How to Create a Bash Script and Execute It
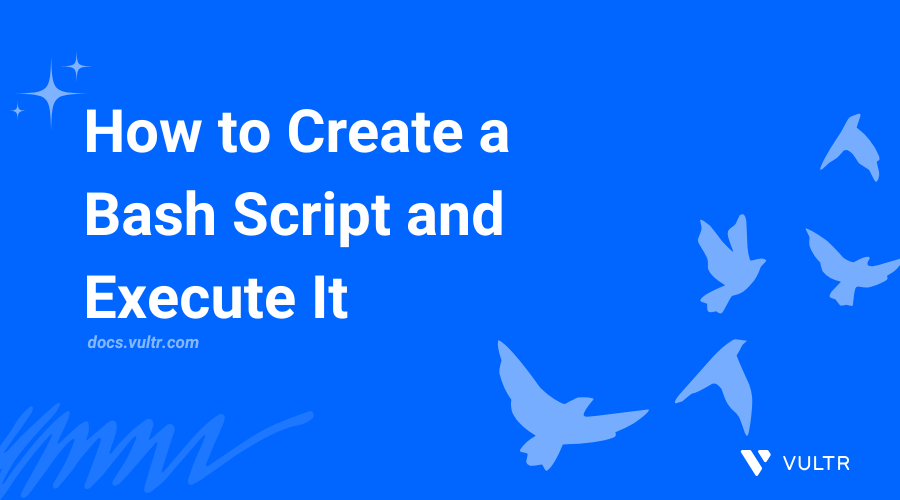
Introduction
Bash scripting is an effective method to create a Bash script that automates repetitive tasks, processes data, or manages system operations by executing a series of commands in a Bash shell environment. It utilizes specific commands and logic to streamline various operations.
This article explains how to create and execute a Bash script.
Bash Script Structure
A Bash script begins with a #!/bin/bash
shebang that specifies the Bash interpreter path. The script body consists of executable commands or logic processed line by line. Constructs such as variables, loops, arrays, and functions are defined in the script body to perform specific tasks. A Bash script does not require ending clauses, stop commands, or special terminators such as ;
that indicate the end of a script.
A Bash script uses the following structure:
#!/bin/bash
# Commands
Visit the Understanding the Bash syntax article for more information about the Bash syntax and supported elements.
Create a Bash Script
You can create a Bash script as a regular file using any extension. The .sh
file extension is commonly used with Bash scripts for improved identification and execution. A script should use the correct shebang to enable execution using the Bash interpreter. Follow the steps below to create a new Bash script using the .sh
file extension.
Create a new
basic.sh
file.console$ touch basic.sh
Log list the files in your working directory and verify that the script is available.
console$ ls -l
Output:
-rw-r--r-- 1 user user 0 Oct 2 14:08 basic.sh
The
basic.sh
file in the above output is a regular file with no content. It's not a valid Bash script until you add a shebang and executable commands to the file.Open the file using a text editor such as
nano
.console$ nano basic.sh
Add the following contents to the file.
bash#!/bin/bash echo "Hello World! This is a Bash script"
Save the file.
The above script uses the Bash interpreter to execute the file and display a
Hello World
message using theecho
command.Create another
exec-order.sh
script.console$ nano exec-order.sh
Add the following contents to the file.
bash#!/bin/bash echo "This is executed first" echo "This is executed next" echo "This is executed last"
Save the file.
Bash executes all commands in the above script sequentially until the end of the file. You can use conditional statements, variables, or loops to reference previously executed commands or manipulate the values to perform specific tasks.
Create another
advanced-exec.sh
file.console$ nano advanced-exec.sh
Add the following contents to the file.
bash#!/bin/bash variable1="Hello World" echo "This is executed second" echo "The $variable1 variable was executed first" echo "The last [ $var_last ] variable's value cannot display because it's executed next and not yet in memory" var_last="This is executed last"
Save the file.
Bash executes all commands within the above script one by one and stores the variables in memory.
variable1
is executed first before the followingecho
commands and its value remains in memory for reference within the script.
Make a Bash Script Executable
A Bash script is not executable when you create it, depending on your file system permissions. The default permissions mode for a Bash script on Linux systems is 644
, allowing the owner to read and write, while the group and other users can only read the file. Specifying the Bash shell when running the file executes the script if the file structure is correct. Follow the steps below to make a Bash script executable.
List the file permissions in your working directory.
console$ ls -l
Output:
total 12 -rw-r--r-- 1 user user 290 Oct 2 14:59 advanced-exec.sh -rw-r--r-- 1 user user 63 Oct 2 14:58 basic.sh -rw-r--r-- 1 user user 119 Oct 2 14:58 exec-order.sh
Verify that the Bash scripts you created earlier are available and do not include the
x
permission based on the above output.Enable execute permissions on the
basic.sh
file to make the Bash script executable.console$ chmod +x basic.sh
Enable execute permissions on the
exec-order.sh
file.console$ chmod +x exec-order.sh
Enable execute permissions on the
advanced-exec.sh
file.console$ chmod +x advanced-exec.sh
Long list the file permissions again and verify that execute permissions are active on all scripts.
console$ ls -l
Output:
-rwxr-xr-x 1 user user 290 Oct 2 14:59 advanced-exec.sh -rwxr-xr-x 1 user user 63 Oct 2 14:58 basic.sh -rwxr-xr-x 1 user user 119 Oct 2 14:58 exec-order.sh
Execute permissions
x
are active for the file owner, group, and other users on the system based on the above output. All users can execute the Bash script on the file system to perform the tasks defined in the files.
Execute a Bash Script
You can execute a Bash script as a regular script or using the Bash interpreter. Follow the steps below to run the previously created Bash scripts and view their command outputs.
Execute the
basic.sh
file as a regular script.console$ ./basic.sh
Output:
Hello World! This is a Bash script
Execute the
exec-order
script using the Bash interpreter.console$ bash exec-order
Output:
This is executed first This is executed next This is executed last
Execute the
advanced-exec.sh
script.console$ ./advanced-exec.sh
Output:
This is executed second The Hello World variable was executed first The last [ ] variable's value cannot display because it's executed next and not yet in the Bash memory
You have executed all Bash scripts in your working directory using the regular script execution and Bash interpreter methods. Executing a Bash script as a regular script allows other tools such as automation programs to run the included commands and perform specific tasks.
Conclusion
In this article, you have created and executed Bash scripts with examples to perform specific tasks on a system. By following the steps to create a Bash script, you can use Bash scripts with functions, conditional statements, variables, and loops to execute or automate specific tasks on a system.