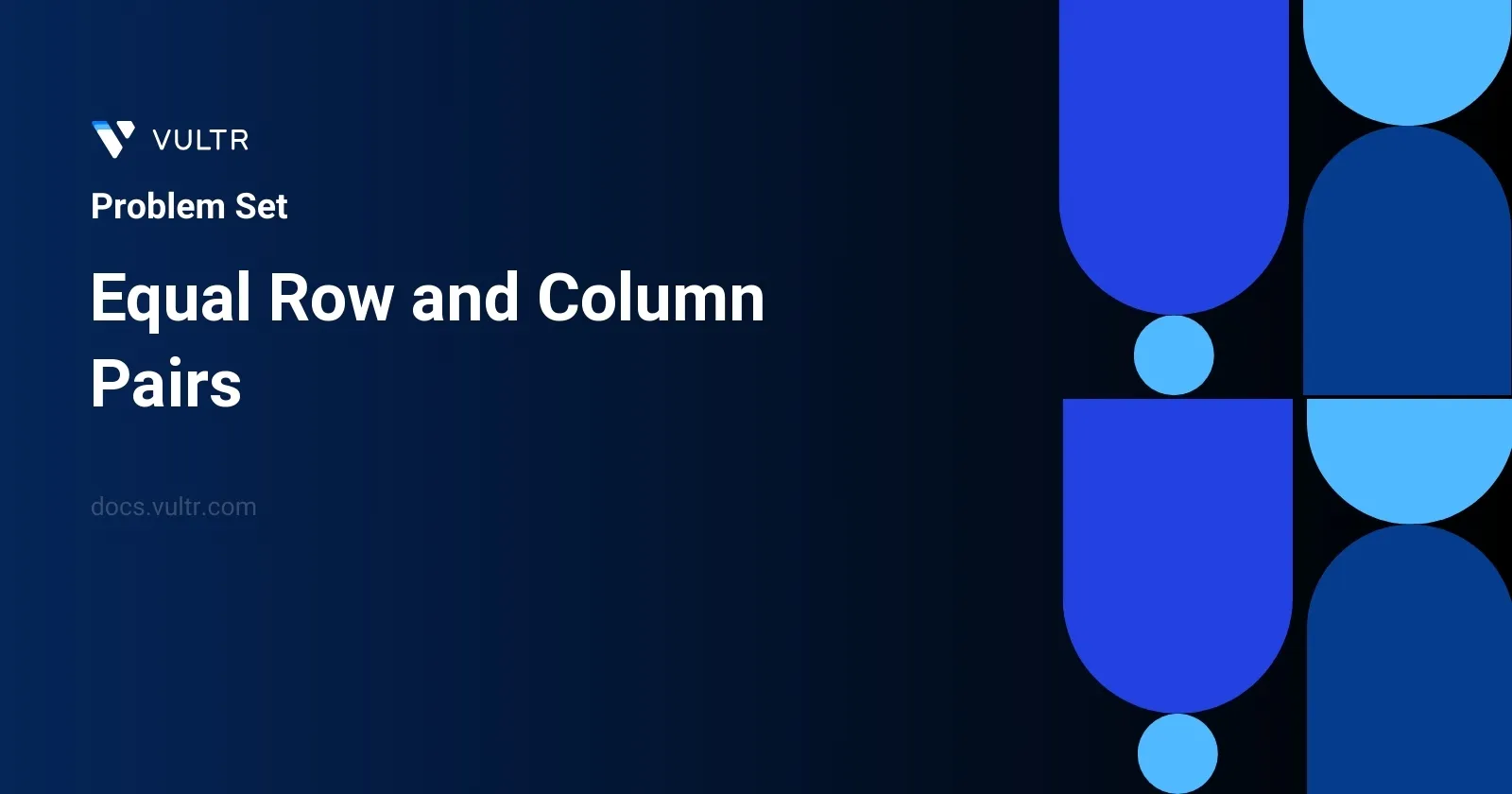
Problem Statement
The problem tasks you with identifying and counting the pairs of rows and columns in a square matrix (grid
) that are identical in terms of both the elements and their order. Specifically, you're given a 0-indexed n x n
integer matrix named grid
. Your objective is to determine how many pairs (ri, cj)
exist where row ri
and column cj
are exactly the same sequence of integers. This involves checking all possible pairs to see if the sequence of numbers in a row matches perfectly with the sequence of numbers in any column.
Examples
Example 1
Input:
grid = [[3,2,1],[1,7,6],[2,7,7]]
Output:
1
Explanation:
There is 1 equal row and column pair: - (Row 2, Column 1): [2,7,7]
Example 2
Input:
grid = [[3,1,2,2],[1,4,4,5],[2,4,2,2],[2,4,2,2]]
Output:
3
Explanation:
There are 3 equal row and column pairs: - (Row 0, Column 0): [3,1,2,2] - (Row 2, Column 2): [2,4,2,2] - (Row 3, Column 2): [2,4,2,2]
Constraints
n == grid.length == grid[i].length
1 <= n <= 200
1 <= grid[i][j] <= 105
Approach and Intuition
The problem revolves around finding identical pairs between rows and columns in an n x n
matrix. It can be visualized as traversing each row and checking it against every column to see if they are identical.
- Loop through each row of the matrix.
- For each row, loop through each column.
- Compare the current row and the current column element by element.
- If they match entirely, increment your count of identical pairs.
- Continue this process efficiently to keep the solution under acceptable runtime limits, considering the constraints provided.
- Key considerations include:
- A square matrix ensures that the number of rows is equal to the number of columns, simplifying iteration logic.
- Given the constraints, the maximum size of the matrix is
200 x 200
, leading to a need for efficient comparison methods, potentially considering hashing or direct list comparisons. - High values of elements (up to
10^5
) imply no special conditions on the range of numbers but might influence memory considerations if transformation of the matrix data (like hashing) is used.
The given examples illustrate the application of these rules to count the matching row-column pairs, providing clear scenarios where the matrix dimensions and the elements vary. This shows how the solution will need to handle different sizes and configurations efficiently.
Solutions
- Java
- Python
class TrieNode {
int countElem;
Map<Integer, TrieNode> childNodes;
public TrieNode() {
this.countElem = 0;
this.childNodes = new HashMap<>();
}
}
class Trie {
TrieNode root;
public Trie() {
this.root = new TrieNode();
}
public void insert(int[] sequence) {
TrieNode current = this.root;
for (int num : sequence) {
if (!current.childNodes.containsKey(num)) {
current.childNodes.put(num, new TrieNode());
}
current = current.childNodes.get(num);
}
current.countElem += 1;
}
public int find(int[] sequence) {
TrieNode current = this.root;
for (int num : sequence) {
if (current.childNodes.containsKey(num)) {
current = current.childNodes.get(num);
} else {
return 0;
}
}
return current.countElem;
}
}
class Solution {
public int equalPairs(int[][] matrix) {
Trie trieData = new Trie();
int matchCount = 0, n = matrix.length;
for (int[] row : matrix) {
trieData.insert(row);
}
for (int col = 0; col < n; ++col) {
int[] columnData = new int[n];
for (int row = 0; row < n; ++row) {
columnData[row] = matrix[row][col];
}
matchCount += trieData.find(columnData);
}
return matchCount;
}
}
The provided Java code defines a solution for determining the number of matching rows and columns in a given matrix using a Trie data structure. Here are the core components and functionality:
TrieNode Class
- This class represents each node of the Trie which contains:
countElem
: Counter for the number of times a sequence (row or column of the matrix) ends at this node.childNodes
: A map (integer to TrieNode) holding children nodes, essentially, the next number in the sequence and its corresponding TrieNode.
- This class represents each node of the Trie which contains:
Trie Class
- This class encompasses the operations related to the Trie:
insert(int[] sequence)
: Takes an array of integers (sequence) and inserts it into the Trie. If a number in the sequence does not exist as a child of the current node, it creates a new TrieNode for that number.find(int[] sequence)
: Checks if a sequence exists in the Trie and returns the count of how many times this exact sequence has been added (stored incountElem
).
- This class encompasses the operations related to the Trie:
Solution Class
- Contains the method
equalPairs(int[][] matrix)
which calculates how many times rows and columns in the matrix are equal.- A Trie named
trieData
is initialized. - Rows of the matrix are inserted into the Trie.
- Each column of the matrix is then constructed and checked against entries in the Trie using the
find
method. The total matches are accumulated inmatchCount
.
- A Trie named
- Contains the method
The final functionality allows the calculation of equal row and column pairs by leveraging a Trie to efficiently match and count the sequences, giving an optimized approach to solving the problem in question. This method highlights the effective use of Trie for multidimensional array operations, where sequences are key elements of the computation.
class TrieNode:
def __init__(self):
self.node_count = 0
self.children_map = {}
class TrieStructure:
def __init__(self):
self.root = TrieNode()
def insert(self, sequence):
node = self.root
for element in sequence:
if element not in node.children_map:
node.children_map[element] = TrieNode()
node = node.children_map[element]
node.node_count += 1
def search(self, sequence):
node = self.root
for element in sequence:
if element in node.children_map:
node = node.children_map[element]
else:
return 0
return node.node_count
class Solution:
def equalPairs(self, grid: List[List[int]]) -> int:
trie = TrieStructure()
pair_count = 0
grid_size = len(grid)
for row in grid:
trie.insert(row)
for index in range(grid_size):
column = [grid[row_index][index] for row_index in range(grid_size)]
pair_count += trie.search(column)
return pair_count
The given Python program implements a solution to the problem of finding equal row and column pairs in a grid using a Trie data structure. Here’s an overview of how the solution works:
Define a
TrieNode
class which contains:node_count
: to track the number of sequences that terminate at this node.children_map
: a dictionary to store child nodes.
Define a
TrieStructure
class which includes:- A root
TrieNode
. insert
method: inserts a sequence (array of integers) into the trie. Each element of the sequence becomes a node in the trie if not already present.search
method: searches for a sequence in the trie and returns the count of how many times that sequence has been inserted.
- A root
Define a
Solution
class with a methodequalPairs
:- Initializes a
TrieStructure
. - Loops through each row of the grid and inserts each row into the trie.
- Constructs each column of the grid iteratively and uses the
search
method to determine how many times the column appears as a row in the trie. This count is added topair_count
.
- Initializes a
The program effectively counts the number of times a row and column in a grid are identical by leveraging the efficient lookup and insert operations of a trie. The final output, pair_count
, represents the total number of pairs of identical rows and columns.
No comments yet.