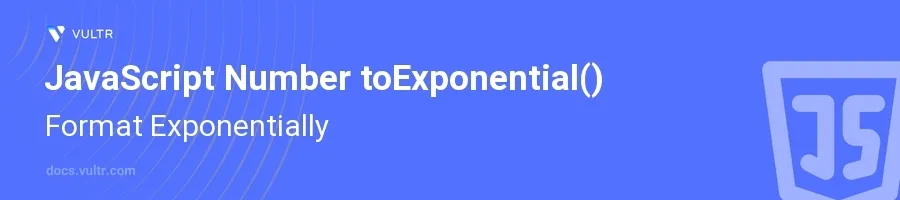
Introduction
The toExponential()
method in JavaScript is a useful tool for formatting a number using exponential (scientific) notation. This method converts numbers into strings that represent the number in exponential form, which can be particularly helpful in handling very large or small numbers in data processing, engineering, or scientific contexts.
In this article, you will learn how to use the toExponential()
method to format numbers exponentially. Explore how this method works with different types of numeric values and discover the impact of specifying fractional digits.
Understanding the toExponential() Method
Basic Usage of toExponential
Start with a basic number.
Call the
toExponential()
method on this number.javascriptlet number = 123456; let exponentialFormat = number.toExponential(); console.log(exponentialFormat);
This code outputs the number
123456
in exponential format, which typically would be something like1.23456e+5
.
Specifying the Number of Digits
Determine the number of digits to appear after the decimal point.
Use the
toExponential()
method with the digit count as an argument.javascriptlet number = 123456; let specificExponentialFormat = number.toExponential(2); console.log(specificExponentialFormat);
Here, the output will be
1.23e+5
, where2
ensures that two digits follow the decimal point.
Handling Small Numbers
Consider a small number that might naturally come in an exponential format.
Apply the
toExponential()
method without specifying a fractional digit count.javascriptlet smallNumber = 0.000123; let smallExponentialFormat = smallNumber.toExponential(); console.log(smallExponentialFormat);
The output will be in scientific notation directly driven by the small magnitude of the number, something like
1.23e-4
.
toExponential with Zero and Negative Numbers
Try the
toExponential()
method on a zero and a negative number.Observe the output to understand how the method handles these cases.
javascriptlet zeroNumber = 0; let negativeNumber = -1500; console.log(zeroNumber.toExponential()); // Output: 0e+0 console.log(negativeNumber.toExponential(3)); // Output: -1.500e+3
The output demonstrates that
toExponential()
correctly formats zero as0e+0
and formats negative numbers with the specified number of decimal places.
Conclusion
The toExponential()
method in JavaScript is a powerful formatting tool that converts numbers into a string representation with exponential notation. This method serves various applications wherein numbers either too large or too small need to be represented in a simplified and readable form. Adjust the number of fractional digits to tailor the precision based on your specific requirements, enhancing both flexibility and precision in data representation and analysis. By applying the techniques discussed, you can manage numeric formats effectively in your JavaScript projects.
No comments yet.