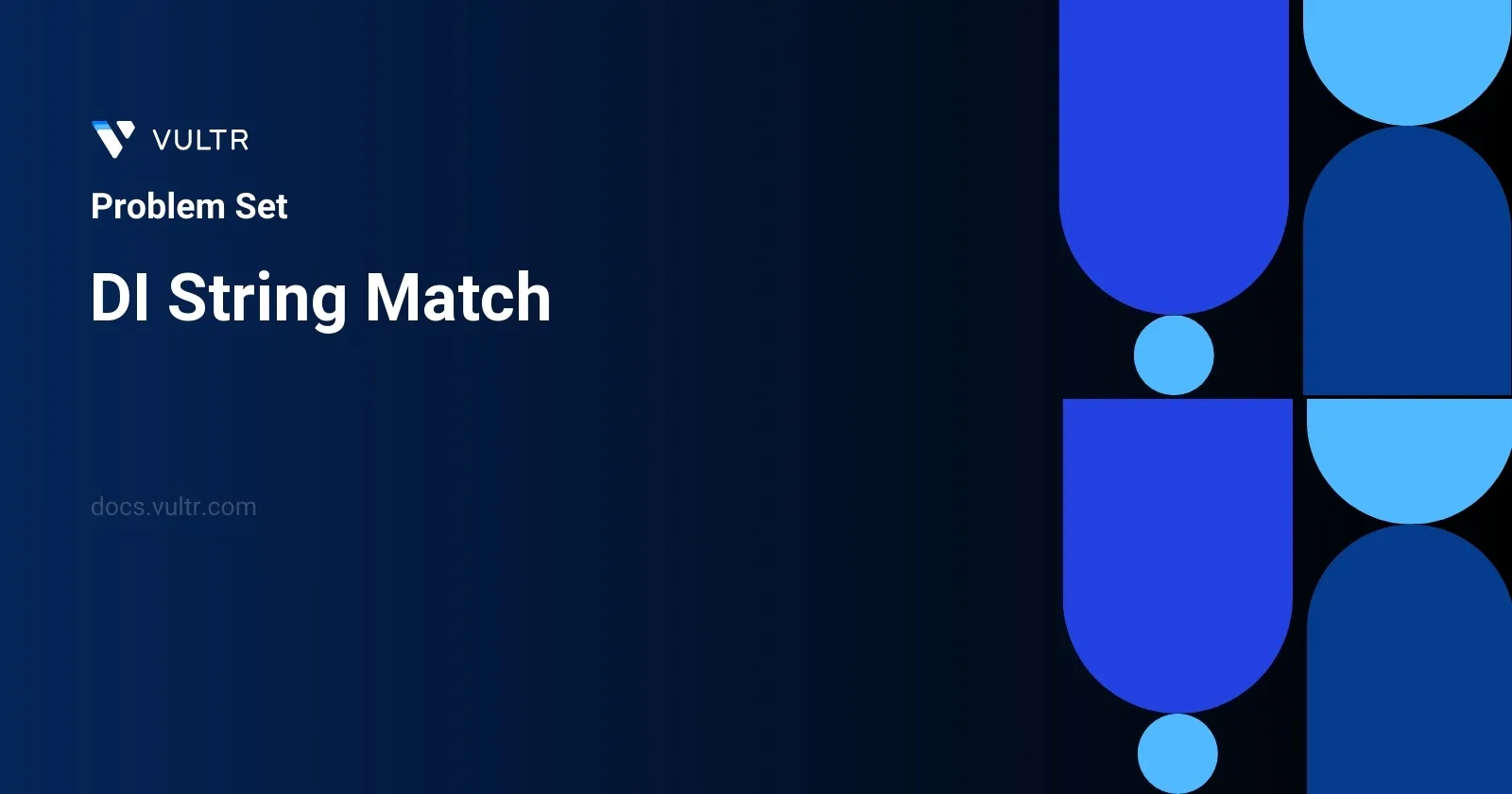
Problem Statement
In this task, we are given a string s
of length n
, which consists of characters 'I' (Increasing) and 'D' (Decreasing). Each character in s
describes the relationship between consecutive elements in a permutation perm
of the integers within the range [0, n]
. Specifically:
s[i] == 'I'
implies thatperm[i] < perm[i + 1]
.s[i] == 'D'
implies thatperm[i] > perm[i + 1]
.
Our goal is to construct any valid permutation perm
that fits the description indicated by the string s
. It's important to note that multiple valid permutations can exist for the same string s
. We simply need to return one of those permutations.
Examples
Example 1
Input:
s = "IDID"
Output:
[0,4,1,3,2]
Example 2
Input:
s = "III"
Output:
[0,1,2,3]
Example 3
Input:
s = "DDI"
Output:
[3,2,0,1]
Constraints
1 <= s.length <= 105
s[i]
is either'I'
or'D'
.
Approach and Intuition
The approach to generating a valid permutation from the string s
uses a straightforward greedy technique, utilizing two pointers to represent the smallest and largest numbers available to construct perm
.
- Initialize the permutation array
perm
of sizen+1
to store the result. - Use two pointers,
low
andhigh
. Setlow
to 0 andhigh
ton
. - Traverse through the string
s
:- If
s[i] == 'I'
, setperm[i]
tolow
and incrementlow
. This uses the smallest available number and ensures the next number is higher. - If
s[i] == 'D'
, setperm[i]
tohigh
and decrementhigh
. This uses the largest available number, guaranteeing the next number is smaller.
- If
- After processing all characters in
s
, setperm[n]
to the remaining value oflow
(which now equalshigh
). - This method efficiently constructs the permutation by picking the extreme available values based on the immediate requirement (either smallest or largest), ensuring the conditions
perm[i] < perm[i + 1]
orperm[i] > perm[i + 1]
are satisfied.
By this approach, the permutation is built in a linear pass based on the relationship flags provided in s
, efficiently and correctly forming a permutation that meets the criteria.
Solutions
- C++
- Java
- Python
class Solution {
public:
vector<int> diStringMatch(string pattern) {
int length = pattern.size();
int minVal = 0, maxVal = length;
vector<int> result(length + 1);
for (int i = 0; i < length; ++i) {
if (pattern[i] == 'I') {
result[i] = minVal++;
} else {
result[i] = maxVal--;
}
}
result[length] = minVal;
return result;
}
};
The provided C++ solution solves the problem of generating a sequence of integers based on a given pattern of 'I' (increasing) and 'D' (decreasing) characters. Here's a breakdown of how the solution works:
- A string
pattern
, consisting of characters 'I' and 'D', defines the order. - Two variables,
minVal
andmaxVal
, are initialized to represent the minimum and maximum possible values in the resulting sequence, set initially to0
and the length ofpattern
respectively. - A
result
vector is created of size equal topattern.length() + 1
to store the resultant sequence. - Loop through each character in
pattern
:- If the character is 'I', assign
minVal
to the current index inresult
and incrementminVal
. - If the character is 'D', assign
maxVal
to the current index inresult
and decrementmaxVal
.
- If the character is 'I', assign
- After the loop, assign
minVal
to the last element inresult
to handle the last index due to 'I' or the smallest remaining number. - Return the
result
vector which contains the desired sequence of integers.
This solution ensures that the sequence correctly follows the 'increase' or 'decrease' order dictated by the pattern string
, leveraging the properties of integers and looping control flow to construct the result efficiently.
class Solution {
public int[] createPermutation(String pattern) {
int size = pattern.length();
int min = 0, max = size;
int[] result = new int[size + 1];
for (int i = 0; i < size; i++) {
if (pattern.charAt(i) == 'I') result[i] = min++;
else result[i] = max--;
}
result[size] = min;
return result;
}
}
The given Java solution addresses the problem of generating an array that matches a specific "DI string" pattern. The method createPermutation
takes a string pattern
consisting of the characters 'D' and 'I'. Based on this pattern, the method generates an array of integers where 'I' indicates that the sequence must increase and 'D' indicates a decrease. Here's a breakdown of how the solution implements this:
- Initialize
size
to the length of the pattern to determine the output array’s size, which will besize + 1
. - Set
min
to 0 andmax
tosize
to represent the smallest and largest numbers to be used in the array. - Create an integer array
result
of lengthsize + 1
. - Loop through each character of the pattern:
- If the character is 'I', assign
result[i]
tomin
and then incrementmin
. - If the character is 'D', assign
result[i]
tomax
and then decrementmax
.
- If the character is 'I', assign
- After exiting the loop, set the last element of
result
tomin
to ensure all numbers from 0 tosize
are utilized.
This implementation efficiently maps the increase/decrease pattern to a concrete number sequence, effectively solving the "DI String Match" problem within the constraints assured by using single-pass logic and direct assignments.
class Solution:
def orderString(self, input_str: str) -> List[int]:
low, high = 0, len(input_str)
result = []
for char in input_str:
if char == "I":
result.append(low)
low += 1
else:
result.append(high)
high -= 1
return result + [low]
This Python solution is designed to solve the DI String Match problem by mapping the input string comprised of 'I' and 'D' characters to an array of integers that satisfy certain conditions. The approach utilizes two pointers, low
and high
, which represent the smallest and largest values yet to be assigned, initialized as 0 and the length of the input string, respectively. As you iterate over the input string:
- Append
low
to the result list and incrementlow
by 1 for every 'I'. - Append
high
to the result list and decrementhigh
by 1 for every 'D'.
After processing all characters, append the current value of low
to the result list to account for the final value. This method ensures no missed or duplicated elements, producing a sequence where each 'I' represents an increment and each 'D' represents a decrement in relation to the previous element. The solution effectively translates the pattern in the input string into a sequence of integers meeting the described criteria.
No comments yet.