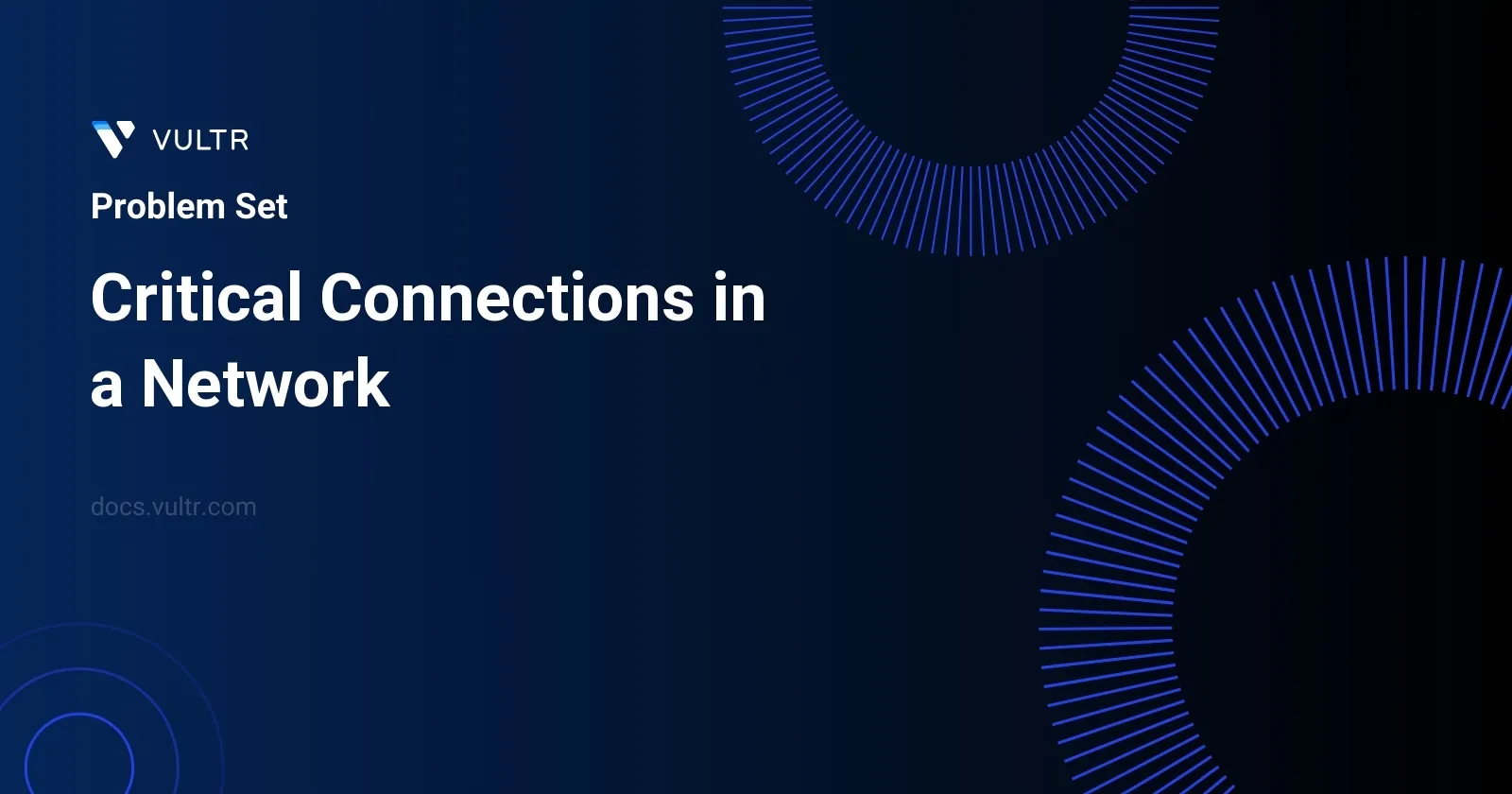
Problem Statement
In the given problem, we have a network consisting of n
servers, each labeled from 0
to n-1
. These servers are interconnected by several undirected server-to-server connections, each being represented as a pair [ai, bi]
. This indicates there is a direct communication link between servers ai
and bi
. It's possible for any server to communicate with any other server through a series of these connections, either directly or indirectly.
Specifically, a connection is termed as critical if removing it prevents at least one server from being able to reach another server, which otherwise could have been reachable when the connection was intact. The task is to identify all such critical connections in the server network.
Examples
Example 1
Input:
n = 4, connections = [[0,1],[1,2],[2,0],[1,3]]
Output:
[[1,3]]
Explanation:
[[3,1]] is also accepted.
Example 2
Input:
n = 2, connections = [[0,1]]
Output:
[[0,1]]
Constraints
2 <= n <= 105
n - 1 <= connections.length <= 105
0 <= ai, bi <= n - 1
ai != bi
- There are no repeated connections.
Approach and Intuition
In solving this problem, we aim to identify the connections that, if removed, would make the servers originally reachable through the network become unreachable. Here's a step-by-step intuitive guide on how one can think about indeed solving this:
Graph Representation: The network of servers can be modeled as an undirected graph where each server is a node and each connection is an edge.
Using Depth First Search (DFS): Through a depth-first search, we can traverse the graph. Here, for each node (or server), we will:
- Keep track of the servers visited.
- Mark the connections or edges visited.
- Establish the minimum reachability of each server based on the DFS traversal.
Articulation Points and Bridges: Critical connections in such a graph can be identified as graph bridges. A bridge in an undirected graph is an edge removing which increases the number of disconnected components in the graph.
- Employ Tarjan’s algorithm, an efficient graph search that can find all bridges (critical connections) in a graph comprehensively.
- This involves exploring depths and low-link values: The depth is a timestamp of when we visit the node during DFS, and low-link values help indicate the highest-up node (in terms of DFS traversal) reachable back from a node.
Processing Constraints: Given the constraints, where the maximum number of servers (
n
) and connections can go up to10^5
, a direct brute force approach of repeatedly removing each connection and testing for connectivity is computationally expensive and impractical. Instead, using the described graph traversal technique (DFS along with Tarjan’s algorithm) is both time-efficient and feasible.
By employing the above method, we can systematically explore the given server network, identify bridge connections, i.e., critical connections, effectively, and solve the problem within the provided constraints.
Solutions
- Java
- Python
class Network {
private Map<Integer, List<Integer>> adjacencyList;
private Map<Integer, Integer> visitOrder;
private Map<Pair<Integer, Integer>, Boolean> criticalEdges;
public List<List<Integer>> criticalConnections(int vertexCount, List<List<Integer>> edgeList) {
this.buildGraph(vertexCount, edgeList);
this.runDFS(0, 0);
List<List<Integer>> outputs = new ArrayList<List<Integer>>();
for (Pair<Integer, Integer> criticalConnection : this.criticalEdges.keySet()) {
outputs.add(new ArrayList<Integer>(Arrays.asList(criticalConnection.getKey(), criticalConnection.getValue())));
}
return outputs;
}
private int runDFS(int vertex, int order) {
// Return if visited
if (this.visitOrder.get(vertex) != null) {
return this.visitOrder.get(vertex);
}
// Set the discovery order of vertex
this.visitOrder.put(vertex, order);
// Initialize minimum rank with its next possible highest rank
int lowestRank = order + 1;
for (Integer adjacentVertex : this.adjacencyList.get(vertex)) {
// Skip reverse to parent.
Integer backRank = this.visitOrder.get(adjacentVertex);
if (backRank != null && backRank == order - 1) {
continue;
}
// Explore DFS for adjacent vertex
int resultantRank = this.runDFS(adjacentVertex, order + 1);
// Remove edge if cycle detected
if (resultantRank <= order) {
int lower = Math.min(vertex, adjacentVertex), higher = Math.max(vertex, adjacentVertex);
this.criticalEdges.remove(new Pair<Integer, Integer>(lower, higher));
}
// Update the lowest found rank.
lowestRank = Math.min(lowestRank, resultantRank);
}
return lowestRank;
}
private void buildGraph(int vertexCount, List<List<Integer>> edgeList) {
this.adjacencyList = new HashMap<Integer, List<Integer>>();
this.visitOrder = new HashMap<Integer, Integer>();
this.criticalEdges = new HashMap<Pair<Integer, Integer>, Boolean>();
for (int i = 0; i < vertexCount; i++) {
this.adjacencyList.put(i, new ArrayList<Integer>());
this.visitOrder.put(i, null);
}
for (List<Integer> edge : edgeList) {
// Graph is bidirectional
int u = edge.get(0), v = edge.get(1);
this.adjacencyList.get(u).add(v);
this.adjacencyList.get(v).add(u);
int lower = Math.min(u, v), higher = Math.max(u, v);
criticalEdges.put(new Pair<Integer, Integer>(lower, higher), true);
}
}
}
In the Java program provided, the objective is to efficiently identify critical connections (bridges) in a network. A bridge in a network is an edge, removal of which increases the number of connected components.
Implement the solution using Depth-First Search (DFS) and leveraging Tarjan's algorithm for finding bridges in a graph. Here is a concise breakdown of the process:
Initialize data structures including
adjacencyList
to manage the graph as a list of edges connected to each vertex,visitOrder
to track the order of nodes visited in DFS, andcriticalEdges
to store edges that are identified as critical.Construct the graph from the input list of edges. For every edge connecting vertices
u
andv
, add each vertex to the other's adjacency list, and mark the edge as a potential critical edge.Launch the DFS from the first vertex (considered vertex 0 in 0-based index). For each vertex exploration, track the lowest order vertex that can be reached from the subtree rooted at the current vertex.
As you iterate through adjacent vertices, avoid revisiting the immediate predecessor in DFS (skip the reverse edge to the parent). If the recursive DFS call finds a connection back to one of the ancestors (indicating a cycle), remove that edge from
criticalEdges
.Continue exploring until all vertices are visited. Finally, convert the set of critical edges into the desired output format and return it.
This method ensures that each edge is examined minimally, keeping the algorithm efficient. The critical edges represent non-redundant connections, vital for maintaining network connectivity, making them indispensable in network architecture analysis, especially in large distributed systems.
class CriticalPathFinder:
vertex_rank = {}
adjacency_list = defaultdict(list)
critical_connections_map = {}
def findCriticalConnections(self, total_nodes: int, linkages: List[List[int]]) -> List[List[int]]:
self.constructGraph(total_nodes, linkages)
self.explore(0, 0)
results = []
for start, end in self.critical_connections_map:
results.append([start, end])
return results
def explore(self, vertex: int, current_rank: int) -> int:
if self.vertex_rank[vertex]:
return self.vertex_rank[vertex]
self.vertex_rank[vertex] = current_rank
lowest_rank = current_rank + 1
for neighbor in self.adjacency_list[vertex]:
if self.vertex_rank[neighbor] and self.vertex_rank[neighbor] == current_rank - 1:
continue
recursive_depth = self.explore(neighbor, current_rank + 1)
if recursive_depth <= current_rank:
del self.critical_connections_map[(min(vertex, neighbor), max(vertex, neighbor))]
lowest_rank = min(lowest_rank, recursive_depth)
return lowest_rank
def constructGraph(self, total_nodes: int, linkages: List[List[int]]):
self.vertex_rank = {}
self.adjacency_list = defaultdict(list)
self.critical_connections_map = {}
for vertex_index in range(total_nodes):
self.vertex_rank[vertex_index] = None
for edge in linkages:
u, v = edge[0], edge[1]
self.adjacency_list[u].append(v)
self.adjacency_list[v].append(u)
self.critical_connections_map[(min(u, v), max(u, v))] = 1
The provided Python code outlines a method for identifying critical connections in a network using a depth-first search strategy. This solution involves several principal components and operations:
Graph Construction: The program accepts the total number of nodes and a list of connections (linkages) to form a graph. It stores the graph in an adjacency list for efficient traversal and also maintains a map of connections, marking each one as visited or processed.
Depth-First Search (DFS): The crux of finding critical connections lies in the depth-first search. The
explore
method is used recursively to navigate through the graph. It maintains a ranking of vertices to identify back edges and determine if a connection is critical. If traversing a connection between two vertices leads to a vertex ranked lower than the current vertex's rank (indicating a cycle or a back edge), then that specific connection is not critical.Detection and Storage of Critical Connections: As the DFS is executed, connections between nodes are dynamically marked as critical or non-critical based on their participation in forming cycles. Only those edges which do not form a cycle are deemed critical. These connections are captured and returned as lists.
Initialization and Result Compilation: Different data structures such as dictionaries and lists are utilized to store node ranks, adjacency details, and critical connections. The initial state begins with each node unvisited, executing the DFS from the first node and progressing through the network.
Remember to initialize and pass the right parameters for the functions to effectively identify all the critical connections in a given network configuration. This approach ensures an efficient computation leveraging depth-first search to identify network vulnerabilities or crucial links.
No comments yet.