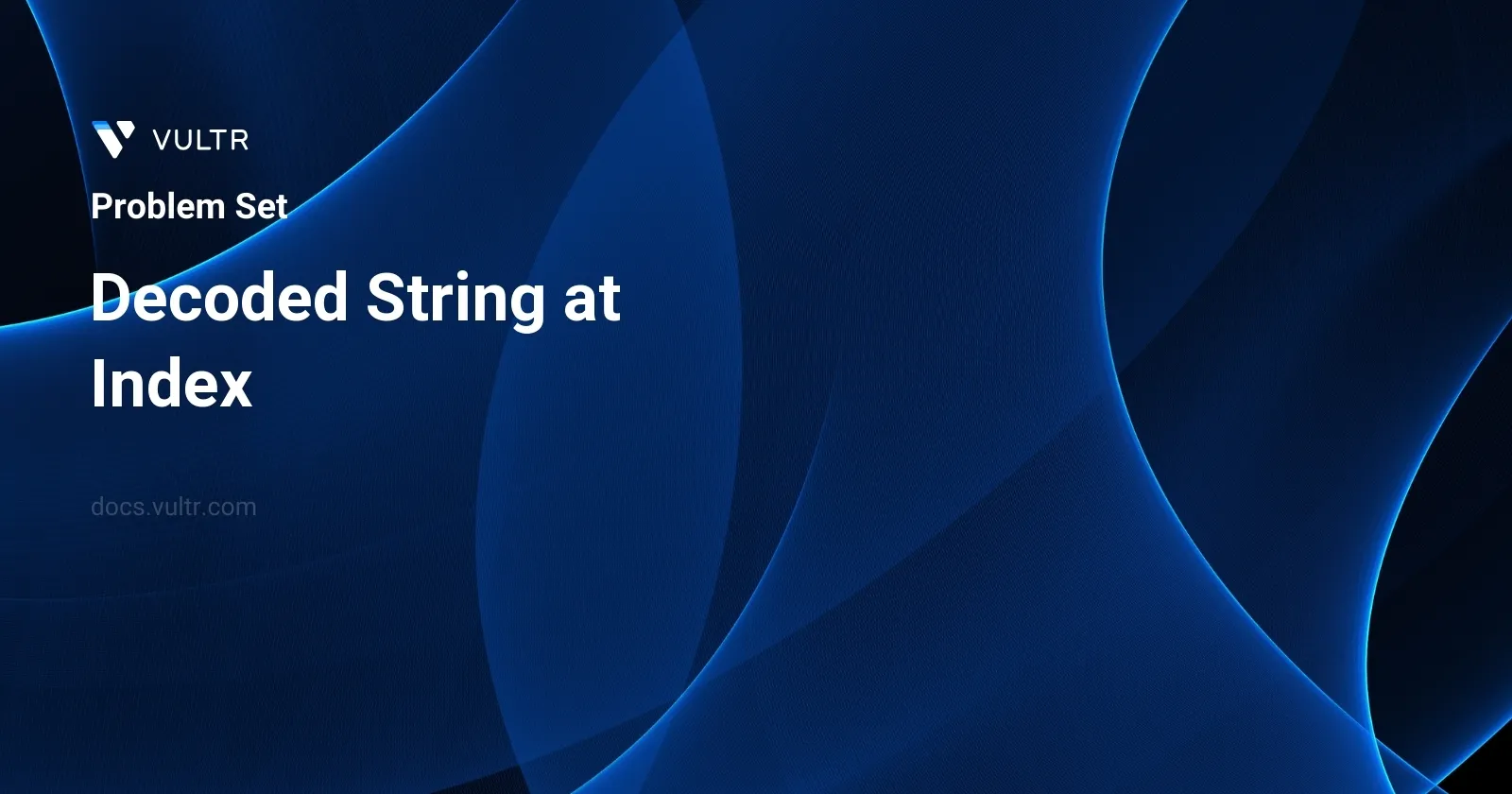
Problem Statement
In this task, you are provided with an encoded string s
. To decode this string, you follow a specific set of operations where each character is processed in sequence:
- If the character is a letter, it is directly written onto an output tape.
- If the character is a digit
d
, it instructs to repeat everything that has been written so far on the taped - 1
additional times.
Given another parameter k
which is a 1-indexed position in the decoded tape, your goal is to determine what letter appears at the kth
position in the fully decoded string.
Examples
Example 1
Input:
s = "Vul2tr3", k = 7
Output:
"o"
Explanation:
The decoded string is "VuVultrVuVultrVuVultr". The 7th letter in the string is "r".
Example 2
Input:
s = "ha22", k = 5
Output:
"h"
Explanation:
The decoded string is "hahahaha". The 5th letter is "h".
Example 3
Input:
s = "a2345678999999999999999", k = 1
Output:
"a"
Explanation:
The decoded string is "a" repeated 8301530446056247680 times. The 1st letter is "a".
Constraints
2 <= s.length <= 100
s
consists of lowercase English letters and digits2
through9
.s
starts with a letter.1 <= k <= 109
- It is guaranteed that
k
is less than or equal to the length of the decoded string. - The decoded string is guaranteed to have less than
263
letters.
Approach and Intuition
The challenge mainly revolves around efficiently determining the kth
character without explicitly constructing the entire decoded string, which can be prohibitively large. Let’s break down the approach with our understanding from the examples:
Recognize that a digit
d
indicates a multiplication of the sequence built so far byd
. Hence, after processing each character (or digit), we know the total length of the constructed sequence at that step.Maintain a running length of the decoded string as we process each character. For letters, this length increases by one. For digits, the current length can potentially multiply, resulting in an exponential growth depending on the digit.
Given that we only need the
kth
character:- If at any point, our running length exceeds or meets
k
, we focus on this segment as it contains our desired character. - If a digit creates a sequence larger than
k
, then backtrack to see which part of the sequence preceding the digit corresponds to thekth
position.
- If at any point, our running length exceeds or meets
Using these observations:
Example 1 ("Vu2ltr3", k = 7):
- After "Vu2", the sequence becomes "VuVu". The length is now longer than earlier but still less than 10.
- Adding "ltr" results in "VuVultr".
- Applying "3" results in "VuVultrVuVultrVuVultr". The 7th character in this sequence is "r".
Example 2 ("ha22", k = 5):
- Applying the two '2's after "ha" causes the sequence "ha" to repeat, becoming "hahahaha". The fifth character in this sequence is "h".
Example 3 ("a2345678999999999999999", k = 1):
- The digits following 'a' expand the sequence exponentially, but since
k=1
, it remains the first character "a".
- The digits following 'a' expand the sequence exponentially, but since
This approach ultimately revolves around using the arithmetic progression to dissect the problem rather than constructing enormous strings, being both time-efficient and memory-friendly. This methodology leverages the understanding of sequential processing and exponential growth induced by the digits.
Solutions
- C++
- Java
- Python
class Solution {
public:
string solve(string inputStr, int position) {
long totalLength = 0;
int length = inputStr.length();
// Calculate total length when the string is fully decoded
for (int index = 0; index < length; ++index) {
if (isdigit(inputStr[index]))
totalLength *= inputStr[index] - '0';
else
totalLength++;
}
for (int index = length - 1; index >= 0; --index) {
position %= totalLength;
if (position == 0 && isalpha(inputStr[index]))
return (string) "" + inputStr[index];
if (isdigit(inputStr[index]))
totalLength /= inputStr[index] - '0';
else
totalLength--;
}
return "";
}
};
The provided C++ code defines a function solve
within a class Solution
that decodes a pseudo-encoded string to find the character at a specific index when the string is fully expanded.
- The function accepts two parameters—a string
inputStr
and an integeri
representing the position in the decoded string to retrieve. - The goal is to determine what character would be at the index
position
in the stringinputStr
after it's fully decoded.
The code operates in two main phases:
Calculate the fully expanded length of the pseudo-encoded string.
- Loop through each character in the
inputStr
. - If the character is a digit, the decoded length is multiplied by the numeric value of the digit.
- If the character is an alphabet, increment the total decoded length by one.
- Loop through each character in the
Identify the character located at
position
without fully expanding the string:- Start iterating from the end of the string.
- Use modulo operation to manage the size of the string's effective length (
totalLength % position
) to find the corresponding character in the compressed format. - If a digit is encountered, decrease the previously calculated total length by dividing it by the numeric value of the digit.
- If the effective position equals zero and the current character is alphabetic, return this character as the result.
The function achieves the objective efficiently by avoiding the actual expansion of the string, using a reverse iteration and modulo operation to account for repeated patterns determined by the digits in the input string. This method is particularly effective for strings with repeats represented by large numbers, ensuring a lower time complexity than if the string were expanded fully.
class Solution {
public String decodeStringAtIndex(String inputStr, int position) {
long totalLength = 0;
int inputLength = inputStr.length();
// Calculate total length of the expanded string
for (int i = 0; i < inputLength; ++i) {
char currentChar = inputStr.charAt(i);
if (Character.isDigit(currentChar))
totalLength *= currentChar - '0';
else
totalLength++;
}
for (int i = inputLength - 1; i >= 0; --i) {
char currentChar = inputStr.charAt(i);
position %= totalLength;
if (position == 0 && Character.isLetter(currentChar))
return Character.toString(currentChar);
if (Character.isDigit(currentChar))
totalLength /= currentChar - '0';
else
totalLength--;
}
throw null; // This should not be reached normally
}
}
The provided Java solution aims to find a specified character from the decoded version of an encoded string without fully expanding it, which would be computationally expensive for large input strings. This method efficiently handles the decoding process by leveraging properties of the encoded pattern and mathematical calculations to avoid full string expansion.
The method, decodeStringAtIndex
, operates in two major parts:
Compute the total length of the fully expanded string:
- Iterate over each character of the input string.
- If the character is a digit, multiply the total length by this number (thus simulating the expansion).
- If it's a letter, increment the total length by one.
Find the character at the specified position from the end to the start:
- Iterate backwards over the string.
- Use modulo operation with the current total length to find the effective position of the character.
- If a letter is found at the adjusted position, return it.
- If a digit is encountered, reduce the total length by dividing it by this digit (this undoes the expansion effect caused by that digit).
This approach ensures that you avoid the enormous memory usage and potential inefficiency of constructing the fully expanded string when it is unnecessary. Remember, an exception or error condition isn't expected to occur with correct input, and the condition to throw null
serves as a fail-safe check in case of unexpected scenarios.
class Solution(object):
def extractCharAtIndex(self, encodedStr, index):
decodedLength = 0
# Compute decoded string length
for char in encodedStr:
if char.isdigit():
decodedLength *= int(char)
else:
decodedLength += 1
for character in reversed(encodedStr):
index %= decodedLength
if index == 0 and character.isalpha():
return character
if character.isdigit():
decodedLength /= int(character)
else:
decodedLength -= 1
This solution is designed to find the character at a given index from an encoded string without decoding the entire string, which increases efficiency, particularly with long strings. The function extractCharAtIndex
in the Python class Solution
accomplishes this through a two-step process:
Calculate the length of the fully decoded string:
- Iterate through each character in the encoded input string. If the character is a digit, multiply the current length by this number to account for the repetition in the encoded pattern. If the character is alphabetical, simply increment the decoded length by one.
Identify the character at the specified index:
- Traverse the string in reverse. During this reverse iteration, adjust the given index using modulo with the current computed length (
index %= decodedLength
). This accounts for repetitive patterns in decoding. - If the reversed character is alphabetical and matches the current position (
index == 0
), return this character as it is the one located at the original index. - If the reversed character is a digit, divide the current decoded length by this number to backtrack through the repetition of segments. If it is alphabetical, just decrement the decoded length reflecting the move back through the string one character at a time.
- Traverse the string in reverse. During this reverse iteration, adjust the given index using modulo with the current computed length (
By using this approach, only the necessary parts of the string are computed, providing a result without unnecessary processing of the entire string. This method is particularly useful when dealing with very large encoded strings and reduces the memory and time complexity significantly.
No comments yet.