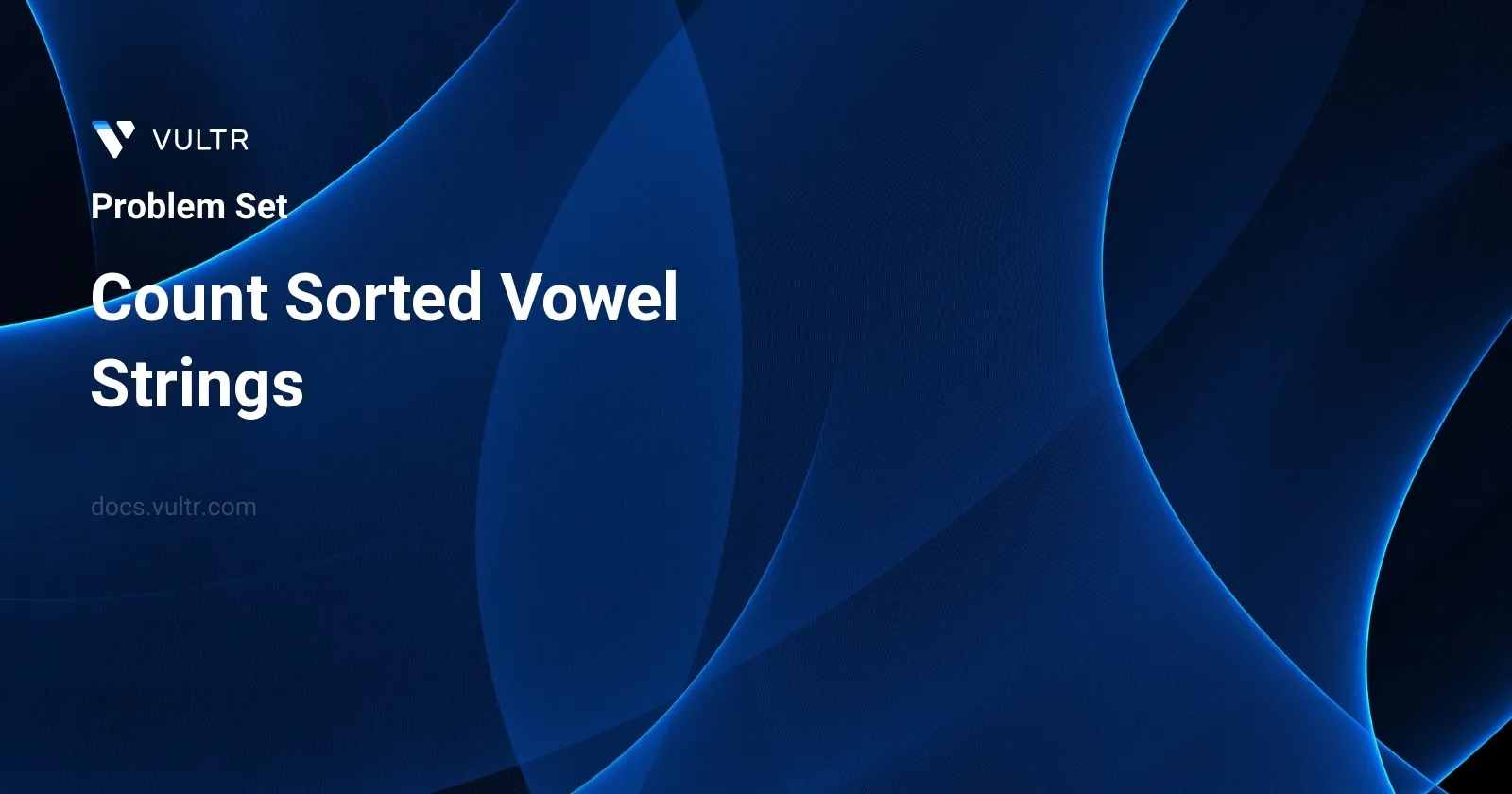
Problem Statement
Given an integer n
, the task is to calculate the number of strings of length n
that are made up solely of the vowels: a
, e
, i
, o
, u
. These strings must adhere to a specific order rule to be considered valid; they must be lexicographically sorted. A string s
meets this criterion if each character s[i]
is either the same as or comes before s[i+1]
in the alphabet. This kind of problem highlights combinatorial logic and the application of combinatorics in string formation under set constraints.
Examples
Example 1
Input:
n = 1
Output:
5
Explanation:
The 5 sorted strings that consist of vowels only are `["a","e","i","o","u"].`
Example 2
Input:
n = 2
Output:
15
Explanation:
The 15 sorted strings that consist of vowels only are ["aa","ae","ai","ao","au","ee","ei","eo","eu","ii","io","iu","oo","ou","uu"]. Note that "ea" is not a valid string since 'e' comes after 'a' in the alphabet.
Example 3
Input:
n = 33
Output:
66045
Constraints
1 <= n <= 50
Approach and Intuition
The examples provided clarify how the outputs are derived based on the input value
n
.- For
n = 1
, every single vowel is a valid string (i.e.,a
,e
,i
,o
,u
). - For
n = 2
, combinations of vowels that are in alphabetical order form valid strings such asaa
,ae
,ai
and so on.
- For
The core observation is that for a string of length
n
to be lexicographically sorted using only the vowels, we are essentially placingn
indistinguishable items (vowels) into 5 distinguishable bins (each bin represents a vowel and all items in a specific bin are of that vowel's type, and bins are ordered lexicographically). The condition that a string must be lexicographically sorted translates into a combinatorial problem of finding combinations with repetition.The problem can be approached combinatorially using:
- The formula for combinations with repetition which is given by "n + k - 1 choose k" where
n
is the number of items to place, andk
is the number of bins. In this context,k
equals 5 (since we have five vowels), andn
is the length of the string. Therefore, it becomes "n + 5 - 1 choose 5".
- The formula for combinations with repetition which is given by "n + k - 1 choose k" where
This formula helps determine the number of ways to distribute
n
indistinguishable objects (vowels of the strings) into 5 distinguishable boxes (the vowel categoriesa
,e
,i
,o
,u
) where the order in each box is not important, but the sequence of boxes themselves follows the lexicographical order. Thus, for any givenn
, this combinatory approach gives the count of valid lexicographically sorted strings.Example analysis:
- For
n = 2
, the combinatorial logic would result in distributing two items into five bins leading to(2 + 5 - 1) choose (5)
which equals15
, matching the output as explained. Forn = 3
, this scales accordingly, illustrating how with increasingn
, the count of valid strings escalates due to the combinatorial possibilities.
- For
Solutions
- C++
- Java
class Solution {
public:
int calculateVowelPermutations(int k) {
return (k + 4) * (k + 3) * (k + 2) * (k + 1) / 24;
}
};
In the provided C++ solution for the problem of counting sorted vowel strings, the approach uses a mathematical formula derived from combinatorics, specifically the combination formula. The solution is encapsulated within a class named Solution
, and it defines a method calculateVowelPermutations
which takes an integer k
as input. The integer k
represents the length of the strings to be considered.
The method computes the number of k-length strings that can be formed by the vowels a, e, i, o, u, where each possible string maintains a non-decreasing order. This is achieved by calculating the combination of 5 vowels taken k
times with repetition, which translates to the formula (k + 4) * (k + 3) * (k + 2) * (k + 1) / 24
. This formula effectively counts the combinations by treating the problem as one of distributing k
indistinguishable items (vowel positions) into 5 distinguishable bins (vowel types), with each bin capable of holding between 0 and k
items.
The method returns this computed value, which represents the total number of valid vowel strings of length k
that are sorted. The division by 24 is derived from the factorial of 4 (4!), which normalizes the overcounted permutations arising from the indistinguishable nature of the items being placed into distinguishable bins.
This concise solution leverages combinatorial mathematics to efficiently solve the problem without requiring iterative string construction or explicit combinatorial enumeration, resulting in a time complexity of O(1).
class Solution {
public int calculateVowelCombinations(int x) {
return (x + 4) * (x + 3) * (x + 2) * (x + 1) / 24;
}
}
The Java implementation provided defines a solution to compute the number of sorted vowel strings of given length using a mathematical approach involving a combination formula. Specifically, the provided method named calculateVowelCombinations
accepts an integer x
, which represents the length of the vowel string, and calculates the result by applying the formula (x + 4) * (x + 3) * (x + 2) * (x + 1) / 24
. This formula is derived from the combinations formula C(n+k-1, k-1)
where n
is the number of vowels (5) and k
is the string length (x
).
- Key steps in the calculation are as follows:
- Add 4, 3, 2, and 1 respectively to the input
x
. - Multiply the resulting values together.
- Divide the product by 24 to compute the number of combinations.
- Add 4, 3, 2, and 1 respectively to the input
This method returns the total count of possible sorted vowel strings of specified length, efficiently using direct computation without the need for loops or recursion. The approach ensures that performances are optimal even for larger values of x
.
No comments yet.