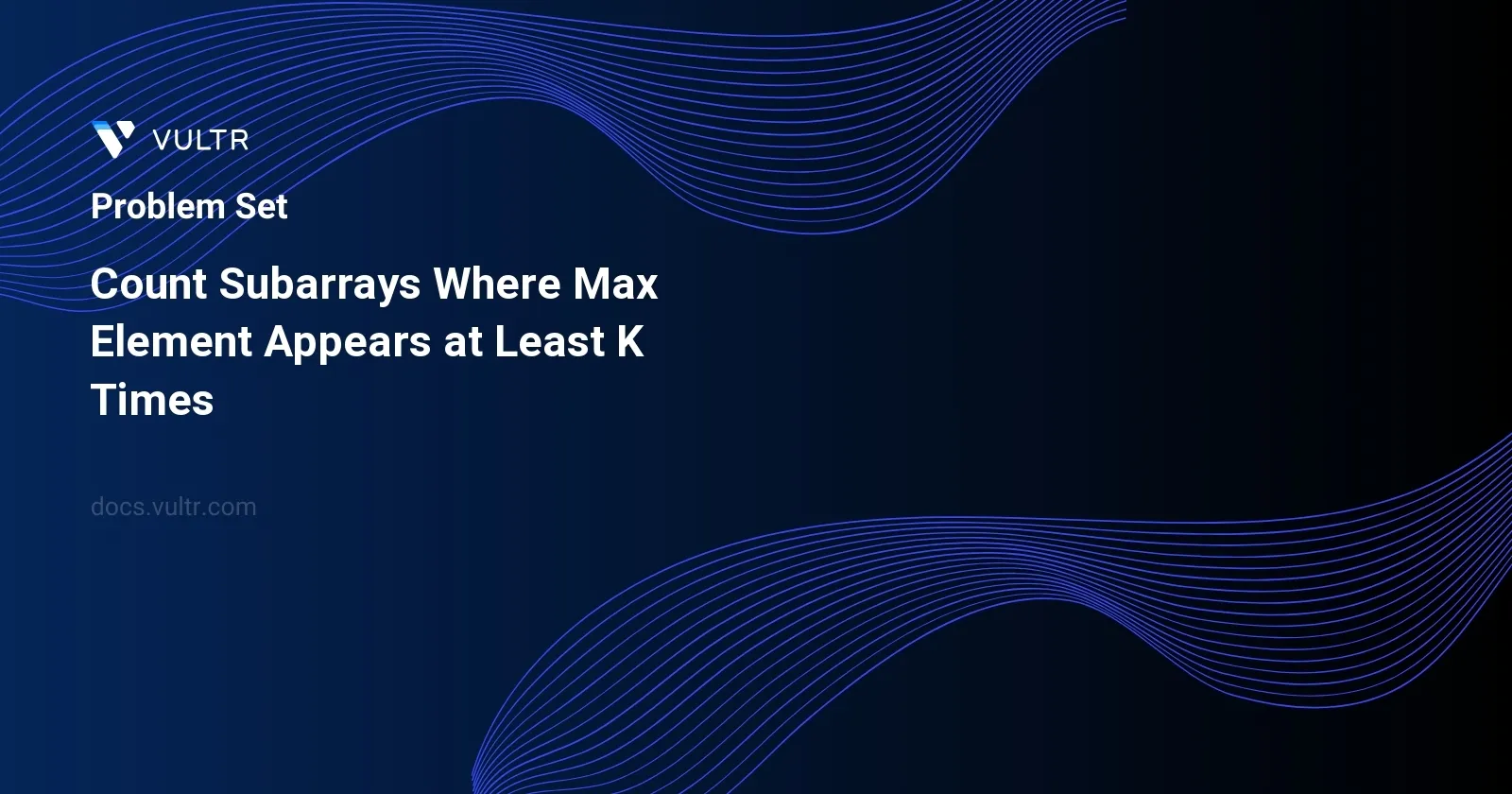
Problem Statement
In this task, you are provided with an integer array nums
and a positive integer k
. Your objective is to calculate how many subarrays exist in which the maximum element of the array appears at least k
times. A subarray is defined as a contiguous part of the main array, maintaining the order of elements as they appear in nums
.
Examples
Example 1
Input:
nums = [1,3,2,3,3], k = 2
Output:
6
Explanation:
The subarrays that contain the element 3 at least 2 times are: [1,3,2,3], [1,3,2,3,3], [3,2,3], [3,2,3,3], [2,3,3] and [3,3].
Example 2
Input:
nums = [1,4,2,1], k = 3
Output:
0
Explanation:
No subarray contains the element 4 at least 3 times.
Constraints
1 <= nums.length <= 105
1 <= nums[i] <= 106
1 <= k <= 105
Approach and Intuition
To solve this problem, consider the following steps and understanding based on the given examples:
- Identify the maximum element in any potential subarray.
- Check if this maximum element appears at least
k
times in that subarray. - Count all such subarrays that meet the criteria.
Analysis based on examples:
For
nums = [1,3,2,3,3]
andk = 2
:- The number (3) is the maximum in various subarrays and appears at least twice. Subarrays like
[1,3,2,3]
,[1,3,2,3,3]
, etc., are valid. - This leads to a count of 6 such subarrays.
- The number (3) is the maximum in various subarrays and appears at least twice. Subarrays like
For
nums = [1,4,2,1]
andk = 3
:- No subarray can have the maximum element appearing at least three times, leading to a count of 0.
Key Constraints to keep in mind:
- The size of the
nums
(nums.length
) can go up to 100,000, implying that efficiency is crucial. - The values within
nums
and the value ofk
can be quite large, up to 1,000,000 and 100,000 respectively. This suggests that handling large values and possibly large arrays is essential for an efficient solution. - Direct brute force approaches that check all subarrays will be inefficient due to the potential high value of
nums.length
. An optimal approach would be necessary to scan through possible subarrays and quickly determine if they meet the criteria without redundant checks.
Solutions
- C++
- Java
- Python
class Solution {
public:
long long computeSubarrays(vector<int>& elements, int threshold) {
int highestValue = *max_element(elements.begin(), elements.end());
vector<int> maxIndices;
long long result = 0;
for (int i = 0; i < elements.size(); i++) {
if (elements[i] == highestValue) {
maxIndices.push_back(i);
}
int count = maxIndices.size();
if (count >= threshold) {
result += maxIndices[count - threshold] + 1;
}
}
return result;
}
};
The provided code consists of a method computeSubarrays
that computes the number of subarrays in which the maximum element appears at least k
times. The method is written in C++ and belongs to the Solution
class.
Start by extracting the highest value in the array using the
max_element
function. This identifies the target value for which the appearances in the subarrays are scrutinized.Initialize a
vector<int>
namedmaxIndices
to store indices where the highest value occurs in the elements vector.Iterate over the vector
elements
. Each time you encounter thehighestValue
, append the current indexi
tomaxIndices
.Maintain a running count of occurrences of the maximum element by keeping track of the size of
maxIndices
.If the count of these occurrences meets or exceeds the threshold
k
, add to the result the number of possible starting positions of subarrays that include the k-th last appearance ofhighestValue
as the maximum. This is calculated asmaxIndices[count - threshold] + 1
.Finally, return the total count stored in
result
. This value represents the number of subarrays where the maximum element appears at leastk
times.
By following this approach, the function efficiently determines and counts suitable subarrays meeting the given condition using a single pass through the input array and leveraging the properties of vector indexing.
class Solution {
public long findSubarrayCountsWithMaxElements(int[] array, int threshold) {
int highestValue = Integer.MIN_VALUE;
for (int num : array) {
if (num > highestValue) {
highestValue = num;
}
}
ArrayList<Integer> maxElementPositions = new ArrayList<>();
long result = 0;
for (int pos = 0; pos < array.length; pos++) {
if (array[pos] == highestValue) {
maxElementPositions.add(pos);
}
int frequency = maxElementPositions.size();
if (frequency >= threshold) {
result += maxElementPositions.get(frequency - threshold) + 1;
}
}
return result;
}
}
This Java solution solves the problem of counting subarrays where the maximum element appears at least 'K' times. It accomplishes this by first identifying the highest value in the array. The method then keeps track of all positions where this maximum element occurs.
Here are the key steps of the implementation process:
- Initialize
highestValue
to the smallest possible integer to ensure any element in the array can update it. - Traverse through the array to identify the maximum value.
- Use a dynamic array,
maxElementPositions
, to store the indices of the array where the maximum value appears. - Iterate through the array again,
- For each position, if the element equals the maximum value, add the position to
maxElementPositions
. - Check if the count of maximum values collected so far meets or exceeds the threshold 'K'.
- If it does, calculate the contribution of the current position to the total count of valid subarrays.
- For each position, if the element equals the maximum value, add the position to
- The results from steps that meet the required condition are tallied to return the total count.
This approach ensures each subarray where the highest element occurs at least 'K' times is considered, leveraging efficient array traversal and dynamic list operations for indexing. The final result represents the total count of such subarrays.
class Solution:
def countSubarrays(self, numbers: List[int], threshold: int) -> int:
highest_value = max(numbers)
max_index_list = []
result_count = 0
for index, value in enumerate(numbers):
if value == highest_value:
max_index_list.append(index)
frequency = len(max_index_list)
if frequency >= threshold:
result_count += max_index_list[-threshold] + 1
return result_count
To tackle the given problem of counting subarrays in which the maximum element appears at least k
times, you need to understand the approach used in the Python solution provided:
First, identify the highest value in the array using the
max()
function. This is crucial because subarrays are defined by the frequency of this maximum value.Store the indices of all occurrences of this maximum element in a list called
max_index_list
. This helps in determining possible subarray starting points.To count the subarrays meeting the condition, iterate through the
numbers
array:- For each element that equals the highest value, add its index to
max_index_list
. - If the count of indices in
max_index_list
is at leastk
, increment theresult_count
by the position that allows the creation of a new valid subarray. This position is calculated asmax_index_list[-k] + 1
, representing the index of the starting element of the new subarray which satisfies the condition.
- For each element that equals the highest value, add its index to
Finally, return the
result_count
which now holds the number of valid subarrays.
This solution leverages indexing and list operations effectively to count subarrays without needing to individually check each potential subarray, thus optimizing performance.
No comments yet.