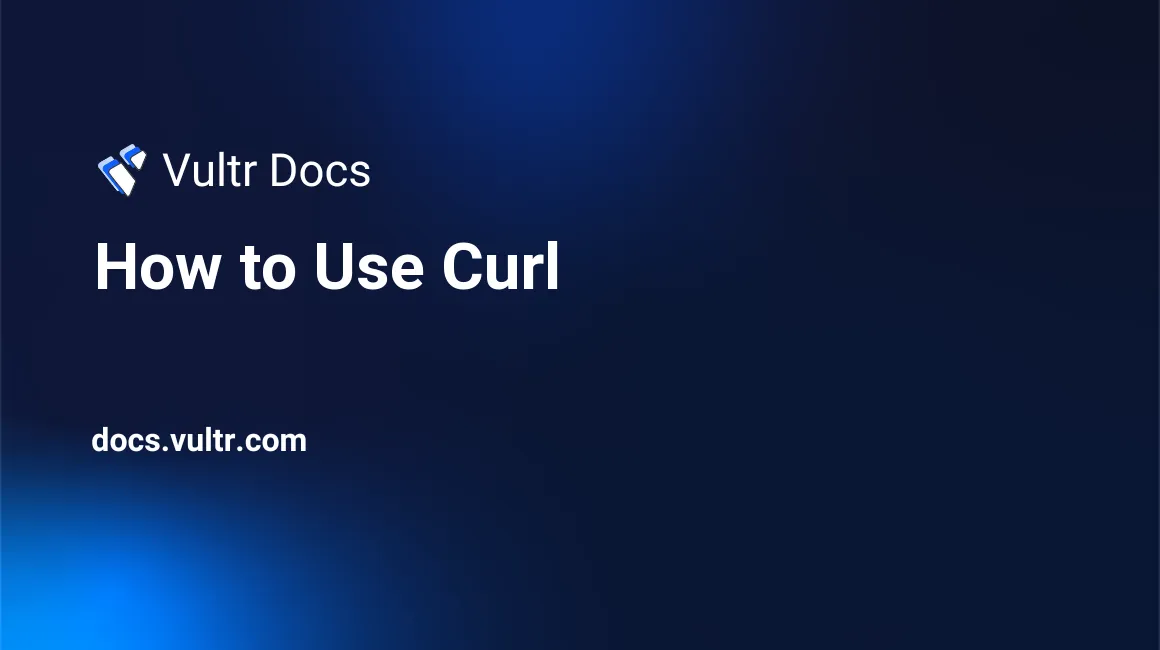
Introduction
Curl is a software project consisting of the libcurl library and the curl command-line tool that supports FTP, FTPS, HTTP, HTTPS, and many other network protocols. Curl is pre-installed in many operating systems, and developers use it extensively to transfer data and make API requests. This guide explains how to use curl and make requests with the tool.
Prerequisites
- A system supported by curl.
- If curl is not installed, install it from the official website.
Using Curl
The basic curl syntax is:
curl [options] [url]
To download a webpage with curl:
$ curl www.example.com/index.html
This downloads the complete webpage and displays the source code to your terminal. If you omit the protocol, curl defaults to https://
.
If you want to use FTP instead:
$ curl ftp://www.example.com/index.html
To save the contents of your download to a file, add the `-o' option followed by the filename:
$ curl -o filename.txt www.example.com/index.html
This downloads the webpage https://www.example.com/index.html
to filename.txt
. You can also omit the filename to take the default name from the server:
$ curl -O www.example.com/index.html
If something interrupts your download, you can resume downloads with the -C
option:
$ curl -C -O www.example.com/index.html
Enable verbose mode with the short option -v
or long option --verbose
:
$ curl -v www.google.com
Making HTTP Requests
Curl has more than 200 options for HTTP requests, a few of which include:
Getting Only Response Headers
To get only the headers from a response excluding the body, use the -I
option:
$ curl -I www.example.com
Following A Redirect
Curl doesn't follow redirects unless you add the -L
option:
$ curl -L http://www.example.com
Changing HTTP Method
By default, curl uses the GET method. To change methods, use the -X
option followed by the method you want to use. For example, to use the DELETE method:
$ curl -X DELETE www.example.com?id=4
Adding Extra Headers
To add extra headers, such as setting the User-Agent or Cache-Control field, use the -H
option:
$ curl -H "User-Agent: Mozilla/5.0 (Macintosh)" -H "Cache-Control: no-cache"
Change the user-agent
You can change the user-agent with the --user-agent
option:
$ curl --user-agent "Mozilla/5.0 (Macintosh)" -H "Cache-Control: no-cache"
Post Data
To send data with a POST request, use the `-d' option followed by the data you want to send:
$ curl -d "option1=value1&option2=value2" -X POST https://www.example.com
The Content-Type is automatically set to "application/x-www-form-urlencoded" in the example above. To send JSON data and explicitly set the Content-Type to application/json, use the -H
option to set the Content-Type:
$ curl -X POST -H "Content-Type: application/json" -d '{"option1":"value1", "option2":"value2"}' https://www.example.com
Use Basic Authentication Credentials
If a resource requires basic HTTP authentication, pass the credentials with the -u
option:
$ curl -u login:password www.example.com
Curl automatically converts the login:password pair to a base64-encoded string token and adds the Authorization: Basic <base64 token>
header to the request.
More Information
This guide doesn't cover every curl option. These are only the most popular uses of the tool. For more information, check the man page.
No comments yet.