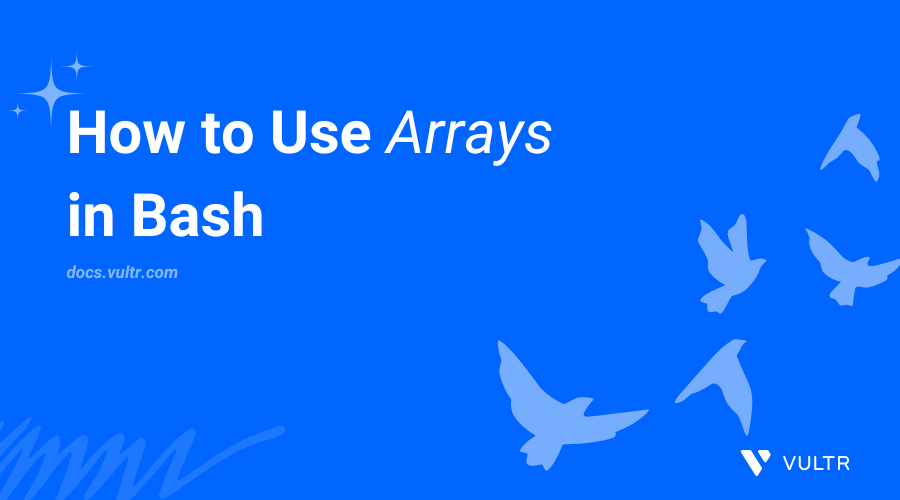
Introduction
Arrays in Bash are fundamental data structures that store multiple values in a single variable. Arrays offer fast and efficient methods to represent and manipulate related data as a single group. Bash supports two types of arrays:
- Indexed Arrays: A collection of ordered values that use indices starting from
0
. - Associative Arrays: A collection of key-value pairs.
This article explains how to use arrays in Bash to store and reference data. Mastering the Bash case statement is crucial for handling conditional logic in scripts efficiently.
Arrays Syntax in Bash
Arrays in Bash use the declare
command to initialize values based on the array type. The lowercase character a
declares an indexed array while an uppercase A
declares an associative array. Bash supports indexed arrays by default unless you use the declare
command.
Direct initialization:
array=(element1 element2 element3)
Initializing encloses an array in brackets
()
and separates values with a space. Direct initialization creates an indexed array you can reference using the numeric index of each element.Indexed arrays:
declare -a array
Associative arrays:
declare -A array
Array reference syntax.
${array[index]}
You can reference Arrays in Bash using the curl braces
${}
and specify an index position to access an element.
Create Arrays in Bash in Script
To create arrays in Bash, use variables and apply one or more elements to the array using the equals sign =
. Follow the steps below to create arrays in Bash and retrieve values based on the index position or key.
Create a new array, such as
new_array
using the direct declaration method and assign it the1
,2
, and3
values.console$ new_array=(1 2 3)
Print all array elements.
console$ echo ${new_array[@]}
Output:
1 2 3
Declare a new indexed array, such as
index_array
.console$ declare -a index_array
Assign
value 1
,value 2
, andvalue 3
values to the array.console$ index_array[0]="value 1" $ index_array[1]="value 2" $ index_array[2]="value 3"
Print a single element in the array using its index. For example, for
value 2
, use an index of1
.console$ echo ${index_array[1]}
Output:
value 2
Declare a new associative array.
console$ declare -A assoc_array
Assign
string 1
,string 2
, andstring 3
values to the array.console$ assoc_array[key1]="string 1" $ assoc_array[key2]="string 2" $ assoc_array[key3]="string 3"
Print a single element in the array.
console$ echo ${assoc_array[key1]}
Output:
string 1
Print all elements in the associative array.
console$ echo ${assoc_array[@]}
Output:
string 2 string 3 string 1
To handle multiple values effectively, learn how to use variables with arrays in Bash for efficient data storage. Check out our in-depth guide on how to use variables with arrays in Bash for more advanced scripting techniques.
Manipulate Arrays in Bash
You can manipulate arrays in Bash to lists, add, or remove new elements without affecting the array structure. This is important when using arrays with loops or conditional statements to set new values for elements. Follow the steps below to create an indexed array and modify its elements in Bash.
Create a new indexed array, such as
my_index_array
and setApache
,Nginx
,MySQL
, andPHP
as sample values.console$ new_index_array=("Apache" "Nginx" "MySQL" "PHP")
Print all elements in the array.
console$ echo ${new_index_array[@]}
Output:
Apache Nginx MySQL PHP
Add new elements to the array using the
+=
operator.console$ new_index_array+=("Angular" "PostgreSQL")
Add a new element to the array and specify its index.
console$ new_index_array[9]="Perl"
Modify an element in the array. For example, change
PHP
toPHP-FPM
.console$ new_index_array[3]="PHP-FPM"
Delete individual elements in the array using the
unset
command.console$ unset new_index_array[2]
Print all elements in the array.
console$ echo ${new_index_array[@]}
Output:
Apache Nginx PHP-FPM Angular PostgreSQL
Count the number of elements in the array.
console$ echo ${#new_index_array[@]}
Output:
5
The
#
prefix in the array name counts all elements.
Use Arrays with User Input
You can use arrays to handle user input and store multiple values as elements. Each space in the user input creates a new element in an array that you can reference using the element's index or key. Follow the steps below to create a Bash script that uses the read
command to store new array elements based on the user's input.
Create a new
user_input.sh
script.console$ nano user_input.sh
Add the following contents to the
user_input.sh
file.bash#!/bin/bash echo "Hello, enter the values Apache Nginx MySQL separated by spaces:" read -a user_array echo "You entered: ${user_array[@]}"
Save and close the file.
The above Bash script prompts a user to input values and stores each value in an indexed
input_array
array before outputting all elements using theecho
command.Run the script using Bash.
console$ bash user_input.sh
Output:
Hello, enter the values Apache Nginx MySQL separated by spaces: Apache Nginx MySQL You entered: Apache Nginx MySQL
Use Arrays with Loops in Bash Scripts
You can iterate through array elements with loops such as the for
loop and continuously execute specific commands or actions. Follow the steps below to create a new Bash script that iterates through an array and prints each element on a new line.
Create a new
for-arrays.sh
script.console$ nano for-arrays.sh
Enter the following contents to the
for-arrays.sh
file.bash#!/bin/bash new_index_array=("Apache" "Nginx" "MySQL" "PHP") for value in ${new_index_array[@]} do echo $value done
Save and close the file.
The above Bash script uses a
for
loop to iterate through an array and prints each element on a new line.Run the script using Bash.
console$ bash for-arrays.sh
Output:
Apache Nginx MySQL PHP
Use Arrays with Regular Expressions (Regex) in Bash
You can use arrays with regular expressions (regex) to match patterns against multiple elements in an array. Follow the steps below to create a bash script that uses an indexed array of strings and a regex pattern to iterate through all elements using a for
loop.
Create a new
array_regex.sh
script.console$ nano array_regex.sh
Add the following contents to the
array_regex.sh
file.bash#!/bin/bash new_index_array=("Apache" "Nginx" "MySQL" "PHP") pattern="^[anmpANMP].*" for app in "${new_index_array[@]}"; do if [[ $app =~ $pattern ]]; then echo "$app can be installed on this system" fi done
Save and exit the file.
The above Bash script creates a new variable with a list of strings and uses the regex pattern
^[].*
to test each element using theif
conditional statement.Run the script using Bash.
console$ bash array_regex.sh
Output:
Apache can be installed on this system Nginx can be installed on this system MySQL can be installed on this system PHP can be installed on this system
Conclusion
You have used arrays in Bash to store data and perform specific operations to retrieve or manipulate elements. Arrays store multiple values and allow you to integrate other Bash operations, such as functions, loops, or conditional statements to execute specific commands or reference data.
No comments yet.