Setup a Next.js Web Application on Ubuntu 20.10
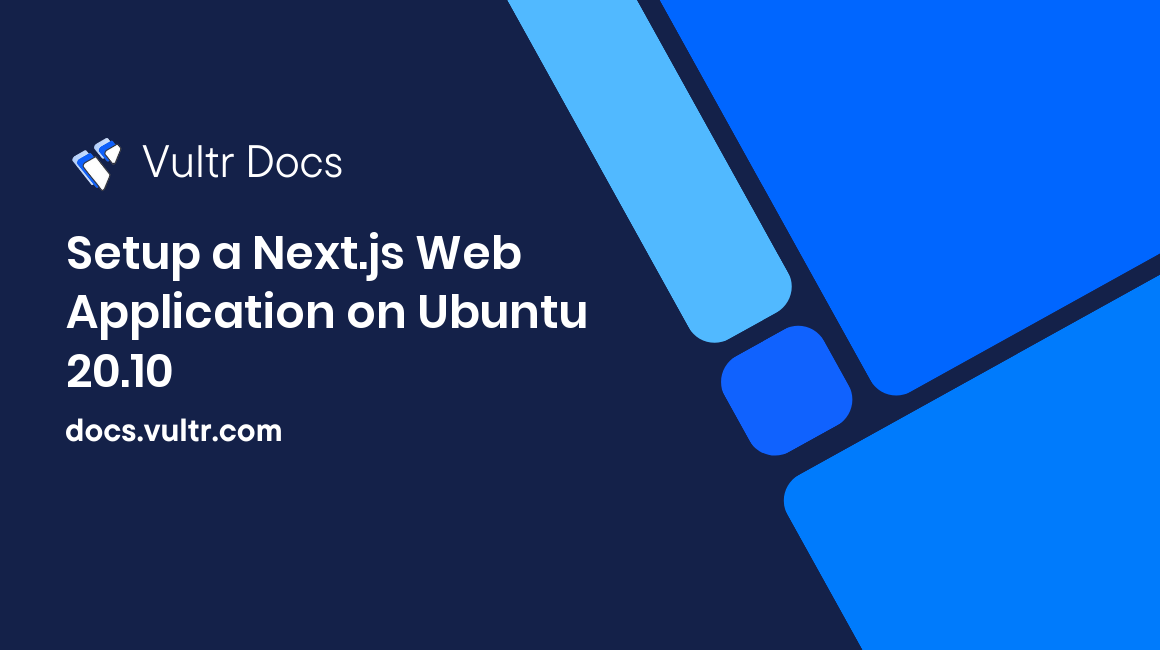
Overview
Next.js is a Javascript framework built on React.js, which allows developers to create server-side rendered and statically generated React applications.
Prerequisites
Add a non-root sudo user.
Follow the best practices to update Ubuntu.
Install build-essential:
$ sudo apt-get install build-essential
Installation
NodeJS and NPM
Install
curl
.$ sudo apt-get install curl
Add the latest stable release of NodeJS.
$ curl -sL https://deb.nodesource.com/setup_14.x | sudo -E bash -
Install NodeJS.
$ sudo apt-get install nodejs
Verify the installations.
$ node -v $ npm -v
Setup
Change to the preferred project root folder.
$ cd /path/to/desired/folder/
Initialize the Next.js project development environment with the
npx
CLI build tool. Replacemy-project
with your project name in all lowercase.$ npx create-next-app my-project
Change to the project directory list the directory contents.
$ cd my-project $ ls
The file structure of the project should resemble the following (excluding
node_modules
andpackage-lock.json
):path/to/project/ ├── package.json ├── pages ├── public ├── README.md └── styles
Start the development server.
$ npm run dev
After the console reports:
ready - started server on http://localhost:3000
Navigate to your server IP on port 3000 in a web browser.
http://192.0.2.123:3000/
Verify the web page says Welcome to Next.js!.
To create an optimized production build of your project, close the development server with Ctrl + C then run:
$ npm run build && npm run start
After the command completes, a web server begins serving your web application. Navigate to your server IP on port 3000 and view the production build. This time it should load much faster.
Custom Server for Static Files
To use a server like Nginx or Apache to host the files, export a static build of your project. Your project may lose some functionality because features like API routes are not available to static sites. To use your server and keep these dynamic functions, refer to the Next.js Custom Server API.
To use your own server, edit the package.json
file in the project's root directory. Add another script under the scripts
object with the key of export
and a value of next export
. Your modified package.json
should look like this:
{
"name": "my-project",
"version": "0.1.0",
"private": true,
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"export": "next export"
},
"dependencies": {
"next": "10.0.3",
"react": "17.0.1",
"react-dom": "17.0.1"
}
}
After editing the package.json
file, run the following the generate a static build of your web application:
$ npm run build && npm run export
Look for a folder named out
in your project directory, which contains your exported project. Use this folder to serve your static web application directly with a server of your choice.