How to Create and Use Bash Alias
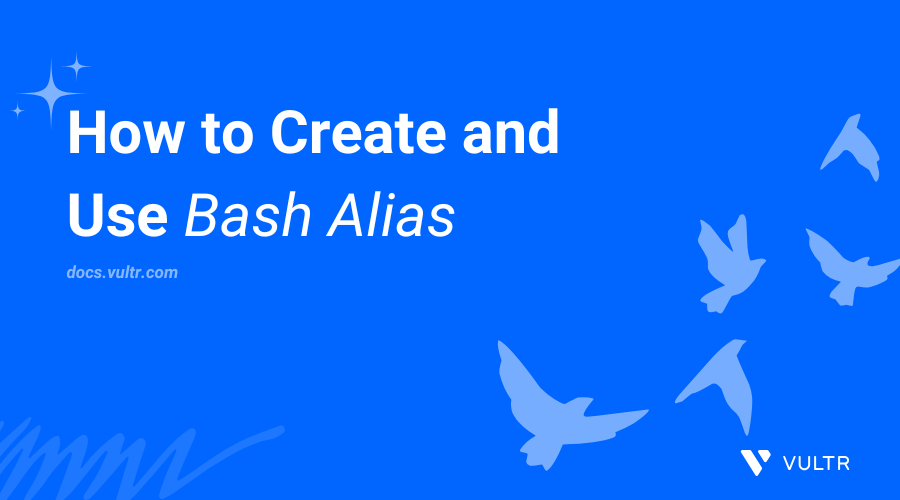
Introduction
A Bash alias is a shortcut for substituting long commands with shorter commands to save time and avoid repetitive typing. In a shell environment, you should store Bash aliases in the .bashrc
file under the user's home directory.
This article explains how to create and use Bash aliases.
The Bash Alias Syntax
The following is a basic Bash alias syntax:
alias alias-name="command"
In the above command:
alias
: Creates a new alias command.alias-name
: Specifies the alias name.command
: Sets the command that you want to substitute. Enclose the command using single or double quotes if the command includes spaces or special characters to prevent shell interpretation.
Create Bash Aliases
Follow the steps below to create and use Bash aliases in a shell environment.
Create a sample alias application, such as
hello-world
that runs theecho
command.console$ alias hello-world='echo "Hello, World! Greetings from Vultr."'
Run the
hello-world
command to display the result.console$ hello-world
Output:
Hello, World! Greetings from Vultr.
Create another
list-files
alias to substitute thels
command.console$ alias list-files='ls'
Run the
list-files
command.console$ list-files
Verify that the command lists all files in your working directory.
Create Permanent Bash Aliases
Bash aliases are temporary in a shell environment unless you save them in the .bashrc
file. Follow the steps below to make the Bash aliases permanent.
Switch to your user's home directory.
console$ cd
Create a new
myaliases
file using a text editor likenano
.console$ nano myaliases
Add the following directives to the
myaliases
file.bashalias hello-world='echo' alias list-files='ls' alias newfile='touch'
Save and close the file.
The
hello-world
,list-files
, andnewfile
aliases in the above configuration execute theecho
,ls
, andtouch
commands respectively.Open the
.bashrc
file.console$ nano .bashrc
Add the following directives at the end of the file.
bashif [ -f myaliases ]; then . myaliases fi
Save and close the file.
The above
if-then
statement finds themyaliases
file in the user's home directory and runs it to load all aliases in the.bashrc
file.Apply the
.bashrc
changes to your shell environment.console$ source .bashrc
Run the
newfile
alias command and specifysample-file
as an argument to create a new file.console$ newfile sample-file
List files using the
list-file
alias in your working directory and verify thatsample-file
is available.console$ list-files
Output:
... sample-file ....
Run the
hello-world
alias and specifyGreetings from Vultr
as an option.console$ hello-world Greetings from Vultr
Output:
Greetings from Vultr
Use Bash Aliases with Functions and Arguments
You can combine aliases with command arguments and functions in Bash scripts to perform specific tasks, such as creating and managing files. Follow the steps below.
Create a new
bashalias.sh
script.console$ nano bashalias.sh
Add the following contents to the
bashalias.sh
file.bash#!/bin/bash myfunction () { mkdir -p "$1" && cd "$1" touch hello_bash } myfunction $1 echo "New Directory and File Created."
Save and close the file.
In the above script the
myfunction
function works as an alias for themkdir
,cd
, andtouch
commands to create a new directory. The function then switches to the directory and creates a newhello_bash
file. You can convertmyfunction
to a global alias command by appending it to the.bashrc
file.Run the script using Bash and specify
test_directory
as the command option.console$ bash bashalias.sh test_directory
List the files in the
test_directory
.console$ ls test_directory/
Output:
hello_world
Use Bash Alias in Bash Script
You can use Bash aliases in scripts to shorten long commands and allow code reusability with other Bash features such as conditional statements and loops. Follow the steps below to use aliases in a Bash script to validate and run specific tasks.
Create a new
advanced-alias.sh
script.console$ nano advanced-alias.sh
Add the following contents to the
advanced-alias.sh
file.bash#!/bin/bash shopt -s expand_aliases alias sysinfo='uname -a && lscpu && free -h' alias lamp='sudo apt update && sudo apt install apache mysql php' alias lemp='sudo apt update && sudo apt install nginx mysql php' alias mean='sudo apt update && sudo apt install -y mongodb npm && sudo npm install -g @angular/cli && sudo npm' echo "Select an option:" echo "1. Show System Information" echo "2. Install LAMP" echo "3. Install LEMP" echo "4. Install MEAN" read -p "Enter your selection (1-4): " selection if [ "$selection" -eq 1 ]; then echo -e "\nGathering system information..." sysinfo elif [ "$selection" -eq 2 ]; then echo -e "\n Installing the LAMP Stack" lamp elif [ "$selection" -eq 3 ]; then echo -e "\n Installing LEMP" lemp elif [ "$selection" -eq 4 ]; then echo -e "\nInstalling MEAN:" mean else echo "Invalid selection. Please select a valid option." exit 1 fi
Save and close the file.
The
shopt -s expand_aliases
command in the above script enables alias expansion while thelamp
,lemp
, andmean
Bash aliases shorten the full installation commands to install specific applications. Theselection
variable stores the user input and uses theif-elif
conditional statement to run the Bash alias commands.Run the script using Bash.
console$ bash advanced-alias.sh
Enter a selection such as
2
to run thelamp
alias command.Select an option: 1. Show System Information 2. Install LAMP 3. Install LEMP 4. Install MEAN Enter your selection (1-4): 2
Output:
Installing the LAMP Stack .................................
Conclusion
You have used aliases in a Bash shell environment to create reusable commands. The bash alias command allows you to run multiple commands, utilizing advanced Bash features such as conditional statements and loops to perform specific tasks.