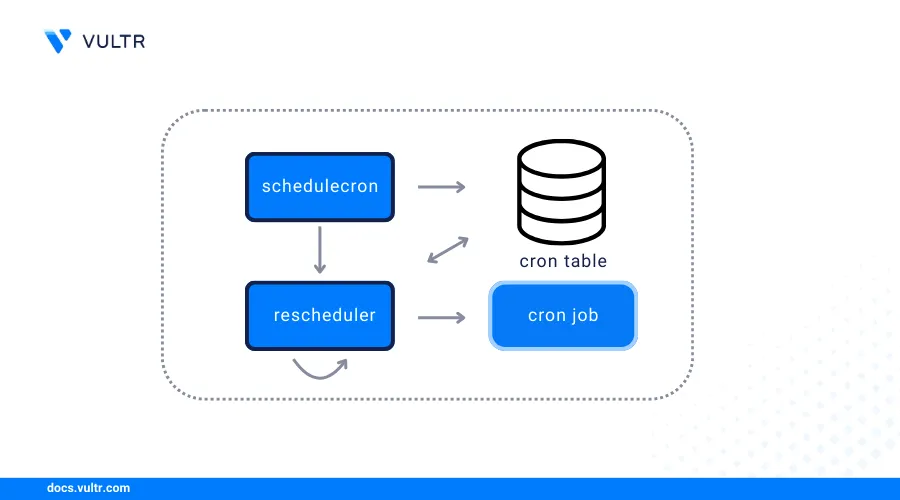
Laravel includes a built-in task scheduling system that simplifies managing recurring tasks. You can use Laravel cron jobs to schedule tasks with multiple PHP syntax elements directly within your Laravel application instead of manually adding entries to the system crontab. The Laravel task scheduler supports flexible frequencies, timezones, output management, and advanced options, allowing you to automate cleanups, generate reports, and send email notifications within your Laravel application.
This article explains how to configure Laravel cron jobs. You will set up the Laravel task scheduler, create custom commands, and a cron job to automatically trigger scheduled tasks in your Laravel project.
Prerequisites
Before you begin, you need to:
- Have access to an existing instance such as Ubuntu 24.04 with an active Laravel project.
- Login to the instance as a non-root user with sudo privileges.
Schedule Tasks in Laravel
Tasks are scheduled operations that execute automatically at defined intervals in Laravel. Tasks include artisan commands you define to run on a schedule in a Laravel application. Follow the steps below to define a new scheduled command, and verify its execution in Laravel.
Navigate to your Laravel project directory such as
/var/www/laravelapp
.console$ cd /var/www/laravelapp
Long list the directory to verify the group ownership details.
console$ ls -l
Your output should be similar to the one below.
drwxr-xr-x 6 www-data www-data 4096 Apr 23 20:57 app/ -rwxr-xr-x 1 www-data www-data 425 Apr 23 20:57 artisan* drwxr-xr-x 3 www-data www-data 4096 Apr 23 20:57 bootstrap/ -rw-r--r-- 1 www-data www-data 2419 Apr 23 20:57 composer.json -rw-r--r-- 1 www-data www-data 297315 Apr 23 20:57 composer.lock drwxr-xr-x 2 www-data www-data 4096 Apr 23 20:57 config/ drwxr-xr-x 5 www-data www-data 4096 Apr 23 20:57 database/ -rw-r--r-- 1 www-data www-data 258 Apr 23 20:57 .editorconfig -rw-r--r-- 1 www-data www-data 1161 Apr 23 20:57 .env -rw-r--r-- 1 www-data www-data 1084 Apr 23 20:57 .env.example -rw-r--r-- 1 www-data www-data 186 Apr 23 20:57 .gitattributes -rw-r--r-- 1 www-data www-data 286 Apr 23 20:57 .gitignore -rw-r--r-- 1 www-data www-data 354 Apr 23 20:57 package.json -rw-r--r-- 1 www-data www-data 1173 Apr 23 20:57 phpunit.xml drwxr-xr-x 2 www-data www-data 4096 Apr 23 20:57 public/ -rw-r--r-- 1 www-data www-data 3932 Apr 23 20:57 README.md drwxr-xr-x 5 www-data www-data 4096 Apr 23 20:57 resources/ drwxr-xr-x 2 www-data www-data 4096 Apr 23 20:57 routes/ drwxr-xr-x 5 www-data www-data 4096 Apr 23 20:57 storage/ drwxr-xr-x 4 www-data www-data 4096 Apr 23 20:57 tests/ drwxr-xr-x 40 www-data www-data 4096 Apr 23 20:57 vendor/ -rw-r--r-- 1 www-data www-data 331 Apr 23 20:57 vite.config.js
The
www-data
group owns the/var/www/laravelapp
directory, allowing any user in the group to access files in the Laravel project directory.Verify the current user you are logged in as.
console$ whoami
Your output should be similar to the one below.
linuxuser
Add the user to the
www-data
group to enable access to the Laravel project directory. Replacelinuxuser
with your actual username.console$ sudo usermod -a -G linuxuser www-data
Grant the user ownership privileges to the
/var/www/laravelapp
directory.console$ sudo chown -R linuxuser:www-data /var/www/laravelapp
Print your working directory and verify that it's the root Laravel project directory.
console$ pwd
Output:
/var/www/laravelapp
Back up the
routes/console.php
file.console$ mv routes/console.php routes/console.php.ORIG
Create a new
routes/console.php
file using a text editor such asnano
.console$ nano routes/console.php
Add the following code to the
routes/console.php
file to define a new scheduled task.php<?php use Illuminate\Support\Facades\Schedule; Schedule::call(function () { \Log::info('This is a scheduled task running at: ' . now()); return 0; })->everySecond(); ?>
Save and close the file.
The above task configuration logs the date and time at every second to the Laravel log file.
Trigger the Laravel scheduler to verify that the task is active.
console$ php artisan schedule:run
Your output should be similar to the one below.
2025-04-14 20:44:07 Running [Callback] ......................... 7.64ms DONE 2025-04-14 20:44:08 Running [Callback] ......................... 0.32ms DONE 2025-04-14 20:44:09 Running [Callback] ......................... 0.34ms DONE 2025-04-14 20:44:10 Running [Callback] ......................... 0.35ms DONE
The scheduled task is active and running based on the above log output.
Press Ctrl + C to stop the Laravel task.
Create Reusable Laravel Commands
Laravel Artisan commands allow you to define reusable, custom CLI commands to encapsulate complex or repetitive tasks. Laravel commands can be scheduled or triggered manually using the artisan
command-line tool. Follow the steps below to create and test a custom Artisan command in Laravel.
Create a new
CacheClearCommand
Artisan command.console$ php artisan make:command CacheClearCommand
The above command generates a new file in the
app/Console/Commands/
directory.Open the generated
CacheClearCommand.php
file using a text editor such asnano
.console$ nano app/Console/Commands/CacheClearCommand.php
Add the following code to the
CacheClearCommand.php
file.php<?php namespace App\Console\Commands; use Illuminate\Console\Command; use Illuminate\Support\Facades\Artisan; class CacheClearCommand extends Command { /** * The name and signature of the console command. * * @var string */ protected $signature = 'app:clear-cache'; /** * The console command description. * * @var string */ protected $description = 'Clear the application cache'; /** * Execute the console command. */ public function handle() { $this->info('Clearing application cache...'); $exitCode = Artisan::call('cache:clear'); if ($exitCode === 0) { $this->info('Application cache cleared successfully!'); \Log::info('Application cache cleared at ' . now()); } else { $this->error('Failed to clear application cache.'); \Log::error('Failed to clear application cache at ' . now()); } return Command::SUCCESS; } } ?>
Save and close the file.
The above application code defines a new
app:clear-cache
command that clears the application cache and logs the result to thelaravel.log
file in thestorage/logs/
directory.Run the following command to clear the application cache.
console$ php artisan app:clear-cache
Output:
Clearing application cache... Application cache cleared successfully!
The above command output confirms that the custom Artisan command is working correctly.
Configure Laravel Cron Jobs To Schedule Commands
The Laravel task scheduling system allows you to automate command executions at defined intervals with a user-friendly syntax. Follow the steps below to schedule the app:clear-cache
command you created earlier to run every two seconds.
Back up the
routes/console.php
file again.console$ mv routes/console.php routes/console.php.bak
Create the
routes/console.php
file again.console$ nano routes/console.php
Add the following code to the
routes/console.php
file.php<?php use Illuminate\Support\Facades\Schedule; Schedule::command('app:clear-cache')->everyTwoSeconds(); ?>
Save and close the file.
The above code schedules the
app:clear-cache
command to run every two seconds. The schedule will only run when theschedule:run
command executes.
Supported Laravel Schedule Frequency Options
Laravel offers several methods to define the frequency and behavior of scheduled tasks. Below are the commonly used scheduling options you can add to your routes/console.php
file to run tasks.
Monthly:
phpSchedule::command('app:clear-cache')->monthly();
The above schedule runs the
app:clear-cache
command once every month.Daily at a specific time:
phpSchedule::command('app:clear-cache')->dailyAt('08:00');
Runs the task daily at a specific time, such as 8:00 AM.
Daily:
phpSchedule::command('app:clear-cache')->daily();
Runs the task once every day at midnight.
Weekly on a specific day and time:
phpSchedule::command('app:clear-cache')->weeklyOn(1, '8:00');
Runs the task on a specific day each week at a set time. Based on the above example, the task runs every Monday at 8:00 AM.
Every hour:
phpSchedule::command('app:clear-cache')->hourly();
Runs the task once every hour.
Every five minutes:
phpSchedule::command('app:clear-cache')->everyFiveMinutes();
Runs the task every 5 minutes.
Every minute:
phpSchedule::command('app:clear-cache')->everyMinute();
Runs the task once every minute.
Using a custom cron expression:
phpSchedule::command('app:clear-cache')->cron('*/5 * * * 1-5');
Runs the task once every 5 minutes from Monday to Friday.
Log the output to a file:
phpSchedule::command('app:clear-cache') ->daily() ->appendOutputTo(storage_path('logs/cleanup.log'));
Runs the task and appends the output to a log file.
Email task output:
phpSchedule::command('app:clear-cache') ->daily() ->emailOutputTo('admin@example.com');
Sends the task's output to an email such as
admin@example.com
.Email only on failure:
phpSchedule::command('app:clear-cache') ->daily() ->emailOutputOnFailure('admin@example.com');
Sends an email if the task fails.
Advanced Scheduling Methods
Laravel provides several advanced scheduling methods to enhance the functionality of scheduled tasks. These methods allow you to customize the behavior of each scheduled task to meet your project requirements. The supported advanced Laravel scheduling methods include the following.
Prevent task overlaps when a task runs longer than expected.
phpSchedule::command('app:clear-cache')->daily()->withoutOverlapping();
Use
withoutOverlapping()
to ensure that a task does not overlap with the next scheduled instance if it takes longer to complete.Ensure tasks run on only one server in a multi-server environment.
phpSchedule::command('app:clear-cache')->daily()->onOneServer();
Use
onOneServer()
to ensure the task runs on only one server in a multi-server setup.Run the tasks in the background.
phpSchedule::command('app:clear-cache')->daily()->runInBackground();
Use
runInBackground()
to run tasks in the background and avoid blocking other processes.Run the tasks even during application maintenance mode.
phpSchedule::command('app:clear-cache')->daily()->evenInMaintenanceMode();
Use
evenInMaintenanceMode()
to run tasks even when the application is in maintenance mode.Run tasks only when certain conditions are met.
phpSchedule::command('app:clear-cache') ->daily() ->when(function () { return \App\Models\User::count() > 100; });
Use
when()
to run tasks based on specific conditions, such as the number of users in the application.Specify time boundaries for when tasks can run.
phpSchedule::command('app:clear-cache') ->hourly() ->between('8:00', '17:00');
Use
between()
to specify a time range in which the task should run.Handle task success and failure events.
phpSchedule::command('app:clear-cache') ->daily() ->onSuccess(function () { \Log::info('Cache cleared successfully'); }) ->onFailure(function () { \Notification::route('mail', 'admin@example.com') ->notify(new \App\Notifications\TaskFailed('Cache Clear')); });
Use
onSuccess()
andonFailure()
to define actions based on the task's outcome.
Automate Laravel Cron Jobs with Cron
You can automate Laravel cron jobs with cron by creating a new task for the Laravel scheduler. Follow the steps below to automate Laravel cron jobs by modifying the system crontab file.
Open the crontab editor.
console$ crontab -e
Choose your preferred text editor such as
nano
when prompted.Add the following cron entry to the file to run the Laravel scheduler every minute.
ini* * * * * cd /var/www/laravelapp && php artisan schedule:run >> /dev/null 2>&1
The above cron task runs the scheduler at every minute within the Laravel project directory. The
php artisan schedule:run
command starts the Laravel scheduler, and the output is redirected to/dev/null
to run the cron job silently without displaying any output.Run the following command to list all cron jobs scheduled for your user and verify that the Laravel task is available.
console$ crontab -l
Your output should be similar to the one below:
# m h dom mon dow command * * * * * cd /var/www/laravelapp && php artisan schedule:run >> /dev/null 2>&1
Test and Validate Laravel Cron Jobs
Follow the steps below to verify that your scheduled tasks appear in the scheduler list and the automated cron job is working correctly.
Print your working directory and verify that it's the root Laravel project directory.
console$ pwd
Output:
/var/www/laravelapp
List all scheduled tasks in your Laravel project.
console$ php artisan schedule:list
Your output should be similar to the one below.
* * * * * php artisan app:clear-cache ....................................................................... Has Mutex › Next Due: 58 seconds from now
The above output verifies the scheduled time, command, and status of each task.
Monitor the Laravel log file and verify that the scheduled tasks are active.
console$ tail -f /var/www/laravelapp/storage/logs/laravel.log
You output should be similar to the one below if the cron job is actively running in your project.
[2025-04-14 20:14:00] local.INFO: Application cache cleared at 2025-04-14 20:14:00 [2025-04-14 20:15:00] local.INFO: Application cache cleared at 2025-04-14 20:15:00 [2025-04-14 20:16:00] local.INFO: Application cache cleared at 2025-04-14 20:16:00 ......................
The timestamps in the above output verify that the scheduled task is running as expected.
Press Ctrl+C to stop the log file output.
Conclusion
You have configured Laravel cron jobs using the built-in task scheduler and automated tasks to run at specific intervals. You can automate recurring tasks in Laravel and efficiently manage the scheduled tasks for execution at a specific time. For more information and advanced scheduling tasks in Laravel, visit the Laravel documentation.
No comments yet.