How to Use Bash Until Loop
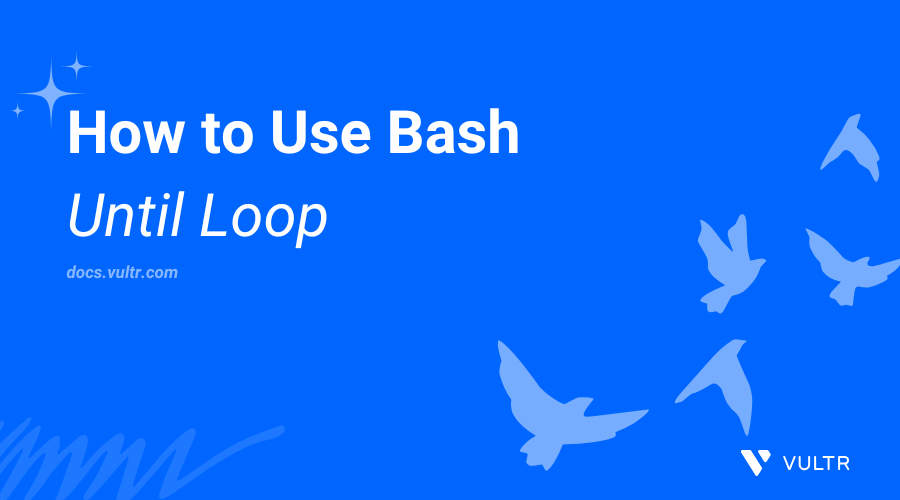
Introduction
Loops are fundamental constructs in Bash that run a series of commands multiple times until a specific condition is met. A Bash Until
Loop continuously executes a block of commands while a specific condition evaluates to false
and stops when the condition evaluates to true
. The until
loops are the opposite of while
loops. The Bash while
loop runs when a condition is true and terminates when a condition returns false
.
This article explains how to use the Bash until
Loop in scripts.
The Bash Until Loop Syntax
The following is a basic until
loop syntax in Bash.
until [CONDITION]
do
# Commands
done
In the above Bash until
loop syntax:
until
: Creates a newuntil
loop.condition
: Specifies the condition to evaluate and run the commands in the loop's body if the condition isfalse
.do
: Specifies the start of the loop's body and runs the commands while the condition evaluates tofalse
.done
: Ends the loop.
Create until
Loops
The until
loops work in Bash shell environment and scripts. You can use inline until
loops to evaluate conditions and perform tasks in a shell or create until
loops to run multiple commands in a script. Follow the steps below.
Create a new inline
until
loop to evaluate if0
is greater than3
and print the result after incrementing the value.console$ count=0; until [ $count -ge 3 ]; do echo "Counter: $count"; ((count++)); done
Output:
Counter: 0 Counter: 1 Counter: 2
Create a new
until.sh
script using a text editor likevim
.console$ vim until.sh
Add the following content to the
until.sh
file.bash#!/bin/bash count=1 until [ "$count" -gt 10 ]; do echo "The current count is: $count" ((count++)) done
Save and close the file.
In the above script, the
until
loop continuously displays the result of thecount
variable when the condition evaluates tofalse
until the value reaches10
. Thecount++
command increments the variable in each loop cycle.Run the script using Bash.
console$ bash until.sh
Output:
The current count is: 1 The current count is: 2 The current count is: 3 The current count is: 4 The current count is: 5 The current count is: 6 The current count is: 7 The current count is: 8 The current count is: 9 The current count is: 10
Infinite until
Loops
An Infinite until
loop continuously runs a specific block of commands unless a user stops the action or if the loop encounters a break
statement. You can create infinite until
loops using constant conditions. Infinite loops are useful when viewing real-time file updates such as logs. Follow the steps below to create an infinite until
loop.
Create a new
infinite.sh
script.console$ vim infinite.sh
Add the following contents to the
infinite.sh
file.bash#!/bin/bash until false; do tail -n 10 /var/log/syslog echo "Press Ctrl + C to stop the loop" sleep 3 done
Save and close the file.
In the above script, the
until
loop continuously runs and displays the last lines in the/var/log/syslog
file using thetail
command and waits3
seconds between the updates. The infinite loop monitors the log file and stops if the user presses Ctrl + C.Run the script using Bash.
console$ bash infinite.sh
Output:
2024-10-16T00:00:17.106598+00:00 acs-server systemd[1]: Starting sysstat-collect.service - system activity accounting tool... 2024-10-16T00:00:17.124697+00:00 acs-server systemd[1]: Starting logrotate.service - Rotate log files... 2024-10-16T00:00:17.139593+00:00 acs-server systemd[1]: sysstat-collect.service: Deactivated successfully. 2024-10-16T00:00:17.142083+00:00 acs-server systemd[1]: Finished sysstat-collect.service - system activity accounting tool. 2024-10-16T00:00:17.187803+00:00 acs-server systemd[1]: dpkg-db-backup.service: Deactivated successfully. 2024-10-16T00:00:17.189907+00:00 acs-server systemd[1]: Finished dpkg-db-backup.service - Daily dpkg database backup service. 2024-10-16T00:00:17.218479+00:00 acs-server systemd[1]: logrotate.service: Deactivated successfully. 2024-10-16T00:00:17.219024+00:00 acs-server systemd[1]: Finished logrotate.service - Rotate log files. Press Ctrl + C to stop the loop
Create Nested until
Loops
Nested until
loops execute commands using multiple levels of conditions to perform a specific task. Such loops use the following syntax to run multiple commands at different levels.
until [CONDITION]
do
# Commands
until [CONDITION]
do
# Commands
done
# Commands
done
Follow the steps below to create nested until
loops to form a visual countdown of numbers.
Create a new
nested-until.sh
script.console$ vim nested-until.sh
Add the following contents to the
nested-until.sh
file.bash#!/bin/bash count=15 until [ $count -le 0 ] do asterisks=0 until [ $asterisks -ge $count ] do echo -n "*" ((asterisks++)) done echo " ($count)" ((count--)) done
Save and close the file.
In the above Bash script, the outer Bash
until
loop checks if thecount
condition is less than1
to assign the inneruntil
loop anasterisk
variable to display asterisks that match thecount
variable value. The outer loop displays the currentcount
variable value and decrements it usingcount--
to repeat the loop.Run the script using Bash.
console$ bash nested-until.sh
Output:
*************** (15) ************** (14) ************* (13) ************ (12) *********** (11) ********** (10) ********* (9) ******** (8) ******* (7) ****** (6) ***** (5) **** (4) *** (3) ** (2) * (1)
Use until
Loop break
and continue
Statements
In Bash, break
and continue
statements affect how the until
loop executes. The break
statement stops the loop and the continue
statement instructs the loop to skip specific commands. Follow the steps below to use the break
and continue
statements with until
loops.
Create a new
break.sh
file.console$ vim break.sh
Add the following contents to the
break.sh
file.bash#!/bin/bash count=10 until [ "$count" -lt 1 ]; do echo "Count: $count" if [ "$count" -eq 5 ]; then echo "Count is 5, terminating the loop." break fi ((count--)) done
Save and close the file.
In the above script, the
until
loop uses a conditional statement to check the currentcount
variable value and terminate the loop using thebreak
statement when thecount
variable evaluates to5
.Run the script using Bash.
console$ bash break.sh
Output:
Count: 10 Count: 9 Count: 8 Count: 7 Count: 6 Count: 5 Count is 5, terminating the loop.
Create a new
continue.sh
script.console$ vim continue.sh
Add the following contents to the
continue.sh
file.bash#!/bin/bash count=10 until [ "$count" -lt 1 ]; do if [ "$count" -eq 5 ]; then echo "Count is 5, skipping the iteration." ((count--)) continue fi echo "Count: $count" ((count--)) done
Save and close the file.
In the above Bash script, the
until
loop uses a conditionalif
statement to test thecount
variable's value. The loop skips the current iteration when thecount
value evaluates to5
using thecontinue
statement.Run the script.
console$ bash continue.sh
Output:
Count: 10 Count: 9 Count: 8 Count: 7 Count: 6 Count is 5, skipping the iteration. Count: 4 Count: 3 Count: 2 Count: 1
Use until
Loops with Conditional Statements
You can use the until
loops with advanced conditional statements to execute a specific set of commands. These Bash conditional statements include if,
, if-else
,case
, and elif
. Follow the steps below to create an until
loop and use an if-else
conditional statement to validate the user input.
Create a new
until-if.sh
script.console$ vim until-if.sh
Add the following contents to the
until-if.sh
file.bash#!/bin/bash user_password="admin123" until [ "$user_input" = "$user_password" ]; do read -p "Enter password: " user_input if [ "$user_input" = "$user_password" ]; then echo "Access granted!" else echo "Incorrect password, try again with the correct password." fi done
Save and close the file.
In the above script, the
until
loop uses theread
command to prompt for user input and continuously runs theif-else
statement until the condition evaluates totrue
and stops the loop.Run the script using Bash.
console$ bash until-if.sh
Enter a wrong password like
11
to test theif-else
statement.Output:
Enter password: 11 Incorrect password, try again with the correct password. Enter password:
Combine until
Loops with While Loops
The until
loops continuously run until a condition evaluates to true
but while
loops continuously run until a condition evaluates to false
. Combining Bash until
and while
loops allows you to validate, control, and execute specific commands based on certain conditions. Follow the steps below to use Bash until
loop with while
loops to validate user input.
Create a new
until-while.sh
file.console$ vim until-while.sh
Add the following contents to the
until-while.sh
file.bash#!/bin/bash user_password="admin123" user_input="" attempts=1 maximum_attempts=5 until [ "$attempts" -gt "$maximum_attempts" ]; do echo "You have $attempts of $maximum_attempts attempts" while [ "$user_input" != "$user_password" ]; do read -p "Enter the password: " user_input if [ "$user_input" == "$user_password" ]; then echo "Access granted!" exit 0 else echo "Incorrect password, try again with the correct password.." break fi done ((attempts++)) done if [ "$attempts" -gt "$maximum_attempts" ]; then echo "Maximum password attempts reached. Access denied!" fi
Save and close the file.
In the above script, the outer
until
loop displays user password attempts and runs the innerwhile
loop to capture the user input using theread
command. Theif-else
conditional statement validates the user input and returns a result. The script increments theattempts
variable to run theuntil
loop again if the innerwhile
loop evaluates tofalse
. The loop stops when theattempts
andmaximum_attempts
variables are equal.Run the script using Bash.
console$ bash until-while.sh
Enter wrong passwords when prompted to test the loop functionalities.
Output:
You have 1 of 5 attempts Enter the password: 1 Incorrect password, try again with the correct password.. You have 2 of 5 attempts Enter the password: 12 Incorrect password, try again with the correct password.. You have 3 of 5 attempts Enter the password: e Incorrect password, try again with the correct password.. You have 4 of 5 attempts Enter the password: rr Incorrect password, try again with the correct password.. You have 5 of 5 attempts Enter the password: 55 Incorrect password, try again with the correct password.. Maximum password attempts reached. Access denied!
Run the script again and enter the correct
admin123
user password .You have 1 of 5 attempts Enter the password: admin123 Access granted!
Conclusion
You have created and used the Bash until
loop to continuously run commands if a condition evaluates to false
. The until
loops run commands when a condition is false
and stops when the condition evaluates to true
. Combining until
loops with conditional statements such as if-else
, while
, and for
loops allows advanced iteration control to run complex commands or automate specific tasks in Bash.