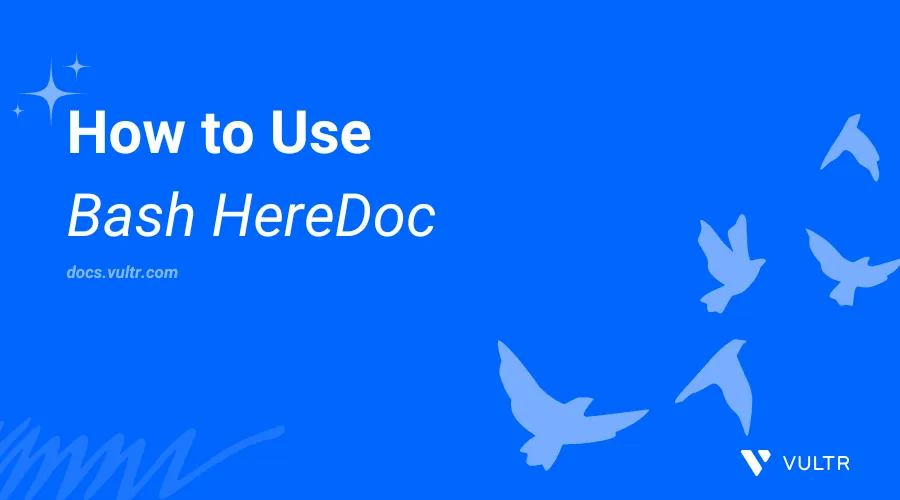
Introduction
Bash HereDoc, also known as Here Document, is an input redirection feature that allows you to enter multiline inputs to a command or a script. HereDoc works with cat
, tee
, variables, and special characters in Bash, making it useful when executing large blocks of code.
This article explains how to use the Bash HereDoc to redirect multiline inputs to commands such as cat
.
The Bash HereDoc Syntax
A HereDoc uses the following syntax in Bash.
[COMMAND] << 'DELIMITER'
# Contents
DELIMITER
In the above command:
COMMAND
: Specifies the command to redirect the HereDoc input to.<<
: Defines the special redirection operator.DELIMITER
: Marks the beginning and the end of a HereDoc. The value can be any string, butEOF
andEND
are commonly used with HereDoc. The beginning and closing delimiters must match to terminate the HereDoc correctly.contents
: Specifies the HereDoc text or input to pass to the command.
The following HereDoc options modify the type of input contents passed to a command for execution.
<<
: Reads the input content until a closing delimiter is detected. All variables and special characters are substituted to the shell before passing the HereDoc to the command.<<-
: Enables indenting input and removing or ignoring all leading tab characters.<< "DELIMITER"
: Treats all HereDoc contents as a single string to interpret variables and escape characters in the input.<< 'DELIMETER'
: Prevents variable expansion and interpretation of escape characters in the input.
Use Here String
A Here string is similar to HereDoc in Bash, but it does not require a delimiter token to pass a string or data as input to a command. A Here string uses the <<<
redirection operator to pass contents to a command without manual input. You can pass variables, special characters, and plain strings similar to any command using the following syntax.
COMMAND <<< # Contents
Create a new Here string that prints your current user.
console$ cat <<< "Your current user is: $(whoami)"
Output:
Your current user is: root
Escape special characters, such as
@
,#
,!
,$
, and^
using single quotes in a Here string to prevent the system from interpreting the characters.console$ cat <<< 'Hello World! Special characters like @,$,% are not interpreted with single quotes ON: !,}'
Output:
Hello World! Special characters like @,$,% are not interpreted with single quotes ON: !,}
Pass contents to a variable using a Here string.
console$ read var1 <<< "Hello World! This Works"
Print the variable to view the contents.
console$ echo $var1
Output:
Hello World! This Works
Bash HereDoc Examples
Follow the steps below to use Bash HereDoc with multiple commands.
Create a HereDoc that prints the working directory, the active user, and the system date.
console$ cat << END Your current user is: $(whoami) Working directory: $PWD System date: $(date) END
Output:
Your current user is: user Working directory: /home/user System date: Wed Oct 09 09:58:14 PM UTC 2024
Create a new
var1
variable that stores the values from a HereDoc.console$ var1=$(cat <<'EOF' Line 1. This is the second line. Just the third line. EOF )
Print the variable.
console$ echo $var1
Output:
Line 1. This is the second line. Just the third line.
Redirect the HereDoc command output to create a new file, such as
doc.txt
.console$ cat << EOF > doc.txt Hello World! EOF
Redirect the output from a HereDoc command and append it to a file.
console$ cat << EOF >> doc.txt This works! EOF
Modify the output of a HereDoc command using
sed
and redirect the output to a file.console$ cat <<'EOF' | sed 's/Hello/Hi/g; s/Works/Functions Well/g' > newfile.txt Hello World! This Works! EOF
Create Configuration Files with Bash HereDoc
You can use Bash HereDoc to write configuration files instead of manually creating the files. This is important in scripts that read specific contents from a configuration file to run specific tasks. You can use a HereDoc to create a file and remove it after performing the task. Follow the steps below to use HereDoc to create new configuration files.
Create a new
configuration.ini
file using HereDoc.console$ cat <<EOF > configuration.ini [Database] host=localhost port=3306 username=admin password=admin123 [Server] host=127.0.0.1 port=8000 EOF
View the contents of the configuration file using the
cat
command.console$ cat configuration.ini
Output:
[Database] host=localhost port=3306 username=admin password=admin123 [Server] host=0.0.0.0 port=8000
Disable Bash Script Contents with HereDoc
You can use comments in Bash to disable specific blocks of code in a script by adding the #
symbol at the beginning of every line to create a comment. HereDoc disables script contents more efficiently using the dummy command :
that does not return any value. Follow the steps below to use Bash HereDoc to disable multiple lines of code in a script.
Create a new
heredoc.sh
script using a text editor likevim
.console$ vim heredoc.sh
Add the following contents to the
heredoc.sh
file.bash#!/bin/bash echo "Hello World! This Works!" : <<'EOF' echo "Hello User! This is disabled!" EOF echo "This runs"
Save the file.
In the above script, all contents between the HereDoc
EOF
delimiters are disabled and do not run in the script.Run the script using Bash.
console$ bash heredoc.sh
Output:
Hello World! This Works! This runs
Use HereDoc with SSH
You can use HereDoc with SSH to run multiple commands on a remote system without an interactive session. SSH works as the command and forwards the HereDoc input that runs when the remote connection establishes.
$ ssh -T user@host.com cat << EOF
Your current user is: $(whoami)
Working directory: $PWD
System date: $(date)
EOF
In the above SSH command, the -T
option disables the interactive shell to input the HereDoc that prints the working directory, the active user, and the remote system's date. Your output should look like the one below when successful.
Your current user is: user
Working directory: /home/user
System date: Wed Oct 09 09:58:14 PM UTC 2024
Use Bash HereDoc with Functions
HereDoc works with functions to process multiline input in Bash scripts. Calling the function name works as the command and the HereDoc redirect the input contents to the function. For example:
Create a new
heredoc-func.sh
script.console$ vim heredoc-func.sh
Add the following contents to the
heredoc-func.sh
file.bash#!/bin/bash my_function() { local var="$1" echo "$var" } my_function "$(cat << 'EOF' Hello World! This Works! EOF )"
Save the file.
In the above script, HereDoc writes multiple messages while calling the function and the
echo
command prints the input values within the function to display the output.Run the script using Bash.
console$ bash heredoc-func.sh
Output:
Hello World! This Works!
Use HereDoc with Custom Delimiters
The HereDoc EOF
and END
delimiters are commonly used in Bash. You can use other delimiters to make files and code more readable. A delimiter can consist of any custom string such as txt
for creating text files, abc
, or xyz
for any other files based on your logic. Follow the steps below to use different delimiters with HereDoc in Bash to write files.
Create a new
var1
variable and set a custom HereDoc delimiter, such assql
.console$ var1=$(cat << 'sql' SELECT * FROM users; sql )
Print the variable.
console$ echo "$var1"
Output:
SELECT * FROM users;
Create a new
config.ini
configuration file from a HereDoc and setcfg
as the delimiter.console$ cat << 'cfg' > config.ini [Database] host=localhost port=3306 username=admin password=admin123 [Server] host=0.0.0.0 port=8000 cfg
Run the following command to use HereDoc with a
php
delimiter to create a new PHP application file.console$ cat << 'php' > index.php echo "Hello World! PHP Works!"; php
Conclusion
You have used Bash HereDoc to create variables and files. Then you've set HereDoc to use custom delimiters. HereDoc is a powerful feature in Bash that allows you to write and structure scripts to execute large blocks of code. You can integrate HereDoc with other Bash features such as loops, arrays, and expressions to perform advanced tasks.
No comments yet.