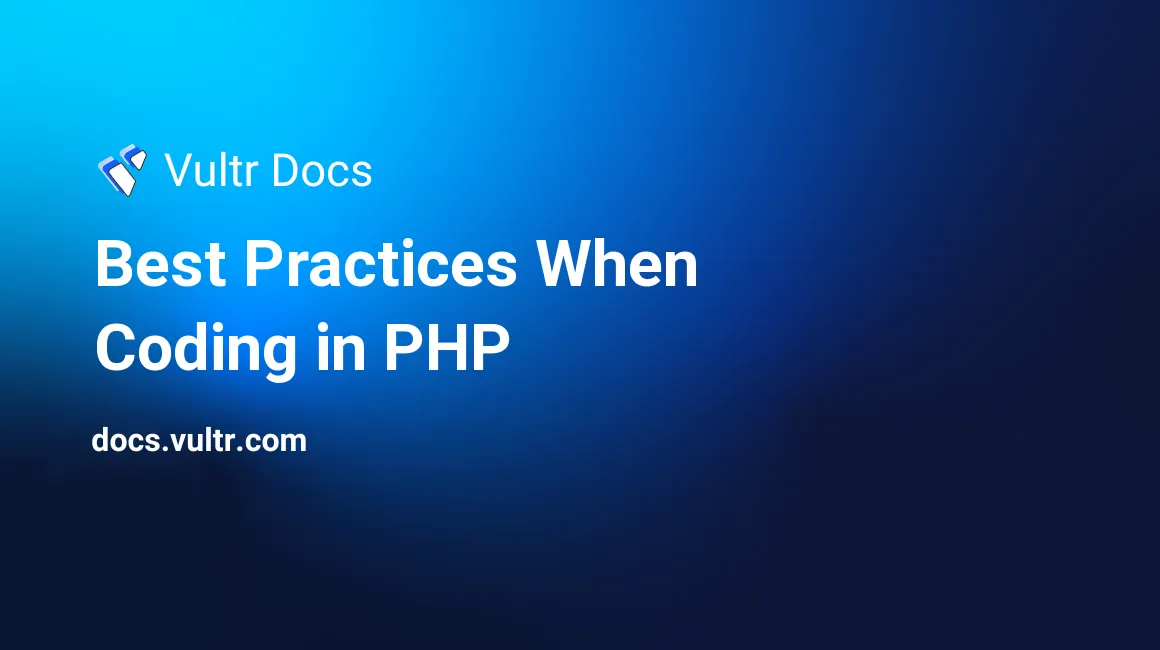
Introduction
PHP is still one of the most popular server-side scripting languages and is supported by a vibrant open-source community. It powers the world's best content management systems(CMSs), including WordPress, Joomla, and Magento. The PHP syntax is easier to learn, but to become a good developer, you must adopt some coding standards.
This reference guide focuses on different principles that you should follow when formating your PHP code for your next project.
Indenting Your Code
When coding on different interactive development environments(IDEs), using a tab may not always guarantee the same number of spaces. However, using four spaces instead of a tab to indent your code is a better idea. A good indentation standard does not only guarantee consistency but allows other developers to easily scan and understand nested code blocks.
Next, if a file contains pure PHP code, omit the closing tag ?>
. This ensures that you don't accidentally put extra white spaces or newlines, which may introduce errors when working with PHP headers
, include()
, and require()
functions.
Here is a good example that illustrates the above principles.
<?php
$account_id = $_POST['account_id'];
if ($account_id == 1) {
echo "Administrator account";
}
Working with Classes and Methods
When working with classes and methods, put the opening braces {
in a new line before the body. Also, ensure the closing braces }
go to a new line after the body.
Always declare the visibility of all class properties(For instance, private $db_gateway
and public $fields
) and methods (For example public function createRecord(...)
). Don't use the var
keyword to declare class properties. Also, separate method arguments (for example, $params, $user, $caller
) with a comma and a space but ensure there is no space before the comma.
When it comes to naming conventions, use lowercase for PHP keywords (for instance, strtolower
) and StudlyCase/PascalCase for class names. For instance Products
, ProductsCategories
, SavingsAccounts
, and CustomerOrders
are good names for classes. Use camelCase to name method names and ensure all constants are in uppercase letters with underscore separators.
For variable names, you can either choose lowercase with underscores for compound words (for example, $account_name
) or camelCase($accountName
). Whatever you choose, stick to it throughout your project.
The PHP code below illustrates the above coding principles.
<?php
private $db_gateway;
public $fields;
const FIELDS = 'product_id, product_name';
const PER_PAGE = 250;
class Products
{
public function createRecord($params, $username, $caller)
{
$user = strtolower($username);
$account_name = $params['account_name']; // or $accountName = $params['account_name'];
}
}
Formatting Control Structures
When coding control structures, put the opening braces {
in the same line with the condition
but ensure the closing brace }
is on the next line after the body. Also, make sure you have a space after the control structure keyword(if (...
) and before the opening brace {
. For readability purposes, indent the control structure's body once.
The following code snippet encompasses the above guidelines.
<?php
...
if ($debit >= $balance) {
echo "Insufficient funds.";
} else {
echo "Transaction processed.";
}
The same spacing and indenting principles apply to the switch
and case
statements, as illustrated below.
<?php
switch ($http_method) {
case 'POST':
echo 'Creating record...';
break;
case 'PUT':
echo 'Updating record...';
break;
case 'DELETE':
echo 'Deleting record...';
break;
case 'GET':
echo 'Retrieving records...';
break;
default:
echo 'Unsupported Method';
break;
}
Formatting Looping Structures and Try Catch Blocks
The PHP while
statement runs a block code as long as a certain condition remains true
. Surround the condition of the statement with one space before and after. Also, put the opening brace {
on the same line with the condition you're evaluating and move the closing brace }
in a new line after the body. Remember to indent the body once.
...
while ($condition) {
// body
}
...
The PHP for
statement is useful in circumstances where you know the number of times you want to execute a block of code. Follow the spacing and formatting conventions below when creating the for
statements.
...
for ($i = 0; $i < 10; $i++) {
// Body you want to be executed in each iteration
}
...
The foreach
statement comes in handy when iterating through arrays. Use the syntax below to space and indent the loop statement.
...
foreach ($arry as $key => $value) {
// code to be executed
}
...
To handle errors effectively in PHP, you should use a try {...} catch (...) {...}
block. The following code snippet shows the proper spacing and formatting guidelines that you should use in your code.
try {
// try body
} catch (Exception $e) {
echo 'Message: ' . $e->getMessage();
}
Concatenation and Spacing
Make sure the concatenation .
, arithmetic (+
, -
, /
, *
), assignment(=
), and logical operators are preceded and followed by at least one space as shown below.
<?php
$username = 'james';
$customer = 'peter';
$sales_id = 1456
$order_amount = 2400;
$tax_amount = 50;
$order_status = 'Shipped';
$total_amount = ($order_amount + $tax_amount);
if ($order_status == 'Shipped') {
$message = 'Dear ' . $customer . ' the status of your order # ' . $sales_id . ' amounting to ' . $total_amount . ' is ' . $order_status ;
}
Autoloading Classes
To avoid unnecessary declarations of require()
, require_once()
, include()
, or include_once()
statements when loading classes, use the PHP auto-loading function. For this function to work effectively, name your class files with the same name as the class names.
Next, create an autoloader
function that takes the class name as the argument($class
) and uses the spl_autoload_register()
function to load the class automatically. Next time you can declare a new class, the spl_autoload_register()
function will load a class with that name.
For instance, in the code snippet below, the new Products();
statement references to a class named Products
.
<?php
function autoloader($class) {
require_once $class . '.php';
}
spl_autoload_register('autoloader');
$products = new Products();
Autoloading PHP classes makes your code neater and shorter, especially if you want to include certain classes in multiple files.
Conclusion
This was a quick reference guide that highlighted the best formatting standards for PHP code. You've seen several principles when it comes to naming conventions, indenting, and formating different blocks of codes in PHP. While the PHP software does not force any of these conventions when executing, the important thing here is consistency and ease of code maintenance. Therefore, follow the above guidelines when coding your next PHP project.
No comments yet.