The Definitive Guide to Laravel Performance Optimization
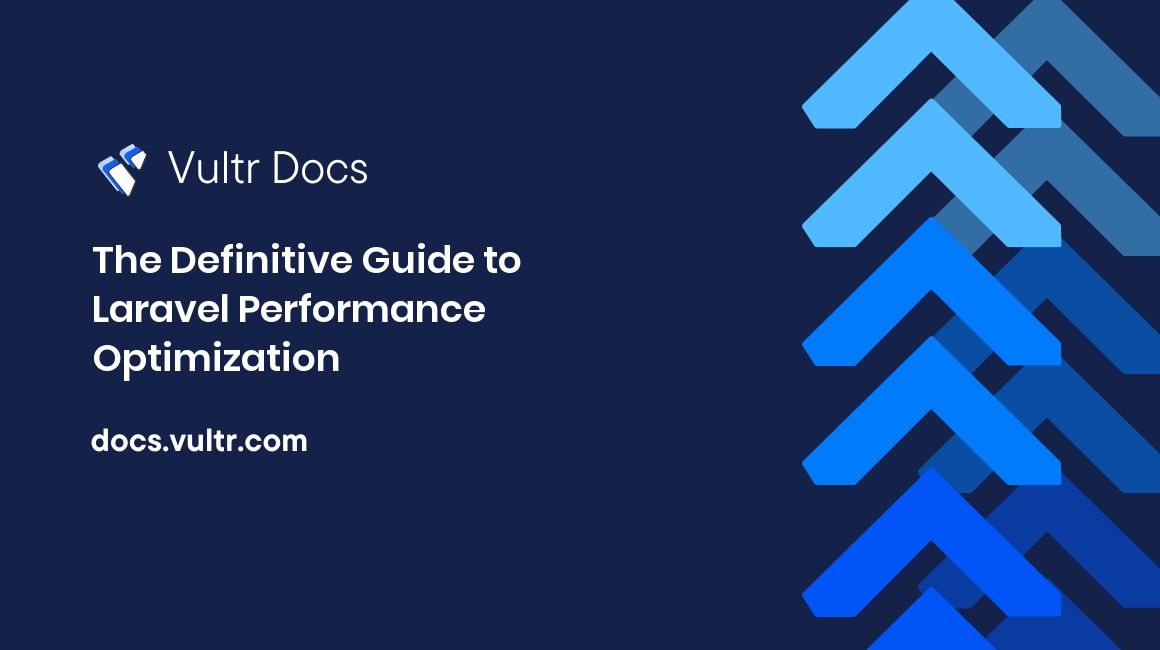
Laravel is one of the most popular PHP frameworks and has garnered a lot of interest in recent years due to its ease of use and the huge library of features it offers. It can be considered a full-stack framework as it integrates well with modern Javascript Frameworks such as Vue and React.
Since it is a full-stack framework, multiple things can affect the performance of Laravel in a production environment.
Why Is Laravel Performance Optimization So Important?
A slow application can lead to a lot of lost revenue in all sectors. In E-commerce, for example, Amazon calculated that a slow page load of just one second could cost it $1.6 billion in sales each year. While this is true, it also means that most people are accustomed to websites loading as soon as they click them. If the website does not load within 400ms according to Google engineers, the user will go to another website, meaning a lost chance for your business to showcase its products and services.
Therefore, optimizing your application's performance is important to give visitors a smooth experience, which could lead to inbound business and sales from interested parties. Here are some practical steps you should consider.
Audit Laravel Application Performance
There are multiple ways how you can audit the performance of a Laravel Application. One good way is to use an Application Performance Monitor, such as Laravel Dusk, which can audit the performance of a Laravel Application and help fine-tune its performance.
Use Eager Loading instead of Lazy Loading
Laravel, by default, uses Lazy loading to retrieve records from the database. Lazy loading is brought about by having extra SQL queries made to the database. This brings the N + 1 query problem, which slows down your application.
Eager loading, on the other hand, means fetching all the data, including related data, in one SQL query. This results in fewer SQL queries which leads to better performance.
For example, you can have a Flight Model and a Ticket Model having a one to Many Relationship where one flight has many tickets available, and a ticket belongs to a flight.
Lazy loading
$tickets = App\Tickets::all();
foreach($tickets as $ticket)
{
$ticket->flight->name;
}
Eager loading
$tickets = App\Tickets::with('flight')->get();
foreach($tickets as $ticket)
{
$ticket->flight->name;
}
Using the with() method can help eager load records improve your Laravel Application performance.
Avoid using Eloquent (Occasionally)
Eloquent ORM is a powerful mapper that makes working with a Database a smooth and fun experience. It does come at a cost as it does take a little bit more time to query the database and prepare the response. This could slow down your application in the case of complex queries. If needed, you could try and write raw SQL queries using the DB:raw() helper for these complex queries, as this can increase the speed and performance of your application.
Cache Assets
Laravel provides a cool way of caching routes, views, events and configurations. Using one command; artisan optimize, you can cache configurations, routes and files
php artisan optimize
Views are cached using the view:cache command.
php artisan view:cache
Note that this can apply if your application is deployed to production. You should not use these commands if you are developing in your local environment because a lot of things will keep changing. You don't want to keep rebuilding your application's cache.
Cache Records
Aside from caching application-specific assets, Laravel allows us to cache database records in memory cache systems such as Redis® and Memcached. This helps reduce the number of queries made to the database and thus increases the application's performance.
Leverage the Power of Queues
Multiple tasks can slow down the performance of a Laravel Application. These tasks include sending emails, generating invoices, and performing backups among others. Using Laravel Queues to offload these tasks can rapidly increase the performance of a Laravel Application.
Laravel support multiple queue drivers such as Amazon SQS, Redis®, Beanstalkd, and even the default database.
Laravel also provides a command to run queued jobs: queue:work
php artisan queue:work
Supervisor can also be used to watch your Laravel queues and restart failed jobs.
Compress Images
Images form a big part of any website. They make any website experience immersive and can be used to display products and services easily.
Images, however, can lead to slow load times because of how large they can get. Compressing images to reduce their size and thus increase the application's performance is necessary. Spatie's package can be used to compress images when they are uploaded and therefore store them as compressed.
Use the Latest Version of PHP
A recent PHP benchmark shows that Laravel is 20.56% faster on PHP 8.1. This makes it a no-brainer to upgrade to the latest PHP version. This will improve your application's performance.
Using PHP Opcache to Increase App Performance
Opcache improves PHP performance by storing precompiled bytes of code in memory, thereby reducing the need to parse PHP scripts on each request.
By enabling it, you are sure that the performance of your Laravel application will increase.
Leveraging the Power of Varnish
Varnish is a web application accelerator. It stores copies of webpages in memory and serves them to the user much faster than the original server can.
Varnish is open-source software, meaning it's free to use and modify and can be used to speed up a Laravel Application and make it more responsive. You can learn how to install and set up Varnish with this guide.
Use CDN for Static Assets
Using a Content Delivery Network(CDN) to load static data such as images will improve the performance of a Laravel Application. This enhances the user experience and content speed as data is loaded from a CDN closest to the user.
Configure Load Balancing
Load Balancing can be a great addition to your application stack in production. It not only negates the problem of a single point of failure but also distributes incoming requests depending on the server weights and algorithm used to set up the load balancer.
This will improve the performance of your Laravel Application.
Using JIT Compiler
PHP is an interpreted language and requires an interpreter to transform code into bytecode. Transforming the source code to bytecode can take a long time leading to a slow application. Using a Just In Time Compiler such as HHVM by Facebook can help reduce the time needed to compile PHP code to bytecode, therefore, increasing the performance of a Laravel Application
Bundle Assets
Laravel bundles assets using Vite and Laravel Mix, with Vite being the preferred build tool. This is because it provides extremely fast development environments and bundles your production application easily.
Laravel merges all your CSS code and Javascript code into one file, making it perform faster as only one file is shipped to the browser for execution.
Minify Assets
CSS files and Javascript files get large as the application's logic increases. This is because Laravel Bundles all the Javascript and CSS code into one file, which can lead to performance issues. These Assets can be compressed, and this can increase the performance of your Laravel Application. You can use the npm command to minify assets.
npm run production
Use Good Hosting
Hosting can be a huge contributor to your Laravel Application's performance. Some hosts have a lot of latency which can affect the performance of your application. Vultr provides some of the best servers with minimal latency, which can help increase the performance of your Laravel Application
Use Lumen for Small Applications
Lumen is a Laravel Micro-framework that provides basic functionalities needed for a particular application. For example, suppose your application only needs Mailing services and Caching and Queues. In that case, it is better to use Lumen than Laravel as it is more performant because of its less boilerplate code and small size.
Optimise Composer Autoload
By default, Laravel scans the whole application structure to determine the classes present. This helps in the development stage as the developer does not need to change the autoload configurations each time a new file is created. Scanning for classes increases the class loading time leading to a slow Application.
To prevent Laravel from scanning the whole filesystem for classes and instead scan for file paths, you can optimize your application using the following command:
composer dump-autoload -o
This will increase the performance of your application.
Reduce Autoloaded Services
When booted, Laravel loads a ton of services. These services are available in the config/app.php file. It is important to know what services are necessary for your application and remove the ones that are not needed by your application.
Remove Unused Packages
Removing Unused Packages can help increase the speed of your Laravel application. This will reduce your application's size and make it easy for process managers such as PHP-FPM to find and process the logic of your application. Packages can be removed using the composer remove command.
composer remove package
Conclusion
Optimizing your Laravel Application's performance can help increase its performance and help reduce load times and bounce rates from users on your site. Following these best practices can help skyrocket your application to the next level leading to more inbound leads, which leads to more inbound sales for your business. Laravel is a powerful framework that can help speed up your development process, and using it for your business can be a huge advantage.