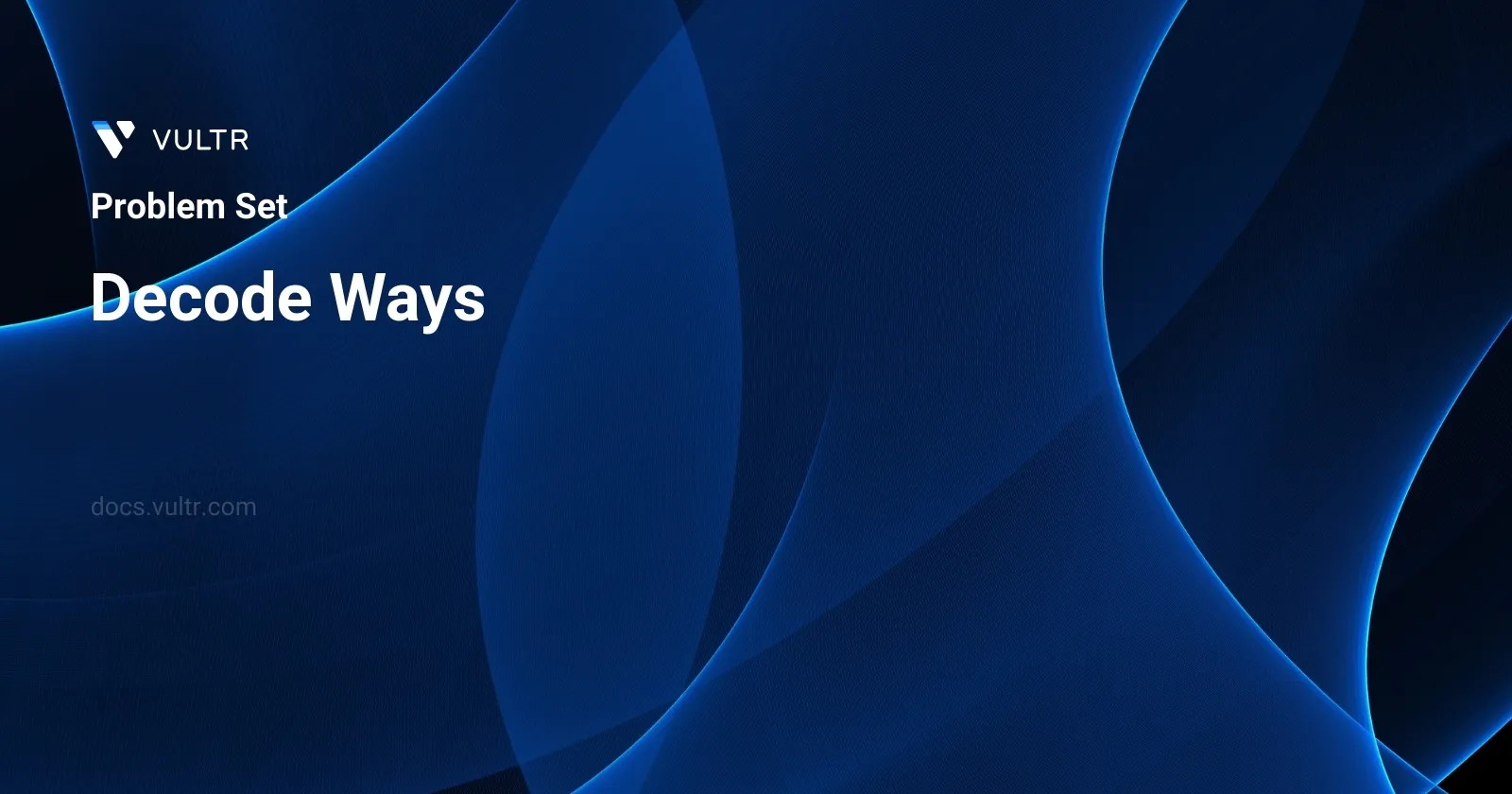
Problem Statement
Decoding a secret message from a sequence of digits is an interesting challenge, primarily because of the varied number of ways one can interpret these digits based on predefined mappings. Each digit from '1' to '9' is mapped to a corresponding letter from 'A' to 'I', and pairs of digits from '10' to '26' map to letters from 'J' to 'Z'. However, the complexity arises in the interpretation of these mappings when digits can be combined or separated in different ways.
For instance, considering a digit string "11106", it poses multiple decoding interpretations such as:
"AAJF"
corresponding to the digit grouping(1, 1, 10, 6)
"KJF"
corresponding to the grouping(11, 10, 6)
However, not all groupings are valid due to rules such as not recognizing digits with leading zeros (e.g., 06
is invalid). Given a string s
entirely composed of numbers, the task is to determine how many distinct valid ways there are to decode this string. If no valid interpretation exists due to the constraints (like presence of non-decodable sequences), the answer should be 0
.
The challenge is to compute the valid ways to decode considering all potential valid and invalid groupings and differentiate cases where no valid decoding exists.
Examples
Example 1
Input:
s = "12"
Output:
2
Explanation:
"12" could be decoded as "AB" (1 2) or "L" (12).
Example 2
Input:
s = "226"
Output:
3
Explanation:
"226" could be decoded as "BZ" (2 26), "VF" (22 6), or "BBF" (2 2 6).
Example 3
Input:
s = "06"
Output:
0
Explanation:
"06" cannot be mapped to "F" because of the leading zero ("6" is different from "06"). In this case, the string is not a valid encoding, so return 0.
Constraints
1 <= s.length <= 100
s
contains only digits and may contain leading zero(s).
Approach and Intuition
The digit to letter mapping described can be approached using a dynamic programming method, which efficiently handles the repetitive substructure and overlaps typical in this problem. Here's a basic rundown of the proposed approach:
- Initialize an array
dp
where each indexi
holds the number of ways to decode the string up to that position. - If the string starts with zero, immediately return
0
as any leading zero renders the encoding invalid. - Set the base case:
dp[0]=1
by convention (one way to decode an empty string). - Iterate through the string:
- For a single digit: Check if it's non-zero (since '0' has no corresponding letter when it stands alone) and add the number of ways to decode up to the previous character.
- For a two-digit number formed with the current and the previous digit: Check if it lies between '10' and '26' (inclusive) to ensure that it maps to a valid letter. If valid, add to the number of ways considering the number two positions back.
- The constraints specify handling for up to 100 characters, and since the loop runs for each character in the string, the approach sufficiently covers all possible string lengths as required.
- Various edge cases such as strings containing non-decodable sequences are naturally handled by ensuring no addition of ways during the zero checks.
Following this method ensures that all possible valid groupings of numbers into corresponding letters are considered, and invalid paths are not counted, thereby accurately counting and returning the total number of ways to decode the message.
Solutions
- C++
- Java
- C
- JavaScript
- Python
class Solution {
public:
int decodeWays(string seq) {
if (seq[0] == '0') return 0;
size_t len = seq.size();
int previous = 1, beforePrevious = 1;
for (size_t idx = 1; idx < len; idx++) {
int count = 0;
if (seq[idx] != '0') {
count = beforePrevious;
}
int pair = stoi(seq.substr(idx - 1, 2));
if (pair >= 10 && pair <= 26) {
count += previous;
}
previous = beforePrevious;
beforePrevious = count;
}
return beforePrevious;
}
};
The "Decode Ways" solution in C++ focuses on counting how many ways a given sequence of digits can be decoded, assuming each number corresponds to a letter (similar to how '1' to '26' would map to 'A' to 'Z'). The function decodeWays
implements a dynamic programming approach to solve the problem efficiently.
Here's a breakdown of the logic:
- Start by handling cases where the sequence begins with '0', as no valid encoding is possible. Immediately return
0
. - Initialize two integer variables,
previous
andbeforePrevious
, both set to1
. These track the number of ways to decode subsequences up to the current and previous positions. - Iterate through the string starting from the second character (since single-digit decoding is straightforward).
- For each character, determine how many new ways to decode are possible:
- Check if the current character is not '0' because '0' does not correspond to any letter by itself but is valid in combinations like '10' or '20'.
- Convert the current and previous characters to a double-digit number. If this number is between 10 and 26, it validly maps to a letter, hence increasing the count of decoding ways by adding the number of ways up to the previous character.
- Update the variables
previous
andbeforePrevious
for the next iteration.
The function ultimately returns beforePrevious
which holds the total number of ways the full sequence can be decoded.
This approach ensures that each potential decoding is counted precisely, handling cases where single or double-digit numbers can form valid mappings. Thus, the program efficiently calculates the number without generating all possible strings but by dynamically adjusting the count of possible decodings.
class Solution {
public int decodeWays(String sequence) {
if (sequence.charAt(0) == '0') {
return 0;
}
int length = sequence.length();
int prevPrev = 1;
int prev = 1;
for (int index = 1; index < length; index++) {
int currentDecode = 0;
if (sequence.charAt(index) != '0') {
currentDecode = prev;
}
int pairNum = Integer.parseInt(sequence.substring(index - 1, index + 1));
if (pairNum >= 10 && pairNum <= 26) {
currentDecode += prevPrev;
}
prevPrev = prev;
prev = currentDecode;
}
return prev;
}
}
The Java method decodeWays
in the provided code interprets a string of digits as encoding options, similar to how letters are encoded in telephone keypads. The method returns the number of ways to decode the given string based on certain constraints. Here's how the algorithm functions:
- First, the method checks if the string starts with '0'. If it does, there are no valid ways to decode it, so it returns 0.
- Two integer variables,
prevPrev
andprev
, are initialized to 1. These are used to store the counts of decode ways from previous computations. - A loop runs from the second character to the last character of the string. Within this loop:
currentDecode
is initialized to 0 for each character.- If the current character is not '0',
currentDecode
is updated to the value ofprev
(which represents the number of ways to decode the substring ending at the previous character). - The algorithm then checks if the two-character substring ending at the current character (composed of the current character and its predecessor) represents a valid pair number between 10 and 26. If valid,
currentDecode
is incremented byprevPrev
(ways to decode excluding the last two characters). - The values of
prevPrev
andprev
are updated for the next iteration.
At the end of the loop, prev
holds the total number of ways to decode the entire string, which is then returned. This solution effectively uses dynamic programming principles to build up the number of decodings iteratively, thereby achieving a time-efficient decoding process.
int decodeWays(char* str) {
if (str[0] == '0') return 0;
int length = strlen(str);
int prev = 1, nextToPrev = 1;
for (int index = 1; index < length; index++) {
int sum = 0;
if (str[index] != '0') {
sum = nextToPrev;
}
char pairDigits[3] = {str[index - 1], str[index], '\0'};
int pairNum = atoi(pairDigits);
if (pairNum >= 10 && pairNum <= 26) {
sum += prev;
}
prev = nextToPrev;
nextToPrev = sum;
}
return nextToPrev;
}
The provided C code illustrates a function decodeWays
designed to compute the number of ways a given numeric string can be decoded, adhering to rules similar to the encoding schemes where 'A' to 'Z' correspond to '1' to '26'.
- The function first checks if the initial character of the string is '0'. Since there's no corresponding letter for '0', the function immediately returns 0.
- It determines the length of the string to manage the iteration over the characters.
- Two integer variables,
prev
andnextToPrev
, are initialized to 1. These track the count of decoding possibilities up to the current and previous characters respectively. - Inside a for loop, which iterates beginning from the second character of the string, it initializes an integer
sum
to 0. This variable accumulates the number of ways the substring up to the current index can be decoded. - If the current character isn't '0',
sum
is updated with the value ofnextToPrev
because the current character could represent a valid letter alone. - The code then constructs a two-character array
pairDigits
containing the current and previous characters, terminated by a null character. This array is converted to an integerpairNum
usingatoi
. - If
pairNum
falls between 10 and 26, both inclusive, it's a valid two-digit encoding, sosum
is incremented by the value ofprev
. - To proceed to the next iteration,
prev
is updated tonextToPrev
andnextToPrev
is updated tosum
.
Finally, after the loop completes, nextToPrev
is returned. This value represents the total number of ways the entire string can be decoded. This function efficiently uses dynamic programming principles, particularly memoization, to ensure each substring is only processed once, achieving optimal performance.
var decodeWays = function (encoded) {
if (encoded.charAt(0) === "0") {
return 0;
}
let len = encoded.length;
let prevPrev = 1;
let prev = 1;
for (let index = 1; index < len; index++) {
let temp = 0;
if (encoded.charAt(index) !== "0") {
temp = prev;
}
let doubleDigit = parseInt(encoded.slice(index - 1, index + 1));
if (doubleDigit >= 10 && doubleDigit <= 26) {
temp += prevPrev;
}
prevPrev = prev;
prev = temp;
}
return prev;
};
The JavaScript function decodeWays
is designed to determine the number of ways a given numeric string can be decoded, considering each number could represent a letter according to a predetermined mapping (similar to how 'A' might be 1, 'B' is 2, etc.). Here's a breakdown of how this function operates:
- First, check if the first character of the input string is "0". If so, return 0, as no valid encoding starts with zero.
- Initialize variables
prevPrev
andprev
to 1. These will keep track of the number of ways to decode the string up to the characters at the current index minus two and minus one, respectively. - Loop through the encoded string starting from the second character. For each character:
- Set a temporary variable
temp
to zero. - If the character is not "0", update
temp
to the value ofprev
. This indicates that current digit can be used as a standalone decode unit, thus inheriting the decode possibilities of the previous sequence. - Check the two-digit number formed with the current and the previous character. If it's between 10 and 26, it can represent a letter. Increase
temp
by the value ofprevPrev
, adding the number of ways to decode considering this two-character combination.
- Set a temporary variable
- Update
prevPrev
with the value ofprev
, andprev
with the value oftemp
for the next iteration of the loop. - After completing the loop,
prev
will contain the total number of ways to decode the entire string.
This approach uses dynamic programming to efficiently solve the problem by breaking it down into smaller sub-problems, each depending only on the last couple of preceding solutions. Thus, the algorithm runs with a complexity of O(n), where n is the length of the input string.
class Solution:
def decodeWays(self, data: str) -> int:
if data[0] == "0":
return 0
prev = 1
prev_prev = 1
for index in range(1, len(data)):
count = 0
if data[index] != "0":
count = prev
pair = int(data[index - 1 : index + 1])
if 10 <= pair <= 26:
count += prev_prev
prev_prev = prev
prev = count
return prev
The provided Python code solves the problem of counting the number of ways a numeric string can be decoded into characters. Assume each number corresponds to a letter (e.g., '1' is 'A', '2' is 'B', ..., '26' is 'Z'). This script uses dynamic programming to efficiently compute the solution by iterating through the string and calculating the number of ways it can be decoded up to each position.
Follow these main points in the code:
- Initialize two variables,
prev
andprev_prev
, to keep track of the ways to decode the string up to the current and the previous position. - Iterate through each character of the string starting from the second character.
- Set
count
to zero. If the current character is not '0', setcount
to the value ofprev
since the current character alone can be decoded. - Check if the current and the previous characters together form a number between 10 and 26. If so, add the value of
prev_prev
tocount
because this pair can be a valid character. - Update
prev_prev
to the value ofprev
, and then updateprev
to the value ofcount
.
- Set
- Return the value of
prev
, which represents the total number of ways to decode the entire string.
This method is efficient with a time complexity of O(n), where n is the length of the string, since it processes each character exactly once.
No comments yet.