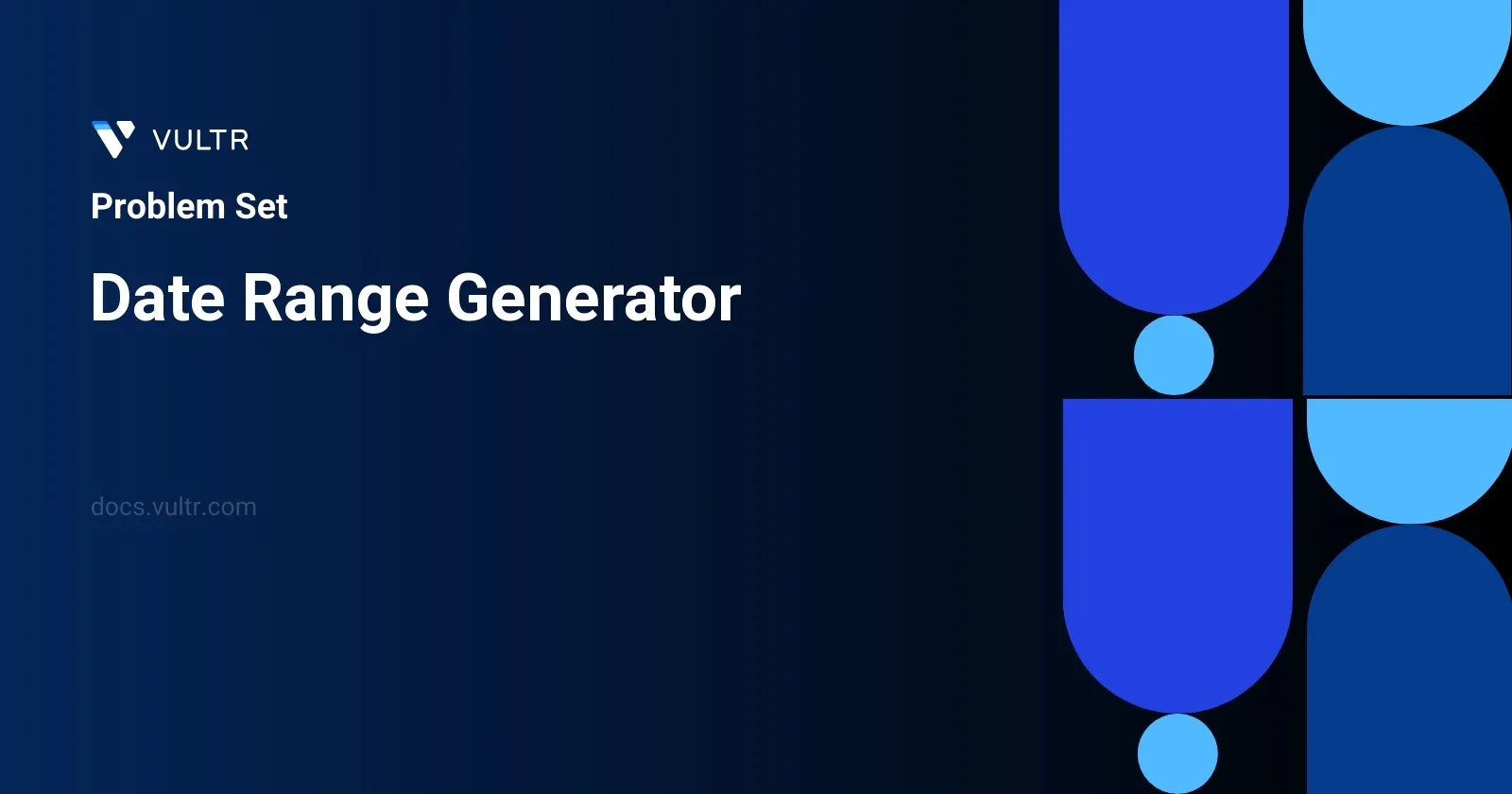
Problem Statement
This task involves creating a generator that yields a sequence of dates from a specified start
date to an end
date, inclusive. The important aspect here is the step
parameter, which determines how many days to skip between each date. Each date in the sequence should adhere to the YYYY-MM-DD
format. The implementation should be robust enough to accommodate different ranges and step sizes, ensuring that the boundaries (start and end dates) are respected and the output is within the given constraints.
Examples
Example 1
Input:
start = "2023-04-01", end = "2023-04-04", step = 1
Output:
["2023-04-01","2023-04-02","2023-04-03","2023-04-04"]
Explanation:
const g = dateRangeGenerator(start, end, step); g.next().value // '2023-04-01' g.next().value // '2023-04-02' g.next().value // '2023-04-03' g.next().value // '2023-04-04'
Example 2
Input:
start = "2023-04-10", end = "2023-04-20", step = 3
Output:
["2023-04-10","2023-04-13","2023-04-16","2023-04-19"]
Explanation:
const g = dateRangeGenerator(start, end, step); g.next().value // '2023-04-10' g.next().value // '2023-04-13' g.next().value // '2023-04-16' g.next().value // '2023-04-19'
Example 3
Input:
start = "2023-04-10", end = "2023-04-10", step = 1
Output:
["2023-04-10"]
Explanation:
const g = dateRangeGenerator(start, end, step); g.next().value // '2023-04-10'
Constraints
new Date(start) <= new Date(end)
start
andend
dates are in the string formatYYYY-MM-DD
0 <= The difference in days between the start date and the end date <= 1500
1 <= step <= 1000
Approach and Intuition
To solve this problem, understanding both the problem domain (dates) and the specific coding constructs (generators) is necessary. Let's break down the approach based on the provided examples and constraints:
Understanding Date Manipulation: The core operation involves advancing a date by a certain number of days (
step
). This requires a fundamental understanding of date operations in programming, which can often be tricky due to issues like leap years and month variations.Generator Basics: Generators are used here because they allow for lazy evaluation, only computing each next date when needed. This is efficient in terms of memory and computation, especially valuable when the range of dates is large but only a few values are used.
Iterative Solution:
- Start from the given
start
date. - Increment the date by
step
days until (or while) the current date is less than or equal to theend
date. - Yield each computed date in the standard format
YYYY-MM-DD
.
- Start from the given
Handling Edge Cases:
- The smallest possible
step
is one day, which would mean returning consecutive dates. - If the start date is the same as the end date and
step
is 1, only a single date should be returned, as observed in Example 3.
- The smallest possible
Validation and Constraints:
- Ensure that the start date is not later than the end date to comply with the constraint
new Date(start) <= new Date(end)
. - The range of possible differences in days (up to 1500) and the range for the step size (1 to 1000) suggest the need for checking that the step does not overshoot the end date in a single increment.
- Ensure that the start date is not later than the end date to comply with the constraint
By understanding and synthesizing these elements, the implementation can effectively leverage Python's datetime and generator capabilities to provide a clean and efficient solution to the problem.
Solutions
- JavaScript
/**
* @param {string} begin
* @param {string} finish
* @param {number} increment
* @yields {string}
*/
var generateDateRange = function* (begin, finish, increment) {
const from = new Date(begin);
const to = new Date(finish);
while (from <= to) {
yield from.toISOString().split('T')[0].trim();
from.setDate(from.getDate() + increment);
}
};
Implement a function in JavaScript to generate a range of dates between specified start and end dates with a defined increment. The function is a generator named generateDateRange
which accepts three arguments: begin
, finish
, and increment
.
begin
: A string representing the start date.finish
: A string representing the end date.increment
: A number that specifies the number of days to add to each successive date in the range.
The function is structured as follows:
- Convert the start (
begin
) and end (finish
) date strings into JavaScript Date objects. - Use a
while
loop to iterate from the start date to the end date. Within each iteration:- Use
toISOString()
andsplit()
to format the current date into a 'YYYY-MM-DD' format and yield it. - Increment the date by adding the specified number of days (
increment
) using thesetDate()
andgetDate()
methods.
- Use
This generator function allows efficient iteration over a range of dates with the flexibility to specify how many days to skip between yielded dates. Use this function to generate dates that can be iterated in a for-loop or converted into an array using spread syntax.
No comments yet.