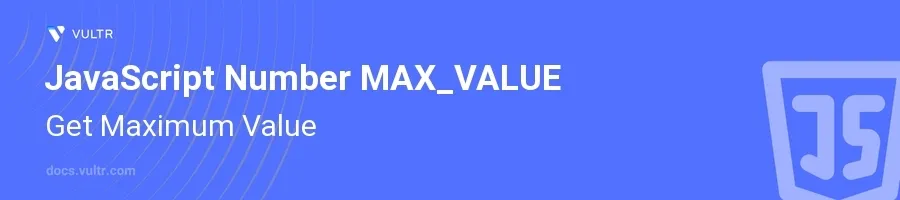
Introduction
The JavaScript Number.MAX_VALUE
property represents the largest positive finite number possible in JavaScript. This property is crucial when you need to compare numbers in tasks that require boundary checks, such as animations, algorithms, or even during validations to prevent overflow errors.
In this article, you will learn how to utilize the Number.MAX_VALUE
property in different practical scenarios. Explore ways to safeguard algorithms against overflow and incorporate boundary constraints efficiently using this numeric constant.
Understanding Number.MAX_VALUE
Recognizing the Value of MAX_VALUE
Log
Number.MAX_VALUE
to see its actual value in your console. This step helps acquaint you with the magnitude of the largest number that JavaScript can handle.javascriptconsole.log(Number.MAX_VALUE);
The output will show the value of
1.7976931348623157e+308
, which is close to the upper limit of what floating point numbers in JavaScript can represent.
Compare Large Numbers
Use
Number.MAX_VALUE
to check if a number exceeds the JavaScript numerical limits. This is particularly useful in functions where numerical input might vary greatly.javascriptfunction checkIfExceedsMax(value) { if (value > Number.MAX_VALUE) { console.log("Value exceeds the maximum limit."); } else { console.log("Value is within the safe limit."); } } checkIfExceedsMax(1.8e+308);
This function tests if a value is greater than
Number.MAX_VALUE
and logs appropriate feedback.
Using Number.MAX_VALUE in Calculations
Performing Boundary-Sensitive Calculations
Implement
Number.MAX_VALUE
as a boundary condition in calculations to prevent overflow, which can lead to Infinity.javascriptfunction calculateProduct(a, b) { if (a > Number.MAX_VALUE / b) { return 'Result would exceed the maximum value'; } return a * b; } console.log(calculateProduct(1e+308, 2));
This function safeguards against potential overflow by checking if the product of two numbers would exceed
Number.MAX_VALUE
.
Resetting to MAX_VALUE on Overflow
Reset numbers to
Number.MAX_VALUE
if an arithmetic operation causes an overflow. This tactic can sometimes be used in simulations or iterative calculations where reaching infinity would cause issues.javascriptfunction secureAddition(a, b) { let result = a + b; if (!isFinite(result)) { return Number.MAX_VALUE; } return result; } console.log(secureAddition(Number.MAX_VALUE, 1000));
Here, addition that overflows defaults back to
Number.MAX_VALUE
, maintaining a finite number range.
Conclusion
Utilizing Number.MAX_VALUE
in JavaScript helps maintain stability and accuracy in your applications by preventing numerical overflow in computations. In addition to being a tool for boundary-checking, it serves as a safeguard in critical calculations, ensuring that operations do not inadvertently exceed the numeric limits of the language. Knowing how to effectively deploy this property ensures more robust and error-resistant code, especially in applications dealing with high-precision calculations or massive numerical data.
No comments yet.