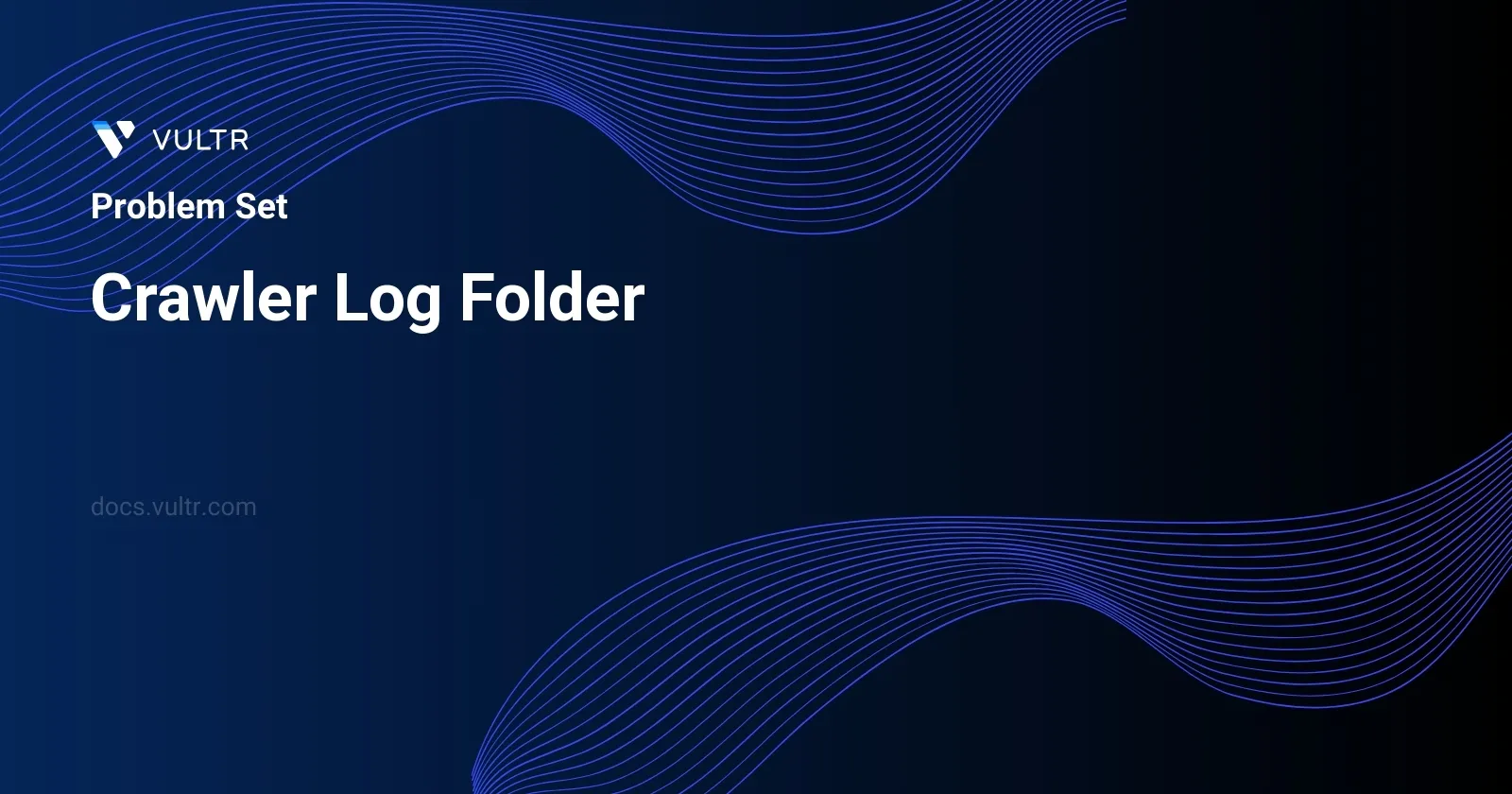
Problem Statement
In the context of navigation within a file system simulated by Vultr, users perform operations that modify their current directory's position. These changes are recorded in a list where each string represents an operation to move within this file system structured hierarchically as folders and subfolders. There are three types of operations:
"../"
denotes moving up to the parent folder. If the operation attempts to move up from the main directory, it is nullified and the position remains unchanged."./"
signifies maintaining the current folder location."x/"
indicates moving into a child folder namedx
, which always exists when requested.
Given a sequence of such operations, the task here is to compute the minimum number of moves required to navigate back to the main folder after all operations have been processed. The complexity arises from maintaining a correct count of folders deep the position is and ensuring actions like moving to a non-existent parent are correctly handled.
Examples
Example 1
Input:
logs = ["d1/","d2/","../","d21/","./"]
Output:
2
Explanation:
Use this change folder operation "../" 2 times and go back to the main folder.
Example 2
Input:
logs = ["d1/","d2/","./","d3/","../","d31/"]
Output:
3
Example 3
Input:
logs = ["d1/","../","../","../"]
Output:
0
Constraints
1 <= logs.length <= 103
2 <= logs[i].length <= 10
logs[i]
contains lowercase English letters, digits,'.'
, and'/'
.logs[i]
follows the format described in the statement.- Folder names consist of lowercase English letters and digits.
Approach and Intuition
To effectively tackle the problem, visualize the directory structure as a tree where each folder can act as a node. The task can be approached by a simulation where a counter tracks the depth of the current directory relative to the main folder:
Initialize a
depth
counter to zero. This will keep track of how deep within the folder structure the current position is.Iterate over the list of folder change operations:
- If the current operation is
"../"
, simulate moving up to the parent directory by decreasing the depth by one, unless the depth is already zero. - If it's
"./"
, ignore the command as it instructs to stay in the current folder. - For operations formatted as
"x/"
, increase the depth by one, representing a move into a child directory.
- If the current operation is
After processing all operations, the depth counter directly indicates how many "../" operations are necessary to return to the main folder, assuming perfect behavior without redundant pop actions when already at the root level.
This approach ensures each operation incurs a constant amount of work, leading to a time complexity linear to the number of operations due to the iteration through the list logs
. Edge cases where the command list is either empty or contains moves that cancel each other out are naturally handled within this iterative approach. The clarity of operation in terms of moving up or down within the file system tree helps in understanding the exact position relative to the main folder at any point, making the final answer straightforward to deduce.
Solutions
- C++
- Java
- Python
class Solution {
public:
int minOperations(vector<string>& logs) {
stack<string> pathStack;
for (auto& log : logs) {
if (log == "../") {
if (!pathStack.empty()) {
pathStack.pop();
}
} else if (log != "./") {
pathStack.push(log);
}
}
return pathStack.size();
}
};
The provided solution handles the problem of simulating a file system crawler's traversal through log folders, each represented by a string in a vector. Written in C++, the solution makes use of a stack to track the directory level the crawler is at after processing all operations listed in the logs.
The function, minOperations
, accepts a vector of strings wherein each log entry can be:
- "../" indicating moving back to the parent directory,
- "./" representing the current directory,
- or a folder name indicating moving into that folder.
The core logic involves iterating through each log entry and using the stack data structure to either push a new log folder, pop the stack when moving back, or do nothing when staying in the current folder. Here's a clear stepwise breakdown of the operations:
Initialize a
stack<string>
to hold the path history.Iterate over each log in the input vector:
- If the log is "../" and the stack is not empty, pop the top entry from the stack.
- If the log is a directory name (i.e., not "./" or "../"), push it onto the stack.
At the end of the loop, return the size of the stack. This represents the minimum number of operations needed to return to the main folder after processing all the log entries.
This approach efficiently leverages the stack's LIFO (Last In, First Out) property to backtrack correctly through the directories and avoids any unnecessary operations for entries referring to the current directory.
class Solution {
public int findDepth(String[] operations) {
Stack<String> directoryStack = new Stack<>();
for (String currentOperation : operations) {
if (currentOperation.equals("../")) {
if (!directoryStack.isEmpty()) {
directoryStack.pop();
}
} else if (!currentOperation.equals("./")) {
directoryStack.push(currentOperation);
}
}
return directoryStack.size();
}
}
The problem "Crawler Log Folder" focuses on determining the minimum steps required to return to the root folder after performing several directory operations represented by strings. The solution uses Java and involves the following:
Define the Method: The
findDepth
method takes an array of stringsoperations
as input, each string representing an operation on the folder structure.Initialize a Stack: A
Stack<String>
nameddirectoryStack
is used to track the depth of the directory structure, pushing and popping directories based on the operations performed.Process Each Operation:
- Loop through each operation represented by the string array.
- If the operation is
"../"
(move up one directory), pop the stack if it's not empty, effectively moving up one level. - If the operation is
"./"
(stay in the current directory), ignore this operation. - Any other operation (assumed to represent moving into a new directory), push this operation onto the stack.
Return Stack Size: After processing all operations, the size of the stack indicates the depth of the final directory level relative to the root.
By the end of this process, obtain the number of steps required to move from the root to the final directory location by returning the size of the directoryStack
. This approach effectively handles all directory moves, correctly mimicking the file navigation.
class Solution:
def calculateDepth(self, operations: List[str]) -> int:
path_depth = []
for operation in operations:
if operation == "../":
if path_depth:
path_depth.pop()
elif operation != "./":
path_depth.append(operation)
return len(path_depth)
The solution for the "Crawler Log Folder" problem calculates the depth of a folder after executing a series of operations. The code is written in Python and utilizes a list to simulate the operations on the folder structure. Each operation in the list either navigates to a parent directory, remains in the current directory, or moves into a subdirectory. Here's how the solution works:
- Initialize an empty list
path_depth
to represent the stack of operations. - Iterate through each operation in the input list
operations
.- If the operation is
"../"
, check ifpath_depth
is not empty to pop the last operation (move to the parent directory). - If the operation is not
"./"
, append it topath_depth
as it indicates moving to a new subdirectory.
- If the operation is
- After processing all operations, the depth of the folder is given by the length of
path_depth
, which is returned as the result.
This approach ensures that any operation leading outside the root directory is ignored, and keeps track of the depth purely based on valid navigational operations. The method is efficient, utilizing list operations to dynamically simulate the folder navigation.
No comments yet.