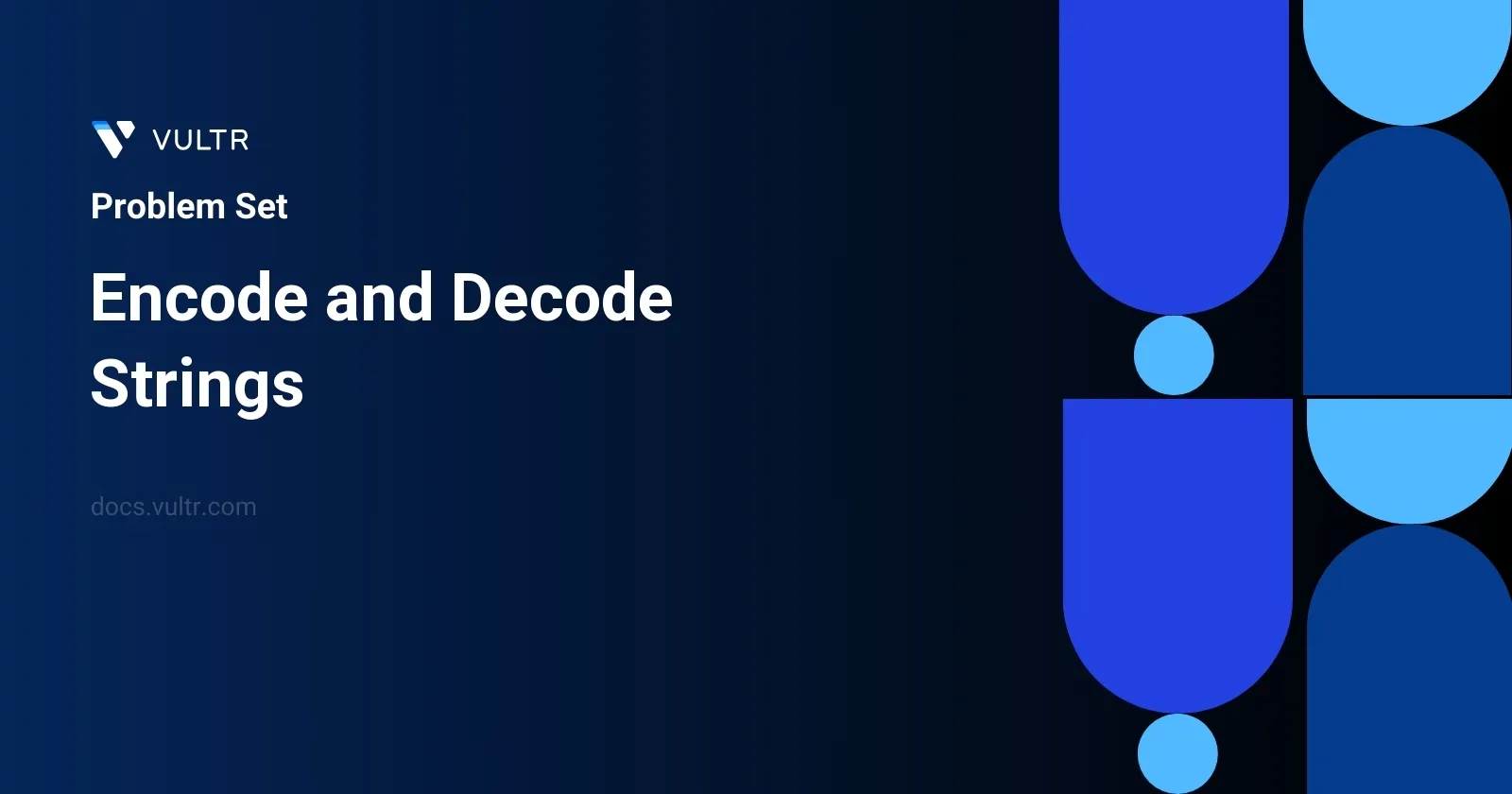
Problem Statement
The challenge involves creating a robust algorithm for encoding a list of strings into a single string format, which can then be transmitted over a network and subsequently decoded back into the original list of strings by another machine. This procedure is to be implemented through two functions:
encode(vector<string> strs)
which is used by Machine 1 (sender) to transform the list of strings into an encoded string.decode(string s)
which allows Machine 2 (receiver) to interpret the encoded string back into the list of strings as originally sent.
The encoded format needs to be such that it can unambiguously differentiate between different strings in the list, even if they are complex or empty strings. The procedure should ensure that after decoding, Machine 2’s output list of strings (strs2
) must be identical to the input list of strings (strs
) used by Machine 1. The solution must avoid using any serialization methods like eval
to meet the challenge requirements.
Examples
Example 1
Input:
dummy_input = ["Hello","World"]
Output:
["Hello","World"]
Explanation:
Machine 1: Codec encoder = new Codec(); String msg = encoder.encode(strs); Machine 1 ---msg---> Machine 2 Machine 2: Codec decoder = new Codec(); String[] strs = decoder.decode(msg);
Example 2
Input:
dummy_input = [""]
Output:
[""]
Constraints
1 <= strs.length <= 200
0 <= strs[i].length <= 200
strs[i]
contains any possible characters out of256
valid ASCII characters.
Approach and Intuition
Given the constraints and requirements, our solution needs to handle variable-length strings as well as a large range of potential character sets. Here's a step-by-step approach to tackle this:
Encoding Approach:
- To uniquely identify where each string starts and ends in the encoded form, use a delimiter based approach that includes the length of each string.
- For each string
s
in the liststrs
, encode it by prefixing with its length followed by a special character (for example, a hash#
), and then the string itself. This will help in clearly demarking where the string begins and ends. - An example encoding might look like
5#Hello5#World
for the input["Hello", "World"]
.
Decoding Approach:
- Parse the encoded string from beginning to end.
- Read until you find a special delimiter (e.g.,
#
), which indicates that the preceding number is the length of the string. - Knowing the length, you can then accurately capture the string of that length that follows.
- Move the parsing cursor forward by the length of the string plus any additional characters used for encoding (like the length and delimiter), and then repeat the process until the entire encoded string is parsed.
This approach carefully handles the constraints:
- The delimiter and length prefix ensure that even strings that contain unusual or special characters (out of the 256 ASCII characters possible) or are empty are accurately encoded and decoded.
- Using the length of the string as part of the encoding ensures that the parsing during the decoding process is straightforward and unambiguous.
Solutions
- C++
- Java
- Python
class Codec {
public:
string serialize(vector<string>& inputStrings) {
string result;
for (string &element : inputStrings) {
result += to_string(element.size()) + "/:" + element;
}
return result;
}
vector<string> deserialize(string data) {
vector<string> output;
size_t startPos = 0;
while (startPos < data.size()) {
size_t delimiter = data.find("/:", startPos);
int len = stoi(data.substr(startPos, delimiter - startPos));
string extracted = data.substr(delimiter + 2, len);
output.push_back(extracted);
startPos = delimiter + 2 + len;
}
return output;
}
};
In this guide, you will explore how to encode and decode strings using C++ programming. The provided solution is implemented within a Codec
class comprising two main functions: serialize
and deserialize
.
Focus first on the
serialize
function:- Begin by initializing an empty string
result
. - Iterate through every string in the input vector
inputStrings
. - For each string, append its length, followed by the delimiter "/:" and the string itself, to
result
. - Return the concatenated
result
, which now holds the encoded form of all input strings.
- Begin by initializing an empty string
Next, examine the
deserialize
function:- Start by initializing an empty vector of strings
output
. - Use a
startPos
to track the current position in the encoded stringdata
. - Use a while loop to process
data
untilstartPos
reaches the end of the string. - Locate the delimiter "/:" from
startPos
to find where the current string's length definition ends. - Extract the length of the string and use it to get the actual string value from
data
. - Place the extracted string into the
output
vector and adjuststartPos
for the next iteration. - Once the loop completes, return
output
, which contains all the decoded strings.
- Start by initializing an empty vector of strings
This solution effectively handles the encoding and decoding of an array of strings through the use of length and delimiter, ensuring string boundaries are maintained irrespective of content. The implementation is both straightforward and efficient, utilizing standard string and vector operations in C++.
public class Codec {
public String serialize(List<String> inputList) {
StringBuilder result = new StringBuilder();
for (String element : inputList) {
result.append(element.length()).append("/:").append(element);
}
return result.toString();
}
public List<String> deserialize(String encoded) {
List<String> resultArray = new ArrayList<>();
int position = 0;
while (position < encoded.length()) {
int marker = encoded.indexOf("/:", position);
int size = Integer.parseInt(encoded.substring(position, marker));
String segment = encoded.substring(marker + 2, marker + 2 + size);
resultArray.add(segment);
position = marker + 2 + size;
}
return resultArray;
}
}
The solution presented in Java provides mechanisms to both encode and decode lists of strings efficiently using a custom serialization format. Follow the techniques below to understand how each part functions:
Encoding Process:
- Instantiate a
StringBuilder
to store the serialized output. - Loop through each string in the input list.
- For each string, append its length and a special separator
"/:"
to theStringBuilder
. Follow this by appending the string itself. - Convert the
StringBuilder
to a string before returning it, which represents the encoded form of the input list.
- Instantiate a
Decoding Process:
- Create an empty
ArrayList<String>
to hold the decoded strings. - Initialize a
position
variable to track the current index within the encoded string. - Use a while loop to process the encoded string until the end is reached.
- Inside the loop, identify the next occurrence of the separator
"/:"
to determine the end of the current length segment. - Extract the length of the next string using
Integer.parseInt()
and determine the exact substring representing the string using the identified length. - Add the extracted string to the list
resultArray
. - Adjust
position
to move past the current string and continue the process.
- Create an empty
The solution effectively handles the encoding and decoding of strings, avoiding common issues such as the interference of special characters within the strings themselves by encoding the length explicitly. This method ensures that each string is accurately retrieved during the decoding process regardless of the string content.
class StringCodec:
def encode(self, strings):
# Prepare an encoded string
encoded_result = ''
for item in strings:
# Join string length, delimiter, and the string
encoded_result += str(len(item)) + '/:' + item
return encoded_result
def decode(self, encoded_value):
# List to keep decoded strings
decoded_list = []
idx = 0
while idx < len(encoded_value):
# Locate the delimiter
delimiter_position = encoded_value.find('/:', idx)
# Extract the substring length
str_length = int(encoded_value[idx:delimiter_position])
# Extract the actual string
extracted_str = encoded_value[delimiter_position+2 : delimiter_position+2+str_length]
# Append the extracted string to list
decoded_list.append(extracted_str)
# Update index to move to the next string part
idx = delimiter_position + 2 + str_length
return decoded_list
The provided Python code defines a class StringCodec
with methods to encode and decode lists of strings. Here's a breakdown of its functionality:
Encoding:
- The
encode
method takes a list of strings and processes each string to form an encoded string. Each string is encoded by prefixing it with its length followed by the special delimiter '/:', and then appending the actual string itself. - For example, encoding ['hello', 'world!'] results in '5/:hello6/:world!'.
- The
Decoding:
- The
decode
method reverses this process. It takes an encoded string as input and decodes it back into the original list of strings. - It iterates over the encoded string, locating the delimiter to determine where one encoded part ends and next begins, extracting both the length of each string and the string itself.
- Using this information, it reconstructs and appends each string to a list, which contains the decoded strings after the loop completes.
- The
For practical usage:
- Initialize an instance of
StringCodec
. - Use
encode()
to convert a list of strings into a single encoded string. - Use
decode()
with an encoded string to get back the original list of strings. This allows for efficient storage and transmission of string data, particularly useful in scenarios where string manipulation and custom storage formats are required.
No comments yet.