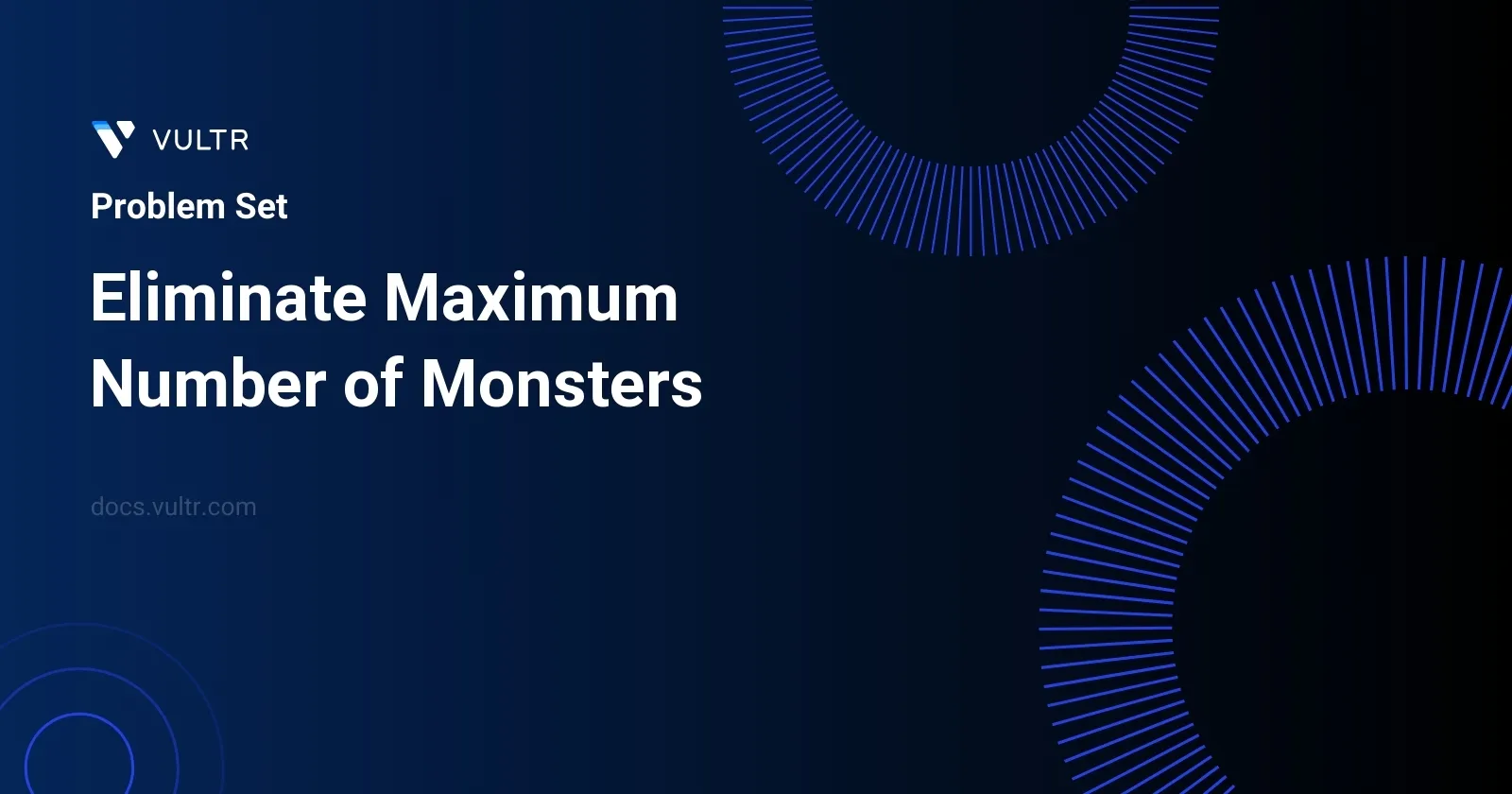
Problem Statement
In a video game scenario, the task involves defending a city against a series of monsters approaching at different speeds and from different distances. The game provides the number of monsters (n
) as well as two primary integer arrays: dist
and speed
. The dist
array represents the initial distances of the monsters from the city, with dist[i]
being the distance for the ith monster. Similarly, speed[i]
in the speed
array denotes how fast the ith monster approaches the city in kilometers per minute.
A vital detail in this challenge is the weapon used to eliminate the monsters. It can destroy a single monster per minute, and you start the game with the weapon charged up. The primary risk and challenge arise because the game ends the moment any monster reaches the city, including the exact time when your weapon is charged.
Your objective is to strategize your weapon's usage to maximize the number of monsters you can eliminate before any of them reach the city, ensuring you don't lose before destroying as many as possible.
Examples
Example 1
Input:
dist = [1,3,4], speed = [1,1,1]
Output:
3
Explanation:
In the beginning, the distances of the monsters are [1,3,4]. You eliminate the first monster. After a minute, the distances of the monsters are [X,2,3]. You eliminate the second monster. After a minute, the distances of the monsters are [X,X,2]. You eliminate the third monster. All 3 monsters can be eliminated.
Example 2
Input:
dist = [1,1,2,3], speed = [1,1,1,1]
Output:
1
Explanation:
In the beginning, the distances of the monsters are [1,1,2,3]. You eliminate the first monster. After a minute, the distances of the monsters are [X,0,1,2], so you lose. You can only eliminate 1 monster.
Example 3
Input:
dist = [3,2,4], speed = [5,3,2]
Output:
1
Explanation:
In the beginning, the distances of the monsters are [3,2,4]. You eliminate the first monster. After a minute, the distances of the monsters are [X,0,2], so you lose. You can only eliminate 1 monster.
Constraints
n == dist.length == speed.length
1 <= n <= 105
1 <= dist[i], speed[i] <= 105
Approach and Intuition
Firstly, you must understand that the crucial factor in this problem is the time it takes for each monster to reach the city. This can be computed by dividing the distance of the monster from the city
dist[i]
by its speedspeed[i]
.To ensure optimal use of your weapon, you need to prioritize eliminating monsters based on how quickly they would reach the city if left unchecked. This means sorting the monsters by their time to reach the city in ascending order.
You start the game with the weapon ready, so you can immediately take out the monster that poses the most immediate threat (i.e., the one that will reach the city soonest).
For each subsequent minute, re-calculate the distance of the monsters from the city given the time that has elapsed since the game started. Eliminate the next most immediate threat.
This strategy continues until either all monsters are eliminated or one reaches the city. Be aware that if a monster reaches the city 'exactly' when your weapon charges, it counts as a loss.
Key tactical takeaways include:
- Always prioritize the monsters that are closest in terms of arrival time.
- Keep track of the elapsed time and the adjusted distances accordingly.
- Test the approach thoroughly with edge cases, considering very fast monsters close to the city and slower ones that are further away.
By following this systematic approach towards calculating threat levels and handling them sequentially, you maximize your defense strategy's effectiveness in the game.
Solutions
- C++
- Java
- Python
class Solution {
public:
int maxEliminations(vector<int>& distances, vector<int>& velocities) {
priority_queue<float, vector<float>, greater<float>> events;
for (int index = 0; index < distances.size(); index++) {
events.push((float) distances[index] / velocities[index]);
}
int count = 0;
while (!events.empty()) {
if (events.top() <= count) {
break;
}
count++;
events.pop();
}
return count;
}
};
In the C++ implementation for solving the problem of eliminating the maximum number of monsters, the solution leverages sorting of arrival times to the city using a priority queue. Each monster has a distance from the city and travels at a fixed velocity. Calculate the time it takes each monster to reach the city by dividing its distance by its velocity, and store these times in a min-heap priority queue.
Follow the steps below to understand the approach:
- Populate the priority queue with the time it takes for each monster to reach the city using the formula ( \text{time} = \frac{\text{distance}}{\text{velocity}} ). This ensures that monsters closer to the city are prioritized.
- Initialize a counter to keep track of how many monsters can be eliminated.
- Continuously remove monsters from the queue starting with the one that arrives first.
- If the arrival time of the monster at the top of the queue is less than or equal to the current time step (initially represented by the counter), stop the process as it indicates simultaneous or earlier arrival of the next monster than you can handle at that point in time.
- Increment the counter each time a monster is eliminated, representing each time step taken to deal with one monster.
This approach ensures that you effectively eliminate as many monsters as possible by prioritizing those who arrive at the city first. The number returned at the end of the loop, represented by the counter, is the maximum number of monsters that can be eliminated before any monster reaches the city.
class Solution {
public int removeMaxMonsters(int[] distance, int[] velocity) {
PriorityQueue<Double> pq = new PriorityQueue<>();
for (int i = 0; i < distance.length; i++) {
pq.offer((double) distance[i] / velocity[i]);
}
int count = 0;
while (!pq.isEmpty()) {
if (pq.poll() <= count) {
break;
}
count++;
}
return count;
}
}
This Java program tackles the problem of determining the maximum number of monsters one can eliminate before they reach the player. Monsters' approach speeds are given in terms of distances and velocities through two input arrays.
- Start with invoking a PriorityQueue to sort the monsters based on the time they will take to reach the player. This time is calculated by dividing each monster's distance by its velocity.
- Iterate over the monster distance and velocity arrays to populate the priority queue with the calculated times.
- Initialize a counter to keep track of the number of monsters you can safely remove.
- Use a while loop to process each monster in the queue:
- Continuously remove monsters from the queue (starting with the monster that takes the least time to reach the player) and check if the current time (represented by the incremented count) exceeds the monster's time to reach. If it does, terminate the loop.
- Otherwise, increment the count of monsters eliminated.
The method finally returns the count of monsters that were removed before any could reach the player. This solution is efficient due to its use of a priority queue that automatically organizes the monsters based on the urgency of their threat, which is determined by the time it will take for them to reach the player.
import heapq
class Solver:
def removeMaxMinions(self, distances: List[int], velocities: List[int]) -> int:
priority_queue = []
for index in range(len(distances)):
priority_queue.append(distances[index] / velocities[index])
heapq.heapify(priority_queue)
total_monsters = 0
while priority_queue:
if heapq.heappop(priority_queue) <= total_monsters:
break
total_monsters += 1
return total_monsters
In this Python solution, you need to calculate how many monsters can be removed before any monster can reach you. The logic uses the concept of a minimum heap (priority queue) to manage the processing times of the monsters based on their distance and velocity.
Here’s the breakdown:
- Initialize a list to function as a priority queue.
- Calculate time each monster will take to reach you by dividing their distance by their velocity and add that time to the priority queue.
- Convert the list into a heap structure to take advantage of efficient minimum value retrieval.
- Continuously pop the minimum time from the heap (representing the monster that will reach you the soonest) and compare it against the total number of monsters removed so far.
- If the time it takes for a monster to reach you is less than or equal to the number of monsters already removed, stop the process.
- Otherwise, increment the count of monsters removed and continue checking.
This solution efficiently determines how many monsters you can eliminate before any reach your position. The use of a priority queue ensures that you always deal with the monster approaching soonest, optimizing your strategy for maximum elimination.
No comments yet.