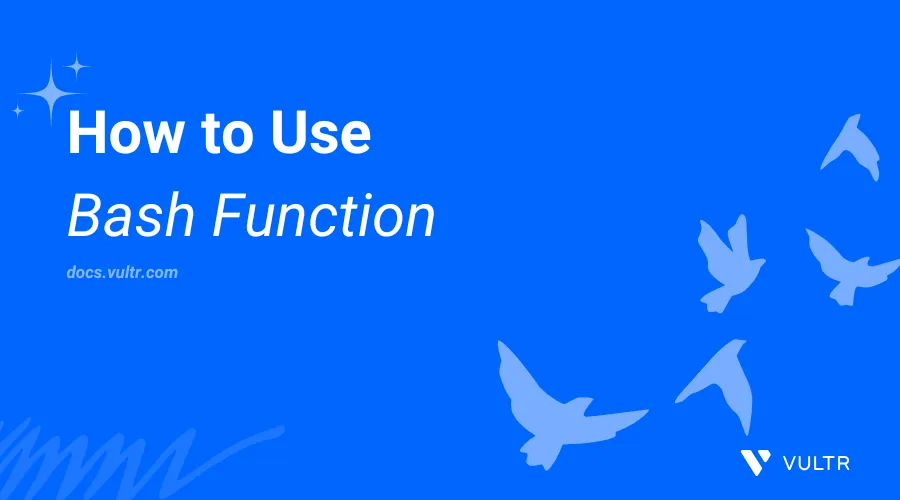
Introduction
Bash functions are reusable blocks of commands that enhance script readability and prevent task duplication by allowing other elements to reference them. A function in Bash allows you to group commands that perform a specific task. You can call and execute functions by referencing their names in any part of a script or a Bash shell environment.
This article explains how to use functions in Bash to efficiently write reusable commands in scripts.
The Bash Function Syntax
You can define a function in Bash using two formats depending on your scripting style. You can use the function name and parenthesis ()
or the function
keyword followed by a function name as detailed below.
Bash function syntax with parenthesis
()
.function_name () { #commands-to-run }
Inline parenthesis format:
function_name () { #commands-to-run; }
Bash function syntax with the
function
keyword.function function_name { #commands-to-run }
Inline
function
format:function function_name { #commands-to-run; }
Create Bash Functions
You can create a function in a Bash shell environment or a script and reuse it by calling its name. Follow the steps below to create Bash functions using the parenthesis and function keyword methods.
Create a new inline
greet
function using the parenthesis method in a shell environment.console$ greet () { echo "Hello World! Greetings from Vultr"; }; greet
Output:
Hello World! Greetings from Vultr
Create an inline
greet
function using thefunction
keyword method.console$ function greet () { echo "Hello World! Greetings from Vultr"; }; greet
Output:
Hello World! Greetings from Vultr
Create a new
greet.sh
script.console$ nano greet.sh
Add the following contents to the
greet.sh
file.bash#!/bin/bash function greet () { echo 'Hello World! This is a function' } greet
Save and close the file.
In the above script, the
greet
function outputs theHello World! This is a function
message using theecho
command when the script calls thegreet
function.Run the script using Bash.
console$ bash greet.sh
Output:
Hello World! This is a function
Call Bash Functions
Calling functions invokes the commands or code within them. You can call a function in Bash using its name and include optional arguments to invoke specific commands within the function. You can pass the following arguments when calling functions in Bash:
$0
: References the function name.$1
,$2
, etc: Refers to positional function parameters.${!variable}
: Accesses the value of variable with indirect referencing.$#
: Stores the number of positional parameters to pass to a function.$*
: Expands all positional parameters into a single string separated by spaces.$@
: Expands positional parameters as separate quoted strings.
Follow the steps below to create, call, and pass function arguments to invoke specific commands.
Create a new
call-functions.sh
script.console$ nano call-functions.sh
Add the following contents to the
call-functions.sh
file.bash#!/bin/bash greet="Hello World!" function greeting () { echo $greet } echo "Calling the function" greeting
Save and close the file.
In the above script, the
greeting
function imports thegreet
variable value and outputs it using theecho
command when the script calls the function.Run the script using Bash.
console$ bash call-functions.sh
Output:
Calling the function Hello World!
Create another
call-function-args.sh
script.console$ nano call-function-args.sh
Add the following contents to the
call-function-args.sh
file.bash#!/bin/bash function calculate() { local a=$1 local b=$2 local sum=$((a + b)) echo "The sum of $a and $b is: $sum" } calculate 5 10
Save and close the file.
In the above code, the script calls the
calculate
function using the$1
and$2
arguments to forward the input result as values to the locala
andb
variables. Thesum
variable stores the sum of thea
andb
variables while theecho
command outputs the final result.Run the script using Bash.
console$ bash call-function-args.sh
Output:
The sum of 5 and 10 is: 15
Return Status Using Functions
Unlike other programming languages, Bash functions do not return status codes automatically. A function returns the status of the last command by default, 0
for success, or a non-zero number between 1
to 255
for failure. Follow the steps below to use the return
statement to terminate a function and display a specific custom status codes.
Create a new
return-function.sh
script.console$ nano return-function.sh
Add the following contents to the
return-function.sh
file.bash#!/bin/bash function greetings() { echo "Hello World!" return 200 } greetings func_status=$? echo "The Return status is: $func_status"
Save and close the file.
In the above code, the script calls the
greetings
function to output aHello World
message, passes the status code to the$?
variable, and returns a non-zero200
status code.Run the script using Bash.
console$ bash return-function.sh
Output:
Hello World! The Return status is: 200
Exit Command in Bash Functions
The exit
command terminates an entire function and returns the exit status code. exit
is similar to return
and allows exiting a function in case of any errors or unmet conditions to stop all additional executions. Follow the steps below to use the exit
command to return the exit status in a function.
Create a new
exit-function.sh
script.console$ nano exit-function.sh
Add the following contents to the
exit-function.sh
file.bash#!/bin/bash function greetings() { echo "Hello World!" exit 2 echo "This can't run, the script has exited" } greetings
Save and close the file.
In the above script, the
exit
command terminates the function from executing any further tasks and returns a non-zero exit code of2
.Run the script using Bash.
console$ bash exit-function.sh
Output:
Hello World!
Use Variables with Functions
Variables in Bash store specific data that you can use with functions to hold repeated values or manipulate data. Functions support global and local variables:
- Global Variables: Declared outside a function body and are accessible in any part of a script.
- Local Variables: Declared inside a function using the
local
keyword and are only accessible inside the function.
Follow the steps below to create and reference variables in functions.
Create a new
var-function.sh
script.console$ nano var-function.sh
Add the following contents to the
var-function.sh
file.bash#!/bin/bash greeting="Hello" read username function greetings() { local name="$1" echo "$greeting, $name!" } greetings $username
Save and close the file.
In the above script, the
greetings
function uses a localname
variable and imports the global variablegreeting
. The function inputs user data from theusername
variable using the read command value as an argument. Theecho
command combines the variables into a single result and displays a message when the script calls the function.Run the script using Bash.
console$ bash var-function.sh
Enter a name such as
John Doe
when prompted to test the function in Bash.John Doe Hello, John!
Use Bash Functions with Loops
You can use Bash functions with loops to continuously execute commands or tasks over several times. Bash functions work with for
, while
, and until
loops to execute repetitive tasks within or outside a function. Follow the steps below to use loops within and outside a Bash function to execute repeated tasks.
Create a new
loop-in-function.sh
script to use internal loops.console$ nano loop-in-function.sh
Add the following contents to the
loop-in-function.sh
file.bash#!/bin/bash function count() { local compare="$1" local var=0 for ((var=0; var<compare; var++)); do echo "Current value: $var" done } count 10
Save and close the file.
In the above script, the
count
function uses afor
loop to continuously increment the value of thevar
variable and print its current value if it's less than the input argument value of10
stored in thecompare
local variable.Run the script using Bash.
console$ bash loop-in-function.sh
Output:
Current value: 0 Current value: 1 Current value: 2 Current value: 3 Current value: 4 Current value: 5 Current value: 6 Current value: 7 Current value: 8 Current value: 9
Create another
loop-out-functions.sh
script to use external loops.console$ nano loop-out-functions.sh
Add the following contents to the
loop-out-functions.sh
file.bash#!/bin/bash function greetings() { local username="$1" echo "Hello, $username!" } names=("John" "Doe" "Admin" "Jane" "root" ) for username in "${names[@]}"; do greetings "$username" done
Save and close the file.
In the above script, the
greetings
function imports theusername
variable from the input argument when the externalfor
loop calls the function. Thefor
loop continuously sets a newusername
value from thenames
array.Run the script using Bash.
console$ bash loop-out-functions.sh
Output:
Hello, John! Hello, Doe! Hello, Admin! Hello, Jane! Hello, root!
Use Bash Functions With Conditional Statements
Bash Functions use conditional statements to execute specific commands or tasks when a condition evaluates to true
. You can use conditional statements such as if
, elif
, if-then
, and case
inside or outside functions to execute specific commands. Follow the steps below to use conditional statements with functions in Bash.
Create a new
if-elif-in-function.sh
script.console$ nano if-elif-in-function.sh
Add the following contents to the
if-elif-in-function.sh
file.bash#!/bin/bash function count() { read var if (( var > 0 )); then echo "$var is greater than zero." elif (( var < 0 )); then echo "$var is less than zero." else echo "$var is zero." fi } count
Save and close the file.
In the above script, the
count
function imports an argument from the user input using theread
command when the script calls the function and assigns it to the localvar
variable . Theif-elif
conditional statement evaluates if thevar
value is greater or less than zero to output a message using theecho
command.Run the script using Bash.
console$ bash if-elif-in-function.sh
Enter a value such as
1
and verify the script's output:1 1 is greater than zero.
Create a new
case-out-function.sh
script.console$ nano case-out-function.sh
Add the following contents to the
case-out-function.sh
file.bash#!/bin/bash function help_page() { echo "Available options:" echo "1. apache" echo "2. mysql" echo "3. php" echo "Please enter a valid command or enter 'help' to view this page" } read -p "Enter a command (apache, mysql, php or help): " user_input case "$user_input" in apache) echo "Use dnf install httpd to install the Apache Web server..." ;; mysql) echo "Use dnf install mysql to install the MySQL database..." ;; php) echo "Use dnf install php to install PHP..." ;; help | -h) help_page ;; *) help_page ;; esac
Save and close the file.
In the above code, the script calls the
help_page
function in acase
conditional statement when the user input fails to match any pattern to display the help information page.Run the script using Bash.
console$ bash case-out-function.sh
Enter
help
when prompted to match the function's pattern to display the help page.Enter a command (apache, mysql, php or help):
Output:
Enter a command (apache, mysql, php or help): help Available options: 1. apache 2. mysql 3. php Please enter a valid command or enter 'help' to view this page
Conclusion
You have used functions in Bash to create reusable commands and run tasks. You can use functions with arrays, loops, expressions, and other elements in Bash to enable code reusability. In addition, you can use functions in Bash to automate scripts or run system administration tasks that require reusable commands.
No comments yet.