numbers between 8 and 10 are [9].
Constraints
0 <= low <= high <= 10^9
Approach and Intuition
To solve this problem efficiently while considering the constraint limitations, observation and mathematical deduction are key. The constraints given (0 <= low <= high <= 10^9
) suggest that a naive approach of iterating through each number between low
and high
would be computationally expensive, especially at the upper limits. Instead, a more mathematical approach can be applied:
Understanding Odd Number Counting:
- Odd numbers are separated by even numbers. Between any two consecutive integers, one will always be even and the other odd, alternatively.
Starting Point Consideration:
- If
low
is odd, the first number in our range is an odd number. Iflow
is even, the count starts from the next number which will be odd.
- If
Ending Point Consideration:
- Similarly, if
high
is odd, it concludes our counting range on an odd number.
- Similarly, if
Calculating the Number of Odds Directly:
- Calculate the number of odds between two consecutive integers directly:
- If both
low
andhigh
are odd, or both are even, the count of odd numbers fromlow
tohigh
is(high - low) / 2 + 1
. - If one is odd and the other is even, then the count is
(high - low) / 2
.
- If both
- Calculate the number of odds between two consecutive integers directly:
This direct calculation does not require looping through all numbers from low
to high
, making it optimal even for the highest limits set by the constraints.
Solutions
- C++
- Java
class Solution {
public:
int findOddCount(int min, int max) {
if (!(min & 1)) {
min++;
}
return min > max ? 0 : (max - min) / 2 + 1;
}
};
This C++ code snippet provides a method named findOddCount
to calculate the count of odd numbers within a given interval range specified by the min
and max
parameters. The function first checks if min
is even by using bitwise AND operation with 1. If min
is even, it increments min
by one to move to the next odd number.
The function then checks if min
is greater than max
. If so, it returns 0, indicating there are no odd numbers in this range. Otherwise, it calculates the count of odd numbers using the formula (max - min) / 2 + 1
. This formula works by finding the number of elements between min
and max
and adding 1 because both min
and max
are odd at this point, ensuring the inclusion of all odd counts in half-bounded intervals.
Using this method is efficient as it avoids iterating through the entire range and directly computes the count using arithmetic operations.
class Solution {
public int countOddNumbers(int start, int end) {
// Increment start if it's even.
if ((start & 1) == 0) {
start++;
}
// Calculate the number of odd numbers in the range.
return start > end ? 0 : (end - start) / 2 + 1;
}
}
In the Java program titled "Count Odd Numbers in an Interval Range", the task focuses on calculating the total count of odd numbers between a specified start and end range, inclusive of both endpoints. You use a method countOddNumbers
that accepts two integer parameters: start
and end
.
The implementation involves the following steps:
Check if the start number is even. If so, increment the start by 1 to make it odd. This adjustment ensures that the counting begins from the first odd number greater than or equal to the original start value.
Compute the count of odd numbers within the modified range from the adjusted
start
toend
. The formula(end - start) / 2 + 1
efficiently calculates the number of odd integers. This formula works under the assumption that bothstart
andend
parameters are now odd.Handle scenarios where the modified
start
value might be greater thanend
, which can happen after incrementing an even start. In such instances, the method returns0
as there are no odd numbers within such a defined range.
This compact, efficient solution efficiently determines the count of odd numbers in a given range and demonstrates an effective use of computational logic to minimize the number of evaluations and iterations.
No comments yet.