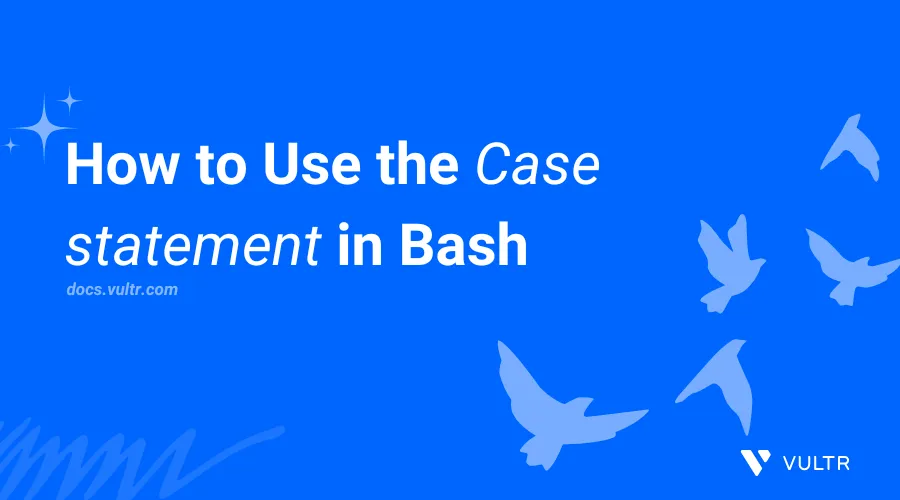
Introduction
The bash case
statement is a conditional structure that lets you test and execute multiple blocks using variable expressions in a script. It is similar to the if..else
statement and uses multiple conditional branches to compare a given variable with a series of patterns and execute a match.
This article explains how to use the case
statement in Bash and execute matching patterns. You will use the case
statement syntax to create example scripts and set up conditions to run specific commands.
Case Statement Syntax and Structure
The case
statement uses an expression to search for a pattern and stops when a condition is met. A default case is executed if the expression does not match any pattern. Below is the basic case
statement syntax.
case EXPRESSION in
PATTERN_1)
Commands or Statements to Execute
;;
*)
Commands or Statements to Execute
;;
esac
A valid statement starts with
case
, the expression to test, andin
that starts the list of patterns to check if the expression meets any of the available conditions. Each pattern is terminated with)
while the included clause terminates with;;
.The wildmask character
*
sets the default pattern to match if no condition is met within the statement. When no pattern is met including the default, the return status is0
or the exit status of the expression. Multiple pattern options are separated using the OR operator|
and the keywordesac
closes a case statement.
Example: Create a Case Statement
The case
statement is compatible with multiple types of expressions such as commands and datasets. In the following example, use case
statement to test for the installation of a particular application in a LAMP (Linux, Apache, MySQL, PHP) stack.
Create a new bash script
LAMP.sh
using your a text editor such as Vim.console$ vim LAMP.sh
Add the following code to the file.
bash#!/bin/bash echo "Below are the Applications in a LAMP Stack, please make a selection: 1. Apache 2. MySQL 3. PHP 4. Install All" echo "Enter the application to view installation command (Apache, MySQL, PHP, or All):" read app case $app in Apache) echo "To install Apache, run the following command:" echo "sudo apt-get install apache2" ;; MySQL) echo "To install a MySQL database server, run the following command:" echo "sudo apt-get install mysql-server" ;; PHP) echo "To install PHP, run the following command:" echo "sudo apt-get install php libapache2-mod-php php-mysql" ;; All) echo "Run the following command to install the entire LAMP stack:" echo "sudo apt-get install apache2 mysql-server php libapache2-mod-php php-mysql" ;; *) echo "Ooops! Invalid selection. Install the entire LAMP stack with the following command:" echo "sudo apt-get install apache2 mysql-server php libapache2-mod-php php-mysql" ;; esac
Save and close the file.
The above Bash script displays a list of LAMP stack applications and reads the user input to store a user's selection in a new
app
variable. Thecase
statement compares the variable data with all defined patterns to display the installation command for the selected application. A joint installation command is listed when the user’s selection does not match any pattern.Run the script using Bash to test the
case
statement.console$ bash LAMP.sh
Output:
Below are the Applications in a LAMP Stack, please make a selection: 1. Apache 2. MySQL 3. PHP 4. Install All
Select a specific result from the list and enter the value. For example,
MySQL
.Enter the application to view installation command (Apache, MySQL, PHP, or All):
The
case
statement executes a pattern that matches theMySQL
condition with the following output before exiting.sudo apt-get install mysql-server
Run the script again.
console$ bash LAMP.sh
Enter an invalid selection such as
Nginx
.Enter the application to view installation command (Apache, MySQL, PHP, or All):
Verify that the default
case
statement is executed with the following output.Ooops! Invalid selection. Install the entire LAMP stack with the following command: sudo apt-get install apache2 mysql-server php libapache2-mod-php php-mysql
Created Nested Case Statements
Nested case
statements enable the execution of multiple levels of conditions if an expression matches a specific pattern. A nested case
statement contains two or more conditions to filter a pattern with a specific expression to handle a combination of results. Use the following example to create nested case
statements to test a LAMP stack selection and install an application based on the user’s input.
Create a new bash script
nested-lamp.sh
.console$ vim nested-lamp.sh
Add the following code to the file.
bash#!/bin/bash echo "Below are the Applications in a LAMP Stack, please make a selection: 1. Apache 2. MySQL 3. PHP 4. Install All" echo "Enter the application to view installation command (Apache, MySQL, PHP, or All):" read app case $app in Apache) echo "sudo apt install apache2 " echo "What would you like to do: 1. Install Apache 2. Update the Server Packages" read selection case $selection in 1) echo "Installing Apache....." sudo apt install apache2 ;; 2) echo "Updating the server packages......" sudo apt update ;; *) echo -e "Ooops! You didn't make a valid selection. Install the entire LAMP stack with the following command: " echo "sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql" exit 1 ;; esac ;; MySQL) echo "sudo apt install mysql-server " echo "What would you like to do: 1. Install MySQL 2. Update the Server Packages " read selection case $selection in 1) echo "Installing MySQL..... " sudo apt install mysql-server ;; 2) echo "Updating the server packages...... " sudo apt update ;; *) echo -e "Ooops! You didn't make a valid selection. Install the entire LAMP stack with the following command: " echo "sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql" exit 1 ;; esac ;; PHP) echo "sudo apt install php libapache2-mod-php php-mysql " echo "What would you like to do: 1. Install PHP and modules 2. Update the Server Packages" read selection case $selection in 1) echo "Installing PHP and modules....." sudo apt install php libapache2-mod-php php-mysql ;; 2) echo "Updating the server packages......" sudo apt update ;; *) echo -e "Ooops! You didn't make a valid selection. Install the entire LAMP stack with the following command: " echo "sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql" exit 1 ;; esac ;; All) echo "sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql " echo "What would you like to do: 1. Install the entire LAMP Stack 2. Update the Server Packages " read selection case $selection in 1) echo "Installing All..... " sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql ;; 2) echo "Updating the server packages......" sudo apt update ;; *) echo -e "Ooops! You didn't make a valid selection. Install the entire LAMP stack with the following command: " echo "sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql" exit 1 ;; esac ;; *) echo "Ooops! Invalid selection. Install the entire LAMP stack with the following command:" echo "sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql" ;; esac
Save and close the file.
In the above example, the user’s original LAMP stack application selection matches a specific pattern that's tested to either install the application or update the server packages without installation. A default pattern is executed in each statement if the user request does not match any pattern.
Run the script.
console$ bash nested-lamp.sh
Output:
Below are the Applications in a LAMP Stack, please make a selection: 1. Apache 2. MySQL 3. PHP 4. Install All Enter the application to view installation command (Apache, MySQL, PHP, or All):
Enter a value such as
Apache
and press Enter to save your selection.Enter the application to view installation command (Apache, MySQL, PHP, or All): Apache
Enter an integer value to make a selection and install the application or update the server packages. For example, enter
2
.sudo apt install apache2 What would you like to do: 1. Install Apache 2. Update the Server Packages
Verify that your server updates before the script exits.
Fetched 55.4 kB in 2s (28.1 kB/s) Reading package lists... Done Building dependency tree... Done Reading state information... Done 15 packages can be upgraded. Run 'apt list --upgradable' to see them.
Test the script return code and verify that it’s
zero
which represents a successful execution.console$ echo $?
Output:
0
Run the script again.
console$ bash nested-lamp.sh
Enter a new application value. For example,
PHP
.Enter the application to view installation command (Apache, MySQL, PHP, or All): PHP
Enter an invalid integer value such as
5
when prompted to install or update the server.What would you like to do: 1. Install PHP and modules 2. Update the Server Packages 5
Verify that the default pattern executes when the value does not match any condition similar to the following output.
Ooops! You didn't make a valid selection. Install the entire LAMP stack with the following command: sudo apt install apache2 mysql-server php libapache2-mod-php php-mysql
Test the script return code and verify that it's
1
meaning an error occurred due to an invalid selection.console$ echo $?
Output:
1
Conclusion
You have used the case
statement in Bash to enables the execution of specific commands when an expression matches any of the available patterns. The case
statement is efficient, uses a default case when a condition does not meet any pattern, and improves your script readability as compared to other conditional statements such as if..else
.
No comments yet.