How to Use Variables in Bash
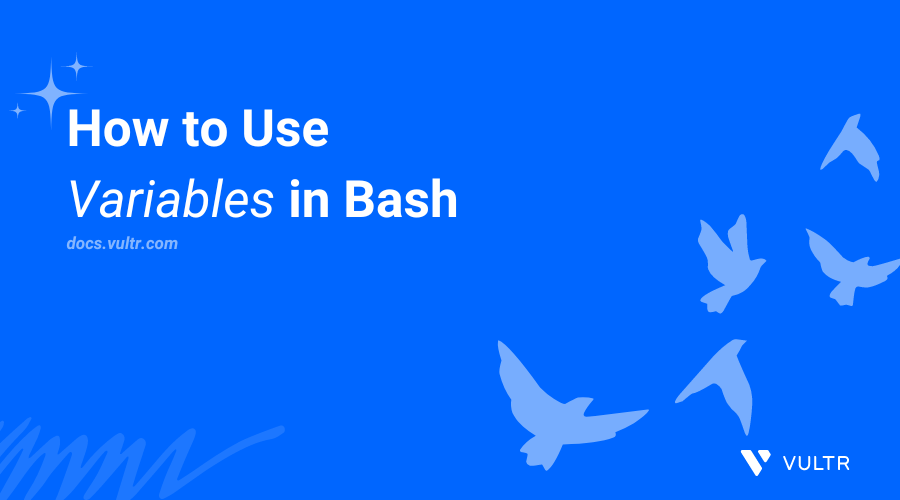
Introduction
Variables in Bash are symbolic names that store data references in a script. You can use variables in conditions, loops, and user input. Variables handle different data types, including arithmetic, symbolic characters, and string values.
This article explains how to use variables in Bash scripting to store and manipulate data.
Variables Syntax in Bash
Variables in Bash use a direct naming syntax followed by an equals sign =
to store a value. A variable name should not contain a space and an equals sign. The following is a basic variable syntax in Bash:
variable_name=value
Create Variables
Variables can store different types of data, including user input. To create a variable, specify a name and assign it a value. You can manipulate variables in different contexts like conditional structures or loops, to store different values when executing specific commands.
Strings and special character values use double quotes ""
to instruct Bash not to interpret the value as a command while arithmetic values do not require quotes ""
to store data. Variable names also support the following characters:
- Letters:
a-z
andA-Z
- Digits:
0-9
(You can not use a digit as the first character in a variable name.) - Underscores:
_
Follow the steps below to create and assign variable in a Bash shell environment or script.
Create a new string variable such as
name
and set the value toJohnDoe
.console$ name="JohnDoe"
You can set a variable in bash to store strings or numbers, and later retrieve them for use in scripts or commands.
Create an integer variable such as
age
and set the value to20
.console$ age=20
Create a new
variable_data2
string variable and use a numeric character2
in its name.console$ variable_data2="Hello World"
Create a new
combine.sh
Bash script file using a text editor like Nano to combine the variables.console$ nano combine.sh
Add the following contents to the
combine.sh
file.bash#!/bin/bash name="JohnDoe" age=20 variable_data2="Hello World" echo "$variable_data2 age: $age name: $name"
Save and close the file.
Run the script.
console$ bash combine.sh
Output:
Hello World age: 20 name: JohnDoe
Retrieve Variable Value
Append the $
character before a variable name to retrieve the variable's value. When you call a variable, Bash retrieves the most recent value. Follow the steps below to retrieve variable data.
Create a new
app_name
variable and set the value toapache
.console$ app_name="apache"
Retrieve the
app_name
variable value using theecho
command.console$ echo $app_name
Output:
apache
Create a
digit1
integer variable and set the value to1
.console$ digit1=1
Create another
digit2
integer variable and set the value to2
.console$ digit2=2
Create a
sum
variable and set the following function to sum thedigit1
anddigit2
variables.console$ sum=$((digit1 + digit2))
Retrieve the
sum
variable value to view the arithmetic operation result.console$ echo "The total is: $sum"
Output:
The total is: 3
Create a new Bash script, such as
script.sh
to combine the variables and output the respective values.console$ nano script.sh
Add the following contents to the file.
bash#!/bin/bash app_name="apache" echo $app_name digit1=1 digit2=2 echo "The variable values are: $digit1, $digit2" sum=$((digit1 + digit2)) echo "The total is: $sum"
Save and close the file.
Run the script using Bash.
console$ bash script.sh
Output:
apache The variable values are: 1, 2 The total is: 3
Use Variables with Arrays in Bash
You can use variables to store multiple values when working with arrays in Bash. You can then retrieve the value of each variable in the array by referencing its index. Variables in arrays help you fetch specific data in operations such as conditional statements. Follow the steps below to use variables with arrays in Bash.
Create a new array variable such as
my_array
and set the following multiple string values.console$ my_array=("value1" "value2" "value3" "value4")
You can set a variable in bash to store arrays, allowing you to access specific elements using indexed positions.
Retrieve the first value in the variable by referencing a
0
index.console$ echo ${my_array[0]}
Output:
value1
Retrieve the fourth value in the variable.
console$ echo ${my_array[3]}
Output:
value4
Create a new
arrays.sh
Bash script to combine the variables in a single file.console$ nano arrays.sh
Add the following contents to the
arrays.sh
file.bash#!/bin/bash my_array=("value1" "value2" "value3" "value4") echo ${my_array[0]} echo ${my_array[3]}
Save and close the file.
Run the script using Bash.
console$ bash arrays.sh
Output:
value1 value4
Use Variables in Bash Scripts to Store and Manipulate Data
Variables store and allow you to manipulate data in Bash scripts when executing specific commands or conditions. Follow the steps below to create a Bash script that reads the user's input, stores it in a new variable, retrieves the variable data, and manipulates the variable with a new value to output a final result.
Create a new
variables.sh
Bash script.console$ nano variables.sh
Add the following code to the
variables.sh
file.bash#!/bin/bash read -p "Please enter your name: " name echo "Hello: $name" name_uppercase="${name^^}" echo "Hello again: $name_uppercase"
Save and close the file.
The above script creates and assign new variable
name
from the user's input and outputs the variable value in bash script. The script then manipulates the variable value and converts it to uppercase using^^
to output the new value.Run the script using Bash.
console$ bash variables.sh
Output:
Please enter your name: example Hello: example Hello again: EXAMPLE
Conclusion
You have used variables in Bash to store and manipulate data. Variables can store different data types when working with arithmetic operations, conditional statements, or loops to generate final results based on the variable's data.