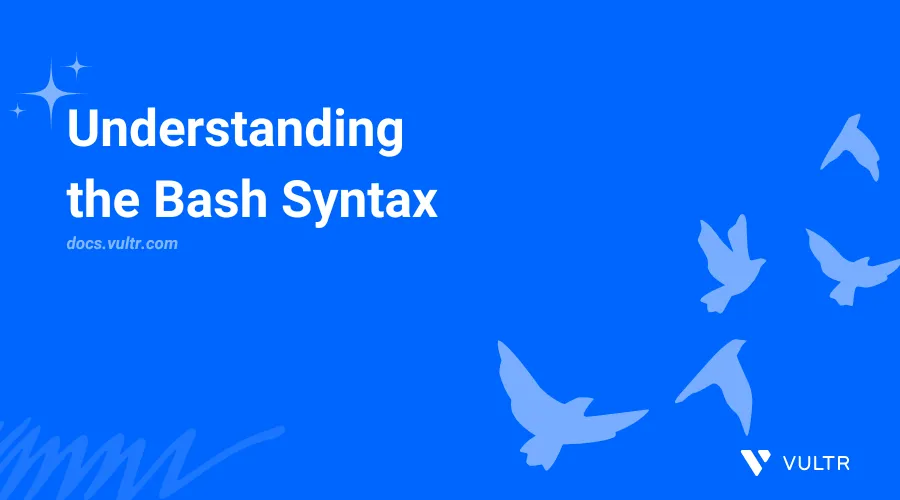
Introduction
Bash syntax defines the rules and structure for writing commands and scripts in the Bash shell. It allows users to efficiently automate tasks, manipulate data, and control system processes using a combination of commands, variables, loops, and conditionals. Bash (Bourne-Again Shell) is the default shell for most Unix and Linux systems, making it an ideal tool for running terminal-based tasks.
This article explains the basic Bash syntax and structure you should use when writing executable scripts.
Bash Script Structure
Bash scripts follow a specific syntax that instructs the system to use the Bash interpreter to execute commands. When writing Bash scripts, you must define the interpreter path at the start of a file before the script contents. The Bash script structure consists of the following elements:
- The Bash Interpreter path
- Commands
- Comments
- Variables
- Conditional statements
Shebang
Hash and bang !#
, also known as shebang, is a syntax that specifies the absolute interpreter path the operating system should use when executing a script. Bash scripts use the following shebang formats.
#!/bin/bash
Or
#!/bin/sh
You can also specify the Bash shell when running a script. For example, script.sh
.
$ bash script.sh
In the above code, the script instructs the operating system to use the Bash interpreter's absolute path to execute the included code. Bash automatically marks the script as Bash script based on the shebang. If you don't specify the shell, the operating system executes the script using the default shell interpreter, which may not be Bash.
Comments
The #
symbol marks all inline script content as comments. The Bash interpreter ignores comments and only processes the following code or commands. The following is a Bash syntax that you should use with comments in a script.
# comment
For example:
#!/bin/bash
# This is a Comment
# Another Comment
echo "Welcome to Bash scripting"
The Bash interpreter executes all contents in the above script and outputs Welcome to Bash scripting
while ignoring all lines that start with the #
symbol.
Bash Script Execution Order
Bash executes script code or commands line by line in ascending order and returns an error code in case of failures. Commands that appear first in a script execute before others in the file. Bash can execute code in a backward format when using interactive constructs such as for
and while
loops.
For example, in the following script, Bash executes the element1
variable before element2
.
#!/bin/bash
element1=value1
element2=value2
Output:
value1
value2
When using constructs, Bash executes code depending on the loop's structure. For example, the following script executes element2
in an array before element1
.
#!/bin/bash
var_array=("element1" "element2")
for i in 1 0; do
echo "${var_array[$i]}"
done
Output:
element2
element1
Run the following command to execute a script using the Bash interpreter. For example, the script.sh
file.
$ bash script.sh
Conclusion
With a solid understanding of bash syntax, you've learned how to create and execute basic Bash scripts. By using the shebang #!/bin/bash
, you've ensured the system runs your scripts in the correct shell environment. Beyond this, more advanced features like conditional statements, loops, and functions enable powerful data manipulation and automation. Mastering these components allows you to create more complex scripts, making Bash a versatile tool for any Unix or Linux system task.
No comments yet.