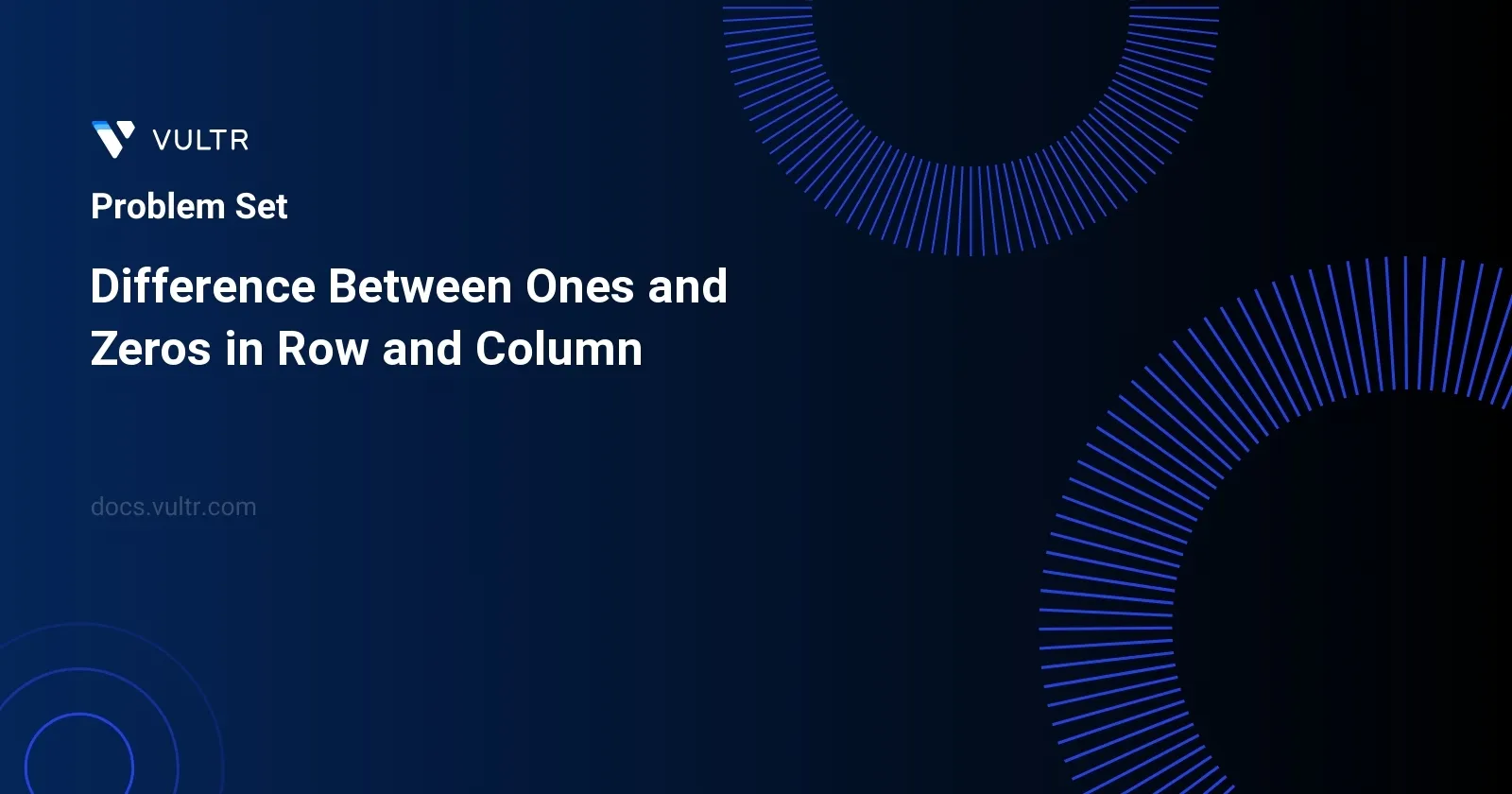
Problem Statement
In this task, you are provided with a m x n
binary matrix named grid
where the indexing starts from 0
. Using this matrix, you need to construct another matrix called diff
of the same dimensions according to a specific set of rules. These rules are directly related to the count of ones (1
s) and zeros (0
s) in each row and column of the original matrix grid
.
For each element at position (i, j)
in the matrix diff
, the value is computed based on the following formula:
diff[i][j] = onesRowi + onesColj - zerosRowi - zerosColj
where:
onesRowi
denotes the count of ones in theith
row of the matrixgrid
.onesColj
denotes the count of ones in thejth
column of the matrixgrid
.zerosRowi
denotes the count of zeros in theith
row of the matrixgrid
.zerosColj
denotes the count of zeros in thejth
column of the matrixgrid
.
The challenge is to correctly compute and return this diff
matrix based on the provided grid
.
Examples
Example 1
Input:
grid = [[0,1,1],[1,0,1],[0,0,1]]
Output:
[[0,0,4],[0,0,4],[-2,-2,2]]
Explanation:
- diff[0][0] = `onesRow0 + onesCol0 - zerosRow0 - zerosCol0` = 2 + 1 - 1 - 2 = 0 - diff[0][1] = `onesRow0 + onesCol1 - zerosRow0 - zerosCol1` = 2 + 1 - 1 - 2 = 0 - diff[0][2] = `onesRow0 + onesCol2 - zerosRow0 - zerosCol2` = 2 + 3 - 1 - 0 = 4 - diff[1][0] = `onesRow1 + onesCol0 - zerosRow1 - zerosCol0` = 2 + 1 - 1 - 2 = 0 - diff[1][1] = `onesRow1 + onesCol1 - zerosRow1 - zerosCol1` = 2 + 1 - 1 - 2 = 0 - diff[1][2] = `onesRow1 + onesCol2 - zerosRow1 - zerosCol2` = 2 + 3 - 1 - 0 = 4 - diff[2][0] = `onesRow2 + onesCol0 - zerosRow2 - zerosCol0` = 1 + 1 - 2 - 2 = -2 - diff[2][1] = `onesRow2 + onesCol1 - zerosRow2 - zerosCol1` = 1 + 1 - 2 - 2 = -2 - diff[2][2] = `onesRow2 + onesCol2 - zerosRow2 - zerosCol2` = 1 + 3 - 2 - 0 = 2
Example 2
Input:
grid = [[1,1,1],[1,1,1]]
Output:
[[5,5,5],[5,5,5]]
Explanation:
- diff[0][0] = onesRow0 + onesCol0 - zerosRow0 - zerosCol0 = 3 + 2 - 0 - 0 = 5 - diff[0][1] = onesRow0 + onesCol1 - zerosRow0 - zerosCol1 = 3 + 2 - 0 - 0 = 5 - diff[0][2] = onesRow0 + onesCol2 - zerosRow0 - zerosCol2 = 3 + 2 - 0 - 0 = 5 - diff[1][0] = onesRow1 + onesCol0 - zerosRow1 - zerosCol0 = 3 + 2 - 0 - 0 = 5 - diff[1][1] = onesRow1 + onesCol1 - zerosRow1 - zerosCol1 = 3 + 2 - 0 - 0 = 5 - diff[1][2] = onesRow1 + onesCol2 - zerosRow1 - zerosCol2 = 3 + 2 - 0 - 0 = 5
Constraints
m == grid.length
n == grid[i].length
1 <= m, n <= 105
1 <= m * n <= 105
grid[i][j]
is either0
or1
.
Approach and Intuition
To solve this problem, follow these steps:
Initialize Count Arrays:
- Create two arrays,
rows
andcols
, each initialized to 0.rows[i]
will store the count of1
s in thei-th
row, andcols[j]
will store the count of1
s in thej-th
column.
- Create two arrays,
Calculate Ones Count:
- Traverse every cell in the input matrix
grid
. For each1
encountered at position(i, j)
, incrementrows[i]
andcols[j]
by1
. This will ultimately give you the total count of ones in each row and column.
- Traverse every cell in the input matrix
Compute Difference Matrix:
- Initialize the result matrix
diff
of the same size asgrid
where each value is initially set to 0. - Use a nested loop to go through each cell
(i, j)
and calculate the value ofdiff[i][j]
using the formula:diff[i][j] = onesRowi + onesColj - zerosRowi - zerosColj
, where:onesRowi = rows[i]
onesColj = cols[j]
zerosRowi = n - rows[i]
(Since total elements in a row minus ones gives zeros)zerosColj = m - cols[j]
(Since total elements in a column minus ones gives zeros).
- This computed matrix will be the desired
diff
matrix reflecting the differences as specified.
- Initialize the result matrix
Solutions
- C++
- Java
class Solution {
public:
vector<vector<int>> computeDiff(vector<vector<int>>& matrix) {
int rows = matrix.size();
int cols = matrix[0].size();
vector<int> rowSum(rows, 0);
vector<int> colSum(cols, 0);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
rowSum[i] += matrix[i][j];
colSum[j] += matrix[i][j];
}
}
vector<vector<int>> result(rows, vector<int>(cols, 0));
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result[i][j] = 2 * rowSum[i] + 2 * colSum[j] - cols - rows;
}
}
return result;
}
};
This solution involves calculating the difference between ones and zeros in each row and column of a given binary matrix and represents the computation using a C++ class named Solution
. The key method computeDiff
receives a binary matrix and computes the required values.
- Start by acquiring the dimensions of the matrix - rows and columns.
- Initialize two vectors,
rowSum
andcolSum
, to keep track of the sum of elements in each row and column respectively. - Iterate over each element of the matrix:
- Update
rowSum
for the current row andcolSum
for the current column based on the matrix values.
- Update
- Create a result matrix of the same size as the input matrix with initial values set to zero.
- Calculate the final values for each element in the result matrix:
- Use the formula
2 * rowSum[i] + 2 * colSum[j] - cols - rows
to compute the value for each cell, which effectively assesses the difference between the number of ones and zeros when considering both the entire row and column of the cell.
- Use the formula
- Return the result matrix as the output of the method.
This process successfully produces a new matrix where each entry reflects the computed difference as specified, making use of straightforward matrix manipulation and arithmetic operations.
class Solution {
public int[][] computeResult(int[][] matrix) {
int rows = matrix.length;
int cols = matrix[0].length;
int[] rowCount = new int[rows];
int[] colCount = new int[cols];
Arrays.fill(rowCount, 0);
Arrays.fill(colCount, 0);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
rowCount[i] += matrix[i][j];
colCount[j] += matrix[i][j];
}
}
int[][] result = new int[rows][cols];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result[i][j] = 2 * rowCount[i] + 2 * colCount[j] - cols - rows;
}
}
return result;
}
}
The provided solution in Java, titled "Difference Between Ones and Zeros in Row and Column," involves computing a matrix that represents the difference count of ones and zeros across the rows and columns of a given binary matrix. The algorithm is structured in a class named Solution
containing a method computeResult
which takes a two-dimensional integer array (matrix
) as input and returns another two-dimensional integer array.
Here's how the solution is structured:
Initialize row and column counters: First, arrays
rowCount
andcolCount
are initialized to keep track of the sum of ones in each row and column respectively. These arrays are initially filled with zeros.Compute row and column sums: Through nested loops, iterate over each element in the input matrix. Increment the corresponding row and column counters by the value of the matrix at the position
[i][j]
, effectively counting the ones.Calculate the result matrix: Another nested loop constructs the result matrix. Each element of the result is calculated using the formula
2 * rowCount[i] + 2 * colCount[j] - cols - rows
. This probably aims to represent a custom metric derived from the counts of ones influenced by the matrix's dimensions.Return the result: Once the entire result matrix is computed, it is returned.
Key considerations include:
- Understandability: The basis of the computation formula (2 * rowCount[i] + 2 * colCount[j] - cols - rows) may require further documentation or explanation based on the problem specific context or intent of the results being used for.
- Efficiency: The solution operates with a time complexity of O(n*m), where n is the number of rows and m the number of columns, which is efficient given the problem constraints.
- Applicability: The method expects a non-empty matrix and does not handle potential anomalies like different column lengths or non-numeric inputs. Handling such cases can be a point for further enhancement.
No comments yet.