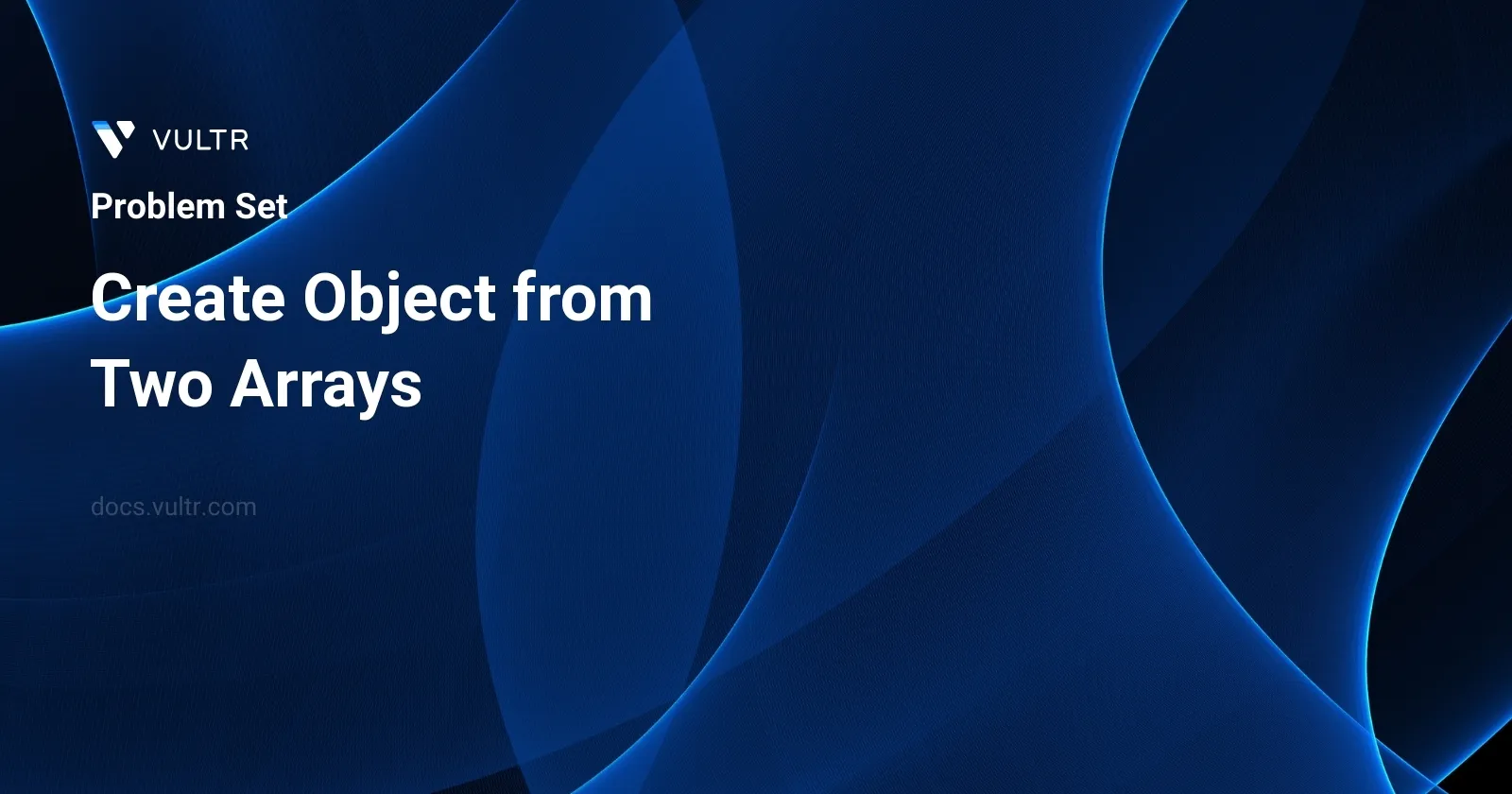
Problem Statement
In the problem, you are given two arrays: keysArr
and valuesArr
. You need to create and return a new object where each key-value pair is determined by correlating keysArr[i]
with valuesArr[i]
. The complexity is added by the conditions that:
- If a key already exists in the object (due to a previous occurrence in
keysArr
), that key-value pair is omitted, ensuring only the first occurrence of a key is used. - Whenever a key is not traditionally a string type, it must be explicitly converted into a string using the
String()
method. This processing ensures the flexibility in handling various data types as keys.
Examples
Example 1
Input:
keysArr = ["a", "b", "c"], valuesArr = [1, 2, 3]
Output:
{"a": 1, "b": 2, "c": 3}
Explanation:
The keys "a", "b", and "c" are paired with the values 1, 2, and 3 respectively.
Example 2
Input:
keysArr = ["1", 1, false], valuesArr = [4, 5, 6]
Output:
{"1": 4, "false": 6}
Explanation:
First, all the elements in keysArr are converted into strings. We can see there are two occurrences of "1". The value associated with the first occurrence of "1" is used: 4.
Example 3
Input:
keysArr = [], valuesArr = []
Output:
{}
Explanation:
There are no keys so an empty object is returned.
Constraints
keysArr
andvaluesArr
are valid JSON arrays2 <= JSON.stringify(keysArr).length, JSON.stringify(valuesArr).length <= 5 * 105
keysArr.length === valuesArr.length
Approach and Intuition
1. Understanding the Output Relationship
From the provided examples, each key from keysArr
maps directly to the value at the same index in valuesArr
. The object is formed by associating these pairs, and conditionally converting any key to a string format ensures consistency in the object's structure.
2. Handling Duplicates
To abide by the constraint of ignoring subsequent duplicate keys, we must first check if a key has already been added to the object. If so, skip to the next pair, otherwise add the new unique key.
- Approach:
- Initialize an empty object.
- Iterate over the length of the
keysArr
. - Convert each key to string (if not already).
- If the key isn't already a property of the object, add it with its corresponding value.
- Proceed to the next key-value pairs.
3. Edge Cases
Consider the scenario where keysArr
is empty. Ideally, the function should return an empty object {}, indicating that there were no keys to process.
- This clean and systematic handling ensures that regardless of the original data types in
keysArr
or any duplicative keys, the function creates a well-formed object with the expected mappings and maintains the integrity and uniqueness of keys.
Solutions
- JavaScript
/**
* @param {Array} keyList
* @param {Array} valueList
* @return {Object}
*/
var buildMap = function(keyList, valueList) {
const map = {};
for (const index in keyList) {
if (!map.hasOwnProperty(keyList[index])) {
map[keyList[index]] = valueList[index];
}
}
return map;
};
Create an object in JavaScript by mapping keys from one array to values from another using the provided function buildMap
. This function accepts two parameters, keyList
and valueList
, and iterates through the keyList
to add entries to an object if the key does not already exist in the object.
Follow these steps to utilize the function:
- Define
keyList
andvalueList
arrays with matching indices where each element in thekeyList
corresponds to an element in thevalueList
at the same index. - Call
buildMap
by passing these arrays as arguments. - Receive the resulting object where each key from
keyList
is mapped to a value fromvalueList
.
Ensure that both arrays are of the same length to avoid mismatches or undefined values. The function uses a for-in loop and the hasOwnProperty
method to avoid overriding existing keys in the object, making it safe against duplicate keys in the keyList
. Each new key-value pair is only added if the key doesn't already exist in the object, preserving initial mappings for keys that appear more than once in the input.
No comments yet.