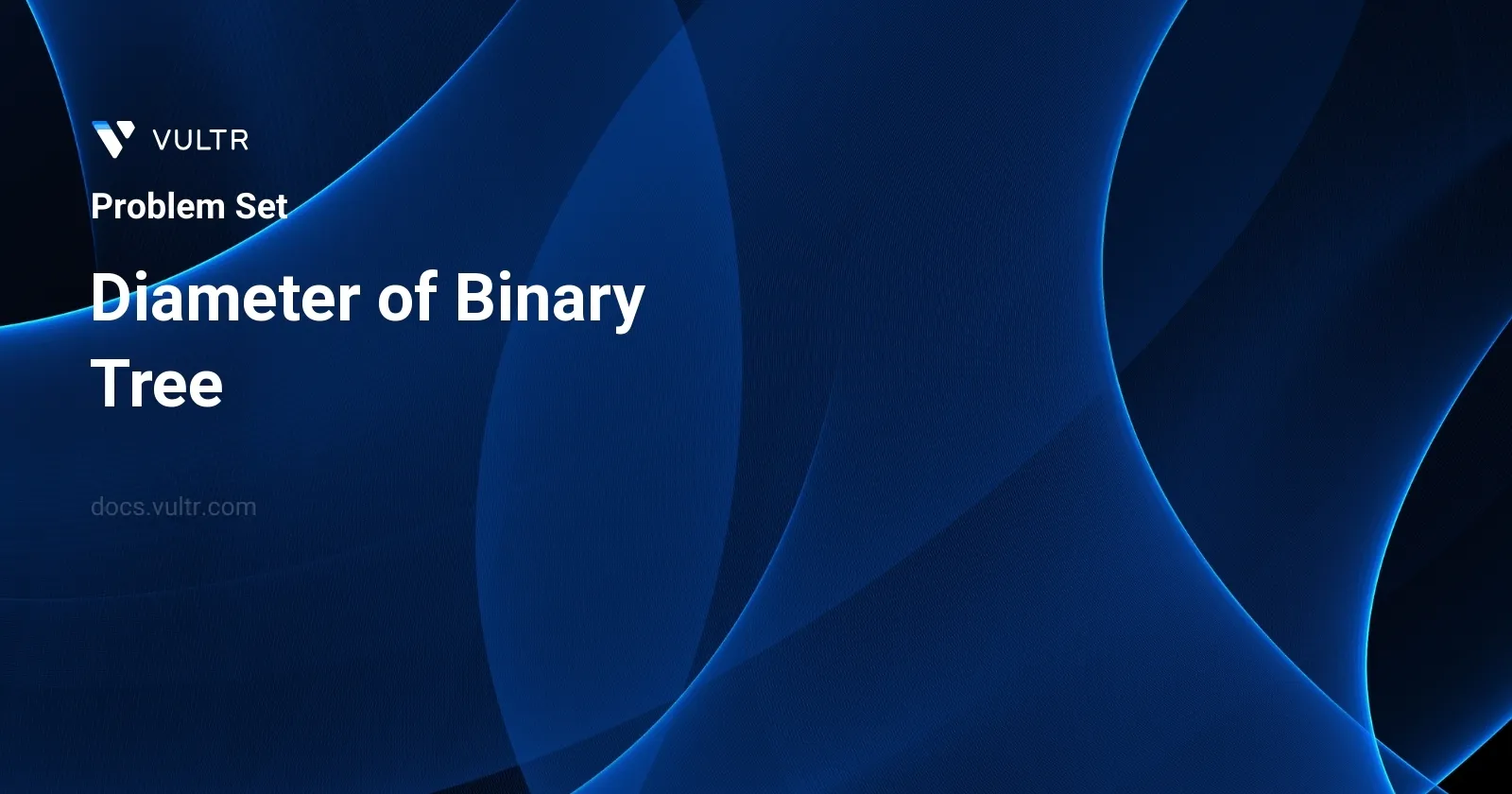
Problem Statement
The problem is straightforward if you understand the structure and properties of a binary tree. You are provided with the root node of a binary tree and must compute the diameter of this tree. The diameter is defined as the length of the longest path between any two nodes within the tree. This path can either pass through the root or not. Critically, what truly marks the diameter is the count of edges in the longest possible path that can be found from one node to another across the tree. This measurement does not take node values into account but purely the structure and connections within the tree.
Examples
Example 1
Input:
root = [1,2,3,4,5]
Output:
3
Explanation:
3 is the length of the path [4,2,1,3] or [5,2,1,3].
Example 2
Input:
root = [1,2]
Output:
1
Constraints
- The number of nodes in the tree is in the range
[1, 104]
. -100 <= Node.val <= 100
Approach and Intuition
To solve the problem, we need to focus on understanding the measurements and the properties of tree traversal:
Maximum Depth Calculation:
- Each time we visit a node, we'll consider the length of the paths to its left and right child nodes.
- The idea revolves around calculating the depth from each node downwards and simultaneously keeping track of the maximum diameter encountered.
Recurrence and Helper Functions:
- Typically, a helper function calculates the depth from the node downward — summing edges to get to the furthest leaf node.
- For each node visited, the potential diameter considering that node as the highest ancestor is the sum of the depths of the left and right subtrees.
Update Global Diameter:
- As we calculate the depth for each node, update a global variable that keeps track of the maximum diameter found so far.
- This diameter will be the sum of the maximum depths reached in the left and right subtrees rooted at the current ancestor node.
Result from Recursion:
- A recursive approach works well here, as we need to traverse the tree and at every node, simultaneously calculate the depth for subtree and potentially update the diameter.
Edge Case:
- When the tree is very small (1 or 2 nodes), handle these cases straightforwardly by direct observation — if there's only one node, the diameter is
0
, and for two nodes, it is1
.
- When the tree is very small (1 or 2 nodes), handle these cases straightforwardly by direct observation — if there's only one node, the diameter is
By applying the above approach, we efficiently track and determine the longest path between nodes in the tree, effectively calculating the diameter. Such problems highlight the power of depth-first search (DFS) in dealing with tree-based data structures, leveraging recursion to simplify depth and path calculations.
Solutions
- Java
- Python
class Solution {
private int maxDiameter;
public int diameterOfBinaryTree(TreeNode root) {
maxDiameter = 0;
calculateMaxPath(root);
return maxDiameter;
}
private int calculateMaxPath(TreeNode node){
if(node == null) return 0;
int leftMax = calculateMaxPath(node.left);
int rightMax = calculateMaxPath(node.right);
maxDiameter = Math.max(maxDiameter, leftMax + rightMax);
return Math.max(leftMax, rightMax) + 1;
}
}
The provided solution in Java is designed to compute the diameter of a binary tree, where the diameter is defined as the longest path between any two nodes in the tree. This path does not necessarily pass through the root. It employs a recursive approach with a helper function to efficiently determine the maximum path from any node.
Here's an explanation of how the code functions:
- The
Solution
class contains a private variablemaxDiameter
which tracks the maximum length found during recursion. - The method
diameterOfBinaryTree(TreeNode root)
initializesmaxDiameter
and starts the recursive helper methodcalculateMaxPath
where the actual calculation is performed. - The
calculateMaxPath
method computes recursively for each node:- Base case: If the node is null, returns 0.
- Recursively finds the maximum path length for the left child
leftMax
and right childrightMax
. - Updates the
maxDiameter
by comparing it to the sum ofleftMax
andrightMax
. This step checks if connecting the two children through the current node results in a longer path than previously recorded. - Returns the height of the current subtree to its parent call, determined by the maximum height of its children plus one.
This approach optimizes the search by calculating diameter and subtree height in one pass, avoiding redundant traversals and ensuring efficiency. The method ultimately returns the value of maxDiameter
after completing the recursive processing, which is the longest diameter of the binary tree.
class Solution:
def treeDiameter(self, root: TreeNode) -> int:
max_diameter = 0
def max_path(node):
if not node:
return 0
nonlocal max_diameter
left_length = max_path(node.left)
right_length = max_path(node.right)
max_diameter = max(max_diameter, left_length + right_length)
return max(left_length, right_length) + 1
max_path(root)
return max_diameter
This Python 3 solution aims to calculate the diameter of a binary tree. The diameter is defined as the longest path between any two nodes in the tree. This is calculated using a depth-first search (DFS) approach encapsulated within an inner function max_path
.
- The solution class,
Solution
, has a methodtreeDiameter
which takesroot
of typeTreeNode
as its argument. - Inside the method, a local variable
max_diameter
is initialized to keep track of the maximum diameter found during traversal. - The
max_path
inner function recursively computes the maximum path length from each node. It:- Returns 0 if the node is None, indicating the base case of recursion.
- Uses a nonlocal declaration to modify
max_diameter
defined in the enclosing scope. - Computes the length of the paths from the current node to the left and right children.
- Updates
max_diameter
to be the maximum value between the currentmax_diameter
and the sum of left and right path lengths. - Returns the maximum path length from the node downward, adding one for the current node.
- Finally, the
max_path
function is called with the root node, and the maximum diameter accumulated during the traversal is returned.
This method effectively handles each node a single time, ensuring the function runs efficiently with time complexity loosely coupled with the number of nodes in the tree, thus providing a robust solution to determine the tree's diameter.
No comments yet.